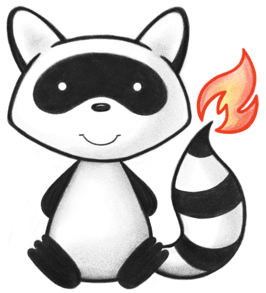
001package org.hl7.fhir.convertors.conv30_40.resources30_40; 002 003import org.hl7.fhir.convertors.VersionConvertorConstants; 004import org.hl7.fhir.convertors.context.ConversionContext30_40; 005import org.hl7.fhir.convertors.conv30_40.datatypes30_40.ContactDetail30_40; 006import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.CodeableConcept30_40; 007import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Coding30_40; 008import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Identifier30_40; 009import org.hl7.fhir.convertors.conv30_40.datatypes30_40.complextypes30_40.Timing30_40; 010import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Boolean30_40; 011import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Code30_40; 012import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Date30_40; 013import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.DateTime30_40; 014import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Integer30_40; 015import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.MarkDown30_40; 016import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.String30_40; 017import org.hl7.fhir.convertors.conv30_40.datatypes30_40.primitivetypes30_40.Uri30_40; 018import org.hl7.fhir.exceptions.FHIRException; 019import org.hl7.fhir.r4.model.BooleanType; 020import org.hl7.fhir.r4.model.ValueSet; 021 022public class ValueSet30_40 { 023 024 public static org.hl7.fhir.dstu3.model.ValueSet.ConceptReferenceComponent convertConceptReferenceComponent(org.hl7.fhir.r4.model.ValueSet.ConceptReferenceComponent src) throws FHIRException { 025 if (src == null) 026 return null; 027 org.hl7.fhir.dstu3.model.ValueSet.ConceptReferenceComponent tgt = new org.hl7.fhir.dstu3.model.ValueSet.ConceptReferenceComponent(); 028 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 029 if (src.hasCode()) 030 tgt.setCodeElement(Code30_40.convertCode(src.getCodeElement())); 031 if (src.hasDisplay()) 032 tgt.setDisplayElement(String30_40.convertString(src.getDisplayElement())); 033 for (org.hl7.fhir.r4.model.ValueSet.ConceptReferenceDesignationComponent t : src.getDesignation()) 034 tgt.addDesignation(convertConceptReferenceDesignationComponent(t)); 035 return tgt; 036 } 037 038 public static org.hl7.fhir.r4.model.ValueSet.ConceptReferenceComponent convertConceptReferenceComponent(org.hl7.fhir.dstu3.model.ValueSet.ConceptReferenceComponent src) throws FHIRException { 039 if (src == null) 040 return null; 041 org.hl7.fhir.r4.model.ValueSet.ConceptReferenceComponent tgt = new org.hl7.fhir.r4.model.ValueSet.ConceptReferenceComponent(); 042 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 043 if (src.hasCode()) 044 tgt.setCodeElement(Code30_40.convertCode(src.getCodeElement())); 045 if (src.hasDisplay()) 046 tgt.setDisplayElement(String30_40.convertString(src.getDisplayElement())); 047 for (org.hl7.fhir.dstu3.model.ValueSet.ConceptReferenceDesignationComponent t : src.getDesignation()) 048 tgt.addDesignation(convertConceptReferenceDesignationComponent(t)); 049 return tgt; 050 } 051 052 public static org.hl7.fhir.dstu3.model.ValueSet.ConceptReferenceDesignationComponent convertConceptReferenceDesignationComponent(org.hl7.fhir.r4.model.ValueSet.ConceptReferenceDesignationComponent src) throws FHIRException { 053 if (src == null) 054 return null; 055 org.hl7.fhir.dstu3.model.ValueSet.ConceptReferenceDesignationComponent tgt = new org.hl7.fhir.dstu3.model.ValueSet.ConceptReferenceDesignationComponent(); 056 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 057 if (src.hasLanguage()) 058 tgt.setLanguageElement(Code30_40.convertCode(src.getLanguageElement())); 059 if (src.hasUse()) 060 tgt.setUse(Coding30_40.convertCoding(src.getUse())); 061 if (src.hasValue()) 062 tgt.setValueElement(String30_40.convertString(src.getValueElement())); 063 return tgt; 064 } 065 066 public static org.hl7.fhir.r4.model.ValueSet.ConceptReferenceDesignationComponent convertConceptReferenceDesignationComponent(org.hl7.fhir.dstu3.model.ValueSet.ConceptReferenceDesignationComponent src) throws FHIRException { 067 if (src == null) 068 return null; 069 org.hl7.fhir.r4.model.ValueSet.ConceptReferenceDesignationComponent tgt = new org.hl7.fhir.r4.model.ValueSet.ConceptReferenceDesignationComponent(); 070 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 071 if (src.hasLanguage()) 072 tgt.setLanguageElement(Code30_40.convertCode(src.getLanguageElement())); 073 if (src.hasUse()) 074 tgt.setUse(Coding30_40.convertCoding(src.getUse())); 075 if (src.hasValue()) 076 tgt.setValueElement(String30_40.convertString(src.getValueElement())); 077 return tgt; 078 } 079 080 public static org.hl7.fhir.dstu3.model.ValueSet.ConceptSetComponent convertConceptSetComponent(org.hl7.fhir.r4.model.ValueSet.ConceptSetComponent src) throws FHIRException { 081 if (src == null) 082 return null; 083 org.hl7.fhir.dstu3.model.ValueSet.ConceptSetComponent tgt = new org.hl7.fhir.dstu3.model.ValueSet.ConceptSetComponent(); 084 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 085 if (src.hasSystem()) 086 tgt.setSystemElement(Uri30_40.convertUri(src.getSystemElement())); 087 if (src.hasVersion()) 088 tgt.setVersionElement(String30_40.convertString(src.getVersionElement())); 089 for (org.hl7.fhir.r4.model.ValueSet.ConceptReferenceComponent t : src.getConcept()) 090 tgt.addConcept(convertConceptReferenceComponent(t)); 091 for (org.hl7.fhir.r4.model.ValueSet.ConceptSetFilterComponent t : src.getFilter()) 092 tgt.addFilter(convertConceptSetFilterComponent(t)); 093 for (org.hl7.fhir.r4.model.UriType t : src.getValueSet()) tgt.addValueSet(t.getValue()); 094 return tgt; 095 } 096 097 public static org.hl7.fhir.r4.model.ValueSet.ConceptSetComponent convertConceptSetComponent(org.hl7.fhir.dstu3.model.ValueSet.ConceptSetComponent src) throws FHIRException { 098 if (src == null) 099 return null; 100 org.hl7.fhir.r4.model.ValueSet.ConceptSetComponent tgt = new org.hl7.fhir.r4.model.ValueSet.ConceptSetComponent(); 101 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 102 if (src.hasSystem()) 103 tgt.setSystemElement(Uri30_40.convertUri(src.getSystemElement())); 104 if (src.hasVersion()) 105 tgt.setVersionElement(String30_40.convertString(src.getVersionElement())); 106 for (org.hl7.fhir.dstu3.model.ValueSet.ConceptReferenceComponent t : src.getConcept()) 107 tgt.addConcept(convertConceptReferenceComponent(t)); 108 for (org.hl7.fhir.dstu3.model.ValueSet.ConceptSetFilterComponent t : src.getFilter()) 109 tgt.addFilter(convertConceptSetFilterComponent(t)); 110 for (org.hl7.fhir.dstu3.model.UriType t : src.getValueSet()) tgt.addValueSet(t.getValue()); 111 return tgt; 112 } 113 114 public static org.hl7.fhir.r4.model.ValueSet.ConceptSetFilterComponent convertConceptSetFilterComponent(org.hl7.fhir.dstu3.model.ValueSet.ConceptSetFilterComponent src) throws FHIRException { 115 if (src == null) 116 return null; 117 org.hl7.fhir.r4.model.ValueSet.ConceptSetFilterComponent tgt = new org.hl7.fhir.r4.model.ValueSet.ConceptSetFilterComponent(); 118 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 119 if (src.hasProperty()) 120 tgt.setPropertyElement(Code30_40.convertCode(src.getPropertyElement())); 121 if (src.hasOp()) 122 tgt.setOpElement(convertFilterOperator(src.getOpElement())); 123 if (src.hasValue()) 124 tgt.setValue(src.getValue()); 125 return tgt; 126 } 127 128 public static org.hl7.fhir.dstu3.model.ValueSet.ConceptSetFilterComponent convertConceptSetFilterComponent(org.hl7.fhir.r4.model.ValueSet.ConceptSetFilterComponent src) throws FHIRException { 129 if (src == null) 130 return null; 131 org.hl7.fhir.dstu3.model.ValueSet.ConceptSetFilterComponent tgt = new org.hl7.fhir.dstu3.model.ValueSet.ConceptSetFilterComponent(); 132 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 133 if (src.hasProperty()) 134 tgt.setPropertyElement(Code30_40.convertCode(src.getPropertyElement())); 135 if (src.hasOp()) 136 tgt.setOpElement(convertFilterOperator(src.getOpElement())); 137 if (src.hasValue()) 138 tgt.setValue(src.getValue()); 139 return tgt; 140 } 141 142 public static org.hl7.fhir.dstu3.model.ValueSet convertValueSet(org.hl7.fhir.r4.model.ValueSet src) throws FHIRException { 143 if (src == null) 144 return null; 145 org.hl7.fhir.dstu3.model.ValueSet tgt = new org.hl7.fhir.dstu3.model.ValueSet(); 146 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 147 if (src.hasUrl()) 148 tgt.setUrlElement(Uri30_40.convertUri(src.getUrlElement())); 149 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 150 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 151 if (src.hasVersion()) 152 tgt.setVersionElement(String30_40.convertString(src.getVersionElement())); 153 if (src.hasName()) 154 tgt.setNameElement(String30_40.convertString(src.getNameElement())); 155 if (src.hasTitle()) 156 tgt.setTitleElement(String30_40.convertString(src.getTitleElement())); 157 if (src.hasStatus()) 158 tgt.setStatusElement(Enumerations30_40.convertPublicationStatus(src.getStatusElement())); 159 if (src.hasExperimental()) 160 tgt.setExperimentalElement(Boolean30_40.convertBoolean(src.getExperimentalElement())); 161 if (src.hasDateElement()) 162 tgt.setDateElement(DateTime30_40.convertDateTime(src.getDateElement())); 163 if (src.hasPublisher()) 164 tgt.setPublisherElement(String30_40.convertString(src.getPublisherElement())); 165 for (org.hl7.fhir.r4.model.ContactDetail t : src.getContact()) 166 tgt.addContact(ContactDetail30_40.convertContactDetail(t)); 167 if (src.hasDescription()) 168 tgt.setDescriptionElement(MarkDown30_40.convertMarkdown(src.getDescriptionElement())); 169 for (org.hl7.fhir.r4.model.UsageContext t : src.getUseContext()) 170 tgt.addUseContext(Timing30_40.convertUsageContext(t)); 171 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getJurisdiction()) 172 tgt.addJurisdiction(CodeableConcept30_40.convertCodeableConcept(t)); 173 if (src.hasImmutable()) 174 tgt.setImmutableElement(Boolean30_40.convertBoolean(src.getImmutableElement())); 175 if (src.hasPurpose()) 176 tgt.setPurposeElement(MarkDown30_40.convertMarkdown(src.getPurposeElement())); 177 if (src.hasCopyright()) 178 tgt.setCopyrightElement(MarkDown30_40.convertMarkdown(src.getCopyrightElement())); 179 if (src.hasExtension(VersionConvertorConstants.EXT_VS_EXTENSIBLE)) 180 tgt.setExtensible(((BooleanType) src.getExtensionByUrl(VersionConvertorConstants.EXT_VS_EXTENSIBLE).getValue()).booleanValue()); 181 if (src.hasCompose()) 182 tgt.setCompose(convertValueSetComposeComponent(src.getCompose())); 183 if (src.hasExpansion()) 184 tgt.setExpansion(convertValueSetExpansionComponent(src.getExpansion())); 185 return tgt; 186 } 187 188 public static org.hl7.fhir.r4.model.ValueSet convertValueSet(org.hl7.fhir.dstu3.model.ValueSet src) throws FHIRException { 189 if (src == null) 190 return null; 191 org.hl7.fhir.r4.model.ValueSet tgt = new org.hl7.fhir.r4.model.ValueSet(); 192 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyDomainResource(src, tgt); 193 if (src.hasUrl()) 194 tgt.setUrlElement(Uri30_40.convertUri(src.getUrlElement())); 195 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 196 tgt.addIdentifier(Identifier30_40.convertIdentifier(t)); 197 if (src.hasVersion()) 198 tgt.setVersionElement(String30_40.convertString(src.getVersionElement())); 199 if (src.hasName()) 200 tgt.setNameElement(String30_40.convertString(src.getNameElement())); 201 if (src.hasTitle()) 202 tgt.setTitleElement(String30_40.convertString(src.getTitleElement())); 203 if (src.hasStatus()) 204 tgt.setStatusElement(Enumerations30_40.convertPublicationStatus(src.getStatusElement())); 205 if (src.hasExperimental()) 206 tgt.setExperimentalElement(Boolean30_40.convertBoolean(src.getExperimentalElement())); 207 if (src.hasDateElement()) 208 tgt.setDateElement(DateTime30_40.convertDateTime(src.getDateElement())); 209 if (src.hasPublisher()) 210 tgt.setPublisherElement(String30_40.convertString(src.getPublisherElement())); 211 for (org.hl7.fhir.dstu3.model.ContactDetail t : src.getContact()) 212 tgt.addContact(ContactDetail30_40.convertContactDetail(t)); 213 if (src.hasDescription()) 214 tgt.setDescriptionElement(MarkDown30_40.convertMarkdown(src.getDescriptionElement())); 215 for (org.hl7.fhir.dstu3.model.UsageContext t : src.getUseContext()) 216 tgt.addUseContext(Timing30_40.convertUsageContext(t)); 217 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getJurisdiction()) 218 tgt.addJurisdiction(CodeableConcept30_40.convertCodeableConcept(t)); 219 if (src.hasImmutable()) 220 tgt.setImmutableElement(Boolean30_40.convertBoolean(src.getImmutableElement())); 221 if (src.hasPurpose()) 222 tgt.setPurposeElement(MarkDown30_40.convertMarkdown(src.getPurposeElement())); 223 if (src.hasCopyright()) 224 tgt.setCopyrightElement(MarkDown30_40.convertMarkdown(src.getCopyrightElement())); 225 if (src.hasExtensible()) 226 tgt.addExtension(VersionConvertorConstants.EXT_VS_EXTENSIBLE, new BooleanType(src.getExtensible())); 227 if (src.hasCompose()) 228 tgt.setCompose(convertValueSetComposeComponent(src.getCompose())); 229 if (src.hasExpansion()) 230 tgt.setExpansion(convertValueSetExpansionComponent(src.getExpansion())); 231 return tgt; 232 } 233 234 public static org.hl7.fhir.dstu3.model.ValueSet.ValueSetComposeComponent convertValueSetComposeComponent(org.hl7.fhir.r4.model.ValueSet.ValueSetComposeComponent src) throws FHIRException { 235 if (src == null) 236 return null; 237 org.hl7.fhir.dstu3.model.ValueSet.ValueSetComposeComponent tgt = new org.hl7.fhir.dstu3.model.ValueSet.ValueSetComposeComponent(); 238 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 239 if (src.hasLockedDate()) 240 tgt.setLockedDateElement(Date30_40.convertDate(src.getLockedDateElement())); 241 if (src.hasInactive()) 242 tgt.setInactiveElement(Boolean30_40.convertBoolean(src.getInactiveElement())); 243 for (org.hl7.fhir.r4.model.ValueSet.ConceptSetComponent t : src.getInclude()) 244 tgt.addInclude(convertConceptSetComponent(t)); 245 for (org.hl7.fhir.r4.model.ValueSet.ConceptSetComponent t : src.getExclude()) 246 tgt.addExclude(convertConceptSetComponent(t)); 247 return tgt; 248 } 249 250 public static org.hl7.fhir.r4.model.ValueSet.ValueSetComposeComponent convertValueSetComposeComponent(org.hl7.fhir.dstu3.model.ValueSet.ValueSetComposeComponent src) throws FHIRException { 251 if (src == null) 252 return null; 253 org.hl7.fhir.r4.model.ValueSet.ValueSetComposeComponent tgt = new org.hl7.fhir.r4.model.ValueSet.ValueSetComposeComponent(); 254 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 255 if (src.hasLockedDate()) 256 tgt.setLockedDateElement(Date30_40.convertDate(src.getLockedDateElement())); 257 if (src.hasInactive()) 258 tgt.setInactiveElement(Boolean30_40.convertBoolean(src.getInactiveElement())); 259 for (org.hl7.fhir.dstu3.model.ValueSet.ConceptSetComponent t : src.getInclude()) 260 tgt.addInclude(convertConceptSetComponent(t)); 261 for (org.hl7.fhir.dstu3.model.ValueSet.ConceptSetComponent t : src.getExclude()) 262 tgt.addExclude(convertConceptSetComponent(t)); 263 return tgt; 264 } 265 266 public static org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionComponent convertValueSetExpansionComponent(org.hl7.fhir.r4.model.ValueSet.ValueSetExpansionComponent src) throws FHIRException { 267 if (src == null) 268 return null; 269 org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionComponent tgt = new org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionComponent(); 270 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 271 if (src.hasIdentifier()) 272 tgt.setIdentifierElement(Uri30_40.convertUri(src.getIdentifierElement())); 273 if (src.hasTimestamp()) 274 tgt.setTimestampElement(DateTime30_40.convertDateTime(src.getTimestampElement())); 275 if (src.hasTotal()) 276 tgt.setTotalElement(Integer30_40.convertInteger(src.getTotalElement())); 277 if (src.hasOffset()) 278 tgt.setOffsetElement(Integer30_40.convertInteger(src.getOffsetElement())); 279 for (org.hl7.fhir.r4.model.ValueSet.ValueSetExpansionParameterComponent t : src.getParameter()) 280 tgt.addParameter(convertValueSetExpansionParameterComponent(t)); 281 for (org.hl7.fhir.r4.model.ValueSet.ValueSetExpansionContainsComponent t : src.getContains()) 282 tgt.addContains(convertValueSetExpansionContainsComponent(t)); 283 return tgt; 284 } 285 286 public static org.hl7.fhir.r4.model.ValueSet.ValueSetExpansionComponent convertValueSetExpansionComponent(org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionComponent src) throws FHIRException { 287 if (src == null) 288 return null; 289 org.hl7.fhir.r4.model.ValueSet.ValueSetExpansionComponent tgt = new org.hl7.fhir.r4.model.ValueSet.ValueSetExpansionComponent(); 290 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 291 if (src.hasIdentifier()) 292 tgt.setIdentifierElement(Uri30_40.convertUri(src.getIdentifierElement())); 293 if (src.hasTimestamp()) 294 tgt.setTimestampElement(DateTime30_40.convertDateTime(src.getTimestampElement())); 295 if (src.hasTotal()) 296 tgt.setTotalElement(Integer30_40.convertInteger(src.getTotalElement())); 297 if (src.hasOffset()) 298 tgt.setOffsetElement(Integer30_40.convertInteger(src.getOffsetElement())); 299 for (org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionParameterComponent t : src.getParameter()) 300 tgt.addParameter(convertValueSetExpansionParameterComponent(t)); 301 for (org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionContainsComponent t : src.getContains()) 302 tgt.addContains(convertValueSetExpansionContainsComponent(t)); 303 return tgt; 304 } 305 306 public static org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionContainsComponent convertValueSetExpansionContainsComponent(org.hl7.fhir.r4.model.ValueSet.ValueSetExpansionContainsComponent src) throws FHIRException { 307 if (src == null) 308 return null; 309 org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionContainsComponent tgt = new org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionContainsComponent(); 310 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 311 if (src.hasSystem()) 312 tgt.setSystemElement(Uri30_40.convertUri(src.getSystemElement())); 313 if (src.hasAbstract()) 314 tgt.setAbstractElement(Boolean30_40.convertBoolean(src.getAbstractElement())); 315 if (src.hasInactive()) 316 tgt.setInactiveElement(Boolean30_40.convertBoolean(src.getInactiveElement())); 317 if (src.hasVersion()) 318 tgt.setVersionElement(String30_40.convertString(src.getVersionElement())); 319 if (src.hasCode()) 320 tgt.setCodeElement(Code30_40.convertCode(src.getCodeElement())); 321 if (src.hasDisplay()) 322 tgt.setDisplayElement(String30_40.convertString(src.getDisplayElement())); 323 for (org.hl7.fhir.r4.model.ValueSet.ConceptReferenceDesignationComponent t : src.getDesignation()) 324 tgt.addDesignation(convertConceptReferenceDesignationComponent(t)); 325 for (org.hl7.fhir.r4.model.ValueSet.ValueSetExpansionContainsComponent t : src.getContains()) 326 tgt.addContains(convertValueSetExpansionContainsComponent(t)); 327 return tgt; 328 } 329 330 public static org.hl7.fhir.r4.model.ValueSet.ValueSetExpansionContainsComponent convertValueSetExpansionContainsComponent(org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionContainsComponent src) throws FHIRException { 331 if (src == null) 332 return null; 333 org.hl7.fhir.r4.model.ValueSet.ValueSetExpansionContainsComponent tgt = new org.hl7.fhir.r4.model.ValueSet.ValueSetExpansionContainsComponent(); 334 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 335 if (src.hasSystem()) 336 tgt.setSystemElement(Uri30_40.convertUri(src.getSystemElement())); 337 if (src.hasAbstract()) 338 tgt.setAbstractElement(Boolean30_40.convertBoolean(src.getAbstractElement())); 339 if (src.hasInactive()) 340 tgt.setInactiveElement(Boolean30_40.convertBoolean(src.getInactiveElement())); 341 if (src.hasVersion()) 342 tgt.setVersionElement(String30_40.convertString(src.getVersionElement())); 343 if (src.hasCode()) 344 tgt.setCodeElement(Code30_40.convertCode(src.getCodeElement())); 345 if (src.hasDisplay()) 346 tgt.setDisplayElement(String30_40.convertString(src.getDisplayElement())); 347 for (org.hl7.fhir.dstu3.model.ValueSet.ConceptReferenceDesignationComponent t : src.getDesignation()) 348 tgt.addDesignation(convertConceptReferenceDesignationComponent(t)); 349 for (org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionContainsComponent t : src.getContains()) 350 tgt.addContains(convertValueSetExpansionContainsComponent(t)); 351 return tgt; 352 } 353 354 public static org.hl7.fhir.r4.model.ValueSet.ValueSetExpansionParameterComponent convertValueSetExpansionParameterComponent(org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionParameterComponent src) throws FHIRException { 355 if (src == null) 356 return null; 357 org.hl7.fhir.r4.model.ValueSet.ValueSetExpansionParameterComponent tgt = new org.hl7.fhir.r4.model.ValueSet.ValueSetExpansionParameterComponent(); 358 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 359 if (src.hasName()) 360 tgt.setNameElement(String30_40.convertString(src.getNameElement())); 361 if (src.hasValue()) 362 tgt.setValue(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getValue())); 363 return tgt; 364 } 365 366 public static org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionParameterComponent convertValueSetExpansionParameterComponent(org.hl7.fhir.r4.model.ValueSet.ValueSetExpansionParameterComponent src) throws FHIRException { 367 if (src == null) 368 return null; 369 org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionParameterComponent tgt = new org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionParameterComponent(); 370 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyBackboneElement(src,tgt); 371 if (src.hasName()) 372 tgt.setNameElement(String30_40.convertString(src.getNameElement())); 373 if (src.hasValue()) 374 tgt.setValue(ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().convertType(src.getValue())); 375 return tgt; 376 } 377 378 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ValueSet.FilterOperator> convertFilterOperator(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ValueSet.FilterOperator> src) throws FHIRException { 379 if (src == null || src.isEmpty()) return null; 380 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ValueSet.FilterOperator> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.ValueSet.FilterOperatorEnumFactory()); 381 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 382 if (src.getValue() == null) { 383 tgt.setValue(null); 384} else { 385 switch(src.getValue()) { 386 case EQUAL: 387 tgt.setValue(ValueSet.FilterOperator.EQUAL); 388 break; 389 case ISA: 390 tgt.setValue(ValueSet.FilterOperator.ISA); 391 break; 392 case DESCENDENTOF: 393 tgt.setValue(ValueSet.FilterOperator.DESCENDENTOF); 394 break; 395 case ISNOTA: 396 tgt.setValue(ValueSet.FilterOperator.ISNOTA); 397 break; 398 case REGEX: 399 tgt.setValue(ValueSet.FilterOperator.REGEX); 400 break; 401 case IN: 402 tgt.setValue(ValueSet.FilterOperator.IN); 403 break; 404 case NOTIN: 405 tgt.setValue(ValueSet.FilterOperator.NOTIN); 406 break; 407 case GENERALIZES: 408 tgt.setValue(ValueSet.FilterOperator.GENERALIZES); 409 break; 410 case EXISTS: 411 tgt.setValue(ValueSet.FilterOperator.EXISTS); 412 break; 413 default: 414 tgt.setValue(ValueSet.FilterOperator.NULL); 415 break; 416 } 417} 418 return tgt; 419 } 420 421 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ValueSet.FilterOperator> convertFilterOperator(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ValueSet.FilterOperator> src) throws FHIRException { 422 if (src == null || src.isEmpty()) return null; 423 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ValueSet.FilterOperator> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.ValueSet.FilterOperatorEnumFactory()); 424 ConversionContext30_40.INSTANCE.getVersionConvertor_30_40().copyElement(src, tgt); 425 if (src.getValue() == null) { 426 tgt.setValue(null); 427} else { 428 switch(src.getValue()) { 429 case EQUAL: 430 tgt.setValue(org.hl7.fhir.dstu3.model.ValueSet.FilterOperator.EQUAL); 431 break; 432 case ISA: 433 tgt.setValue(org.hl7.fhir.dstu3.model.ValueSet.FilterOperator.ISA); 434 break; 435 case DESCENDENTOF: 436 tgt.setValue(org.hl7.fhir.dstu3.model.ValueSet.FilterOperator.DESCENDENTOF); 437 break; 438 case ISNOTA: 439 tgt.setValue(org.hl7.fhir.dstu3.model.ValueSet.FilterOperator.ISNOTA); 440 break; 441 case REGEX: 442 tgt.setValue(org.hl7.fhir.dstu3.model.ValueSet.FilterOperator.REGEX); 443 break; 444 case IN: 445 tgt.setValue(org.hl7.fhir.dstu3.model.ValueSet.FilterOperator.IN); 446 break; 447 case NOTIN: 448 tgt.setValue(org.hl7.fhir.dstu3.model.ValueSet.FilterOperator.NOTIN); 449 break; 450 case GENERALIZES: 451 tgt.setValue(org.hl7.fhir.dstu3.model.ValueSet.FilterOperator.GENERALIZES); 452 break; 453 case EXISTS: 454 tgt.setValue(org.hl7.fhir.dstu3.model.ValueSet.FilterOperator.EXISTS); 455 break; 456 default: 457 tgt.setValue(org.hl7.fhir.dstu3.model.ValueSet.FilterOperator.NULL); 458 break; 459 } 460} 461 return tgt; 462 } 463}