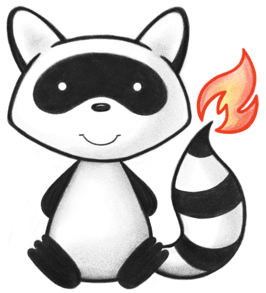
001package org.hl7.fhir.convertors.conv30_50.datatypes30_50; 002 003import java.util.List; 004import java.util.stream.Collectors; 005 006import org.hl7.fhir.convertors.VersionConvertorConstants; 007import org.hl7.fhir.convertors.context.ConversionContext30_50; 008import org.hl7.fhir.convertors.conv30_50.VersionConvertor_30_50; 009import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Coding30_50; 010import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Boolean30_50; 011import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Code30_50; 012import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Id30_50; 013import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Integer30_50; 014import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.MarkDown30_50; 015import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.String30_50; 016import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.UnsignedInt30_50; 017import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Uri30_50; 018import org.hl7.fhir.convertors.conv30_50.resources30_50.Enumerations30_50; 019import org.hl7.fhir.exceptions.FHIRException; 020import org.hl7.fhir.r5.model.CanonicalType; 021import org.hl7.fhir.r5.model.DataType; 022import org.hl7.fhir.r5.model.ElementDefinition; 023import org.hl7.fhir.utilities.Utilities; 024 025public class ElementDefinition30_50 { 026 public static org.hl7.fhir.r5.model.ElementDefinition convertElementDefinition(org.hl7.fhir.dstu3.model.ElementDefinition src) throws FHIRException { 027 if (src == null) return null; 028 org.hl7.fhir.r5.model.ElementDefinition tgt = new org.hl7.fhir.r5.model.ElementDefinition(); 029 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt, "http://hl7.org/fhir/5.0/StructureDefinition/extension-ElementDefinition.mustHaveValue", "http://hl7.org/fhir/5.0/StructureDefinition/extension-ElementDefinition.valueAlternatives"); 030 if (src.hasPath()) tgt.setPathElement(String30_50.convertString(src.getPathElement())); 031 tgt.setRepresentation(src.getRepresentation().stream().map(ElementDefinition30_50::convertPropertyRepresentation).collect(Collectors.toList())); 032 if (src.hasSliceName()) tgt.setSliceNameElement(String30_50.convertString(src.getSliceNameElement())); 033 if (src.hasLabel()) tgt.setLabelElement(String30_50.convertString(src.getLabelElement())); 034 for (org.hl7.fhir.dstu3.model.Coding t : src.getCode()) tgt.addCode(Coding30_50.convertCoding(t)); 035 if (src.hasSlicing()) tgt.setSlicing(convertElementDefinitionSlicingComponent(src.getSlicing())); 036 if (src.hasShort()) tgt.setShortElement(String30_50.convertString(src.getShortElement())); 037 if (src.hasDefinition()) tgt.setDefinitionElement(MarkDown30_50.convertMarkdown(src.getDefinitionElement())); 038 if (src.hasComment()) tgt.setCommentElement(MarkDown30_50.convertMarkdown(src.getCommentElement())); 039 if (src.hasRequirements()) tgt.setRequirementsElement(MarkDown30_50.convertMarkdown(src.getRequirementsElement())); 040 for (org.hl7.fhir.dstu3.model.StringType t : src.getAlias()) tgt.addAlias(t.getValue()); 041 if (src.hasMin()) tgt.setMinElement(UnsignedInt30_50.convertUnsignedInt(src.getMinElement())); 042 if (src.hasMax()) tgt.setMaxElement(String30_50.convertString(src.getMaxElement())); 043 if (src.hasBase()) tgt.setBase(convertElementDefinitionBaseComponent(src.getBase())); 044 if (src.hasContentReference()) 045 tgt.setContentReferenceElement(Uri30_50.convertUri(src.getContentReferenceElement())); 046 for (org.hl7.fhir.dstu3.model.ElementDefinition.TypeRefComponent t : src.getType()) 047 convertTypeRefComponent(t, tgt.getType()); 048 if (src.hasDefaultValue()) 049 tgt.setDefaultValue(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getDefaultValue())); 050 if (src.hasMeaningWhenMissing()) 051 tgt.setMeaningWhenMissingElement(MarkDown30_50.convertMarkdown(src.getMeaningWhenMissingElement())); 052 if (src.hasOrderMeaning()) tgt.setOrderMeaningElement(String30_50.convertString(src.getOrderMeaningElement())); 053 if (src.hasFixed()) 054 tgt.setFixed(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getFixed())); 055 if (src.hasPattern()) 056 tgt.setPattern(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getPattern())); 057 for (org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionExampleComponent t : src.getExample()) 058 tgt.addExample(convertElementDefinitionExampleComponent(t)); 059 if (src.hasMinValue()) 060 tgt.setMinValue(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getMinValue())); 061 if (src.hasMaxValue()) 062 tgt.setMaxValue(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getMaxValue())); 063 if (src.hasMaxLength()) tgt.setMaxLengthElement(Integer30_50.convertInteger(src.getMaxLengthElement())); 064 for (org.hl7.fhir.dstu3.model.IdType t : src.getCondition()) tgt.addCondition(t.getValue()); 065 for (org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionConstraintComponent t : src.getConstraint()) 066 tgt.addConstraint(convertElementDefinitionConstraintComponent(t)); 067 if (src.hasMustSupport()) tgt.setMustSupportElement(Boolean30_50.convertBoolean(src.getMustSupportElement())); 068 if (src.hasIsModifier()) tgt.setIsModifierElement(Boolean30_50.convertBoolean(src.getIsModifierElement())); 069 if (tgt.getIsModifier()) { 070 String reason = org.hl7.fhir.dstu3.utils.ToolingExtensions.readStringExtension(src, VersionConvertorConstants.MODIFIER_REASON_EXTENSION); 071 if (Utilities.noString(reason)) reason = VersionConvertorConstants.MODIFIER_REASON_LEGACY; 072 tgt.setIsModifierReason(reason); 073 } 074 if (src.hasIsSummary()) tgt.setIsSummaryElement(Boolean30_50.convertBoolean(src.getIsSummaryElement())); 075 if (src.hasBinding()) tgt.setBinding(convertElementDefinitionBindingComponent(src.getBinding())); 076 for (org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionMappingComponent t : src.getMapping()) 077 tgt.addMapping(convertElementDefinitionMappingComponent(t)); 078 079 if (src.hasExtension("http://hl7.org/fhir/5.0/StructureDefinition/extension-ElementDefinition.mustHaveValue")) { 080 tgt.setMustHaveValueElement(Boolean30_50.convertBoolean((org.hl7.fhir.dstu3.model.BooleanType) src.getExtensionByUrl("http://hl7.org/fhir/5.0/StructureDefinition/extension-ElementDefinition.mustHaveValue").getValueAsPrimitive())); 081 } 082 for (org.hl7.fhir.dstu3.model.Extension ext : src.getExtensionsByUrl("http://hl7.org/fhir/5.0/StructureDefinition/extension-ElementDefinition.valueAlternatives")) { 083 tgt.addValueAlternative(Uri30_50.convertCanonical((org.hl7.fhir.dstu3.model.UriType)ext.getValue())); 084 } 085 086 return tgt; 087 } 088 089 public static org.hl7.fhir.dstu3.model.ElementDefinition convertElementDefinition(org.hl7.fhir.r5.model.ElementDefinition src) throws FHIRException { 090 if (src == null) return null; 091 org.hl7.fhir.dstu3.model.ElementDefinition tgt = new org.hl7.fhir.dstu3.model.ElementDefinition(); 092 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 093 if (src.hasPath()) tgt.setPathElement(String30_50.convertString(src.getPathElement())); 094 tgt.setRepresentation(src.getRepresentation().stream().map(ElementDefinition30_50::convertPropertyRepresentation).collect(Collectors.toList())); 095 if (src.hasSliceName()) tgt.setSliceNameElement(String30_50.convertString(src.getSliceNameElement())); 096 if (src.hasLabel()) tgt.setLabelElement(String30_50.convertString(src.getLabelElement())); 097 for (org.hl7.fhir.r5.model.Coding t : src.getCode()) tgt.addCode(Coding30_50.convertCoding(t)); 098 if (src.hasSlicing()) tgt.setSlicing(convertElementDefinitionSlicingComponent(src.getSlicing())); 099 if (src.hasShort()) tgt.setShortElement(String30_50.convertString(src.getShortElement())); 100 if (src.hasDefinition()) tgt.setDefinitionElement(MarkDown30_50.convertMarkdown(src.getDefinitionElement())); 101 if (src.hasComment()) tgt.setCommentElement(MarkDown30_50.convertMarkdown(src.getCommentElement())); 102 if (src.hasRequirements()) tgt.setRequirementsElement(MarkDown30_50.convertMarkdown(src.getRequirementsElement())); 103 for (org.hl7.fhir.r5.model.StringType t : src.getAlias()) tgt.addAlias(t.getValue()); 104 if (src.hasMin()) tgt.setMinElement(UnsignedInt30_50.convertUnsignedInt(src.getMinElement())); 105 if (src.hasMax()) tgt.setMaxElement(String30_50.convertString(src.getMaxElement())); 106 if (src.hasBase()) tgt.setBase(convertElementDefinitionBaseComponent(src.getBase())); 107 if (src.hasContentReference()) 108 tgt.setContentReferenceElement(Uri30_50.convertUri(src.getContentReferenceElement())); 109 for (org.hl7.fhir.r5.model.ElementDefinition.TypeRefComponent t : src.getType()) 110 convertTypeRefComponent(t, tgt.getType()); 111 if (src.hasDefaultValue()) 112 tgt.setDefaultValue(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getDefaultValue())); 113 if (src.hasMeaningWhenMissing()) 114 tgt.setMeaningWhenMissingElement(MarkDown30_50.convertMarkdown(src.getMeaningWhenMissingElement())); 115 if (src.hasOrderMeaning()) tgt.setOrderMeaningElement(String30_50.convertString(src.getOrderMeaningElement())); 116 if (src.hasFixed()) 117 tgt.setFixed(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getFixed())); 118 if (src.hasPattern()) 119 tgt.setPattern(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getPattern())); 120 for (org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionExampleComponent t : src.getExample()) 121 tgt.addExample(convertElementDefinitionExampleComponent(t)); 122 if (src.hasMinValue()) 123 tgt.setMinValue(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getMinValue())); 124 if (src.hasMaxValue()) 125 tgt.setMaxValue(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getMaxValue())); 126 if (src.hasMaxLength()) tgt.setMaxLengthElement(Integer30_50.convertInteger(src.getMaxLengthElement())); 127 for (org.hl7.fhir.r5.model.IdType t : src.getCondition()) tgt.addCondition(t.getValue()); 128 for (org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionConstraintComponent t : src.getConstraint()) 129 tgt.addConstraint(convertElementDefinitionConstraintComponent(t)); 130 if (src.hasMustSupport()) tgt.setMustSupportElement(Boolean30_50.convertBoolean(src.getMustSupportElement())); 131 if (src.hasIsModifier()) tgt.setIsModifierElement(Boolean30_50.convertBoolean(src.getIsModifierElement())); 132 if (src.hasIsModifierReason() && !VersionConvertorConstants.MODIFIER_REASON_LEGACY.equals(src.getIsModifierReason())) 133 org.hl7.fhir.dstu3.utils.ToolingExtensions.setStringExtension(tgt, VersionConvertorConstants.MODIFIER_REASON_EXTENSION, src.getIsModifierReason()); 134 if (src.hasIsSummary()) tgt.setIsSummaryElement(Boolean30_50.convertBoolean(src.getIsSummaryElement())); 135 if (src.hasBinding()) tgt.setBinding(convertElementDefinitionBindingComponent(src.getBinding())); 136 for (org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionMappingComponent t : src.getMapping()) 137 tgt.addMapping(convertElementDefinitionMappingComponent(t)); 138 if (src.hasMustHaveValue()) { 139 tgt.addExtension("http://hl7.org/fhir/5.0/StructureDefinition/extension-ElementDefinition.mustHaveValue", Boolean30_50.convertBoolean(src.getMustHaveValueElement())); 140 } 141 for (org.hl7.fhir.r5.model.CanonicalType ct : src.getValueAlternatives()) { 142 tgt.addExtension("http://hl7.org/fhir/5.0/StructureDefinition/extension-ElementDefinition.valueAlternatives", Uri30_50.convertCanonical(ct)); 143 } 144 145 return tgt; 146 } 147 148 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ElementDefinition.PropertyRepresentation> convertPropertyRepresentation(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ElementDefinition.PropertyRepresentation> src) throws FHIRException { 149 if (src == null || src.isEmpty()) return null; 150 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ElementDefinition.PropertyRepresentation> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.ElementDefinition.PropertyRepresentationEnumFactory()); 151 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 152 if (src.getValue() == null) { 153 tgt.setValue(org.hl7.fhir.r5.model.ElementDefinition.PropertyRepresentation.NULL); 154 } else { 155 switch (src.getValue()) { 156 case XMLATTR: 157 tgt.setValue(org.hl7.fhir.r5.model.ElementDefinition.PropertyRepresentation.XMLATTR); 158 break; 159 case XMLTEXT: 160 tgt.setValue(org.hl7.fhir.r5.model.ElementDefinition.PropertyRepresentation.XMLTEXT); 161 break; 162 case TYPEATTR: 163 tgt.setValue(org.hl7.fhir.r5.model.ElementDefinition.PropertyRepresentation.TYPEATTR); 164 break; 165 case CDATEXT: 166 tgt.setValue(org.hl7.fhir.r5.model.ElementDefinition.PropertyRepresentation.CDATEXT); 167 break; 168 case XHTML: 169 tgt.setValue(org.hl7.fhir.r5.model.ElementDefinition.PropertyRepresentation.XHTML); 170 break; 171 default: 172 tgt.setValue(org.hl7.fhir.r5.model.ElementDefinition.PropertyRepresentation.NULL); 173 break; 174 } 175 } 176 return tgt; 177 } 178 179 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ElementDefinition.PropertyRepresentation> convertPropertyRepresentation(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ElementDefinition.PropertyRepresentation> src) throws FHIRException { 180 if (src == null || src.isEmpty()) return null; 181 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ElementDefinition.PropertyRepresentation> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.ElementDefinition.PropertyRepresentationEnumFactory()); 182 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 183 if (src.getValue() == null) { 184 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.PropertyRepresentation.NULL); 185 } else { 186 switch (src.getValue()) { 187 case XMLATTR: 188 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.PropertyRepresentation.XMLATTR); 189 break; 190 case XMLTEXT: 191 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.PropertyRepresentation.XMLTEXT); 192 break; 193 case TYPEATTR: 194 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.PropertyRepresentation.TYPEATTR); 195 break; 196 case CDATEXT: 197 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.PropertyRepresentation.CDATEXT); 198 break; 199 case XHTML: 200 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.PropertyRepresentation.XHTML); 201 break; 202 default: 203 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.PropertyRepresentation.NULL); 204 break; 205 } 206 } 207 return tgt; 208 } 209 210 public static org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionSlicingComponent convertElementDefinitionSlicingComponent(org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionSlicingComponent src) throws FHIRException { 211 if (src == null) return null; 212 org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionSlicingComponent tgt = new org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionSlicingComponent(); 213 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 214 for (org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionSlicingDiscriminatorComponent t : src.getDiscriminator()) 215 tgt.addDiscriminator(convertElementDefinitionSlicingDiscriminatorComponent(t)); 216 if (src.hasDescription()) tgt.setDescriptionElement(String30_50.convertString(src.getDescriptionElement())); 217 if (src.hasOrdered()) tgt.setOrderedElement(Boolean30_50.convertBoolean(src.getOrderedElement())); 218 if (src.hasRules()) tgt.setRulesElement(convertSlicingRules(src.getRulesElement())); 219 return tgt; 220 } 221 222 public static org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionSlicingComponent convertElementDefinitionSlicingComponent(org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionSlicingComponent src) throws FHIRException { 223 if (src == null) return null; 224 org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionSlicingComponent tgt = new org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionSlicingComponent(); 225 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 226 for (org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionSlicingDiscriminatorComponent t : src.getDiscriminator()) 227 tgt.addDiscriminator(convertElementDefinitionSlicingDiscriminatorComponent(t)); 228 if (src.hasDescription()) tgt.setDescriptionElement(String30_50.convertString(src.getDescriptionElement())); 229 if (src.hasOrdered()) tgt.setOrderedElement(Boolean30_50.convertBoolean(src.getOrderedElement())); 230 if (src.hasRules()) tgt.setRulesElement(convertSlicingRules(src.getRulesElement())); 231 return tgt; 232 } 233 234 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ElementDefinition.SlicingRules> convertSlicingRules(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ElementDefinition.SlicingRules> src) throws FHIRException { 235 if (src == null || src.isEmpty()) return null; 236 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ElementDefinition.SlicingRules> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.ElementDefinition.SlicingRulesEnumFactory()); 237 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 238 if (src.getValue() == null) { 239 tgt.setValue(org.hl7.fhir.r5.model.ElementDefinition.SlicingRules.NULL); 240 } else { 241 switch (src.getValue()) { 242 case CLOSED: 243 tgt.setValue(org.hl7.fhir.r5.model.ElementDefinition.SlicingRules.CLOSED); 244 break; 245 case OPEN: 246 tgt.setValue(org.hl7.fhir.r5.model.ElementDefinition.SlicingRules.OPEN); 247 break; 248 case OPENATEND: 249 tgt.setValue(org.hl7.fhir.r5.model.ElementDefinition.SlicingRules.OPENATEND); 250 break; 251 default: 252 tgt.setValue(org.hl7.fhir.r5.model.ElementDefinition.SlicingRules.NULL); 253 break; 254 } 255 } 256 return tgt; 257 } 258 259 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ElementDefinition.SlicingRules> convertSlicingRules(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ElementDefinition.SlicingRules> src) throws FHIRException { 260 if (src == null || src.isEmpty()) return null; 261 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ElementDefinition.SlicingRules> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.ElementDefinition.SlicingRulesEnumFactory()); 262 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 263 if (src.getValue() == null) { 264 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.SlicingRules.NULL); 265 } else { 266 switch (src.getValue()) { 267 case CLOSED: 268 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.SlicingRules.CLOSED); 269 break; 270 case OPEN: 271 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.SlicingRules.OPEN); 272 break; 273 case OPENATEND: 274 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.SlicingRules.OPENATEND); 275 break; 276 default: 277 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.SlicingRules.NULL); 278 break; 279 } 280 } 281 return tgt; 282 } 283 284 public static org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionSlicingDiscriminatorComponent convertElementDefinitionSlicingDiscriminatorComponent(org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionSlicingDiscriminatorComponent src) throws FHIRException { 285 if (src == null) return null; 286 org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionSlicingDiscriminatorComponent tgt = new org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionSlicingDiscriminatorComponent(); 287 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 288 if (src.hasType()) tgt.setTypeElement(convertDiscriminatorType(src.getTypeElement())); 289 if (src.hasPath()) tgt.setPathElement(String30_50.convertString(src.getPathElement())); 290 return tgt; 291 } 292 293 public static org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionSlicingDiscriminatorComponent convertElementDefinitionSlicingDiscriminatorComponent(org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionSlicingDiscriminatorComponent src) throws FHIRException { 294 if (src == null) return null; 295 org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionSlicingDiscriminatorComponent tgt = new org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionSlicingDiscriminatorComponent(); 296 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 297 if (src.hasType()) tgt.setTypeElement(convertDiscriminatorType(src.getTypeElement())); 298 if (src.hasPath()) tgt.setPathElement(String30_50.convertString(src.getPathElement())); 299 return tgt; 300 } 301 302 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ElementDefinition.DiscriminatorType> convertDiscriminatorType(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ElementDefinition.DiscriminatorType> src) throws FHIRException { 303 if (src == null || src.isEmpty()) return null; 304 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ElementDefinition.DiscriminatorType> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.ElementDefinition.DiscriminatorTypeEnumFactory()); 305 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 306 if (src.getValue() == null) { 307 tgt.setValue(org.hl7.fhir.r5.model.ElementDefinition.DiscriminatorType.NULL); 308 } else { 309 switch (src.getValue()) { 310 case VALUE: 311 tgt.setValue(org.hl7.fhir.r5.model.ElementDefinition.DiscriminatorType.VALUE); 312 break; 313 case EXISTS: 314 tgt.setValue(org.hl7.fhir.r5.model.ElementDefinition.DiscriminatorType.EXISTS); 315 break; 316 case PATTERN: 317 tgt.setValue(org.hl7.fhir.r5.model.ElementDefinition.DiscriminatorType.PATTERN); 318 break; 319 case TYPE: 320 tgt.setValue(org.hl7.fhir.r5.model.ElementDefinition.DiscriminatorType.TYPE); 321 break; 322 case PROFILE: 323 tgt.setValue(org.hl7.fhir.r5.model.ElementDefinition.DiscriminatorType.PROFILE); 324 break; 325 default: 326 tgt.setValue(org.hl7.fhir.r5.model.ElementDefinition.DiscriminatorType.NULL); 327 break; 328 } 329 } 330 return tgt; 331 } 332 333 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ElementDefinition.DiscriminatorType> convertDiscriminatorType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ElementDefinition.DiscriminatorType> src) throws FHIRException { 334 if (src == null || src.isEmpty()) return null; 335 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ElementDefinition.DiscriminatorType> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.ElementDefinition.DiscriminatorTypeEnumFactory()); 336 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 337 if (src.getValue() == null) { 338 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.DiscriminatorType.NULL); 339 } else { 340 switch (src.getValue()) { 341 case VALUE: 342 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.DiscriminatorType.VALUE); 343 break; 344 case EXISTS: 345 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.DiscriminatorType.EXISTS); 346 break; 347 case PATTERN: 348 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.DiscriminatorType.PATTERN); 349 break; 350 case TYPE: 351 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.DiscriminatorType.TYPE); 352 break; 353 case PROFILE: 354 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.DiscriminatorType.PROFILE); 355 break; 356 default: 357 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.DiscriminatorType.NULL); 358 break; 359 } 360 } 361 return tgt; 362 } 363 364 public static org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionBaseComponent convertElementDefinitionBaseComponent(org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionBaseComponent src) throws FHIRException { 365 if (src == null) return null; 366 org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionBaseComponent tgt = new org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionBaseComponent(); 367 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 368 if (src.hasPath()) tgt.setPathElement(String30_50.convertString(src.getPathElement())); 369 if (src.hasMin()) tgt.setMinElement(UnsignedInt30_50.convertUnsignedInt(src.getMinElement())); 370 if (src.hasMax()) tgt.setMaxElement(String30_50.convertString(src.getMaxElement())); 371 return tgt; 372 } 373 374 public static org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionBaseComponent convertElementDefinitionBaseComponent(org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionBaseComponent src) throws FHIRException { 375 if (src == null) return null; 376 org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionBaseComponent tgt = new org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionBaseComponent(); 377 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 378 if (src.hasPath()) tgt.setPathElement(String30_50.convertString(src.getPathElement())); 379 if (src.hasMin()) tgt.setMinElement(UnsignedInt30_50.convertUnsignedInt(src.getMinElement())); 380 if (src.hasMax()) tgt.setMaxElement(String30_50.convertString(src.getMaxElement())); 381 return tgt; 382 } 383 384 public static void convertTypeRefComponent(org.hl7.fhir.dstu3.model.ElementDefinition.TypeRefComponent src, List<ElementDefinition.TypeRefComponent> list) throws FHIRException { 385 if (src == null) return; 386 ElementDefinition.TypeRefComponent tgt = null; 387 for (ElementDefinition.TypeRefComponent t : list) 388 if (t.getCode().equals(src.getCode())) tgt = t; 389 if (tgt == null) { 390 tgt = new ElementDefinition.TypeRefComponent(); 391 list.add(tgt); 392 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 393 tgt.setCodeElement(Uri30_50.convertUri(src.getCodeElement())); 394 } 395 if (src.hasProfile()) { 396 boolean found = false; 397 for (CanonicalType p : tgt.getProfile()) { 398 if (p.equals(src.getProfile())) found = true; 399 } 400 if (!found) tgt.addProfile(src.getProfile()); 401 } 402 if (src.hasTargetProfile()) tgt.addTargetProfile(src.getTargetProfile()); 403 for (org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ElementDefinition.AggregationMode> t : src.getAggregation()) { 404 org.hl7.fhir.r5.model.Enumeration<ElementDefinition.AggregationMode> a = convertAggregationMode(t); 405 if (!tgt.hasAggregation(a.getValue())) 406 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(t, tgt.addAggregation(a.getValue())); 407 } 408 if (src.hasVersioning()) tgt.setVersioningElement(convertReferenceVersionRules(src.getVersioningElement())); 409 } 410 411 public static void convertTypeRefComponent(ElementDefinition.TypeRefComponent src, List<org.hl7.fhir.dstu3.model.ElementDefinition.TypeRefComponent> list) throws FHIRException { 412 if (src == null) return; 413 org.hl7.fhir.dstu3.model.ElementDefinition.TypeRefComponent tgt = new org.hl7.fhir.dstu3.model.ElementDefinition.TypeRefComponent(); 414 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 415 tgt.setCodeElement(Uri30_50.convertUri(src.getCodeElement())); 416 list.add(tgt); 417 if (src.hasTarget()) { 418 if (src.hasProfile()) tgt.setProfile(src.getProfile().get(0).getValue()); 419 for (org.hl7.fhir.r5.model.UriType u : src.getTargetProfile()) { 420 if (tgt.hasTargetProfile()) { 421 tgt = new org.hl7.fhir.dstu3.model.ElementDefinition.TypeRefComponent(); 422 list.add(tgt); 423 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 424 tgt.setCode(src.getCode()); 425 if (src.hasProfile()) tgt.setProfile(src.getProfile().get(0).getValue()); 426 } 427 tgt.setTargetProfile(u.getValue()); 428 } 429 } else { 430 for (org.hl7.fhir.r5.model.UriType u : src.getProfile()) { 431 if (tgt.hasProfile()) { 432 tgt = new org.hl7.fhir.dstu3.model.ElementDefinition.TypeRefComponent(); 433 list.add(tgt); 434 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 435 tgt.setCode(src.getCode()); 436 } 437 tgt.setProfile(u.getValue()); 438 } 439 } 440 for (org.hl7.fhir.r5.model.Enumeration<ElementDefinition.AggregationMode> t : src.getAggregation()) { 441 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ElementDefinition.AggregationMode> a = convertAggregationMode(t); 442 if (!tgt.hasAggregation(a.getValue())) 443 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(t, tgt.addAggregation(a.getValue())); 444 } 445 } 446 447 static public org.hl7.fhir.r5.model.Enumeration<ElementDefinition.AggregationMode> convertAggregationMode(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ElementDefinition.AggregationMode> src) throws FHIRException { 448 if (src == null || src.isEmpty()) return null; 449 org.hl7.fhir.r5.model.Enumeration<ElementDefinition.AggregationMode> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new ElementDefinition.AggregationModeEnumFactory()); 450 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 451 if (src.getValue() == null) { 452 tgt.setValue(ElementDefinition.AggregationMode.NULL); 453 } else { 454 switch (src.getValue()) { 455 case CONTAINED: 456 tgt.setValue(ElementDefinition.AggregationMode.CONTAINED); 457 break; 458 case REFERENCED: 459 tgt.setValue(ElementDefinition.AggregationMode.REFERENCED); 460 break; 461 case BUNDLED: 462 tgt.setValue(ElementDefinition.AggregationMode.BUNDLED); 463 break; 464 default: 465 tgt.setValue(ElementDefinition.AggregationMode.NULL); 466 break; 467 } 468 } 469 return tgt; 470 } 471 472 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ElementDefinition.AggregationMode> convertAggregationMode(org.hl7.fhir.r5.model.Enumeration<ElementDefinition.AggregationMode> src) throws FHIRException { 473 if (src == null || src.isEmpty()) return null; 474 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ElementDefinition.AggregationMode> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.ElementDefinition.AggregationModeEnumFactory()); 475 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 476 if (src.getValue() == null) { 477 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.AggregationMode.NULL); 478 } else { 479 switch (src.getValue()) { 480 case CONTAINED: 481 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.AggregationMode.CONTAINED); 482 break; 483 case REFERENCED: 484 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.AggregationMode.REFERENCED); 485 break; 486 case BUNDLED: 487 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.AggregationMode.BUNDLED); 488 break; 489 default: 490 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.AggregationMode.NULL); 491 break; 492 } 493 } 494 return tgt; 495 } 496 497 static public org.hl7.fhir.r5.model.Enumeration<ElementDefinition.ReferenceVersionRules> convertReferenceVersionRules(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ElementDefinition.ReferenceVersionRules> src) throws FHIRException { 498 if (src == null || src.isEmpty()) return null; 499 org.hl7.fhir.r5.model.Enumeration<ElementDefinition.ReferenceVersionRules> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new ElementDefinition.ReferenceVersionRulesEnumFactory()); 500 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 501 if (src.getValue() == null) { 502 tgt.setValue(ElementDefinition.ReferenceVersionRules.NULL); 503 } else { 504 switch (src.getValue()) { 505 case EITHER: 506 tgt.setValue(ElementDefinition.ReferenceVersionRules.EITHER); 507 break; 508 case INDEPENDENT: 509 tgt.setValue(ElementDefinition.ReferenceVersionRules.INDEPENDENT); 510 break; 511 case SPECIFIC: 512 tgt.setValue(ElementDefinition.ReferenceVersionRules.SPECIFIC); 513 break; 514 default: 515 tgt.setValue(ElementDefinition.ReferenceVersionRules.NULL); 516 break; 517 } 518 } 519 return tgt; 520 } 521 522 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ElementDefinition.ReferenceVersionRules> convertReferenceVersionRules(org.hl7.fhir.r5.model.Enumeration<ElementDefinition.ReferenceVersionRules> src) throws FHIRException { 523 if (src == null || src.isEmpty()) return null; 524 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ElementDefinition.ReferenceVersionRules> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.ElementDefinition.ReferenceVersionRulesEnumFactory()); 525 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 526 if (src.getValue() == null) { 527 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.ReferenceVersionRules.NULL); 528 } else { 529 switch (src.getValue()) { 530 case EITHER: 531 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.ReferenceVersionRules.EITHER); 532 break; 533 case INDEPENDENT: 534 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.ReferenceVersionRules.INDEPENDENT); 535 break; 536 case SPECIFIC: 537 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.ReferenceVersionRules.SPECIFIC); 538 break; 539 default: 540 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.ReferenceVersionRules.NULL); 541 break; 542 } 543 } 544 return tgt; 545 } 546 547 public static ElementDefinition.ElementDefinitionExampleComponent convertElementDefinitionExampleComponent(org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionExampleComponent src) throws FHIRException { 548 if (src == null) return null; 549 ElementDefinition.ElementDefinitionExampleComponent tgt = new ElementDefinition.ElementDefinitionExampleComponent(); 550 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 551 if (src.hasLabel()) tgt.setLabelElement(String30_50.convertString(src.getLabelElement())); 552 if (src.hasValue()) 553 tgt.setValue(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getValue())); 554 return tgt; 555 } 556 557 public static org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionExampleComponent convertElementDefinitionExampleComponent(ElementDefinition.ElementDefinitionExampleComponent src) throws FHIRException { 558 if (src == null) return null; 559 org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionExampleComponent tgt = new org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionExampleComponent(); 560 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 561 if (src.hasLabel()) tgt.setLabelElement(String30_50.convertString(src.getLabelElement())); 562 if (src.hasValue()) 563 tgt.setValue(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getValue())); 564 return tgt; 565 } 566 567 public static ElementDefinition.ElementDefinitionConstraintComponent convertElementDefinitionConstraintComponent(org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionConstraintComponent src) throws FHIRException { 568 if (src == null) return null; 569 ElementDefinition.ElementDefinitionConstraintComponent tgt = new ElementDefinition.ElementDefinitionConstraintComponent(); 570 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 571 if (src.hasKey()) tgt.setKeyElement(Id30_50.convertId(src.getKeyElement())); 572 if (src.hasRequirements()) tgt.setRequirementsElement(String30_50.convertStringToMarkdown(src.getRequirementsElement())); 573 if (src.hasSeverity()) tgt.setSeverityElement(convertConstraintSeverity(src.getSeverityElement())); 574 if (src.hasHuman()) tgt.setHumanElement(String30_50.convertString(src.getHumanElement())); 575 if (src.hasExpression()) tgt.setExpressionElement(String30_50.convertString(src.getExpressionElement())); 576 if (src.hasXpath()) { 577 tgt.addExtension(new org.hl7.fhir.r5.model.Extension(org.hl7.fhir.r5.utils.ToolingExtensions.EXT_XPATH_CONSTRAINT, new org.hl7.fhir.r5.model.StringType(src.getXpath()))); 578 } 579 if (src.hasSource()) tgt.setSource(src.getSource()); 580 return tgt; 581 } 582 583 public static org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionConstraintComponent convertElementDefinitionConstraintComponent(ElementDefinition.ElementDefinitionConstraintComponent src) throws FHIRException { 584 if (src == null) return null; 585 org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionConstraintComponent tgt = new org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionConstraintComponent(); 586 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt, org.hl7.fhir.r5.utils.ToolingExtensions.EXT_XPATH_CONSTRAINT); 587 if (src.hasKey()) tgt.setKeyElement(Id30_50.convertId(src.getKeyElement())); 588 if (src.hasRequirements()) tgt.setRequirementsElement(String30_50.convertString(src.getRequirementsElement())); 589 if (src.hasSeverity()) tgt.setSeverityElement(convertConstraintSeverity(src.getSeverityElement())); 590 if (src.hasHuman()) tgt.setHumanElement(String30_50.convertString(src.getHumanElement())); 591 if (src.hasExpression()) tgt.setExpressionElement(String30_50.convertString(src.getExpressionElement())); 592 if (org.hl7.fhir.r5.utils.ToolingExtensions.hasExtension(src, org.hl7.fhir.r5.utils.ToolingExtensions.EXT_XPATH_CONSTRAINT)) { 593 tgt.setXpath(org.hl7.fhir.r5.utils.ToolingExtensions.readStringExtension(src, org.hl7.fhir.r5.utils.ToolingExtensions.EXT_XPATH_CONSTRAINT)); 594 } 595 if (src.hasSource()) tgt.setSource(src.getSource()); 596 return tgt; 597 } 598 599 static public org.hl7.fhir.r5.model.Enumeration<ElementDefinition.ConstraintSeverity> convertConstraintSeverity(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ElementDefinition.ConstraintSeverity> src) throws FHIRException { 600 if (src == null || src.isEmpty()) return null; 601 org.hl7.fhir.r5.model.Enumeration<ElementDefinition.ConstraintSeverity> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new ElementDefinition.ConstraintSeverityEnumFactory()); 602 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 603 if (src.getValue() == null) { 604 tgt.setValue(ElementDefinition.ConstraintSeverity.NULL); 605 } else { 606 switch (src.getValue()) { 607 case ERROR: 608 tgt.setValue(ElementDefinition.ConstraintSeverity.ERROR); 609 break; 610 case WARNING: 611 tgt.setValue(ElementDefinition.ConstraintSeverity.WARNING); 612 break; 613 default: 614 tgt.setValue(ElementDefinition.ConstraintSeverity.NULL); 615 break; 616 } 617 } 618 return tgt; 619 } 620 621 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ElementDefinition.ConstraintSeverity> convertConstraintSeverity(org.hl7.fhir.r5.model.Enumeration<ElementDefinition.ConstraintSeverity> src) throws FHIRException { 622 if (src == null || src.isEmpty()) return null; 623 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ElementDefinition.ConstraintSeverity> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.ElementDefinition.ConstraintSeverityEnumFactory()); 624 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 625 if (src.getValue() == null) { 626 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.ConstraintSeverity.NULL); 627 } else { 628 switch (src.getValue()) { 629 case ERROR: 630 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.ConstraintSeverity.ERROR); 631 break; 632 case WARNING: 633 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.ConstraintSeverity.WARNING); 634 break; 635 default: 636 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.ConstraintSeverity.NULL); 637 break; 638 } 639 } 640 return tgt; 641 } 642 643 public static ElementDefinition.ElementDefinitionBindingComponent convertElementDefinitionBindingComponent(org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionBindingComponent src) throws FHIRException { 644 if (src == null) return null; 645 ElementDefinition.ElementDefinitionBindingComponent tgt = new ElementDefinition.ElementDefinitionBindingComponent(); 646 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt, VersionConvertor_30_50.EXT_SRC_TYPE); 647 if (src.hasStrength()) tgt.setStrengthElement(Enumerations30_50.convertBindingStrength(src.getStrengthElement())); 648 if (src.hasDescription()) tgt.setDescriptionElement(String30_50.convertStringToMarkdown(src.getDescriptionElement())); 649 if (src.hasValueSet()) { 650 DataType t = ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getValueSet()); 651 if (t instanceof org.hl7.fhir.r5.model.Reference) { 652 tgt.setValueSet(((org.hl7.fhir.r5.model.Reference) t).getReference()); 653 tgt.getValueSetElement().addExtension(VersionConvertor_30_50.EXT_SRC_TYPE, new org.hl7.fhir.r5.model.UrlType("Reference")); 654 } else { 655 tgt.setValueSet(t.primitiveValue()); 656 tgt.getValueSetElement().addExtension(VersionConvertor_30_50.EXT_SRC_TYPE, new org.hl7.fhir.r5.model.UrlType("uri")); 657 } 658 tgt.setValueSet(VersionConvertorConstants.refToVS(tgt.getValueSet())); 659 } 660 return tgt; 661 } 662 663 public static org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionBindingComponent convertElementDefinitionBindingComponent(ElementDefinition.ElementDefinitionBindingComponent src) throws FHIRException { 664 if (src == null) return null; 665 org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionBindingComponent tgt = new org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionBindingComponent(); 666 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt, VersionConvertor_30_50.EXT_SRC_TYPE); 667 if (src.hasStrength()) tgt.setStrengthElement(Enumerations30_50.convertBindingStrength(src.getStrengthElement())); 668 if (src.hasDescription()) tgt.setDescriptionElement(String30_50.convertString(src.getDescriptionElement())); 669 if (src.hasValueSet()) { 670 org.hl7.fhir.r5.model.Extension ex = src.getValueSetElement().getExtensionByUrl(VersionConvertor_30_50.EXT_SRC_TYPE); 671 String vsr = VersionConvertorConstants.vsToRef(src.getValueSet()); 672 if (ex != null) { 673 if ("uri".equals(ex.getValue().primitiveValue())) { 674 tgt.setValueSet(new org.hl7.fhir.dstu3.model.UriType(vsr == null ? src.getValueSet() : vsr)); 675 } else { 676 tgt.setValueSet(new org.hl7.fhir.dstu3.model.Reference(src.getValueSet())); 677 } 678 } else { 679 if (vsr != null) tgt.setValueSet(new org.hl7.fhir.dstu3.model.UriType(vsr)); 680 else tgt.setValueSet(new org.hl7.fhir.dstu3.model.Reference(src.getValueSet())); 681 } 682 } 683 return tgt; 684 } 685 686 public static ElementDefinition.ElementDefinitionMappingComponent convertElementDefinitionMappingComponent(org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionMappingComponent src) throws FHIRException { 687 if (src == null) return null; 688 ElementDefinition.ElementDefinitionMappingComponent tgt = new ElementDefinition.ElementDefinitionMappingComponent(); 689 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 690 if (src.hasIdentity()) tgt.setIdentityElement(Id30_50.convertId(src.getIdentityElement())); 691 if (src.hasLanguage()) tgt.setLanguageElement(Code30_50.convertCode(src.getLanguageElement())); 692 if (src.hasMap()) tgt.setMapElement(String30_50.convertString(src.getMapElement())); 693 if (src.hasComment()) tgt.setCommentElement(String30_50.convertStringToMarkdown(src.getCommentElement())); 694 return tgt; 695 } 696 697 public static org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionMappingComponent convertElementDefinitionMappingComponent(ElementDefinition.ElementDefinitionMappingComponent src) throws FHIRException { 698 if (src == null) return null; 699 org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionMappingComponent tgt = new org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionMappingComponent(); 700 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 701 if (src.hasIdentity()) tgt.setIdentityElement(Id30_50.convertId(src.getIdentityElement())); 702 if (src.hasLanguage()) tgt.setLanguageElement(Code30_50.convertCode(src.getLanguageElement())); 703 if (src.hasMap()) tgt.setMapElement(String30_50.convertString(src.getMapElement())); 704 if (src.hasComment()) tgt.setCommentElement(String30_50.convertString(src.getCommentElement())); 705 return tgt; 706 } 707}