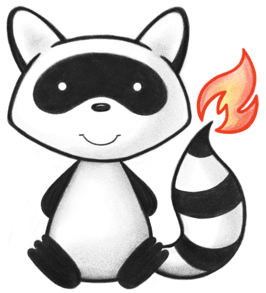
001package org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_50; 004import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Boolean30_50; 005import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Code30_50; 006import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.String30_50; 007import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Uri30_50; 008import org.hl7.fhir.exceptions.FHIRException; 009 010public class Coding30_50 { 011 public static org.hl7.fhir.r5.model.Coding convertCoding(org.hl7.fhir.dstu3.model.Coding src) throws FHIRException { 012 if (src == null) return null; 013 org.hl7.fhir.r5.model.Coding tgt = new org.hl7.fhir.r5.model.Coding(); 014 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 015 if (src.hasSystem()) tgt.setSystemElement(Uri30_50.convertUri(src.getSystemElement())); 016 if (src.hasVersion()) tgt.setVersionElement(String30_50.convertString(src.getVersionElement())); 017 if (src.hasCode()) tgt.setCodeElement(Code30_50.convertCode(src.getCodeElement())); 018 if (src.hasDisplay()) tgt.setDisplayElement(String30_50.convertString(src.getDisplayElement())); 019 if (src.hasUserSelected()) tgt.setUserSelectedElement(Boolean30_50.convertBoolean(src.getUserSelectedElement())); 020 return tgt; 021 } 022 023 public static org.hl7.fhir.r5.model.Coding convertCoding(org.hl7.fhir.dstu3.model.CodeType src) throws FHIRException { 024 if (src == null) return null; 025 org.hl7.fhir.r5.model.Coding tgt = new org.hl7.fhir.r5.model.Coding(); 026 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 027 tgt.setCode(src.getValue()); 028 return tgt; 029 } 030 031 public static org.hl7.fhir.r5.model.CodeableConcept convertCodingToCodeableConcept(org.hl7.fhir.dstu3.model.Coding src) throws FHIRException { 032 if (src == null) return null; 033 org.hl7.fhir.r5.model.CodeableConcept tgt = new org.hl7.fhir.r5.model.CodeableConcept(); 034 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 035 if (src.hasSystem()) tgt.getCodingFirstRep().setSystem(src.getSystem()); 036 if (src.hasVersion()) tgt.getCodingFirstRep().setVersion(src.getVersion()); 037 if (src.hasCode()) tgt.getCodingFirstRep().setCode(src.getCode()); 038 if (src.hasDisplay()) tgt.getCodingFirstRep().setDisplay(src.getDisplay()); 039 if (src.hasUserSelected()) tgt.getCodingFirstRep().setUserSelected(src.getUserSelected()); 040 return tgt; 041 } 042 043 public static org.hl7.fhir.r5.model.Coding convertCoding(org.hl7.fhir.dstu3.model.CodeableConcept src) throws FHIRException { 044 if (src == null) return null; 045 org.hl7.fhir.r5.model.Coding tgt = new org.hl7.fhir.r5.model.Coding(); 046 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 047 if (src.hasCoding()) { 048 if (src.getCodingFirstRep().hasSystem()) tgt.setSystem(src.getCodingFirstRep().getSystem()); 049 if (src.getCodingFirstRep().hasVersion()) tgt.setVersion(src.getCodingFirstRep().getVersion()); 050 if (src.getCodingFirstRep().hasCode()) tgt.setCode(src.getCodingFirstRep().getCode()); 051 if (src.getCodingFirstRep().hasDisplay()) tgt.setDisplay(src.getCodingFirstRep().getDisplay()); 052 if (src.getCodingFirstRep().hasUserSelected()) tgt.setUserSelected(src.getCodingFirstRep().getUserSelected()); 053 } 054 return tgt; 055 } 056 057 public static org.hl7.fhir.dstu3.model.Coding convertCoding(org.hl7.fhir.r5.model.CodeableConcept src) throws FHIRException { 058 if (src == null) return null; 059 org.hl7.fhir.dstu3.model.Coding tgt = new org.hl7.fhir.dstu3.model.Coding(); 060 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 061 if (src.hasCoding()) { 062 if (src.getCodingFirstRep().hasSystem()) tgt.setSystem(src.getCodingFirstRep().getSystem()); 063 if (src.getCodingFirstRep().hasVersion()) tgt.setVersion(src.getCodingFirstRep().getVersion()); 064 if (src.getCodingFirstRep().hasCode()) tgt.setCode(src.getCodingFirstRep().getCode()); 065 if (src.getCodingFirstRep().hasDisplay()) tgt.setDisplay(src.getCodingFirstRep().getDisplay()); 066 if (src.getCodingFirstRep().hasUserSelected()) tgt.setUserSelected(src.getCodingFirstRep().getUserSelected()); 067 } 068 return tgt; 069 } 070 071 public static org.hl7.fhir.dstu3.model.Coding convertCoding(org.hl7.fhir.r5.model.Coding src) throws FHIRException { 072 if (src == null) return null; 073 org.hl7.fhir.dstu3.model.Coding tgt = new org.hl7.fhir.dstu3.model.Coding(); 074 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 075 if (src.hasSystem()) tgt.setSystemElement(Uri30_50.convertUri(src.getSystemElement())); 076 if (src.hasVersion()) tgt.setVersionElement(String30_50.convertString(src.getVersionElement())); 077 if (src.hasCode()) tgt.setCodeElement(Code30_50.convertCode(src.getCodeElement())); 078 if (src.hasDisplay()) tgt.setDisplayElement(String30_50.convertString(src.getDisplayElement())); 079 if (src.hasUserSelected()) tgt.setUserSelectedElement(Boolean30_50.convertBoolean(src.getUserSelectedElement())); 080 return tgt; 081 } 082 083 public static String convertCoding2Uri(org.hl7.fhir.dstu3.model.Coding code) { 084 return code.getSystem() + "/" + code.getCode(); 085 } 086 087 public static org.hl7.fhir.dstu3.model.Coding convertUri2Coding(String uri) { 088 int i = uri.lastIndexOf("/"); 089 return new org.hl7.fhir.dstu3.model.Coding().setSystem(uri.substring(0, i)).setCode(uri.substring(i + 1)); 090 } 091}