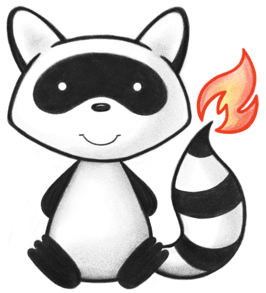
001package org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_50; 004import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Code30_50; 005import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Decimal30_50; 006import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.String30_50; 007import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Uri30_50; 008import org.hl7.fhir.dstu3.model.Quantity; 009import org.hl7.fhir.exceptions.FHIRException; 010import org.hl7.fhir.r5.model.Enumerations; 011 012public class Quantity30_50 { 013 public static void copyQuantity(org.hl7.fhir.dstu3.model.Quantity src, org.hl7.fhir.r5.model.Quantity tgt) throws FHIRException { 014 if (src == null || tgt == null) return; 015 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 016 if (src.hasValue()) tgt.setValue(src.getValue()); 017 if (src.hasComparator()) tgt.setComparatorElement(convertQuantityComparator(src.getComparatorElement())); 018 if (src.hasUnit()) tgt.setUnitElement(String30_50.convertString(src.getUnitElement())); 019 if (src.hasSystem()) tgt.setSystemElement(Uri30_50.convertUri(src.getSystemElement())); 020 if (src.hasCode()) tgt.setCodeElement(Code30_50.convertCode(src.getCodeElement())); 021 } 022 023 public static void copyQuantity(org.hl7.fhir.r5.model.Quantity src, org.hl7.fhir.dstu3.model.Quantity tgt) throws FHIRException { 024 if (src == null || tgt == null) return; 025 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 026 if (src.hasValue()) tgt.setValue(src.getValue()); 027 if (src.hasComparator()) tgt.setComparatorElement(convertQuantityComparator(src.getComparatorElement())); 028 if (src.hasUnit()) tgt.setUnitElement(String30_50.convertString(src.getUnitElement())); 029 if (src.hasSystem()) tgt.setSystemElement(Uri30_50.convertUri(src.getSystemElement())); 030 if (src.hasCode()) tgt.setCodeElement(Code30_50.convertCode(src.getCodeElement())); 031 } 032 033 public static org.hl7.fhir.r5.model.Quantity convertQuantity(org.hl7.fhir.dstu3.model.Quantity src) throws FHIRException { 034 if (src == null) return null; 035 org.hl7.fhir.r5.model.Quantity tgt = new org.hl7.fhir.r5.model.Quantity(); 036 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 037 if (src.hasValue()) tgt.setValueElement(Decimal30_50.convertDecimal(src.getValueElement())); 038 if (src.hasComparator()) tgt.setComparatorElement(convertQuantityComparator(src.getComparatorElement())); 039 if (src.hasUnit()) tgt.setUnitElement(String30_50.convertString(src.getUnitElement())); 040 if (src.hasSystem()) tgt.setSystemElement(Uri30_50.convertUri(src.getSystemElement())); 041 if (src.hasCode()) tgt.setCodeElement(Code30_50.convertCode(src.getCodeElement())); 042 return tgt; 043 } 044 045 public static org.hl7.fhir.dstu3.model.Quantity convertQuantity(org.hl7.fhir.r5.model.Quantity src) throws FHIRException { 046 if (src == null) return null; 047 org.hl7.fhir.dstu3.model.Quantity tgt = new org.hl7.fhir.dstu3.model.Quantity(); 048 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 049 if (src.hasValue()) tgt.setValueElement(Decimal30_50.convertDecimal(src.getValueElement())); 050 if (src.hasComparator()) tgt.setComparatorElement(convertQuantityComparator(src.getComparatorElement())); 051 if (src.hasUnit()) tgt.setUnitElement(String30_50.convertString(src.getUnitElement())); 052 if (src.hasSystem()) tgt.setSystemElement(Uri30_50.convertUri(src.getSystemElement())); 053 if (src.hasCode()) tgt.setCodeElement(Code30_50.convertCode(src.getCodeElement())); 054 return tgt; 055 } 056 057 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.QuantityComparator> convertQuantityComparator(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Quantity.QuantityComparator> src) throws FHIRException { 058 if (src == null || src.isEmpty()) return null; 059 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.QuantityComparator> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Enumerations.QuantityComparatorEnumFactory()); 060 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 061 if (src.getValue() == null) { 062 tgt.setValue(null); 063} else { 064 switch(src.getValue()) { 065 case LESS_THAN: 066 tgt.setValue(Enumerations.QuantityComparator.LESS_THAN); 067 break; 068 case LESS_OR_EQUAL: 069 tgt.setValue(Enumerations.QuantityComparator.LESS_OR_EQUAL); 070 break; 071 case GREATER_OR_EQUAL: 072 tgt.setValue(Enumerations.QuantityComparator.GREATER_OR_EQUAL); 073 break; 074 case GREATER_THAN: 075 tgt.setValue(Enumerations.QuantityComparator.GREATER_THAN); 076 break; 077 default: 078 tgt.setValue(Enumerations.QuantityComparator.NULL); 079 break; 080 } 081} 082 return tgt; 083 } 084 085 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Quantity.QuantityComparator> convertQuantityComparator(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.QuantityComparator> src) throws FHIRException { 086 if (src == null || src.isEmpty()) return null; 087 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Quantity.QuantityComparator> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Quantity.QuantityComparatorEnumFactory()); 088 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 089 if (src.getValue() == null) { 090 tgt.setValue(null); 091} else { 092 switch(src.getValue()) { 093 case LESS_THAN: 094 tgt.setValue(Quantity.QuantityComparator.LESS_THAN); 095 break; 096 case LESS_OR_EQUAL: 097 tgt.setValue(Quantity.QuantityComparator.LESS_OR_EQUAL); 098 break; 099 case GREATER_OR_EQUAL: 100 tgt.setValue(Quantity.QuantityComparator.GREATER_OR_EQUAL); 101 break; 102 case GREATER_THAN: 103 tgt.setValue(Quantity.QuantityComparator.GREATER_THAN); 104 break; 105 default: 106 tgt.setValue(Quantity.QuantityComparator.NULL); 107 break; 108 } 109} 110 return tgt; 111 } 112}