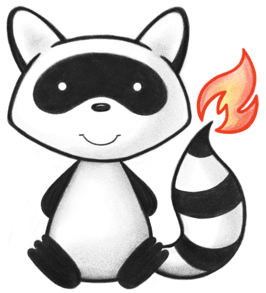
001package org.hl7.fhir.convertors.conv30_50.resources30_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_50; 004import org.hl7.fhir.convertors.conv30_50.datatypes30_50.Reference30_50; 005import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.CodeableConcept30_50; 006import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Identifier30_50; 007import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Period30_50; 008import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.DateTime30_50; 009import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Instant30_50; 010import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.PositiveInt30_50; 011import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.String30_50; 012import org.hl7.fhir.dstu3.model.Appointment; 013import org.hl7.fhir.dstu3.model.Enumeration; 014import org.hl7.fhir.dstu3.model.UnsignedIntType; 015import org.hl7.fhir.exceptions.FHIRException; 016import org.hl7.fhir.r5.model.BooleanType; 017import org.hl7.fhir.r5.model.CodeableConcept; 018import org.hl7.fhir.r5.model.CodeableReference; 019 020public class Appointment30_50 { 021 022 public static org.hl7.fhir.dstu3.model.Appointment convertAppointment(org.hl7.fhir.r5.model.Appointment src) throws FHIRException { 023 if (src == null) 024 return null; 025 org.hl7.fhir.dstu3.model.Appointment tgt = new org.hl7.fhir.dstu3.model.Appointment(); 026 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 027 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 028 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 029 if (src.hasStatus()) 030 tgt.setStatusElement(convertAppointmentStatus(src.getStatusElement())); 031 if (src.hasServiceCategory()) 032 tgt.setServiceCategory(CodeableConcept30_50.convertCodeableConcept(src.getServiceCategoryFirstRep())); 033 for (org.hl7.fhir.r5.model.CodeableReference t : src.getServiceType()) 034 tgt.addServiceType(CodeableConcept30_50.convertCodeableConcept(t.getConcept())); 035 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getSpecialty()) 036 tgt.addSpecialty(CodeableConcept30_50.convertCodeableConcept(t)); 037 if (src.hasAppointmentType()) 038 tgt.setAppointmentType(CodeableConcept30_50.convertCodeableConcept(src.getAppointmentType())); 039 for (CodeableReference t : src.getReason()) 040 if (t.hasConcept()) 041 tgt.addReason(CodeableConcept30_50.convertCodeableConcept(t.getConcept())); 042 for (CodeableReference t : src.getReason()) 043 if (t.hasReference()) 044 tgt.addIndication(Reference30_50.convertReference(t.getReference())); 045 if (src.hasPriority()) 046 tgt.setPriorityElement(convertAppointmentPriority(src.getPriority())); 047 if (src.hasDescription()) 048 tgt.setDescriptionElement(String30_50.convertString(src.getDescriptionElement())); 049 for (org.hl7.fhir.r5.model.Reference t : src.getSupportingInformation()) 050 tgt.addSupportingInformation(Reference30_50.convertReference(t)); 051 if (src.hasStart()) 052 tgt.setStartElement(Instant30_50.convertInstant(src.getStartElement())); 053 if (src.hasEnd()) 054 tgt.setEndElement(Instant30_50.convertInstant(src.getEndElement())); 055 if (src.hasMinutesDuration()) 056 tgt.setMinutesDurationElement(PositiveInt30_50.convertPositiveInt(src.getMinutesDurationElement())); 057 for (org.hl7.fhir.r5.model.Reference t : src.getSlot()) tgt.addSlot(Reference30_50.convertReference(t)); 058 if (src.hasCreated()) 059 tgt.setCreatedElement(DateTime30_50.convertDateTime(src.getCreatedElement())); 060 if (src.hasNote()) 061 tgt.setCommentElement(String30_50.convertString(src.getNoteFirstRep().getTextElement())); 062 for (org.hl7.fhir.r5.model.Reference t : src.getBasedOn()) 063 tgt.addIncomingReferral(Reference30_50.convertReference(t)); 064 for (org.hl7.fhir.r5.model.Appointment.AppointmentParticipantComponent t : src.getParticipant()) 065 tgt.addParticipant(convertAppointmentParticipantComponent(t)); 066 for (org.hl7.fhir.r5.model.Period t : src.getRequestedPeriod()) 067 tgt.addRequestedPeriod(Period30_50.convertPeriod(t)); 068 return tgt; 069 } 070 071 072 private static UnsignedIntType convertAppointmentPriority(CodeableConcept src) { 073 UnsignedIntType tgt = new UnsignedIntType(convertAppointmentPriorityFromR5(src)); 074 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 075 return tgt; 076 } 077 078 private static CodeableConcept convertAppointmentPriority(UnsignedIntType src) { 079 CodeableConcept tgt = src.hasValue() ? convertAppointmentPriorityToR5(src.getValue()) : new CodeableConcept(); 080 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 081 return tgt; 082 } 083 084 public static org.hl7.fhir.r5.model.CodeableConcept convertAppointmentPriorityToR5(int priority) { 085 return null; 086 } 087 088 public static int convertAppointmentPriorityFromR5(org.hl7.fhir.r5.model.CodeableConcept priority) { 089 return 0; 090 } 091 092 public static org.hl7.fhir.r5.model.Appointment convertAppointment(org.hl7.fhir.dstu3.model.Appointment src) throws FHIRException { 093 if (src == null) 094 return null; 095 org.hl7.fhir.r5.model.Appointment tgt = new org.hl7.fhir.r5.model.Appointment(); 096 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 097 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 098 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 099 if (src.hasStatus()) 100 tgt.setStatusElement(convertAppointmentStatus(src.getStatusElement())); 101 if (src.hasServiceCategory()) 102 tgt.addServiceCategory(CodeableConcept30_50.convertCodeableConcept(src.getServiceCategory())); 103 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getServiceType()) 104 tgt.addServiceType().setConcept(CodeableConcept30_50.convertCodeableConcept(t)); 105 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getSpecialty()) 106 tgt.addSpecialty(CodeableConcept30_50.convertCodeableConcept(t)); 107 if (src.hasAppointmentType()) 108 tgt.setAppointmentType(CodeableConcept30_50.convertCodeableConcept(src.getAppointmentType())); 109 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getReason()) 110 tgt.addReason(Reference30_50.convertCodeableConceptToCodableReference(t)); 111 for (org.hl7.fhir.dstu3.model.Reference t : src.getIndication()) 112 tgt.addReason(Reference30_50.convertReferenceToCodableReference(t)); 113 if (src.hasPriority()) 114 tgt.setPriority(convertAppointmentPriority(src.getPriorityElement())); 115 if (src.hasDescription()) 116 tgt.setDescriptionElement(String30_50.convertString(src.getDescriptionElement())); 117 for (org.hl7.fhir.dstu3.model.Reference t : src.getSupportingInformation()) 118 tgt.addSupportingInformation(Reference30_50.convertReference(t)); 119 if (src.hasStart()) 120 tgt.setStartElement(Instant30_50.convertInstant(src.getStartElement())); 121 if (src.hasEnd()) 122 tgt.setEndElement(Instant30_50.convertInstant(src.getEndElement())); 123 if (src.hasMinutesDuration()) 124 tgt.setMinutesDurationElement(PositiveInt30_50.convertPositiveInt(src.getMinutesDurationElement())); 125 for (org.hl7.fhir.dstu3.model.Reference t : src.getSlot()) tgt.addSlot(Reference30_50.convertReference(t)); 126 if (src.hasCreated()) 127 tgt.setCreatedElement(DateTime30_50.convertDateTime(src.getCreatedElement())); 128 if (src.hasComment()) 129 tgt.getNoteFirstRep().setTextElement(String30_50.convertStringToMarkdown(src.getCommentElement())); 130 for (org.hl7.fhir.dstu3.model.Reference t : src.getIncomingReferral()) 131 tgt.addBasedOn(Reference30_50.convertReference(t)); 132 for (org.hl7.fhir.dstu3.model.Appointment.AppointmentParticipantComponent t : src.getParticipant()) 133 tgt.addParticipant(convertAppointmentParticipantComponent(t)); 134 for (org.hl7.fhir.dstu3.model.Period t : src.getRequestedPeriod()) 135 tgt.addRequestedPeriod(Period30_50.convertPeriod(t)); 136 return tgt; 137 } 138 139 public static org.hl7.fhir.dstu3.model.Appointment.AppointmentParticipantComponent convertAppointmentParticipantComponent(org.hl7.fhir.r5.model.Appointment.AppointmentParticipantComponent src) throws FHIRException { 140 if (src == null) 141 return null; 142 org.hl7.fhir.dstu3.model.Appointment.AppointmentParticipantComponent tgt = new org.hl7.fhir.dstu3.model.Appointment.AppointmentParticipantComponent(); 143 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 144 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getType()) 145 tgt.addType(CodeableConcept30_50.convertCodeableConcept(t)); 146 if (src.hasActor()) 147 tgt.setActor(Reference30_50.convertReference(src.getActor())); 148 if (src.hasRequired()) 149 tgt.setRequiredElement(convertParticipantRequired(src.getRequiredElement())); 150 if (src.hasStatus()) 151 tgt.setStatusElement(convertParticipationStatus(src.getStatusElement())); 152 return tgt; 153 } 154 155 public static org.hl7.fhir.r5.model.Appointment.AppointmentParticipantComponent convertAppointmentParticipantComponent(org.hl7.fhir.dstu3.model.Appointment.AppointmentParticipantComponent src) throws FHIRException { 156 if (src == null) 157 return null; 158 org.hl7.fhir.r5.model.Appointment.AppointmentParticipantComponent tgt = new org.hl7.fhir.r5.model.Appointment.AppointmentParticipantComponent(); 159 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 160 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getType()) 161 tgt.addType(CodeableConcept30_50.convertCodeableConcept(t)); 162 if (src.hasActor()) 163 tgt.setActor(Reference30_50.convertReference(src.getActor())); 164 if (src.hasRequired()) 165 tgt.setRequiredElement(convertParticipantRequired(src.getRequiredElement())); 166 if (src.hasStatus()) 167 tgt.setStatusElement(convertParticipationStatus(src.getStatusElement())); 168 return tgt; 169 } 170 171 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Appointment.AppointmentStatus> convertAppointmentStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Appointment.AppointmentStatus> src) throws FHIRException { 172 if (src == null || src.isEmpty()) 173 return null; 174 Enumeration<Appointment.AppointmentStatus> tgt = new Enumeration<>(new Appointment.AppointmentStatusEnumFactory()); 175 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 176 if (src.getValue() == null) { 177 tgt.setValue(null); 178 } else { 179 switch (src.getValue()) { 180 case PROPOSED: 181 tgt.setValue(Appointment.AppointmentStatus.PROPOSED); 182 break; 183 case PENDING: 184 tgt.setValue(Appointment.AppointmentStatus.PENDING); 185 break; 186 case BOOKED: 187 tgt.setValue(Appointment.AppointmentStatus.BOOKED); 188 break; 189 case ARRIVED: 190 tgt.setValue(Appointment.AppointmentStatus.ARRIVED); 191 break; 192 case FULFILLED: 193 tgt.setValue(Appointment.AppointmentStatus.FULFILLED); 194 break; 195 case CANCELLED: 196 tgt.setValue(Appointment.AppointmentStatus.CANCELLED); 197 break; 198 case NOSHOW: 199 tgt.setValue(Appointment.AppointmentStatus.NOSHOW); 200 break; 201 case ENTEREDINERROR: 202 tgt.setValue(Appointment.AppointmentStatus.ENTEREDINERROR); 203 break; 204 default: 205 tgt.setValue(Appointment.AppointmentStatus.NULL); 206 break; 207 } 208 } 209 return tgt; 210 } 211 212 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Appointment.AppointmentStatus> convertAppointmentStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Appointment.AppointmentStatus> src) throws FHIRException { 213 if (src == null || src.isEmpty()) 214 return null; 215 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Appointment.AppointmentStatus> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Appointment.AppointmentStatusEnumFactory()); 216 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 217 if (src.getValue() == null) { 218 tgt.setValue(null); 219 } else { 220 switch (src.getValue()) { 221 case PROPOSED: 222 tgt.setValue(org.hl7.fhir.r5.model.Appointment.AppointmentStatus.PROPOSED); 223 break; 224 case PENDING: 225 tgt.setValue(org.hl7.fhir.r5.model.Appointment.AppointmentStatus.PENDING); 226 break; 227 case BOOKED: 228 tgt.setValue(org.hl7.fhir.r5.model.Appointment.AppointmentStatus.BOOKED); 229 break; 230 case ARRIVED: 231 tgt.setValue(org.hl7.fhir.r5.model.Appointment.AppointmentStatus.ARRIVED); 232 break; 233 case FULFILLED: 234 tgt.setValue(org.hl7.fhir.r5.model.Appointment.AppointmentStatus.FULFILLED); 235 break; 236 case CANCELLED: 237 tgt.setValue(org.hl7.fhir.r5.model.Appointment.AppointmentStatus.CANCELLED); 238 break; 239 case NOSHOW: 240 tgt.setValue(org.hl7.fhir.r5.model.Appointment.AppointmentStatus.NOSHOW); 241 break; 242 case ENTEREDINERROR: 243 tgt.setValue(org.hl7.fhir.r5.model.Appointment.AppointmentStatus.ENTEREDINERROR); 244 break; 245 default: 246 tgt.setValue(org.hl7.fhir.r5.model.Appointment.AppointmentStatus.NULL); 247 break; 248 } 249 } 250 return tgt; 251 } 252 253 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Appointment.ParticipantRequired> convertParticipantRequired(org.hl7.fhir.r5.model.BooleanType src) throws FHIRException { 254 if (src == null || src.isEmpty()) 255 return null; 256 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Appointment.ParticipantRequired> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Appointment.ParticipantRequiredEnumFactory()); 257 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 258 if (src.getValue()) { // case REQUIRED: 259 tgt.setValue(org.hl7.fhir.dstu3.model.Appointment.ParticipantRequired.REQUIRED); 260 } else { // case OPTIONAL and others: 261 tgt.setValue(org.hl7.fhir.dstu3.model.Appointment.ParticipantRequired.OPTIONAL); 262 } 263 return tgt; 264 } 265 266 static public org.hl7.fhir.r5.model.BooleanType convertParticipantRequired(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Appointment.ParticipantRequired> src) throws FHIRException { 267 if (src == null || src.isEmpty()) 268 return null; 269 BooleanType tgt = new BooleanType(); 270 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 271 if (src.getValue() == null) { 272 tgt.setValue(null); 273 } else { 274 switch (src.getValue()) { 275 case REQUIRED: 276 tgt.setValue(true); 277 break; 278 case OPTIONAL: 279 tgt.setValue(false); 280 break; 281 case INFORMATIONONLY: 282 tgt.setValue(false); 283 break; 284 default: 285 break; 286 } 287 } 288 return tgt; 289 } 290 291 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Appointment.ParticipationStatus> convertParticipationStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Appointment.ParticipationStatus> src) throws FHIRException { 292 if (src == null || src.isEmpty()) 293 return null; 294 Enumeration<Appointment.ParticipationStatus> tgt = new Enumeration<>(new Appointment.ParticipationStatusEnumFactory()); 295 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 296 if (src.getValue() == null) { 297 tgt.setValue(null); 298 } else { 299 switch (src.getValue()) { 300 case ACCEPTED: 301 tgt.setValue(Appointment.ParticipationStatus.ACCEPTED); 302 break; 303 case DECLINED: 304 tgt.setValue(Appointment.ParticipationStatus.DECLINED); 305 break; 306 case TENTATIVE: 307 tgt.setValue(Appointment.ParticipationStatus.TENTATIVE); 308 break; 309 case NEEDSACTION: 310 tgt.setValue(Appointment.ParticipationStatus.NEEDSACTION); 311 break; 312 default: 313 tgt.setValue(Appointment.ParticipationStatus.NULL); 314 break; 315 } 316 } 317 return tgt; 318 } 319 320 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Appointment.ParticipationStatus> convertParticipationStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Appointment.ParticipationStatus> src) throws FHIRException { 321 if (src == null || src.isEmpty()) 322 return null; 323 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Appointment.ParticipationStatus> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Appointment.ParticipationStatusEnumFactory()); 324 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 325 if (src.getValue() == null) { 326 tgt.setValue(null); 327 } else { 328 switch (src.getValue()) { 329 case ACCEPTED: 330 tgt.setValue(org.hl7.fhir.r5.model.Appointment.ParticipationStatus.ACCEPTED); 331 break; 332 case DECLINED: 333 tgt.setValue(org.hl7.fhir.r5.model.Appointment.ParticipationStatus.DECLINED); 334 break; 335 case TENTATIVE: 336 tgt.setValue(org.hl7.fhir.r5.model.Appointment.ParticipationStatus.TENTATIVE); 337 break; 338 case NEEDSACTION: 339 tgt.setValue(org.hl7.fhir.r5.model.Appointment.ParticipationStatus.NEEDSACTION); 340 break; 341 default: 342 tgt.setValue(org.hl7.fhir.r5.model.Appointment.ParticipationStatus.NULL); 343 break; 344 } 345 } 346 return tgt; 347 } 348}