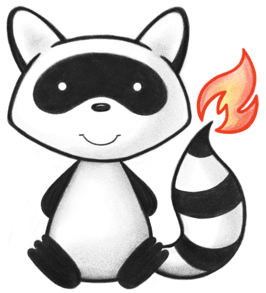
001package org.hl7.fhir.convertors.conv30_50.resources30_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_50; 004import org.hl7.fhir.convertors.conv30_50.datatypes30_50.Reference30_50; 005import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.CodeableConcept30_50; 006import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Coding30_50; 007import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Identifier30_50; 008import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Base64Binary30_50; 009import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Boolean30_50; 010import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Instant30_50; 011import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.String30_50; 012import org.hl7.fhir.exceptions.FHIRException; 013import org.hl7.fhir.r5.model.CodeableConcept; 014 015public class AuditEvent30_50 { 016 017 public static org.hl7.fhir.r5.model.AuditEvent convertAuditEvent(org.hl7.fhir.dstu3.model.AuditEvent src) throws FHIRException { 018 if (src == null) 019 return null; 020 org.hl7.fhir.r5.model.AuditEvent tgt = new org.hl7.fhir.r5.model.AuditEvent(); 021 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 022 if (src.hasType()) 023 tgt.getCategoryFirstRep().addCoding(Coding30_50.convertCoding(src.getType())); 024 for (org.hl7.fhir.dstu3.model.Coding t : src.getSubtype()) tgt.getCode().addCoding(Coding30_50.convertCoding(t)); 025 if (src.hasAction()) 026 tgt.setActionElement(convertAuditEventAction(src.getActionElement())); 027 if (src.hasRecorded()) 028 tgt.setRecordedElement(Instant30_50.convertInstant(src.getRecordedElement())); 029 if (src.hasOutcome()) 030 tgt.getOutcome().getCode().setSystem("http://terminology.hl7.org/CodeSystem/audit-event-outcome").setCode(src.getOutcome().toCode()); 031 if (src.hasOutcomeDesc()) 032 tgt.getOutcome().getDetailFirstRep().setTextElement(String30_50.convertString(src.getOutcomeDescElement())); 033 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getPurposeOfEvent()) 034 tgt.addAuthorization(CodeableConcept30_50.convertCodeableConcept(t)); 035 for (org.hl7.fhir.dstu3.model.AuditEvent.AuditEventAgentComponent t : src.getAgent()) 036 tgt.addAgent(convertAuditEventAgentComponent(t)); 037 if (src.hasSource()) 038 tgt.setSource(convertAuditEventSourceComponent(src.getSource())); 039 for (org.hl7.fhir.dstu3.model.AuditEvent.AuditEventEntityComponent t : src.getEntity()) 040 tgt.addEntity(convertAuditEventEntityComponent(t)); 041 return tgt; 042 } 043 044 public static org.hl7.fhir.dstu3.model.AuditEvent convertAuditEvent(org.hl7.fhir.r5.model.AuditEvent src) throws FHIRException { 045 if (src == null) 046 return null; 047 org.hl7.fhir.dstu3.model.AuditEvent tgt = new org.hl7.fhir.dstu3.model.AuditEvent(); 048 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 049 if (src.getCategoryFirstRep().hasCoding()) { 050 tgt.setType(Coding30_50.convertCoding(src.getCategoryFirstRep().getCodingFirstRep())); 051 } 052 for (org.hl7.fhir.r5.model.Coding t : src.getCode().getCoding()) tgt.addSubtype(Coding30_50.convertCoding(t)); 053 if (src.hasAction()) 054 tgt.setActionElement(convertAuditEventAction(src.getActionElement())); 055 if (src.hasRecorded()) 056 tgt.setRecordedElement(Instant30_50.convertInstant(src.getRecordedElement())); 057 if (src.hasOutcome() && "http://terminology.hl7.org/CodeSystem/audit-event-outcome".equals(src.getOutcome().getCode().getSystem())) 058 tgt.getOutcomeElement().setValueAsString(src.getOutcome().getCode().getCode()); 059 if (src.getOutcome().getDetailFirstRep().hasText()) 060 tgt.setOutcomeDescElement(String30_50.convertString(src.getOutcome().getDetailFirstRep().getTextElement())); 061 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getAuthorization()) 062 tgt.addPurposeOfEvent(CodeableConcept30_50.convertCodeableConcept(t)); 063 for (org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentComponent t : src.getAgent()) 064 tgt.addAgent(convertAuditEventAgentComponent(t)); 065 if (src.hasSource()) 066 tgt.setSource(convertAuditEventSourceComponent(src.getSource())); 067 for (org.hl7.fhir.r5.model.AuditEvent.AuditEventEntityComponent t : src.getEntity()) 068 tgt.addEntity(convertAuditEventEntityComponent(t)); 069 return tgt; 070 } 071 072 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.AuditEvent.AuditEventAction> convertAuditEventAction(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.AuditEvent.AuditEventAction> src) throws FHIRException { 073 if (src == null || src.isEmpty()) 074 return null; 075 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.AuditEvent.AuditEventAction> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.AuditEvent.AuditEventActionEnumFactory()); 076 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 077 switch (src.getValue()) { 078 case C: 079 tgt.setValue(org.hl7.fhir.r5.model.AuditEvent.AuditEventAction.C); 080 break; 081 case R: 082 tgt.setValue(org.hl7.fhir.r5.model.AuditEvent.AuditEventAction.R); 083 break; 084 case U: 085 tgt.setValue(org.hl7.fhir.r5.model.AuditEvent.AuditEventAction.U); 086 break; 087 case D: 088 tgt.setValue(org.hl7.fhir.r5.model.AuditEvent.AuditEventAction.D); 089 break; 090 case E: 091 tgt.setValue(org.hl7.fhir.r5.model.AuditEvent.AuditEventAction.E); 092 break; 093 default: 094 tgt.setValue(org.hl7.fhir.r5.model.AuditEvent.AuditEventAction.NULL); 095 break; 096 } 097 return tgt; 098 } 099 100 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.AuditEvent.AuditEventAction> convertAuditEventAction(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.AuditEvent.AuditEventAction> src) throws FHIRException { 101 if (src == null || src.isEmpty()) 102 return null; 103 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.AuditEvent.AuditEventAction> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.AuditEvent.AuditEventActionEnumFactory()); 104 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 105 switch (src.getValue()) { 106 case C: 107 tgt.setValue(org.hl7.fhir.dstu3.model.AuditEvent.AuditEventAction.C); 108 break; 109 case R: 110 tgt.setValue(org.hl7.fhir.dstu3.model.AuditEvent.AuditEventAction.R); 111 break; 112 case U: 113 tgt.setValue(org.hl7.fhir.dstu3.model.AuditEvent.AuditEventAction.U); 114 break; 115 case D: 116 tgt.setValue(org.hl7.fhir.dstu3.model.AuditEvent.AuditEventAction.D); 117 break; 118 case E: 119 tgt.setValue(org.hl7.fhir.dstu3.model.AuditEvent.AuditEventAction.E); 120 break; 121 default: 122 tgt.setValue(org.hl7.fhir.dstu3.model.AuditEvent.AuditEventAction.NULL); 123 break; 124 } 125 return tgt; 126 } 127 128 public static org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentComponent convertAuditEventAgentComponent(org.hl7.fhir.dstu3.model.AuditEvent.AuditEventAgentComponent src) throws FHIRException { 129 if (src == null) 130 return null; 131 org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentComponent tgt = new org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentComponent(); 132 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 133 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getRole()) 134 tgt.addRole(CodeableConcept30_50.convertCodeableConcept(t)); 135 if (src.hasReference()) 136 tgt.setWho(Reference30_50.convertReference(src.getReference())); 137 if (src.hasUserId()) 138 tgt.getWho().setIdentifier(Identifier30_50.convertIdentifier(src.getUserId())); 139// if (src.hasAltId()) 140// tgt.setAltIdElement(String30_50.convertString(src.getAltIdElement())); 141// if (src.hasName()) 142// tgt.setNameElement(String30_50.convertString(src.getNameElement())); 143 if (src.hasRequestor()) 144 tgt.setRequestorElement(Boolean30_50.convertBoolean(src.getRequestorElement())); 145 if (src.hasLocation()) 146 tgt.setLocation(Reference30_50.convertReference(src.getLocation())); 147 for (org.hl7.fhir.dstu3.model.UriType t : src.getPolicy()) tgt.addPolicy(t.getValue()); 148// if (src.hasMedia()) 149// tgt.setMedia(Coding30_50.convertCoding(src.getMedia())); 150// if (src.hasNetwork()) 151// tgt.setNetwork(convertAuditEventAgentNetworkComponent(src.getNetwork())); 152 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getPurposeOfUse()) 153 tgt.addAuthorization(CodeableConcept30_50.convertCodeableConcept(t)); 154 return tgt; 155 } 156 157 public static org.hl7.fhir.dstu3.model.AuditEvent.AuditEventAgentComponent convertAuditEventAgentComponent(org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentComponent src) throws FHIRException { 158 if (src == null) 159 return null; 160 org.hl7.fhir.dstu3.model.AuditEvent.AuditEventAgentComponent tgt = new org.hl7.fhir.dstu3.model.AuditEvent.AuditEventAgentComponent(); 161 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 162 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getRole()) 163 tgt.addRole(CodeableConcept30_50.convertCodeableConcept(t)); 164 if (src.hasWho()) { 165 if (src.getWho().hasIdentifier()) 166 tgt.setUserId(Identifier30_50.convertIdentifier(src.getWho().getIdentifier())); 167 if (src.getWho().hasReference() || src.getWho().hasDisplay() || src.getWho().hasExtension() || src.getWho().hasId()) 168 tgt.setReference(Reference30_50.convertReference(src.getWho())); 169 } 170// if (src.hasAltId()) 171// tgt.setAltIdElement(String30_50.convertString(src.getAltIdElement())); 172// if (src.hasName()) 173// tgt.setNameElement(String30_50.convertString(src.getNameElement())); 174 if (src.hasRequestor()) 175 tgt.setRequestorElement(Boolean30_50.convertBoolean(src.getRequestorElement())); 176 if (src.hasLocation()) 177 tgt.setLocation(Reference30_50.convertReference(src.getLocation())); 178 for (org.hl7.fhir.r5.model.UriType t : src.getPolicy()) tgt.addPolicy(t.getValue()); 179// if (src.hasMedia()) 180// tgt.setMedia(Coding30_50.convertCoding(src.getMedia())); 181// if (src.hasNetwork()) 182// tgt.setNetwork(convertAuditEventAgentNetworkComponent(src.getNetwork())); 183 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getAuthorization()) 184 tgt.addPurposeOfUse(CodeableConcept30_50.convertCodeableConcept(t)); 185 return tgt; 186 } 187// 188// public static org.hl7.fhir.dstu3.model.AuditEvent.AuditEventAgentNetworkComponent convertAuditEventAgentNetworkComponent(org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentNetworkComponent src) throws FHIRException { 189// if (src == null) 190// return null; 191// org.hl7.fhir.dstu3.model.AuditEvent.AuditEventAgentNetworkComponent tgt = new org.hl7.fhir.dstu3.model.AuditEvent.AuditEventAgentNetworkComponent(); 192// ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 193// if (src.hasAddress()) 194// tgt.setAddressElement(String30_50.convertString(src.getAddressElement())); 195// if (src.hasType()) 196// tgt.setTypeElement(convertAuditEventAgentNetworkType(src.getTypeElement())); 197// return tgt; 198// } 199 200// public static org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentNetworkComponent convertAuditEventAgentNetworkComponent(org.hl7.fhir.dstu3.model.AuditEvent.AuditEventAgentNetworkComponent src) throws FHIRException { 201// if (src == null) 202// return null; 203// org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentNetworkComponent tgt = new org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentNetworkComponent(); 204// ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 205// if (src.hasAddress()) 206// tgt.setAddressElement(String30_50.convertString(src.getAddressElement())); 207// if (src.hasType()) 208// tgt.setTypeElement(convertAuditEventAgentNetworkType(src.getTypeElement())); 209// return tgt; 210// } 211// 212// static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentNetworkType> convertAuditEventAgentNetworkType(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.AuditEvent.AuditEventAgentNetworkType> src) throws FHIRException { 213// if (src == null || src.isEmpty()) 214// return null; 215// org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentNetworkType> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentNetworkTypeEnumFactory()); 216// ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 217// switch (src.getValue()) { 218// case _1: 219// tgt.setValue(org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentNetworkType._1); 220// break; 221// case _2: 222// tgt.setValue(org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentNetworkType._2); 223// break; 224// case _3: 225// tgt.setValue(org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentNetworkType._3); 226// break; 227// case _4: 228// tgt.setValue(org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentNetworkType._4); 229// break; 230// case _5: 231// tgt.setValue(org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentNetworkType._5); 232// break; 233// default: 234// tgt.setValue(org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentNetworkType.NULL); 235// break; 236// } 237// return tgt; 238// } 239// 240// static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.AuditEvent.AuditEventAgentNetworkType> convertAuditEventAgentNetworkType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentNetworkType> src) throws FHIRException { 241// if (src == null || src.isEmpty()) 242// return null; 243// org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.AuditEvent.AuditEventAgentNetworkType> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.AuditEvent.AuditEventAgentNetworkTypeEnumFactory()); 244// ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 245// switch (src.getValue()) { 246// case _1: 247// tgt.setValue(org.hl7.fhir.dstu3.model.AuditEvent.AuditEventAgentNetworkType._1); 248// break; 249// case _2: 250// tgt.setValue(org.hl7.fhir.dstu3.model.AuditEvent.AuditEventAgentNetworkType._2); 251// break; 252// case _3: 253// tgt.setValue(org.hl7.fhir.dstu3.model.AuditEvent.AuditEventAgentNetworkType._3); 254// break; 255// case _4: 256// tgt.setValue(org.hl7.fhir.dstu3.model.AuditEvent.AuditEventAgentNetworkType._4); 257// break; 258// case _5: 259// tgt.setValue(org.hl7.fhir.dstu3.model.AuditEvent.AuditEventAgentNetworkType._5); 260// break; 261// default: 262// tgt.setValue(org.hl7.fhir.dstu3.model.AuditEvent.AuditEventAgentNetworkType.NULL); 263// break; 264// } 265// return tgt; 266// } 267// 268 public static org.hl7.fhir.r5.model.AuditEvent.AuditEventEntityComponent convertAuditEventEntityComponent(org.hl7.fhir.dstu3.model.AuditEvent.AuditEventEntityComponent src) throws FHIRException { 269 if (src == null) 270 return null; 271 org.hl7.fhir.r5.model.AuditEvent.AuditEventEntityComponent tgt = new org.hl7.fhir.r5.model.AuditEvent.AuditEventEntityComponent(); 272 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 273 if (src.hasIdentifier()) 274 tgt.getWhat().setIdentifier(Identifier30_50.convertIdentifier(src.getIdentifier())); 275 if (src.hasReference()) 276 tgt.setWhat(Reference30_50.convertReference(src.getReference())); 277// if (src.hasType()) 278// tgt.setType(Coding30_50.convertCoding(src.getType())); 279 if (src.hasRole()) 280 tgt.getRole().addCoding(Coding30_50.convertCoding(src.getRole())); 281// if (src.hasLifecycle()) 282// tgt.setLifecycle(Coding30_50.convertCoding(src.getLifecycle())); 283 for (org.hl7.fhir.dstu3.model.Coding t : src.getSecurityLabel()) tgt.addSecurityLabel().addCoding(Coding30_50.convertCoding(t)); 284// if (src.hasName()) 285// tgt.setNameElement(String30_50.convertString(src.getNameElement())); 286 if (src.hasQuery()) 287 tgt.setQueryElement(Base64Binary30_50.convertBase64Binary(src.getQueryElement())); 288 for (org.hl7.fhir.dstu3.model.AuditEvent.AuditEventEntityDetailComponent t : src.getDetail()) 289 tgt.addDetail(convertAuditEventEntityDetailComponent(t)); 290 return tgt; 291 } 292 293 public static org.hl7.fhir.dstu3.model.AuditEvent.AuditEventEntityComponent convertAuditEventEntityComponent(org.hl7.fhir.r5.model.AuditEvent.AuditEventEntityComponent src) throws FHIRException { 294 if (src == null) 295 return null; 296 org.hl7.fhir.dstu3.model.AuditEvent.AuditEventEntityComponent tgt = new org.hl7.fhir.dstu3.model.AuditEvent.AuditEventEntityComponent(); 297 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 298 if (src.hasWhat()) { 299 if (src.getWhat().hasIdentifier()) 300 tgt.setIdentifier(Identifier30_50.convertIdentifier(src.getWhat().getIdentifier())); 301 if (src.getWhat().hasReference() || src.getWhat().hasDisplay() || src.getWhat().hasExtension() || src.getWhat().hasId()) 302 tgt.setReference(Reference30_50.convertReference(src.getWhat())); 303 } 304// if (src.hasType()) 305// tgt.setType(Coding30_50.convertCoding(src.getType())); 306 if (src.hasRole()) 307 tgt.setRole(Coding30_50.convertCoding(src.getRole())); 308// if (src.hasLifecycle()) 309// tgt.setLifecycle(Coding30_50.convertCoding(src.getLifecycle())); 310 for (CodeableConcept t : src.getSecurityLabel()) tgt.addSecurityLabel(Coding30_50.convertCoding(t.getCodingFirstRep())); 311// if (src.hasName()) 312// tgt.setNameElement(String30_50.convertString(src.getNameElement())); 313 if (src.hasQuery()) 314 tgt.setQueryElement(Base64Binary30_50.convertBase64Binary(src.getQueryElement())); 315 for (org.hl7.fhir.r5.model.AuditEvent.AuditEventEntityDetailComponent t : src.getDetail()) 316 tgt.addDetail(convertAuditEventEntityDetailComponent(t)); 317 return tgt; 318 } 319 320 public static org.hl7.fhir.dstu3.model.AuditEvent.AuditEventEntityDetailComponent convertAuditEventEntityDetailComponent(org.hl7.fhir.r5.model.AuditEvent.AuditEventEntityDetailComponent src) throws FHIRException { 321 if (src == null) 322 return null; 323 org.hl7.fhir.dstu3.model.AuditEvent.AuditEventEntityDetailComponent tgt = new org.hl7.fhir.dstu3.model.AuditEvent.AuditEventEntityDetailComponent(); 324 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 325 if (src.getType().hasTextElement()) 326 tgt.setTypeElement(String30_50.convertString(src.getType().getTextElement())); 327 if (src.hasValueStringType()) 328 tgt.setValue(src.getValueStringType().getValue().getBytes()); 329 else if (src.hasValueBase64BinaryType()) 330 tgt.setValue(src.getValueBase64BinaryType().getValue()); 331 return tgt; 332 } 333 334 public static org.hl7.fhir.r5.model.AuditEvent.AuditEventEntityDetailComponent convertAuditEventEntityDetailComponent(org.hl7.fhir.dstu3.model.AuditEvent.AuditEventEntityDetailComponent src) throws FHIRException { 335 if (src == null) 336 return null; 337 org.hl7.fhir.r5.model.AuditEvent.AuditEventEntityDetailComponent tgt = new org.hl7.fhir.r5.model.AuditEvent.AuditEventEntityDetailComponent(); 338 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 339 if (src.hasType()) 340 tgt.getType().setTextElement(String30_50.convertString(src.getTypeElement())); 341 if (src.hasValue()) 342 tgt.setValue(new org.hl7.fhir.r5.model.Base64BinaryType(src.getValue())); 343 return tgt; 344 } 345 346 347 public static org.hl7.fhir.dstu3.model.AuditEvent.AuditEventSourceComponent convertAuditEventSourceComponent(org.hl7.fhir.r5.model.AuditEvent.AuditEventSourceComponent src) throws FHIRException { 348 if (src == null) 349 return null; 350 org.hl7.fhir.dstu3.model.AuditEvent.AuditEventSourceComponent tgt = new org.hl7.fhir.dstu3.model.AuditEvent.AuditEventSourceComponent(); 351 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 352// if (src.hasSite()) 353// tgt.setSiteElement(String30_50.convertString(src.getSiteElement())); 354 if (src.hasObserver()) 355 tgt.setIdentifier(Identifier30_50.convertIdentifier(src.getObserver().getIdentifier())); 356 for (CodeableConcept t : src.getType()) tgt.addType(Coding30_50.convertCoding(t.getCodingFirstRep())); 357 return tgt; 358 } 359 360 public static org.hl7.fhir.r5.model.AuditEvent.AuditEventSourceComponent convertAuditEventSourceComponent(org.hl7.fhir.dstu3.model.AuditEvent.AuditEventSourceComponent src) throws FHIRException { 361 if (src == null) 362 return null; 363 org.hl7.fhir.r5.model.AuditEvent.AuditEventSourceComponent tgt = new org.hl7.fhir.r5.model.AuditEvent.AuditEventSourceComponent(); 364 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 365// if (src.hasSite()) 366// tgt.setSiteElement(String30_50.convertString(src.getSiteElement())); 367 if (src.hasIdentifier()) 368 tgt.getObserver().setIdentifier(Identifier30_50.convertIdentifier(src.getIdentifier())); 369 for (org.hl7.fhir.dstu3.model.Coding t : src.getType()) tgt.addType().addCoding(Coding30_50.convertCoding(t)); 370 return tgt; 371 } 372}