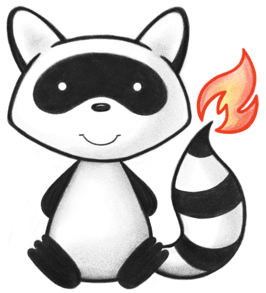
001package org.hl7.fhir.convertors.conv30_50.resources30_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_50; 004import org.hl7.fhir.convertors.conv30_50.datatypes30_50.Reference30_50; 005import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Base64Binary30_50; 006import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Code30_50; 007import org.hl7.fhir.exceptions.FHIRException; 008 009public class Binary30_50 { 010 011 public static org.hl7.fhir.r5.model.Binary convertBinary(org.hl7.fhir.dstu3.model.Binary src) throws FHIRException { 012 if (src == null) 013 return null; 014 org.hl7.fhir.r5.model.Binary tgt = new org.hl7.fhir.r5.model.Binary(); 015 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyResource(src, tgt); 016 if (src.hasContentType()) 017 tgt.setContentTypeElement(Code30_50.convertCode(src.getContentTypeElement())); 018 if (src.hasSecurityContext()) 019 tgt.setSecurityContext(Reference30_50.convertReference(src.getSecurityContext())); 020 if (src.hasContent()) 021 tgt.setDataElement(Base64Binary30_50.convertBase64Binary(src.getContentElement())); 022 return tgt; 023 } 024 025 public static org.hl7.fhir.dstu3.model.Binary convertBinary(org.hl7.fhir.r5.model.Binary src) throws FHIRException { 026 if (src == null) 027 return null; 028 org.hl7.fhir.dstu3.model.Binary tgt = new org.hl7.fhir.dstu3.model.Binary(); 029 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyResource(src, tgt); 030 if (src.hasContentType()) 031 tgt.setContentTypeElement(Code30_50.convertCode(src.getContentTypeElement())); 032 if (src.hasSecurityContext()) 033 tgt.setSecurityContext(Reference30_50.convertReference(src.getSecurityContext())); 034 if (src.hasData()) 035 tgt.setContentElement(Base64Binary30_50.convertBase64Binary(src.getDataElement())); 036 return tgt; 037 } 038}