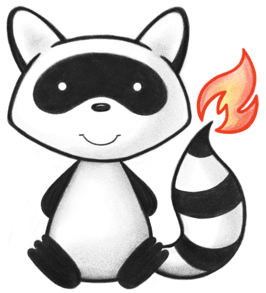
001package org.hl7.fhir.convertors.conv30_50.resources30_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_50; 004import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Identifier30_50; 005import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Signature30_50; 006import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Decimal30_50; 007import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Instant30_50; 008import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.String30_50; 009import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.UnsignedInt30_50; 010import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Uri30_50; 011import org.hl7.fhir.dstu3.model.Bundle; 012import org.hl7.fhir.dstu3.model.Enumeration; 013import org.hl7.fhir.exceptions.FHIRException; 014import org.hl7.fhir.r5.model.Bundle.LinkRelationTypes; 015 016public class Bundle30_50 { 017 018 public static org.hl7.fhir.r5.model.Bundle convertBundle(org.hl7.fhir.dstu3.model.Bundle src) throws FHIRException { 019 if (src == null) 020 return null; 021 org.hl7.fhir.r5.model.Bundle tgt = new org.hl7.fhir.r5.model.Bundle(); 022 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyResource(src, tgt); 023 if (src.hasIdentifier()) 024 tgt.setIdentifier(Identifier30_50.convertIdentifier(src.getIdentifier())); 025 if (src.hasType()) 026 tgt.setTypeElement(convertBundleType(src.getTypeElement())); 027 if (src.hasTotal()) 028 tgt.setTotalElement(UnsignedInt30_50.convertUnsignedInt(src.getTotalElement())); 029 for (org.hl7.fhir.dstu3.model.Bundle.BundleLinkComponent t : src.getLink()) 030 tgt.addLink(convertBundleLinkComponent(t)); 031 for (org.hl7.fhir.dstu3.model.Bundle.BundleEntryComponent t : src.getEntry()) 032 tgt.addEntry(convertBundleEntryComponent(t)); 033 if (src.hasSignature()) 034 tgt.setSignature(Signature30_50.convertSignature(src.getSignature())); 035 return tgt; 036 } 037 038 public static org.hl7.fhir.dstu3.model.Bundle convertBundle(org.hl7.fhir.r5.model.Bundle src) throws FHIRException { 039 if (src == null) 040 return null; 041 org.hl7.fhir.dstu3.model.Bundle tgt = new org.hl7.fhir.dstu3.model.Bundle(); 042 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyResource(src, tgt); 043 if (src.hasIdentifier()) 044 tgt.setIdentifier(Identifier30_50.convertIdentifier(src.getIdentifier())); 045 if (src.hasType()) 046 tgt.setTypeElement(convertBundleType(src.getTypeElement())); 047 if (src.hasTotal()) 048 tgt.setTotalElement(UnsignedInt30_50.convertUnsignedInt(src.getTotalElement())); 049 for (org.hl7.fhir.r5.model.Bundle.BundleLinkComponent t : src.getLink()) tgt.addLink(convertBundleLinkComponent(t)); 050 for (org.hl7.fhir.r5.model.Bundle.BundleEntryComponent t : src.getEntry()) 051 tgt.addEntry(convertBundleEntryComponent(t)); 052 if (src.hasSignature()) 053 tgt.setSignature(Signature30_50.convertSignature(src.getSignature())); 054 return tgt; 055 } 056 057 public static org.hl7.fhir.dstu3.model.Bundle.BundleEntryComponent convertBundleEntryComponent(org.hl7.fhir.r5.model.Bundle.BundleEntryComponent src) throws FHIRException { 058 if (src == null) 059 return null; 060 org.hl7.fhir.dstu3.model.Bundle.BundleEntryComponent tgt = new org.hl7.fhir.dstu3.model.Bundle.BundleEntryComponent(); 061 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 062 for (org.hl7.fhir.r5.model.Bundle.BundleLinkComponent t : src.getLink()) tgt.addLink(convertBundleLinkComponent(t)); 063 if (src.hasFullUrl()) 064 tgt.setFullUrlElement(Uri30_50.convertUri(src.getFullUrlElement())); 065 if (src.hasResource()) 066 tgt.setResource(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertResource(src.getResource())); 067 if (src.hasSearch()) 068 tgt.setSearch(convertBundleEntrySearchComponent(src.getSearch())); 069 if (src.hasRequest()) 070 tgt.setRequest(convertBundleEntryRequestComponent(src.getRequest())); 071 if (src.hasResponse()) 072 tgt.setResponse(convertBundleEntryResponseComponent(src.getResponse())); 073 return tgt; 074 } 075 076 public static org.hl7.fhir.r5.model.Bundle.BundleEntryComponent convertBundleEntryComponent(org.hl7.fhir.dstu3.model.Bundle.BundleEntryComponent src) throws FHIRException { 077 if (src == null) 078 return null; 079 org.hl7.fhir.r5.model.Bundle.BundleEntryComponent tgt = new org.hl7.fhir.r5.model.Bundle.BundleEntryComponent(); 080 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 081 for (org.hl7.fhir.dstu3.model.Bundle.BundleLinkComponent t : src.getLink()) 082 tgt.addLink(convertBundleLinkComponent(t)); 083 if (src.hasFullUrl()) 084 tgt.setFullUrlElement(Uri30_50.convertUri(src.getFullUrlElement())); 085 if (src.hasResource()) 086 tgt.setResource(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertResource(src.getResource())); 087 if (src.hasSearch()) 088 tgt.setSearch(convertBundleEntrySearchComponent(src.getSearch())); 089 if (src.hasRequest()) 090 tgt.setRequest(convertBundleEntryRequestComponent(src.getRequest())); 091 if (src.hasResponse()) 092 tgt.setResponse(convertBundleEntryResponseComponent(src.getResponse())); 093 return tgt; 094 } 095 096 public static org.hl7.fhir.r5.model.Bundle.BundleEntryRequestComponent convertBundleEntryRequestComponent(org.hl7.fhir.dstu3.model.Bundle.BundleEntryRequestComponent src) throws FHIRException { 097 if (src == null) 098 return null; 099 org.hl7.fhir.r5.model.Bundle.BundleEntryRequestComponent tgt = new org.hl7.fhir.r5.model.Bundle.BundleEntryRequestComponent(); 100 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 101 if (src.hasMethod()) 102 tgt.setMethodElement(convertHTTPVerb(src.getMethodElement())); 103 if (src.hasUrl()) 104 tgt.setUrlElement(Uri30_50.convertUri(src.getUrlElement())); 105 if (src.hasIfNoneMatch()) 106 tgt.setIfNoneMatchElement(String30_50.convertString(src.getIfNoneMatchElement())); 107 if (src.hasIfModifiedSince()) 108 tgt.setIfModifiedSinceElement(Instant30_50.convertInstant(src.getIfModifiedSinceElement())); 109 if (src.hasIfMatch()) 110 tgt.setIfMatchElement(String30_50.convertString(src.getIfMatchElement())); 111 if (src.hasIfNoneExist()) 112 tgt.setIfNoneExistElement(String30_50.convertString(src.getIfNoneExistElement())); 113 return tgt; 114 } 115 116 public static org.hl7.fhir.dstu3.model.Bundle.BundleEntryRequestComponent convertBundleEntryRequestComponent(org.hl7.fhir.r5.model.Bundle.BundleEntryRequestComponent src) throws FHIRException { 117 if (src == null) 118 return null; 119 org.hl7.fhir.dstu3.model.Bundle.BundleEntryRequestComponent tgt = new org.hl7.fhir.dstu3.model.Bundle.BundleEntryRequestComponent(); 120 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 121 if (src.hasMethod()) 122 tgt.setMethodElement(convertHTTPVerb(src.getMethodElement())); 123 if (src.hasUrl()) 124 tgt.setUrlElement(Uri30_50.convertUri(src.getUrlElement())); 125 if (src.hasIfNoneMatch()) 126 tgt.setIfNoneMatchElement(String30_50.convertString(src.getIfNoneMatchElement())); 127 if (src.hasIfModifiedSince()) 128 tgt.setIfModifiedSinceElement(Instant30_50.convertInstant(src.getIfModifiedSinceElement())); 129 if (src.hasIfMatch()) 130 tgt.setIfMatchElement(String30_50.convertString(src.getIfMatchElement())); 131 if (src.hasIfNoneExist()) 132 tgt.setIfNoneExistElement(String30_50.convertString(src.getIfNoneExistElement())); 133 return tgt; 134 } 135 136 public static org.hl7.fhir.r5.model.Bundle.BundleEntryResponseComponent convertBundleEntryResponseComponent(org.hl7.fhir.dstu3.model.Bundle.BundleEntryResponseComponent src) throws FHIRException { 137 if (src == null) 138 return null; 139 org.hl7.fhir.r5.model.Bundle.BundleEntryResponseComponent tgt = new org.hl7.fhir.r5.model.Bundle.BundleEntryResponseComponent(); 140 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 141 if (src.hasStatus()) 142 tgt.setStatusElement(String30_50.convertString(src.getStatusElement())); 143 if (src.hasLocation()) 144 tgt.setLocationElement(Uri30_50.convertUri(src.getLocationElement())); 145 if (src.hasEtag()) 146 tgt.setEtagElement(String30_50.convertString(src.getEtagElement())); 147 if (src.hasLastModified()) 148 tgt.setLastModifiedElement(Instant30_50.convertInstant(src.getLastModifiedElement())); 149 if (src.hasOutcome()) 150 tgt.setOutcome(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertResource(src.getOutcome())); 151 return tgt; 152 } 153 154 public static org.hl7.fhir.dstu3.model.Bundle.BundleEntryResponseComponent convertBundleEntryResponseComponent(org.hl7.fhir.r5.model.Bundle.BundleEntryResponseComponent src) throws FHIRException { 155 if (src == null) 156 return null; 157 org.hl7.fhir.dstu3.model.Bundle.BundleEntryResponseComponent tgt = new org.hl7.fhir.dstu3.model.Bundle.BundleEntryResponseComponent(); 158 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 159 if (src.hasStatus()) 160 tgt.setStatusElement(String30_50.convertString(src.getStatusElement())); 161 if (src.hasLocation()) 162 tgt.setLocationElement(Uri30_50.convertUri(src.getLocationElement())); 163 if (src.hasEtag()) 164 tgt.setEtagElement(String30_50.convertString(src.getEtagElement())); 165 if (src.hasLastModified()) 166 tgt.setLastModifiedElement(Instant30_50.convertInstant(src.getLastModifiedElement())); 167 if (src.hasOutcome()) 168 tgt.setOutcome(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertResource(src.getOutcome())); 169 return tgt; 170 } 171 172 public static org.hl7.fhir.dstu3.model.Bundle.BundleEntrySearchComponent convertBundleEntrySearchComponent(org.hl7.fhir.r5.model.Bundle.BundleEntrySearchComponent src) throws FHIRException { 173 if (src == null) 174 return null; 175 org.hl7.fhir.dstu3.model.Bundle.BundleEntrySearchComponent tgt = new org.hl7.fhir.dstu3.model.Bundle.BundleEntrySearchComponent(); 176 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 177 if (src.hasMode()) 178 tgt.setModeElement(convertSearchEntryMode(src.getModeElement())); 179 if (src.hasScore()) 180 tgt.setScoreElement(Decimal30_50.convertDecimal(src.getScoreElement())); 181 return tgt; 182 } 183 184 public static org.hl7.fhir.r5.model.Bundle.BundleEntrySearchComponent convertBundleEntrySearchComponent(org.hl7.fhir.dstu3.model.Bundle.BundleEntrySearchComponent src) throws FHIRException { 185 if (src == null) 186 return null; 187 org.hl7.fhir.r5.model.Bundle.BundleEntrySearchComponent tgt = new org.hl7.fhir.r5.model.Bundle.BundleEntrySearchComponent(); 188 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 189 if (src.hasMode()) 190 tgt.setModeElement(convertSearchEntryMode(src.getModeElement())); 191 if (src.hasScore()) 192 tgt.setScoreElement(Decimal30_50.convertDecimal(src.getScoreElement())); 193 return tgt; 194 } 195 196 public static org.hl7.fhir.r5.model.Bundle.BundleLinkComponent convertBundleLinkComponent(org.hl7.fhir.dstu3.model.Bundle.BundleLinkComponent src) throws FHIRException { 197 if (src == null) 198 return null; 199 org.hl7.fhir.r5.model.Bundle.BundleLinkComponent tgt = new org.hl7.fhir.r5.model.Bundle.BundleLinkComponent(); 200 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 201 if (src.hasRelation()) 202 tgt.setRelation(LinkRelationTypes.fromCode(src.getRelation())); 203 if (src.hasUrl()) 204 tgt.setUrlElement(Uri30_50.convertUri(src.getUrlElement())); 205 return tgt; 206 } 207 208 public static org.hl7.fhir.dstu3.model.Bundle.BundleLinkComponent convertBundleLinkComponent(org.hl7.fhir.r5.model.Bundle.BundleLinkComponent src) throws FHIRException { 209 if (src == null) 210 return null; 211 org.hl7.fhir.dstu3.model.Bundle.BundleLinkComponent tgt = new org.hl7.fhir.dstu3.model.Bundle.BundleLinkComponent(); 212 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 213 if (src.hasRelation()) 214 tgt.setRelation((src.getRelation().toCode())); 215 if (src.hasUrl()) 216 tgt.setUrlElement(Uri30_50.convertUri(src.getUrlElement())); 217 return tgt; 218 } 219 220 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Bundle.BundleType> convertBundleType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Bundle.BundleType> src) throws FHIRException { 221 if (src == null || src.isEmpty()) 222 return null; 223 Enumeration<Bundle.BundleType> tgt = new Enumeration<>(new Bundle.BundleTypeEnumFactory()); 224 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 225 if (src.getValue() == null) { 226 tgt.setValue(null); 227 } else { 228 switch (src.getValue()) { 229 case DOCUMENT: 230 tgt.setValue(Bundle.BundleType.DOCUMENT); 231 break; 232 case MESSAGE: 233 tgt.setValue(Bundle.BundleType.MESSAGE); 234 break; 235 case TRANSACTION: 236 tgt.setValue(Bundle.BundleType.TRANSACTION); 237 break; 238 case TRANSACTIONRESPONSE: 239 tgt.setValue(Bundle.BundleType.TRANSACTIONRESPONSE); 240 break; 241 case BATCH: 242 tgt.setValue(Bundle.BundleType.BATCH); 243 break; 244 case BATCHRESPONSE: 245 tgt.setValue(Bundle.BundleType.BATCHRESPONSE); 246 break; 247 case HISTORY: 248 tgt.setValue(Bundle.BundleType.HISTORY); 249 break; 250 case SEARCHSET: 251 tgt.setValue(Bundle.BundleType.SEARCHSET); 252 break; 253 case COLLECTION: 254 tgt.setValue(Bundle.BundleType.COLLECTION); 255 break; 256 default: 257 tgt.setValue(Bundle.BundleType.NULL); 258 break; 259 } 260 } 261 return tgt; 262 } 263 264 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Bundle.BundleType> convertBundleType(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Bundle.BundleType> src) throws FHIRException { 265 if (src == null || src.isEmpty()) 266 return null; 267 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Bundle.BundleType> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Bundle.BundleTypeEnumFactory()); 268 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 269 if (src.getValue() == null) { 270 tgt.setValue(null); 271 } else { 272 switch (src.getValue()) { 273 case DOCUMENT: 274 tgt.setValue(org.hl7.fhir.r5.model.Bundle.BundleType.DOCUMENT); 275 break; 276 case MESSAGE: 277 tgt.setValue(org.hl7.fhir.r5.model.Bundle.BundleType.MESSAGE); 278 break; 279 case TRANSACTION: 280 tgt.setValue(org.hl7.fhir.r5.model.Bundle.BundleType.TRANSACTION); 281 break; 282 case TRANSACTIONRESPONSE: 283 tgt.setValue(org.hl7.fhir.r5.model.Bundle.BundleType.TRANSACTIONRESPONSE); 284 break; 285 case BATCH: 286 tgt.setValue(org.hl7.fhir.r5.model.Bundle.BundleType.BATCH); 287 break; 288 case BATCHRESPONSE: 289 tgt.setValue(org.hl7.fhir.r5.model.Bundle.BundleType.BATCHRESPONSE); 290 break; 291 case HISTORY: 292 tgt.setValue(org.hl7.fhir.r5.model.Bundle.BundleType.HISTORY); 293 break; 294 case SEARCHSET: 295 tgt.setValue(org.hl7.fhir.r5.model.Bundle.BundleType.SEARCHSET); 296 break; 297 case COLLECTION: 298 tgt.setValue(org.hl7.fhir.r5.model.Bundle.BundleType.COLLECTION); 299 break; 300 default: 301 tgt.setValue(org.hl7.fhir.r5.model.Bundle.BundleType.NULL); 302 break; 303 } 304 } 305 return tgt; 306 } 307 308 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Bundle.HTTPVerb> convertHTTPVerb(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Bundle.HTTPVerb> src) throws FHIRException { 309 if (src == null || src.isEmpty()) 310 return null; 311 Enumeration<Bundle.HTTPVerb> tgt = new Enumeration<>(new Bundle.HTTPVerbEnumFactory()); 312 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 313 if (src.getValue() == null) { 314 tgt.setValue(null); 315 } else { 316 switch (src.getValue()) { 317 case GET: 318 tgt.setValue(Bundle.HTTPVerb.GET); 319 break; 320 case POST: 321 tgt.setValue(Bundle.HTTPVerb.POST); 322 break; 323 case PUT: 324 tgt.setValue(Bundle.HTTPVerb.PUT); 325 break; 326 case DELETE: 327 tgt.setValue(Bundle.HTTPVerb.DELETE); 328 break; 329 default: 330 tgt.setValue(Bundle.HTTPVerb.NULL); 331 break; 332 } 333 } 334 return tgt; 335 } 336 337 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Bundle.HTTPVerb> convertHTTPVerb(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Bundle.HTTPVerb> src) throws FHIRException { 338 if (src == null || src.isEmpty()) 339 return null; 340 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Bundle.HTTPVerb> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Bundle.HTTPVerbEnumFactory()); 341 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 342 if (src.getValue() == null) { 343 tgt.setValue(null); 344 } else { 345 switch (src.getValue()) { 346 case GET: 347 tgt.setValue(org.hl7.fhir.r5.model.Bundle.HTTPVerb.GET); 348 break; 349 case POST: 350 tgt.setValue(org.hl7.fhir.r5.model.Bundle.HTTPVerb.POST); 351 break; 352 case PUT: 353 tgt.setValue(org.hl7.fhir.r5.model.Bundle.HTTPVerb.PUT); 354 break; 355 case DELETE: 356 tgt.setValue(org.hl7.fhir.r5.model.Bundle.HTTPVerb.DELETE); 357 break; 358 default: 359 tgt.setValue(org.hl7.fhir.r5.model.Bundle.HTTPVerb.NULL); 360 break; 361 } 362 } 363 return tgt; 364 } 365 366 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Bundle.SearchEntryMode> convertSearchEntryMode(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Bundle.SearchEntryMode> src) throws FHIRException { 367 if (src == null || src.isEmpty()) 368 return null; 369 Enumeration<Bundle.SearchEntryMode> tgt = new Enumeration<>(new Bundle.SearchEntryModeEnumFactory()); 370 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 371 if (src.getValue() == null) { 372 tgt.setValue(null); 373 } else { 374 switch (src.getValue()) { 375 case MATCH: 376 tgt.setValue(Bundle.SearchEntryMode.MATCH); 377 break; 378 case INCLUDE: 379 tgt.setValue(Bundle.SearchEntryMode.INCLUDE); 380 break; 381 case OUTCOME: 382 tgt.setValue(Bundle.SearchEntryMode.OUTCOME); 383 break; 384 default: 385 tgt.setValue(Bundle.SearchEntryMode.NULL); 386 break; 387 } 388 } 389 return tgt; 390 } 391 392 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Bundle.SearchEntryMode> convertSearchEntryMode(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Bundle.SearchEntryMode> src) throws FHIRException { 393 if (src == null || src.isEmpty()) 394 return null; 395 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Bundle.SearchEntryMode> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Bundle.SearchEntryModeEnumFactory()); 396 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 397 if (src.getValue() == null) { 398 tgt.setValue(null); 399 } else { 400 switch (src.getValue()) { 401 case MATCH: 402 tgt.setValue(org.hl7.fhir.r5.model.Bundle.SearchEntryMode.MATCH); 403 break; 404 case INCLUDE: 405 tgt.setValue(org.hl7.fhir.r5.model.Bundle.SearchEntryMode.INCLUDE); 406 break; 407 case OUTCOME: 408 tgt.setValue(org.hl7.fhir.r5.model.Bundle.SearchEntryMode.OUTCOME); 409 break; 410 default: 411 tgt.setValue(org.hl7.fhir.r5.model.Bundle.SearchEntryMode.NULL); 412 break; 413 } 414 } 415 return tgt; 416 } 417}