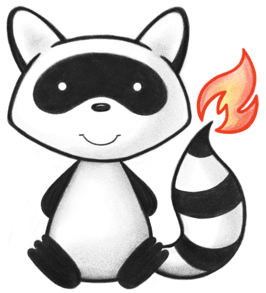
001package org.hl7.fhir.convertors.conv30_50.resources30_50; 002 003import java.util.stream.Collectors; 004 005import org.hl7.fhir.convertors.VersionConvertorConstants; 006import org.hl7.fhir.convertors.context.ConversionContext30_50; 007import org.hl7.fhir.convertors.conv30_50.datatypes30_50.ContactDetail30_50; 008import org.hl7.fhir.convertors.conv30_50.datatypes30_50.Reference30_50; 009import org.hl7.fhir.convertors.conv30_50.datatypes30_50.UsageContext30_50; 010import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.CodeableConcept30_50; 011import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Coding30_50; 012import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Boolean30_50; 013import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Code30_50; 014import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.DateTime30_50; 015import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.MarkDown30_50; 016import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.String30_50; 017import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.UnsignedInt30_50; 018import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Uri30_50; 019import org.hl7.fhir.dstu3.model.CapabilityStatement; 020import org.hl7.fhir.dstu3.model.Enumeration; 021import org.hl7.fhir.exceptions.FHIRException; 022import org.hl7.fhir.r5.model.Enumerations; 023 024public class CapabilityStatement30_50 { 025 026 public static final String ACCEPT_UNKNOWN_EXTENSION_URL = "http://hl7.org/fhir/3.0/StructureDefinition/extension-CapabilityStatement.acceptUnknown"; 027 public static final String PROFILE_EXTENSION_URL = "http://hl7.org/fhir/3.0/StructureDefinition/extension-CapabilityStatement.profile"; 028 029 private static final String[] IGNORED_EXTENSION_URLS = new String[]{ 030 ACCEPT_UNKNOWN_EXTENSION_URL, 031 PROFILE_EXTENSION_URL 032 }; 033 034 public static org.hl7.fhir.r5.model.CapabilityStatement convertCapabilityStatement(org.hl7.fhir.dstu3.model.CapabilityStatement src) throws FHIRException { 035 if (src == null) 036 return null; 037 org.hl7.fhir.r5.model.CapabilityStatement tgt = new org.hl7.fhir.r5.model.CapabilityStatement(); 038 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 039 if (src.hasUrl()) 040 tgt.setUrlElement(Uri30_50.convertUri(src.getUrlElement())); 041 if (src.hasVersion()) 042 tgt.setVersionElement(String30_50.convertString(src.getVersionElement())); 043 if (src.hasName()) 044 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 045 if (src.hasTitle()) 046 tgt.setTitleElement(String30_50.convertString(src.getTitleElement())); 047 if (src.hasStatus()) 048 tgt.setStatusElement(Enumerations30_50.convertPublicationStatus(src.getStatusElement())); 049 if (src.hasExperimental()) 050 tgt.setExperimentalElement(Boolean30_50.convertBoolean(src.getExperimentalElement())); 051 if (src.hasDate()) 052 tgt.setDateElement(DateTime30_50.convertDateTime(src.getDateElement())); 053 if (src.hasPublisher()) 054 tgt.setPublisherElement(String30_50.convertString(src.getPublisherElement())); 055 for (org.hl7.fhir.dstu3.model.ContactDetail t : src.getContact()) 056 tgt.addContact(ContactDetail30_50.convertContactDetail(t)); 057 if (src.hasDescription()) 058 tgt.setDescriptionElement(MarkDown30_50.convertMarkdown(src.getDescriptionElement())); 059 for (org.hl7.fhir.dstu3.model.UsageContext t : src.getUseContext()) 060 tgt.addUseContext(UsageContext30_50.convertUsageContext(t)); 061 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getJurisdiction()) 062 tgt.addJurisdiction(CodeableConcept30_50.convertCodeableConcept(t)); 063 if (src.hasPurpose()) 064 tgt.setPurposeElement(MarkDown30_50.convertMarkdown(src.getPurposeElement())); 065 if (src.hasCopyright()) 066 tgt.setCopyrightElement(MarkDown30_50.convertMarkdown(src.getCopyrightElement())); 067 if (src.hasKind()) 068 tgt.setKindElement(convertCapabilityStatementKind(src.getKindElement())); 069 for (org.hl7.fhir.dstu3.model.UriType t : src.getInstantiates()) tgt.addInstantiates(t.getValue()); 070 if (src.hasSoftware()) 071 tgt.setSoftware(convertCapabilityStatementSoftwareComponent(src.getSoftware())); 072 if (src.hasImplementation()) 073 tgt.setImplementation(convertCapabilityStatementImplementationComponent(src.getImplementation())); 074 if (src.hasFhirVersion()) 075 tgt.setFhirVersion(org.hl7.fhir.r5.model.Enumerations.FHIRVersion.fromCode(fixCode(src.getFhirVersion()))); 076 if (src.hasAcceptUnknown()) 077 tgt.addExtension().setUrl(ACCEPT_UNKNOWN_EXTENSION_URL).setValue(new org.hl7.fhir.r5.model.CodeType(src.getAcceptUnknownElement().asStringValue())); 078 for (org.hl7.fhir.dstu3.model.CodeType t : src.getFormat()) tgt.addFormat(t.getValue()); 079 for (org.hl7.fhir.dstu3.model.CodeType t : src.getPatchFormat()) tgt.addPatchFormat(t.getValue()); 080 for (org.hl7.fhir.dstu3.model.UriType t : src.getImplementationGuide()) tgt.addImplementationGuide(t.getValue()); 081 for (org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementRestComponent t : src.getRest()) 082 tgt.addRest(convertCapabilityStatementRestComponent(t)); 083 for (org.hl7.fhir.dstu3.model.Reference t : src.getProfile()) 084 tgt.addExtension(PROFILE_EXTENSION_URL, Reference30_50.convertReference(t)); 085 for (org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementMessagingComponent t : src.getMessaging()) 086 tgt.addMessaging(convertCapabilityStatementMessagingComponent(t)); 087 for (org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementDocumentComponent t : src.getDocument()) 088 tgt.addDocument(convertCapabilityStatementDocumentComponent(t)); 089 return tgt; 090 } 091 092 private static String fixCode(String v) { 093 if ("STU3".equals(v)) { 094 return "3.0.2"; 095 } else { 096 return v; 097 } 098 } 099 100 public static org.hl7.fhir.dstu3.model.CapabilityStatement convertCapabilityStatement(org.hl7.fhir.r5.model.CapabilityStatement src) throws FHIRException { 101 if (src == null) 102 return null; 103 org.hl7.fhir.dstu3.model.CapabilityStatement tgt = new org.hl7.fhir.dstu3.model.CapabilityStatement(); 104 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt, IGNORED_EXTENSION_URLS); 105 if (src.hasUrl()) 106 tgt.setUrlElement(Uri30_50.convertUri(src.getUrlElement())); 107 if (src.hasVersion()) 108 tgt.setVersionElement(String30_50.convertString(src.getVersionElement())); 109 if (src.hasName()) 110 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 111 if (src.hasTitle()) 112 tgt.setTitleElement(String30_50.convertString(src.getTitleElement())); 113 if (src.hasStatus()) 114 tgt.setStatusElement(Enumerations30_50.convertPublicationStatus(src.getStatusElement())); 115 if (src.hasExperimental()) 116 tgt.setExperimentalElement(Boolean30_50.convertBoolean(src.getExperimentalElement())); 117 if (src.hasDate()) 118 tgt.setDateElement(DateTime30_50.convertDateTime(src.getDateElement())); 119 if (src.hasPublisher()) 120 tgt.setPublisherElement(String30_50.convertString(src.getPublisherElement())); 121 for (org.hl7.fhir.r5.model.ContactDetail t : src.getContact()) 122 tgt.addContact(ContactDetail30_50.convertContactDetail(t)); 123 if (src.hasDescription()) 124 tgt.setDescriptionElement(MarkDown30_50.convertMarkdown(src.getDescriptionElement())); 125 for (org.hl7.fhir.r5.model.UsageContext t : src.getUseContext()) 126 tgt.addUseContext(UsageContext30_50.convertUsageContext(t)); 127 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getJurisdiction()) 128 tgt.addJurisdiction(CodeableConcept30_50.convertCodeableConcept(t)); 129 if (src.hasPurpose()) 130 tgt.setPurposeElement(MarkDown30_50.convertMarkdown(src.getPurposeElement())); 131 if (src.hasCopyright()) 132 tgt.setCopyrightElement(MarkDown30_50.convertMarkdown(src.getCopyrightElement())); 133 if (src.hasKind()) 134 tgt.setKindElement(convertCapabilityStatementKind(src.getKindElement())); 135 for (org.hl7.fhir.r5.model.UriType t : src.getInstantiates()) tgt.addInstantiates(t.getValue()); 136 if (src.hasSoftware()) 137 tgt.setSoftware(convertCapabilityStatementSoftwareComponent(src.getSoftware())); 138 if (src.hasImplementation()) 139 tgt.setImplementation(convertCapabilityStatementImplementationComponent(src.getImplementation())); 140 if (src.hasFhirVersion()) 141 tgt.setFhirVersion(src.getFhirVersion().toCode()); 142 if (src.hasExtension(ACCEPT_UNKNOWN_EXTENSION_URL)) 143 tgt.setAcceptUnknown(org.hl7.fhir.dstu3.model.CapabilityStatement.UnknownContentCode.fromCode(src.getExtensionByUrl(ACCEPT_UNKNOWN_EXTENSION_URL).getValue().primitiveValue())); 144 for (org.hl7.fhir.r5.model.CodeType t : src.getFormat()) tgt.addFormat(t.getValue()); 145 for (org.hl7.fhir.r5.model.CodeType t : src.getPatchFormat()) tgt.addPatchFormat(t.getValue()); 146 for (org.hl7.fhir.r5.model.UriType t : src.getImplementationGuide()) tgt.addImplementationGuide(t.getValue()); 147 for (org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestComponent r : src.getRest()) 148 for (org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceComponent rr : r.getResource()) 149 for (org.hl7.fhir.r5.model.CanonicalType t : rr.getSupportedProfile()) 150 tgt.addProfile(Reference30_50.convertCanonicalToReference(t)); 151 for (org.hl7.fhir.r5.model.Extension ext : src.getExtension()) { 152 if (PROFILE_EXTENSION_URL.equals(ext.getUrl())) { 153 tgt.addProfile(Reference30_50.convertReference((org.hl7.fhir.r5.model.Reference) ext.getValue())); 154 } 155 } 156 for (org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestComponent t : src.getRest()) 157 tgt.addRest(convertCapabilityStatementRestComponent(t)); 158 for (org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementMessagingComponent t : src.getMessaging()) 159 tgt.addMessaging(convertCapabilityStatementMessagingComponent(t)); 160 for (org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementDocumentComponent t : src.getDocument()) 161 tgt.addDocument(convertCapabilityStatementDocumentComponent(t)); 162 return tgt; 163 } 164 165 public static org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementDocumentComponent convertCapabilityStatementDocumentComponent(org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementDocumentComponent src) throws FHIRException { 166 if (src == null) 167 return null; 168 org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementDocumentComponent tgt = new org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementDocumentComponent(); 169 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 170 if (src.hasMode()) 171 tgt.setModeElement(convertDocumentMode(src.getModeElement())); 172 if (src.hasDocumentation()) 173 tgt.setDocumentation(src.getDocumentation()); 174 if (src.hasProfile()) 175 tgt.setProfileElement(Reference30_50.convertReferenceToCanonical(src.getProfile())); 176 return tgt; 177 } 178 179 public static org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementDocumentComponent convertCapabilityStatementDocumentComponent(org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementDocumentComponent src) throws FHIRException { 180 if (src == null) 181 return null; 182 org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementDocumentComponent tgt = new org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementDocumentComponent(); 183 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 184 if (src.hasMode()) 185 tgt.setModeElement(convertDocumentMode(src.getModeElement())); 186 if (src.hasDocumentation()) 187 tgt.setDocumentation(src.getDocumentation()); 188 if (src.hasProfile()) 189 tgt.setProfile(Reference30_50.convertCanonicalToReference(src.getProfileElement())); 190 return tgt; 191 } 192 193 public static org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementImplementationComponent convertCapabilityStatementImplementationComponent(org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementImplementationComponent src) throws FHIRException { 194 if (src == null) 195 return null; 196 org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementImplementationComponent tgt = new org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementImplementationComponent(); 197 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 198 if (src.hasDescription()) 199 tgt.setDescriptionElement(String30_50.convertStringToMarkdown(src.getDescriptionElement())); 200 if (src.hasUrl()) 201 tgt.setUrl(src.getUrl()); 202 return tgt; 203 } 204 205 public static org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementImplementationComponent convertCapabilityStatementImplementationComponent(org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementImplementationComponent src) throws FHIRException { 206 if (src == null) 207 return null; 208 org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementImplementationComponent tgt = new org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementImplementationComponent(); 209 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 210 if (src.hasDescription()) 211 tgt.setDescriptionElement(String30_50.convertString(src.getDescriptionElement())); 212 if (src.hasUrl()) 213 tgt.setUrl(src.getUrl()); 214 return tgt; 215 } 216 217 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementKind> convertCapabilityStatementKind(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.CapabilityStatementKind> src) throws FHIRException { 218 if (src == null || src.isEmpty()) 219 return null; 220 Enumeration<CapabilityStatement.CapabilityStatementKind> tgt = new Enumeration<>(new CapabilityStatement.CapabilityStatementKindEnumFactory()); 221 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 222 if (src.getValue() == null) { 223 tgt.setValue(null); 224 } else { 225 switch (src.getValue()) { 226 case INSTANCE: 227 tgt.setValue(CapabilityStatement.CapabilityStatementKind.INSTANCE); 228 break; 229 case CAPABILITY: 230 tgt.setValue(CapabilityStatement.CapabilityStatementKind.CAPABILITY); 231 break; 232 case REQUIREMENTS: 233 tgt.setValue(CapabilityStatement.CapabilityStatementKind.REQUIREMENTS); 234 break; 235 default: 236 tgt.setValue(CapabilityStatement.CapabilityStatementKind.NULL); 237 break; 238 } 239 } 240 return tgt; 241 } 242 243 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.CapabilityStatementKind> convertCapabilityStatementKind(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementKind> src) throws FHIRException { 244 if (src == null || src.isEmpty()) 245 return null; 246 org.hl7.fhir.r5.model.Enumeration<Enumerations.CapabilityStatementKind> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new Enumerations.CapabilityStatementKindEnumFactory()); 247 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 248 if (src.getValue() == null) { 249 tgt.setValue(null); 250 } else { 251 switch (src.getValue()) { 252 case INSTANCE: 253 tgt.setValue(Enumerations.CapabilityStatementKind.INSTANCE); 254 break; 255 case CAPABILITY: 256 tgt.setValue(Enumerations.CapabilityStatementKind.CAPABILITY); 257 break; 258 case REQUIREMENTS: 259 tgt.setValue(Enumerations.CapabilityStatementKind.REQUIREMENTS); 260 break; 261 default: 262 tgt.setValue(Enumerations.CapabilityStatementKind.NULL); 263 break; 264 } 265 } 266 return tgt; 267 } 268 269 public static org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementMessagingComponent convertCapabilityStatementMessagingComponent(org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementMessagingComponent src) throws FHIRException { 270 if (src == null) 271 return null; 272 org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementMessagingComponent tgt = new org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementMessagingComponent(); 273 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 274 for (org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementMessagingEndpointComponent t : src.getEndpoint()) 275 tgt.addEndpoint(convertCapabilityStatementMessagingEndpointComponent(t)); 276 if (src.hasReliableCache()) 277 tgt.setReliableCacheElement(UnsignedInt30_50.convertUnsignedInt(src.getReliableCacheElement())); 278 if (src.hasDocumentation()) 279 tgt.setDocumentation(src.getDocumentation()); 280 for (org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementMessagingSupportedMessageComponent t : src.getSupportedMessage()) 281 tgt.addSupportedMessage(convertCapabilityStatementMessagingSupportedMessageComponent(t)); 282 for (org.hl7.fhir.r5.model.Extension e : src.getExtensionsByUrl(VersionConvertorConstants.EXT_IG_CONFORMANCE_MESSAGE_EVENT)) { 283 org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementMessagingEventComponent event = new org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementMessagingEventComponent(); 284 tgt.addEvent(event); 285 event.setCode(Coding30_50.convertCoding((org.hl7.fhir.r5.model.Coding) e.getExtensionByUrl("code").getValue())); 286 if (e.hasExtension("category")) 287 event.setCategory(org.hl7.fhir.dstu3.model.CapabilityStatement.MessageSignificanceCategory.fromCode(e.getExtensionByUrl("category").getValue().toString())); 288 event.setMode(org.hl7.fhir.dstu3.model.CapabilityStatement.EventCapabilityMode.fromCode(e.getExtensionByUrl("mode").getValue().toString())); 289 org.hl7.fhir.r5.model.Extension focusE = e.getExtensionByUrl("focus"); 290 if (focusE.getValue().hasPrimitiveValue()) 291 event.setFocus(focusE.getValue().toString()); 292 else { 293 event.setFocusElement(new org.hl7.fhir.dstu3.model.CodeType()); 294 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(focusE.getValue(), event.getFocusElement()); 295 } 296 event.setRequest(Reference30_50.convertReference((org.hl7.fhir.r5.model.Reference) e.getExtensionByUrl("request").getValue())); 297 event.setResponse(Reference30_50.convertReference((org.hl7.fhir.r5.model.Reference) e.getExtensionByUrl("response").getValue())); 298 if (e.hasExtension("documentation")) 299 event.setDocumentation(e.getExtensionByUrl("documentation").getValue().toString()); 300 } 301 return tgt; 302 } 303 304 public static org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementMessagingComponent convertCapabilityStatementMessagingComponent(org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementMessagingComponent src) throws FHIRException { 305 if (src == null) 306 return null; 307 org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementMessagingComponent tgt = new org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementMessagingComponent(); 308 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 309 for (org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementMessagingEndpointComponent t : src.getEndpoint()) 310 tgt.addEndpoint(convertCapabilityStatementMessagingEndpointComponent(t)); 311 if (src.hasReliableCache()) 312 tgt.setReliableCacheElement(UnsignedInt30_50.convertUnsignedInt(src.getReliableCacheElement())); 313 if (src.hasDocumentation()) 314 tgt.setDocumentation(src.getDocumentation()); 315 for (org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementMessagingSupportedMessageComponent t : src.getSupportedMessage()) 316 tgt.addSupportedMessage(convertCapabilityStatementMessagingSupportedMessageComponent(t)); 317 for (org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementMessagingEventComponent t : src.getEvent()) { 318 org.hl7.fhir.r5.model.Extension e = new org.hl7.fhir.r5.model.Extension(VersionConvertorConstants.EXT_IG_CONFORMANCE_MESSAGE_EVENT); 319 e.addExtension(new org.hl7.fhir.r5.model.Extension("code", Coding30_50.convertCoding(t.getCode()))); 320 if (t.hasCategory()) 321 e.addExtension(new org.hl7.fhir.r5.model.Extension("category", new org.hl7.fhir.r5.model.CodeType(t.getCategory().toCode()))); 322 e.addExtension(new org.hl7.fhir.r5.model.Extension("mode", new org.hl7.fhir.r5.model.CodeType(t.getMode().toCode()))); 323 if (t.getFocusElement().hasValue()) 324 e.addExtension(new org.hl7.fhir.r5.model.Extension("focus", new org.hl7.fhir.r5.model.StringType(t.getFocus()))); 325 else { 326 org.hl7.fhir.r5.model.CodeType focus = new org.hl7.fhir.r5.model.CodeType(); 327 org.hl7.fhir.r5.model.Extension focusE = new org.hl7.fhir.r5.model.Extension("focus", focus); 328 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(t.getFocusElement(), focus); 329 e.addExtension(focusE); 330 } 331 e.addExtension(new org.hl7.fhir.r5.model.Extension("request", Reference30_50.convertReference(t.getRequest()))); 332 e.addExtension(new org.hl7.fhir.r5.model.Extension("response", Reference30_50.convertReference(t.getResponse()))); 333 if (t.hasDocumentation()) 334 e.addExtension(new org.hl7.fhir.r5.model.Extension("documentation", new org.hl7.fhir.r5.model.StringType(t.getDocumentation()))); 335 tgt.addExtension(e); 336 } 337 return tgt; 338 } 339 340 public static org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementMessagingEndpointComponent convertCapabilityStatementMessagingEndpointComponent(org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementMessagingEndpointComponent src) throws FHIRException { 341 if (src == null) 342 return null; 343 org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementMessagingEndpointComponent tgt = new org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementMessagingEndpointComponent(); 344 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 345 if (src.hasProtocol()) 346 tgt.setProtocol(Coding30_50.convertCoding(src.getProtocol())); 347 if (src.hasAddress()) 348 tgt.setAddress(src.getAddress()); 349 return tgt; 350 } 351 352 public static org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementMessagingEndpointComponent convertCapabilityStatementMessagingEndpointComponent(org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementMessagingEndpointComponent src) throws FHIRException { 353 if (src == null) 354 return null; 355 org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementMessagingEndpointComponent tgt = new org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementMessagingEndpointComponent(); 356 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 357 if (src.hasProtocol()) 358 tgt.setProtocol(Coding30_50.convertCoding(src.getProtocol())); 359 if (src.hasAddress()) 360 tgt.setAddress(src.getAddress()); 361 return tgt; 362 } 363 364 public static org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementMessagingSupportedMessageComponent convertCapabilityStatementMessagingSupportedMessageComponent(org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementMessagingSupportedMessageComponent src) throws FHIRException { 365 if (src == null) 366 return null; 367 org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementMessagingSupportedMessageComponent tgt = new org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementMessagingSupportedMessageComponent(); 368 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 369 if (src.hasMode()) 370 tgt.setModeElement(convertEventCapabilityMode(src.getModeElement())); 371 if (src.hasDefinition()) 372 tgt.setDefinition(Reference30_50.convertCanonicalToReference(src.getDefinitionElement())); 373 return tgt; 374 } 375 376 public static org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementMessagingSupportedMessageComponent convertCapabilityStatementMessagingSupportedMessageComponent(org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementMessagingSupportedMessageComponent src) throws FHIRException { 377 if (src == null) 378 return null; 379 org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementMessagingSupportedMessageComponent tgt = new org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementMessagingSupportedMessageComponent(); 380 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 381 if (src.hasMode()) 382 tgt.setModeElement(convertEventCapabilityMode(src.getModeElement())); 383 if (src.hasDefinition()) 384 tgt.setDefinitionElement(Reference30_50.convertReferenceToCanonical(src.getDefinition())); 385 return tgt; 386 } 387 388 public static org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementRestComponent convertCapabilityStatementRestComponent(org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestComponent src) throws FHIRException { 389 if (src == null) 390 return null; 391 org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementRestComponent tgt = new org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementRestComponent(); 392 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 393 if (src.hasMode()) 394 tgt.setModeElement(convertRestfulCapabilityMode(src.getModeElement())); 395 if (src.hasDocumentation()) 396 tgt.setDocumentation(src.getDocumentation()); 397 if (src.hasSecurity()) 398 tgt.setSecurity(convertCapabilityStatementRestSecurityComponent(src.getSecurity())); 399 for (org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceComponent t : src.getResource()) 400 tgt.addResource(convertCapabilityStatementRestResourceComponent(t)); 401 for (org.hl7.fhir.r5.model.CapabilityStatement.SystemInteractionComponent t : src.getInteraction()) 402 tgt.addInteraction(convertSystemInteractionComponent(t)); 403 for (org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceSearchParamComponent t : src.getSearchParam()) 404 tgt.addSearchParam(convertCapabilityStatementRestResourceSearchParamComponent(t)); 405 for (org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceOperationComponent t : src.getOperation()) 406 tgt.addOperation(convertCapabilityStatementRestOperationComponent(t)); 407 for (org.hl7.fhir.r5.model.UriType t : src.getCompartment()) tgt.addCompartment(t.getValue()); 408 return tgt; 409 } 410 411 public static org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestComponent convertCapabilityStatementRestComponent(org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementRestComponent src) throws FHIRException { 412 if (src == null) 413 return null; 414 org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestComponent tgt = new org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestComponent(); 415 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 416 if (src.hasMode()) 417 tgt.setModeElement(convertRestfulCapabilityMode(src.getModeElement())); 418 if (src.hasDocumentation()) 419 tgt.setDocumentation(src.getDocumentation()); 420 if (src.hasSecurity()) 421 tgt.setSecurity(convertCapabilityStatementRestSecurityComponent(src.getSecurity())); 422 for (org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementRestResourceComponent t : src.getResource()) 423 tgt.addResource(convertCapabilityStatementRestResourceComponent(t)); 424 for (org.hl7.fhir.dstu3.model.CapabilityStatement.SystemInteractionComponent t : src.getInteraction()) 425 tgt.addInteraction(convertSystemInteractionComponent(t)); 426 for (org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementRestResourceSearchParamComponent t : src.getSearchParam()) 427 tgt.addSearchParam(convertCapabilityStatementRestResourceSearchParamComponent(t)); 428 for (org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementRestOperationComponent t : src.getOperation()) 429 tgt.addOperation(convertCapabilityStatementRestOperationComponent(t)); 430 for (org.hl7.fhir.dstu3.model.UriType t : src.getCompartment()) tgt.addCompartment(t.getValue()); 431 return tgt; 432 } 433 434 public static org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementRestOperationComponent convertCapabilityStatementRestOperationComponent(org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceOperationComponent src) throws FHIRException { 435 if (src == null) 436 return null; 437 org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementRestOperationComponent tgt = new org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementRestOperationComponent(); 438 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 439 if (src.hasName()) 440 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 441 if (src.hasDefinition()) 442 tgt.setDefinition(Reference30_50.convertCanonicalToReference(src.getDefinitionElement())); 443 return tgt; 444 } 445 446 public static org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceOperationComponent convertCapabilityStatementRestOperationComponent(org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementRestOperationComponent src) throws FHIRException { 447 if (src == null) 448 return null; 449 org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceOperationComponent tgt = new org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceOperationComponent(); 450 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 451 if (src.hasName()) 452 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 453 if (src.hasDefinition()) 454 tgt.setDefinitionElement(Reference30_50.convertReferenceToCanonical(src.getDefinition())); 455 return tgt; 456 } 457 458 public static org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementRestResourceComponent convertCapabilityStatementRestResourceComponent(org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceComponent src) throws FHIRException { 459 if (src == null) 460 return null; 461 org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementRestResourceComponent tgt = new org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementRestResourceComponent(); 462 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 463 if (src.hasType()) 464 tgt.setTypeElement(Code30_50.convertCode(src.getTypeElement())); 465 if (src.hasProfile()) 466 tgt.setProfile(Reference30_50.convertCanonicalToReference(src.getProfileElement())); 467 if (src.hasDocumentation()) 468 tgt.setDocumentationElement(MarkDown30_50.convertMarkdown(src.getDocumentationElement())); 469 for (org.hl7.fhir.r5.model.CapabilityStatement.ResourceInteractionComponent t : src.getInteraction()) 470 tgt.addInteraction(convertResourceInteractionComponent(t)); 471 if (src.hasVersioning()) 472 tgt.setVersioningElement(convertResourceVersionPolicy(src.getVersioningElement())); 473 if (src.hasReadHistory()) 474 tgt.setReadHistoryElement(Boolean30_50.convertBoolean(src.getReadHistoryElement())); 475 if (src.hasUpdateCreate()) 476 tgt.setUpdateCreateElement(Boolean30_50.convertBoolean(src.getUpdateCreateElement())); 477 if (src.hasConditionalCreate()) 478 tgt.setConditionalCreateElement(Boolean30_50.convertBoolean(src.getConditionalCreateElement())); 479 if (src.hasConditionalRead()) 480 tgt.setConditionalReadElement(convertConditionalReadStatus(src.getConditionalReadElement())); 481 if (src.hasConditionalUpdate()) 482 tgt.setConditionalUpdateElement(Boolean30_50.convertBoolean(src.getConditionalUpdateElement())); 483 if (src.hasConditionalDelete()) 484 tgt.setConditionalDeleteElement(convertConditionalDeleteStatus(src.getConditionalDeleteElement())); 485 tgt.setReferencePolicy(src.getReferencePolicy().stream() 486 .map(CapabilityStatement30_50::convertReferenceHandlingPolicy) 487 .collect(Collectors.toList())); 488 for (org.hl7.fhir.r5.model.StringType t : src.getSearchInclude()) tgt.addSearchInclude(t.getValue()); 489 for (org.hl7.fhir.r5.model.StringType t : src.getSearchRevInclude()) tgt.addSearchRevInclude(t.getValue()); 490 for (org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceSearchParamComponent t : src.getSearchParam()) 491 tgt.addSearchParam(convertCapabilityStatementRestResourceSearchParamComponent(t)); 492 return tgt; 493 } 494 495 public static org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceComponent convertCapabilityStatementRestResourceComponent(org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementRestResourceComponent src) throws FHIRException { 496 if (src == null) 497 return null; 498 org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceComponent tgt = new org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceComponent(); 499 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 500 if (src.hasType()) 501 tgt.setTypeElement(Code30_50.convertCode(src.getTypeElement())); 502 if (src.hasProfile()) 503 tgt.setProfileElement(Reference30_50.convertReferenceToCanonical(src.getProfile())); 504 if (src.hasDocumentation()) 505 tgt.setDocumentationElement(MarkDown30_50.convertMarkdown(src.getDocumentationElement())); 506 for (org.hl7.fhir.dstu3.model.CapabilityStatement.ResourceInteractionComponent t : src.getInteraction()) 507 tgt.addInteraction(convertResourceInteractionComponent(t)); 508 if (src.hasVersioning()) 509 tgt.setVersioningElement(convertResourceVersionPolicy(src.getVersioningElement())); 510 if (src.hasReadHistory()) 511 tgt.setReadHistoryElement(Boolean30_50.convertBoolean(src.getReadHistoryElement())); 512 if (src.hasUpdateCreate()) 513 tgt.setUpdateCreateElement(Boolean30_50.convertBoolean(src.getUpdateCreateElement())); 514 if (src.hasConditionalCreate()) 515 tgt.setConditionalCreateElement(Boolean30_50.convertBoolean(src.getConditionalCreateElement())); 516 if (src.hasConditionalRead()) 517 tgt.setConditionalReadElement(convertConditionalReadStatus(src.getConditionalReadElement())); 518 if (src.hasConditionalUpdate()) 519 tgt.setConditionalUpdateElement(Boolean30_50.convertBoolean(src.getConditionalUpdateElement())); 520 if (src.hasConditionalDelete()) 521 tgt.setConditionalDeleteElement(convertConditionalDeleteStatus(src.getConditionalDeleteElement())); 522 tgt.setReferencePolicy(src.getReferencePolicy().stream() 523 .map(CapabilityStatement30_50::convertReferenceHandlingPolicy) 524 .collect(Collectors.toList())); 525 for (org.hl7.fhir.dstu3.model.StringType t : src.getSearchInclude()) tgt.addSearchInclude(t.getValue()); 526 for (org.hl7.fhir.dstu3.model.StringType t : src.getSearchRevInclude()) tgt.addSearchRevInclude(t.getValue()); 527 for (org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementRestResourceSearchParamComponent t : src.getSearchParam()) 528 tgt.addSearchParam(convertCapabilityStatementRestResourceSearchParamComponent(t)); 529 return tgt; 530 } 531 532 public static org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementRestResourceSearchParamComponent convertCapabilityStatementRestResourceSearchParamComponent(org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceSearchParamComponent src) throws FHIRException { 533 if (src == null) 534 return null; 535 org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementRestResourceSearchParamComponent tgt = new org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementRestResourceSearchParamComponent(); 536 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 537 if (src.hasName()) 538 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 539 if (src.hasDefinition()) 540 tgt.setDefinition(src.getDefinition()); 541 if (src.hasType()) 542 tgt.setTypeElement(Enumerations30_50.convertSearchParamType(src.getTypeElement())); 543 if (src.hasDocumentation()) 544 tgt.setDocumentation(src.getDocumentation()); 545 return tgt; 546 } 547 548 public static org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceSearchParamComponent convertCapabilityStatementRestResourceSearchParamComponent(org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementRestResourceSearchParamComponent src) throws FHIRException { 549 if (src == null) 550 return null; 551 org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceSearchParamComponent tgt = new org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceSearchParamComponent(); 552 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 553 if (src.hasName()) 554 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 555 if (src.hasDefinition()) 556 tgt.setDefinition(src.getDefinition()); 557 if (src.hasType()) 558 tgt.setTypeElement(Enumerations30_50.convertSearchParamType(src.getTypeElement())); 559 if (src.hasDocumentation()) 560 tgt.setDocumentation(src.getDocumentation()); 561 return tgt; 562 } 563 564 public static org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementRestSecurityComponent convertCapabilityStatementRestSecurityComponent(org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestSecurityComponent src) throws FHIRException { 565 if (src == null) 566 return null; 567 org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementRestSecurityComponent tgt = new org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementRestSecurityComponent(); 568 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 569 if (src.hasCors()) 570 tgt.setCorsElement(Boolean30_50.convertBoolean(src.getCorsElement())); 571 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getService()) 572 tgt.addService(CodeableConcept30_50.convertCodeableConcept(t)); 573 if (src.hasDescription()) 574 tgt.setDescription(src.getDescription()); 575 return tgt; 576 } 577 578 public static org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestSecurityComponent convertCapabilityStatementRestSecurityComponent(org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementRestSecurityComponent src) throws FHIRException { 579 if (src == null) 580 return null; 581 org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestSecurityComponent tgt = new org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestSecurityComponent(); 582 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 583 if (src.hasCors()) 584 tgt.setCorsElement(Boolean30_50.convertBoolean(src.getCorsElement())); 585 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getService()) 586 tgt.addService(CodeableConcept30_50.convertCodeableConcept(t)); 587 if (src.hasDescription()) 588 tgt.setDescription(src.getDescription()); 589 return tgt; 590 } 591 592 public static org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementSoftwareComponent convertCapabilityStatementSoftwareComponent(org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementSoftwareComponent src) throws FHIRException { 593 if (src == null) 594 return null; 595 org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementSoftwareComponent tgt = new org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementSoftwareComponent(); 596 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 597 if (src.hasName()) 598 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 599 if (src.hasVersion()) 600 tgt.setVersionElement(String30_50.convertString(src.getVersionElement())); 601 if (src.hasReleaseDate()) 602 tgt.setReleaseDateElement(DateTime30_50.convertDateTime(src.getReleaseDateElement())); 603 return tgt; 604 } 605 606 public static org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementSoftwareComponent convertCapabilityStatementSoftwareComponent(org.hl7.fhir.dstu3.model.CapabilityStatement.CapabilityStatementSoftwareComponent src) throws FHIRException { 607 if (src == null) 608 return null; 609 org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementSoftwareComponent tgt = new org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementSoftwareComponent(); 610 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 611 if (src.hasName()) 612 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 613 if (src.hasVersion()) 614 tgt.setVersionElement(String30_50.convertString(src.getVersionElement())); 615 if (src.hasReleaseDate()) 616 tgt.setReleaseDateElement(DateTime30_50.convertDateTime(src.getReleaseDateElement())); 617 return tgt; 618 } 619 620 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CapabilityStatement.ConditionalDeleteStatus> convertConditionalDeleteStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.ConditionalDeleteStatus> src) throws FHIRException { 621 if (src == null || src.isEmpty()) 622 return null; 623 Enumeration<CapabilityStatement.ConditionalDeleteStatus> tgt = new Enumeration<>(new CapabilityStatement.ConditionalDeleteStatusEnumFactory()); 624 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 625 if (src.getValue() == null) { 626 tgt.setValue(null); 627 } else { 628 switch (src.getValue()) { 629 case NOTSUPPORTED: 630 tgt.setValue(CapabilityStatement.ConditionalDeleteStatus.NOTSUPPORTED); 631 break; 632 case SINGLE: 633 tgt.setValue(CapabilityStatement.ConditionalDeleteStatus.SINGLE); 634 break; 635 case MULTIPLE: 636 tgt.setValue(CapabilityStatement.ConditionalDeleteStatus.MULTIPLE); 637 break; 638 default: 639 tgt.setValue(CapabilityStatement.ConditionalDeleteStatus.NULL); 640 break; 641 } 642 } 643 return tgt; 644 } 645 646 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.ConditionalDeleteStatus> convertConditionalDeleteStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CapabilityStatement.ConditionalDeleteStatus> src) throws FHIRException { 647 if (src == null || src.isEmpty()) 648 return null; 649 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.ConditionalDeleteStatus> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.CapabilityStatement.ConditionalDeleteStatusEnumFactory()); 650 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 651 if (src.getValue() == null) { 652 tgt.setValue(null); 653 } else { 654 switch (src.getValue()) { 655 case NOTSUPPORTED: 656 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.ConditionalDeleteStatus.NOTSUPPORTED); 657 break; 658 case SINGLE: 659 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.ConditionalDeleteStatus.SINGLE); 660 break; 661 case MULTIPLE: 662 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.ConditionalDeleteStatus.MULTIPLE); 663 break; 664 default: 665 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.ConditionalDeleteStatus.NULL); 666 break; 667 } 668 } 669 return tgt; 670 } 671 672 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CapabilityStatement.ConditionalReadStatus> convertConditionalReadStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.ConditionalReadStatus> src) throws FHIRException { 673 if (src == null || src.isEmpty()) 674 return null; 675 Enumeration<CapabilityStatement.ConditionalReadStatus> tgt = new Enumeration<>(new CapabilityStatement.ConditionalReadStatusEnumFactory()); 676 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 677 if (src.getValue() == null) { 678 tgt.setValue(null); 679 } else { 680 switch (src.getValue()) { 681 case NOTSUPPORTED: 682 tgt.setValue(CapabilityStatement.ConditionalReadStatus.NOTSUPPORTED); 683 break; 684 case MODIFIEDSINCE: 685 tgt.setValue(CapabilityStatement.ConditionalReadStatus.MODIFIEDSINCE); 686 break; 687 case NOTMATCH: 688 tgt.setValue(CapabilityStatement.ConditionalReadStatus.NOTMATCH); 689 break; 690 case FULLSUPPORT: 691 tgt.setValue(CapabilityStatement.ConditionalReadStatus.FULLSUPPORT); 692 break; 693 default: 694 tgt.setValue(CapabilityStatement.ConditionalReadStatus.NULL); 695 break; 696 } 697 } 698 return tgt; 699 } 700 701 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.ConditionalReadStatus> convertConditionalReadStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CapabilityStatement.ConditionalReadStatus> src) throws FHIRException { 702 if (src == null || src.isEmpty()) 703 return null; 704 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.ConditionalReadStatus> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.CapabilityStatement.ConditionalReadStatusEnumFactory()); 705 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 706 if (src.getValue() == null) { 707 tgt.setValue(null); 708 } else { 709 switch (src.getValue()) { 710 case NOTSUPPORTED: 711 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.ConditionalReadStatus.NOTSUPPORTED); 712 break; 713 case MODIFIEDSINCE: 714 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.ConditionalReadStatus.MODIFIEDSINCE); 715 break; 716 case NOTMATCH: 717 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.ConditionalReadStatus.NOTMATCH); 718 break; 719 case FULLSUPPORT: 720 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.ConditionalReadStatus.FULLSUPPORT); 721 break; 722 default: 723 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.ConditionalReadStatus.NULL); 724 break; 725 } 726 } 727 return tgt; 728 } 729 730 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CapabilityStatement.DocumentMode> convertDocumentMode(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.DocumentMode> src) throws FHIRException { 731 if (src == null || src.isEmpty()) 732 return null; 733 Enumeration<CapabilityStatement.DocumentMode> tgt = new Enumeration<>(new CapabilityStatement.DocumentModeEnumFactory()); 734 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 735 if (src.getValue() == null) { 736 tgt.setValue(null); 737 } else { 738 switch (src.getValue()) { 739 case PRODUCER: 740 tgt.setValue(CapabilityStatement.DocumentMode.PRODUCER); 741 break; 742 case CONSUMER: 743 tgt.setValue(CapabilityStatement.DocumentMode.CONSUMER); 744 break; 745 default: 746 tgt.setValue(CapabilityStatement.DocumentMode.NULL); 747 break; 748 } 749 } 750 return tgt; 751 } 752 753 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.DocumentMode> convertDocumentMode(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CapabilityStatement.DocumentMode> src) throws FHIRException { 754 if (src == null || src.isEmpty()) 755 return null; 756 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.DocumentMode> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.CapabilityStatement.DocumentModeEnumFactory()); 757 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 758 if (src.getValue() == null) { 759 tgt.setValue(null); 760 } else { 761 switch (src.getValue()) { 762 case PRODUCER: 763 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.DocumentMode.PRODUCER); 764 break; 765 case CONSUMER: 766 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.DocumentMode.CONSUMER); 767 break; 768 default: 769 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.DocumentMode.NULL); 770 break; 771 } 772 } 773 return tgt; 774 } 775 776 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.EventCapabilityMode> convertEventCapabilityMode(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CapabilityStatement.EventCapabilityMode> src) throws FHIRException { 777 if (src == null || src.isEmpty()) 778 return null; 779 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.EventCapabilityMode> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.CapabilityStatement.EventCapabilityModeEnumFactory()); 780 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 781 if (src.getValue() == null) { 782 tgt.setValue(null); 783 } else { 784 switch (src.getValue()) { 785 case SENDER: 786 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.EventCapabilityMode.SENDER); 787 break; 788 case RECEIVER: 789 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.EventCapabilityMode.RECEIVER); 790 break; 791 default: 792 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.EventCapabilityMode.NULL); 793 break; 794 } 795 } 796 return tgt; 797 } 798 799 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CapabilityStatement.EventCapabilityMode> convertEventCapabilityMode(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.EventCapabilityMode> src) throws FHIRException { 800 if (src == null || src.isEmpty()) 801 return null; 802 Enumeration<CapabilityStatement.EventCapabilityMode> tgt = new Enumeration<>(new CapabilityStatement.EventCapabilityModeEnumFactory()); 803 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 804 if (src.getValue() == null) { 805 tgt.setValue(null); 806 } else { 807 switch (src.getValue()) { 808 case SENDER: 809 tgt.setValue(CapabilityStatement.EventCapabilityMode.SENDER); 810 break; 811 case RECEIVER: 812 tgt.setValue(CapabilityStatement.EventCapabilityMode.RECEIVER); 813 break; 814 default: 815 tgt.setValue(CapabilityStatement.EventCapabilityMode.NULL); 816 break; 817 } 818 } 819 return tgt; 820 } 821 822 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CapabilityStatement.ReferenceHandlingPolicy> convertReferenceHandlingPolicy(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.ReferenceHandlingPolicy> src) throws FHIRException { 823 if (src == null || src.isEmpty()) 824 return null; 825 Enumeration<CapabilityStatement.ReferenceHandlingPolicy> tgt = new Enumeration<>(new CapabilityStatement.ReferenceHandlingPolicyEnumFactory()); 826 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 827 if (src.getValue() == null) { 828 tgt.setValue(null); 829 } else { 830 switch (src.getValue()) { 831 case LITERAL: 832 tgt.setValue(CapabilityStatement.ReferenceHandlingPolicy.LITERAL); 833 break; 834 case LOGICAL: 835 tgt.setValue(CapabilityStatement.ReferenceHandlingPolicy.LOGICAL); 836 break; 837 case RESOLVES: 838 tgt.setValue(CapabilityStatement.ReferenceHandlingPolicy.RESOLVES); 839 break; 840 case ENFORCED: 841 tgt.setValue(CapabilityStatement.ReferenceHandlingPolicy.ENFORCED); 842 break; 843 case LOCAL: 844 tgt.setValue(CapabilityStatement.ReferenceHandlingPolicy.LOCAL); 845 break; 846 default: 847 tgt.setValue(CapabilityStatement.ReferenceHandlingPolicy.NULL); 848 break; 849 } 850 } 851 return tgt; 852 } 853 854 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.ReferenceHandlingPolicy> convertReferenceHandlingPolicy(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CapabilityStatement.ReferenceHandlingPolicy> src) throws FHIRException { 855 if (src == null || src.isEmpty()) 856 return null; 857 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.ReferenceHandlingPolicy> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.CapabilityStatement.ReferenceHandlingPolicyEnumFactory()); 858 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 859 if (src.getValue() == null) { 860 tgt.setValue(null); 861 } else { 862 switch (src.getValue()) { 863 case LITERAL: 864 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.ReferenceHandlingPolicy.LITERAL); 865 break; 866 case LOGICAL: 867 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.ReferenceHandlingPolicy.LOGICAL); 868 break; 869 case RESOLVES: 870 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.ReferenceHandlingPolicy.RESOLVES); 871 break; 872 case ENFORCED: 873 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.ReferenceHandlingPolicy.ENFORCED); 874 break; 875 case LOCAL: 876 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.ReferenceHandlingPolicy.LOCAL); 877 break; 878 default: 879 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.ReferenceHandlingPolicy.NULL); 880 break; 881 } 882 } 883 return tgt; 884 } 885 886 public static org.hl7.fhir.r5.model.CapabilityStatement.ResourceInteractionComponent convertResourceInteractionComponent(org.hl7.fhir.dstu3.model.CapabilityStatement.ResourceInteractionComponent src) throws FHIRException { 887 if (src == null) 888 return null; 889 org.hl7.fhir.r5.model.CapabilityStatement.ResourceInteractionComponent tgt = new org.hl7.fhir.r5.model.CapabilityStatement.ResourceInteractionComponent(); 890 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 891 if (src.hasCode()) 892 tgt.setCodeElement(convertTypeRestfulInteraction(src.getCodeElement())); 893 if (src.hasDocumentation()) 894 tgt.setDocumentation(src.getDocumentation()); 895 return tgt; 896 } 897 898 public static org.hl7.fhir.dstu3.model.CapabilityStatement.ResourceInteractionComponent convertResourceInteractionComponent(org.hl7.fhir.r5.model.CapabilityStatement.ResourceInteractionComponent src) throws FHIRException { 899 if (src == null) 900 return null; 901 org.hl7.fhir.dstu3.model.CapabilityStatement.ResourceInteractionComponent tgt = new org.hl7.fhir.dstu3.model.CapabilityStatement.ResourceInteractionComponent(); 902 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 903 if (src.hasCode()) 904 tgt.setCodeElement(convertTypeRestfulInteraction(src.getCodeElement())); 905 if (src.hasDocumentation()) 906 tgt.setDocumentation(src.getDocumentation()); 907 return tgt; 908 } 909 910 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.ResourceVersionPolicy> convertResourceVersionPolicy(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CapabilityStatement.ResourceVersionPolicy> src) throws FHIRException { 911 if (src == null || src.isEmpty()) 912 return null; 913 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.ResourceVersionPolicy> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.CapabilityStatement.ResourceVersionPolicyEnumFactory()); 914 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 915 if (src.getValue() == null) { 916 tgt.setValue(null); 917 } else { 918 switch (src.getValue()) { 919 case NOVERSION: 920 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.ResourceVersionPolicy.NOVERSION); 921 break; 922 case VERSIONED: 923 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.ResourceVersionPolicy.VERSIONED); 924 break; 925 case VERSIONEDUPDATE: 926 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.ResourceVersionPolicy.VERSIONEDUPDATE); 927 break; 928 default: 929 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.ResourceVersionPolicy.NULL); 930 break; 931 } 932 } 933 return tgt; 934 } 935 936 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CapabilityStatement.ResourceVersionPolicy> convertResourceVersionPolicy(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.ResourceVersionPolicy> src) throws FHIRException { 937 if (src == null || src.isEmpty()) 938 return null; 939 Enumeration<CapabilityStatement.ResourceVersionPolicy> tgt = new Enumeration<>(new CapabilityStatement.ResourceVersionPolicyEnumFactory()); 940 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 941 if (src.getValue() == null) { 942 tgt.setValue(null); 943 } else { 944 switch (src.getValue()) { 945 case NOVERSION: 946 tgt.setValue(CapabilityStatement.ResourceVersionPolicy.NOVERSION); 947 break; 948 case VERSIONED: 949 tgt.setValue(CapabilityStatement.ResourceVersionPolicy.VERSIONED); 950 break; 951 case VERSIONEDUPDATE: 952 tgt.setValue(CapabilityStatement.ResourceVersionPolicy.VERSIONEDUPDATE); 953 break; 954 default: 955 tgt.setValue(CapabilityStatement.ResourceVersionPolicy.NULL); 956 break; 957 } 958 } 959 return tgt; 960 } 961 962 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CapabilityStatement.RestfulCapabilityMode> convertRestfulCapabilityMode(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.RestfulCapabilityMode> src) throws FHIRException { 963 if (src == null || src.isEmpty()) 964 return null; 965 Enumeration<CapabilityStatement.RestfulCapabilityMode> tgt = new Enumeration<>(new CapabilityStatement.RestfulCapabilityModeEnumFactory()); 966 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 967 if (src.getValue() == null) { 968 tgt.setValue(null); 969 } else { 970 switch (src.getValue()) { 971 case CLIENT: 972 tgt.setValue(CapabilityStatement.RestfulCapabilityMode.CLIENT); 973 break; 974 case SERVER: 975 tgt.setValue(CapabilityStatement.RestfulCapabilityMode.SERVER); 976 break; 977 default: 978 tgt.setValue(CapabilityStatement.RestfulCapabilityMode.NULL); 979 break; 980 } 981 } 982 return tgt; 983 } 984 985 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.RestfulCapabilityMode> convertRestfulCapabilityMode(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CapabilityStatement.RestfulCapabilityMode> src) throws FHIRException { 986 if (src == null || src.isEmpty()) 987 return null; 988 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.RestfulCapabilityMode> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.CapabilityStatement.RestfulCapabilityModeEnumFactory()); 989 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 990 if (src.getValue() == null) { 991 tgt.setValue(null); 992 } else { 993 switch (src.getValue()) { 994 case CLIENT: 995 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.RestfulCapabilityMode.CLIENT); 996 break; 997 case SERVER: 998 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.RestfulCapabilityMode.SERVER); 999 break; 1000 default: 1001 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.RestfulCapabilityMode.NULL); 1002 break; 1003 } 1004 } 1005 return tgt; 1006 } 1007 1008 public static org.hl7.fhir.dstu3.model.CapabilityStatement.SystemInteractionComponent convertSystemInteractionComponent(org.hl7.fhir.r5.model.CapabilityStatement.SystemInteractionComponent src) throws FHIRException { 1009 if (src == null) 1010 return null; 1011 org.hl7.fhir.dstu3.model.CapabilityStatement.SystemInteractionComponent tgt = new org.hl7.fhir.dstu3.model.CapabilityStatement.SystemInteractionComponent(); 1012 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 1013 if (src.hasCode()) 1014 tgt.setCodeElement(convertSystemRestfulInteraction(src.getCodeElement())); 1015 if (src.hasDocumentation()) 1016 tgt.setDocumentation(src.getDocumentation()); 1017 return tgt; 1018 } 1019 1020 public static org.hl7.fhir.r5.model.CapabilityStatement.SystemInteractionComponent convertSystemInteractionComponent(org.hl7.fhir.dstu3.model.CapabilityStatement.SystemInteractionComponent src) throws FHIRException { 1021 if (src == null) 1022 return null; 1023 org.hl7.fhir.r5.model.CapabilityStatement.SystemInteractionComponent tgt = new org.hl7.fhir.r5.model.CapabilityStatement.SystemInteractionComponent(); 1024 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 1025 if (src.hasCode()) 1026 tgt.setCodeElement(convertSystemRestfulInteraction(src.getCodeElement())); 1027 if (src.hasDocumentation()) 1028 tgt.setDocumentation(src.getDocumentation()); 1029 return tgt; 1030 } 1031 1032 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.SystemRestfulInteraction> convertSystemRestfulInteraction(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CapabilityStatement.SystemRestfulInteraction> src) throws FHIRException { 1033 if (src == null || src.isEmpty()) 1034 return null; 1035 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.SystemRestfulInteraction> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.CapabilityStatement.SystemRestfulInteractionEnumFactory()); 1036 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 1037 if (src.getValue() == null) { 1038 tgt.setValue(null); 1039 } else { 1040 switch (src.getValue()) { 1041 case TRANSACTION: 1042 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.SystemRestfulInteraction.TRANSACTION); 1043 break; 1044 case BATCH: 1045 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.SystemRestfulInteraction.BATCH); 1046 break; 1047 case SEARCHSYSTEM: 1048 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.SystemRestfulInteraction.SEARCHSYSTEM); 1049 break; 1050 case HISTORYSYSTEM: 1051 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.SystemRestfulInteraction.HISTORYSYSTEM); 1052 break; 1053 default: 1054 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.SystemRestfulInteraction.NULL); 1055 break; 1056 } 1057 } 1058 return tgt; 1059 } 1060 1061 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CapabilityStatement.SystemRestfulInteraction> convertSystemRestfulInteraction(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.SystemRestfulInteraction> src) throws FHIRException { 1062 if (src == null || src.isEmpty()) 1063 return null; 1064 Enumeration<CapabilityStatement.SystemRestfulInteraction> tgt = new Enumeration<>(new CapabilityStatement.SystemRestfulInteractionEnumFactory()); 1065 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 1066 if (src.getValue() == null) { 1067 tgt.setValue(null); 1068 } else { 1069 switch (src.getValue()) { 1070 case TRANSACTION: 1071 tgt.setValue(CapabilityStatement.SystemRestfulInteraction.TRANSACTION); 1072 break; 1073 case BATCH: 1074 tgt.setValue(CapabilityStatement.SystemRestfulInteraction.BATCH); 1075 break; 1076 case SEARCHSYSTEM: 1077 tgt.setValue(CapabilityStatement.SystemRestfulInteraction.SEARCHSYSTEM); 1078 break; 1079 case HISTORYSYSTEM: 1080 tgt.setValue(CapabilityStatement.SystemRestfulInteraction.HISTORYSYSTEM); 1081 break; 1082 default: 1083 tgt.setValue(CapabilityStatement.SystemRestfulInteraction.NULL); 1084 break; 1085 } 1086 } 1087 return tgt; 1088 } 1089 1090 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.TypeRestfulInteraction> convertTypeRestfulInteraction(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CapabilityStatement.TypeRestfulInteraction> src) throws FHIRException { 1091 if (src == null || src.isEmpty()) 1092 return null; 1093 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.TypeRestfulInteraction> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.CapabilityStatement.TypeRestfulInteractionEnumFactory()); 1094 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 1095 if (src.getValue() == null) { 1096 tgt.setValue(null); 1097 } else { 1098 switch (src.getValue()) { 1099 case READ: 1100 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.TypeRestfulInteraction.READ); 1101 break; 1102 case VREAD: 1103 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.TypeRestfulInteraction.VREAD); 1104 break; 1105 case UPDATE: 1106 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.TypeRestfulInteraction.UPDATE); 1107 break; 1108 case PATCH: 1109 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.TypeRestfulInteraction.PATCH); 1110 break; 1111 case DELETE: 1112 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.TypeRestfulInteraction.DELETE); 1113 break; 1114 case HISTORYINSTANCE: 1115 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.TypeRestfulInteraction.HISTORYINSTANCE); 1116 break; 1117 case HISTORYTYPE: 1118 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.TypeRestfulInteraction.HISTORYTYPE); 1119 break; 1120 case CREATE: 1121 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.TypeRestfulInteraction.CREATE); 1122 break; 1123 case SEARCHTYPE: 1124 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.TypeRestfulInteraction.SEARCHTYPE); 1125 break; 1126 default: 1127 tgt.setValue(org.hl7.fhir.r5.model.CapabilityStatement.TypeRestfulInteraction.NULL); 1128 break; 1129 } 1130 } 1131 return tgt; 1132 } 1133 1134 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CapabilityStatement.TypeRestfulInteraction> convertTypeRestfulInteraction(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.TypeRestfulInteraction> src) throws FHIRException { 1135 if (src == null || src.isEmpty()) 1136 return null; 1137 Enumeration<CapabilityStatement.TypeRestfulInteraction> tgt = new Enumeration<>(new CapabilityStatement.TypeRestfulInteractionEnumFactory()); 1138 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 1139 if (src.getValue() == null) { 1140 tgt.setValue(null); 1141 } else { 1142 switch (src.getValue()) { 1143 case READ: 1144 tgt.setValue(CapabilityStatement.TypeRestfulInteraction.READ); 1145 break; 1146 case VREAD: 1147 tgt.setValue(CapabilityStatement.TypeRestfulInteraction.VREAD); 1148 break; 1149 case UPDATE: 1150 tgt.setValue(CapabilityStatement.TypeRestfulInteraction.UPDATE); 1151 break; 1152 case PATCH: 1153 tgt.setValue(CapabilityStatement.TypeRestfulInteraction.PATCH); 1154 break; 1155 case DELETE: 1156 tgt.setValue(CapabilityStatement.TypeRestfulInteraction.DELETE); 1157 break; 1158 case HISTORYINSTANCE: 1159 tgt.setValue(CapabilityStatement.TypeRestfulInteraction.HISTORYINSTANCE); 1160 break; 1161 case HISTORYTYPE: 1162 tgt.setValue(CapabilityStatement.TypeRestfulInteraction.HISTORYTYPE); 1163 break; 1164 case CREATE: 1165 tgt.setValue(CapabilityStatement.TypeRestfulInteraction.CREATE); 1166 break; 1167 case SEARCHTYPE: 1168 tgt.setValue(CapabilityStatement.TypeRestfulInteraction.SEARCHTYPE); 1169 break; 1170 default: 1171 tgt.setValue(CapabilityStatement.TypeRestfulInteraction.NULL); 1172 break; 1173 } 1174 } 1175 return tgt; 1176 } 1177}