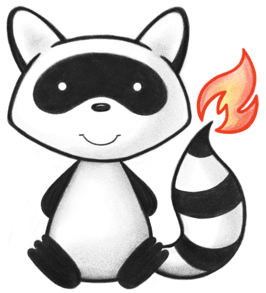
001package org.hl7.fhir.convertors.conv30_50.resources30_50; 002 003import java.util.List; 004 005import org.hl7.fhir.convertors.context.ConversionContext30_50; 006import org.hl7.fhir.convertors.conv30_50.datatypes30_50.Reference30_50; 007import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Annotation30_50; 008import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.CodeableConcept30_50; 009import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Identifier30_50; 010import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Period30_50; 011import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.String30_50; 012import org.hl7.fhir.dstu3.model.CarePlan; 013import org.hl7.fhir.dstu3.model.Enumeration; 014import org.hl7.fhir.dstu3.model.Reference; 015import org.hl7.fhir.exceptions.FHIRException; 016import org.hl7.fhir.r5.model.CodeableReference; 017import org.hl7.fhir.r5.model.Enumerations; 018 019public class CarePlan30_50 { 020 021 private static final String CarePlanActivityDetailComponentExtension = "http://hl7.org/fhir/3.0/StructureDefinition/extension-CarePlan.activity.detail.category"; 022 023 public static org.hl7.fhir.r5.model.CarePlan convertCarePlan(org.hl7.fhir.dstu3.model.CarePlan src) throws FHIRException { 024 if (src == null) 025 return null; 026 org.hl7.fhir.r5.model.CarePlan tgt = new org.hl7.fhir.r5.model.CarePlan(); 027 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 028 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 029 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 030 for (org.hl7.fhir.dstu3.model.Reference t : src.getBasedOn()) { 031 tgt.addBasedOn(Reference30_50.convertReference(t)); 032 } 033 for (org.hl7.fhir.dstu3.model.Reference t : src.getReplaces()) { 034 tgt.addReplaces(Reference30_50.convertReference(t)); 035 } 036 for (org.hl7.fhir.dstu3.model.Reference t : src.getPartOf()) { 037 tgt.addPartOf(Reference30_50.convertReference(t)); 038 } 039 if (src.hasStatus()) { 040 if (src.hasStatus()) 041 tgt.setStatusElement(convertCarePlanStatus(src.getStatusElement())); 042 } 043 if (src.hasIntent()) { 044 if (src.hasIntent()) 045 tgt.setIntentElement(convertCarePlanIntent(src.getIntentElement())); 046 } 047 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getCategory()) { 048 tgt.addCategory(CodeableConcept30_50.convertCodeableConcept(t)); 049 } 050 if (src.hasTitle()) { 051 if (src.hasTitleElement()) 052 tgt.setTitleElement(String30_50.convertString(src.getTitleElement())); 053 } 054 if (src.hasDescription()) { 055 if (src.hasDescriptionElement()) 056 tgt.setDescriptionElement(String30_50.convertString(src.getDescriptionElement())); 057 } 058 if (src.hasSubject()) { 059 if (src.hasSubject()) 060 tgt.setSubject(Reference30_50.convertReference(src.getSubject())); 061 } 062 if (src.hasContext()) { 063 if (src.hasContext()) 064 tgt.setEncounter(Reference30_50.convertReference(src.getContext())); 065 } 066 if (src.hasPeriod()) { 067 if (src.hasPeriod()) 068 tgt.setPeriod(Period30_50.convertPeriod(src.getPeriod())); 069 } 070 List<Reference> authors = src.getAuthor(); 071 if (authors.size() > 0) { 072 tgt.setCustodian(Reference30_50.convertReference(authors.get(0))); 073 if (authors.size() > 1) { 074 } 075 } 076 for (org.hl7.fhir.dstu3.model.Reference t : src.getCareTeam()) { 077 tgt.addCareTeam(Reference30_50.convertReference(t)); 078 } 079 for (org.hl7.fhir.dstu3.model.Reference t : src.getAddresses()) { 080 tgt.addAddresses(Reference30_50.convertReferenceToCodableReference(t)); 081 } 082 for (org.hl7.fhir.dstu3.model.Reference t : src.getSupportingInfo()) { 083 tgt.addSupportingInfo(Reference30_50.convertReference(t)); 084 } 085 for (org.hl7.fhir.dstu3.model.Reference t : src.getGoal()) { 086 tgt.addGoal(Reference30_50.convertReference(t)); 087 } 088 for (org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityComponent t : src.getActivity()) { 089 tgt.addActivity(convertCarePlanActivityComponent(t)); 090 } 091 for (org.hl7.fhir.dstu3.model.Annotation t : src.getNote()) { 092 tgt.addNote(Annotation30_50.convertAnnotation(t)); 093 } 094 return tgt; 095 } 096 097 public static org.hl7.fhir.dstu3.model.CarePlan convertCarePlan(org.hl7.fhir.r5.model.CarePlan src) throws FHIRException { 098 if (src == null) 099 return null; 100 org.hl7.fhir.dstu3.model.CarePlan tgt = new org.hl7.fhir.dstu3.model.CarePlan(); 101 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 102 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 103 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 104 for (org.hl7.fhir.r5.model.Reference t : src.getBasedOn()) { 105 tgt.addBasedOn(Reference30_50.convertReference(t)); 106 } 107 for (org.hl7.fhir.r5.model.Reference t : src.getReplaces()) { 108 tgt.addReplaces(Reference30_50.convertReference(t)); 109 } 110 for (org.hl7.fhir.r5.model.Reference t : src.getPartOf()) { 111 tgt.addPartOf(Reference30_50.convertReference(t)); 112 } 113 if (src.hasStatus()) { 114 if (src.hasStatus()) 115 tgt.setStatusElement(convertCarePlanStatus(src.getStatusElement())); 116 } 117 if (src.hasIntent()) { 118 if (src.hasIntent()) 119 tgt.setIntentElement(convertCarePlanIntent(src.getIntentElement())); 120 } 121 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getCategory()) { 122 tgt.addCategory(CodeableConcept30_50.convertCodeableConcept(t)); 123 } 124 if (src.hasTitle()) { 125 if (src.hasTitleElement()) 126 tgt.setTitleElement(String30_50.convertString(src.getTitleElement())); 127 } 128 if (src.hasDescription()) { 129 if (src.hasDescriptionElement()) 130 tgt.setDescriptionElement(String30_50.convertString(src.getDescriptionElement())); 131 } 132 if (src.hasSubject()) { 133 if (src.hasSubject()) 134 tgt.setSubject(Reference30_50.convertReference(src.getSubject())); 135 } 136 if (src.hasEncounter()) { 137 if (src.hasEncounter()) 138 tgt.setContext(Reference30_50.convertReference(src.getEncounter())); 139 } 140 if (src.hasPeriod()) { 141 if (src.hasPeriod()) 142 tgt.setPeriod(Period30_50.convertPeriod(src.getPeriod())); 143 } 144 if (src.hasCustodian()) { 145 tgt.addAuthor(Reference30_50.convertReference(src.getCustodian())); 146 } 147 for (org.hl7.fhir.r5.model.Reference t : src.getCareTeam()) { 148 tgt.addCareTeam(Reference30_50.convertReference(t)); 149 } 150 for (CodeableReference t : src.getAddresses()) { 151 if (t.hasReference()) 152 tgt.addAddresses(Reference30_50.convertReference(t.getReference())); 153 } 154 for (org.hl7.fhir.r5.model.Reference t : src.getSupportingInfo()) { 155 tgt.addSupportingInfo(Reference30_50.convertReference(t)); 156 } 157 for (org.hl7.fhir.r5.model.Reference t : src.getGoal()) { 158 tgt.addGoal(Reference30_50.convertReference(t)); 159 } 160 for (org.hl7.fhir.r5.model.CarePlan.CarePlanActivityComponent t : src.getActivity()) { 161 tgt.addActivity(convertCarePlanActivityComponent(t)); 162 } 163 for (org.hl7.fhir.r5.model.Annotation t : src.getNote()) { 164 tgt.addNote(Annotation30_50.convertAnnotation(t)); 165 } 166 return tgt; 167 } 168 169 public static org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityComponent convertCarePlanActivityComponent(org.hl7.fhir.r5.model.CarePlan.CarePlanActivityComponent src) throws FHIRException { 170 if (src == null) 171 return null; 172 org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityComponent tgt = new org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityComponent(); 173 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 174 for (CodeableReference t : src.getPerformedActivity()) { 175 if (t.hasConcept()) 176 tgt.addOutcomeCodeableConcept(CodeableConcept30_50.convertCodeableConcept(t.getConcept())); 177 } 178 for (CodeableReference t : src.getPerformedActivity()) { 179 if (t.hasReference()) 180 tgt.addOutcomeReference(Reference30_50.convertReference(t.getReference())); 181 } 182 for (org.hl7.fhir.r5.model.Annotation t : src.getProgress()) { 183 tgt.addProgress(Annotation30_50.convertAnnotation(t)); 184 } 185 if (src.hasPlannedActivityReference()) { 186 if (src.hasPlannedActivityReference()) 187 tgt.setReference(Reference30_50.convertReference(src.getPlannedActivityReference())); 188 } 189// if (src.hasPlannedActivityDetail()) { 190// if (src.hasPlannedActivityDetail()) 191// tgt.setDetail(convertCarePlanActivityDetailComponent(src.getPlannedActivityDetail())); 192// } 193 return tgt; 194 } 195 196 public static org.hl7.fhir.r5.model.CarePlan.CarePlanActivityComponent convertCarePlanActivityComponent(org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityComponent src) throws FHIRException { 197 if (src == null) 198 return null; 199 org.hl7.fhir.r5.model.CarePlan.CarePlanActivityComponent tgt = new org.hl7.fhir.r5.model.CarePlan.CarePlanActivityComponent(); 200 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 201 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getOutcomeCodeableConcept()) { 202 tgt.addPerformedActivity(Reference30_50.convertCodeableConceptToCodableReference(t)); 203 } 204 for (org.hl7.fhir.dstu3.model.Reference t : src.getOutcomeReference()) { 205 tgt.addPerformedActivity(Reference30_50.convertReferenceToCodableReference(t)); 206 } 207 for (org.hl7.fhir.dstu3.model.Annotation t : src.getProgress()) { 208 tgt.addProgress(Annotation30_50.convertAnnotation(t)); 209 } 210 if (src.hasReference()) { 211 if (src.hasReference()) 212 tgt.setPlannedActivityReference(Reference30_50.convertReference(src.getReference())); 213 } 214// if (src.hasDetail()) { 215// if (src.hasDetail()) 216// tgt.setPlannedActivityDetail(convertCarePlanActivityDetailComponent(src.getDetail())); 217// } 218 return tgt; 219 } 220 221// public static org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityDetailComponent convertCarePlanActivityDetailComponent(org.hl7.fhir.r5.model.CarePlan.CarePlanActivityPlannedActivityDetailComponent src) throws FHIRException { 222// if (src == null) 223// return null; 224// org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityDetailComponent tgt = new org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityDetailComponent(); 225// ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 226// if (src.hasExtension()) { 227// org.hl7.fhir.r5.model.Extension extension = src.getExtensionByUrl(CarePlanActivityDetailComponentExtension); 228// if (extension != null) { 229// org.hl7.fhir.r5.model.DataType value = extension.getValue(); 230// if (value instanceof org.hl7.fhir.r5.model.CodeableConcept) { 231// tgt.setCategory(CodeableConcept30_50.convertCodeableConcept((org.hl7.fhir.r5.model.CodeableConcept) value)); 232// } 233// } 234// } 235// if (src.hasCode()) { 236// if (src.hasCode()) 237// tgt.setCode(CodeableConcept30_50.convertCodeableConcept(src.getCode())); 238// } 239// for (CodeableReference t : src.getReason()) { 240// if (t.hasConcept()) 241// tgt.addReasonCode(CodeableConcept30_50.convertCodeableConcept(t.getConcept())); 242// } 243// for (CodeableReference t : src.getReason()) { 244// if (t.hasReference()) 245// tgt.addReasonReference(Reference30_50.convertReference(t.getReference())); 246// } 247// for (org.hl7.fhir.r5.model.Reference t : src.getGoal()) { 248// tgt.addGoal(Reference30_50.convertReference(t)); 249// } 250// if (src.hasStatus()) { 251// if (src.hasStatus()) 252// tgt.setStatusElement(convertCarePlanActivityStatus(src.getStatusElement())); 253// } 254// if (src.hasStatusReason()) { 255// List<Coding> coding = src.getStatusReason().getCoding(); 256// if (coding.size() > 0) { 257// tgt.setStatusReason(coding.get(0).getCode()); 258// } 259// } 260// if (src.hasDoNotPerform()) { 261// if (src.hasDoNotPerformElement()) 262// tgt.setProhibitedElement(Boolean30_50.convertBoolean(src.getDoNotPerformElement())); 263// } 264// if (src.hasScheduled()) { 265// if (src.hasScheduled()) 266// tgt.setScheduled(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getScheduled())); 267// } 268// if (src.hasLocation()) { 269// if (src.getLocation().hasReference()) 270// tgt.setLocation(Reference30_50.convertReference(src.getLocation().getReference())); 271// } 272// for (org.hl7.fhir.r5.model.Reference t : src.getPerformer()) { 273// tgt.addPerformer(Reference30_50.convertReference(t)); 274// } 275// if (src.hasProduct()) { 276// if (src.hasProduct()) 277// tgt.setProduct(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getProduct())); 278// } 279// if (src.hasDailyAmount()) { 280// if (src.hasDailyAmount()) 281// tgt.setDailyAmount(SimpleQuantity30_50.convertSimpleQuantity(src.getDailyAmount())); 282// } 283// if (src.hasQuantity()) { 284// if (src.hasQuantity()) 285// tgt.setQuantity(SimpleQuantity30_50.convertSimpleQuantity(src.getQuantity())); 286// } 287// if (src.hasDescription()) { 288// if (src.hasDescriptionElement()) 289// tgt.setDescriptionElement(String30_50.convertString(src.getDescriptionElement())); 290// } 291// return tgt; 292// } 293// 294// public static org.hl7.fhir.r5.model.CarePlan.CarePlanActivityPlannedActivityDetailComponent convertCarePlanActivityDetailComponent(org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityDetailComponent src) throws FHIRException { 295// if (src == null) 296// return null; 297// org.hl7.fhir.r5.model.CarePlan.CarePlanActivityPlannedActivityDetailComponent tgt = new org.hl7.fhir.r5.model.CarePlan.CarePlanActivityPlannedActivityDetailComponent(); 298// ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 299// if (src.hasCategory()) { 300// org.hl7.fhir.r5.model.Extension t = new org.hl7.fhir.r5.model.Extension(); 301// t.setUrl(CarePlanActivityDetailComponentExtension); 302// t.setValue(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getCategory())); 303// tgt.addExtension(t); 304// } 305// if (src.hasCode()) { 306// if (src.hasCode()) 307// tgt.setCode(CodeableConcept30_50.convertCodeableConcept(src.getCode())); 308// } 309// for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getReasonCode()) { 310// tgt.addReason(Reference30_50.convertCodeableConceptToCodableReference(t)); 311// } 312// for (org.hl7.fhir.dstu3.model.Reference t : src.getReasonReference()) { 313// tgt.addReason(Reference30_50.convertReferenceToCodableReference(t)); 314// } 315// for (org.hl7.fhir.dstu3.model.Reference t : src.getGoal()) { 316// tgt.addGoal(Reference30_50.convertReference(t)); 317// } 318// if (src.hasStatus()) { 319// if (src.hasStatus()) 320// tgt.setStatusElement(convertCarePlanActivityStatus(src.getStatusElement())); 321// } 322// if (src.hasStatusReason()) { 323// org.hl7.fhir.r5.model.Coding code = new org.hl7.fhir.r5.model.Coding(); 324// code.setCode(src.getStatusReason()); 325// org.hl7.fhir.r5.model.CodeableConcept t = new org.hl7.fhir.r5.model.CodeableConcept(code); 326// tgt.setStatusReason(t); 327// } 328// if (src.hasProhibited()) { 329// if (src.hasProhibitedElement()) 330// tgt.setDoNotPerformElement(Boolean30_50.convertBoolean(src.getProhibitedElement())); 331// } 332// if (src.hasScheduled()) { 333// if (src.hasScheduled()) 334// tgt.setScheduled(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getScheduled())); 335// } 336// if (src.hasLocation()) { 337// if (src.hasLocation()) 338// tgt.getLocation().setReference(Reference30_50.convertReference(src.getLocation())); 339// } 340// for (org.hl7.fhir.dstu3.model.Reference t : src.getPerformer()) { 341// tgt.addPerformer(Reference30_50.convertReference(t)); 342// } 343// if (src.hasProduct()) { 344// if (src.hasProduct()) 345// tgt.setProduct(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getProduct())); 346// } 347// if (src.hasDailyAmount()) { 348// if (src.hasDailyAmount()) 349// tgt.setDailyAmount(SimpleQuantity30_50.convertSimpleQuantity(src.getDailyAmount())); 350// } 351// if (src.hasQuantity()) { 352// if (src.hasQuantity()) 353// tgt.setQuantity(SimpleQuantity30_50.convertSimpleQuantity(src.getQuantity())); 354// } 355// if (src.hasDescription()) { 356// if (src.hasDescriptionElement()) 357// tgt.setDescriptionElement(String30_50.convertString(src.getDescriptionElement())); 358// } 359// return tgt; 360// } 361// 362// static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CarePlan.CarePlanActivityStatus> convertCarePlanActivityStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityStatus> src) throws FHIRException { 363// if (src == null || src.isEmpty()) 364// return null; 365// org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CarePlan.CarePlanActivityStatus> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.CarePlan.CarePlanActivityStatusEnumFactory()); 366// ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 367// switch (src.getValue()) { 368// case CANCELLED: 369// tgt.setValue(org.hl7.fhir.r5.model.CarePlan.CarePlanActivityStatus.CANCELLED); 370// break; 371// case COMPLETED: 372// tgt.setValue(org.hl7.fhir.r5.model.CarePlan.CarePlanActivityStatus.COMPLETED); 373// break; 374// case INPROGRESS: 375// tgt.setValue(org.hl7.fhir.r5.model.CarePlan.CarePlanActivityStatus.INPROGRESS); 376// break; 377// case NOTSTARTED: 378// tgt.setValue(org.hl7.fhir.r5.model.CarePlan.CarePlanActivityStatus.NOTSTARTED); 379// break; 380// case ONHOLD: 381// tgt.setValue(org.hl7.fhir.r5.model.CarePlan.CarePlanActivityStatus.ONHOLD); 382// break; 383// case SCHEDULED: 384// tgt.setValue(org.hl7.fhir.r5.model.CarePlan.CarePlanActivityStatus.SCHEDULED); 385// break; 386// case UNKNOWN: 387// tgt.setValue(org.hl7.fhir.r5.model.CarePlan.CarePlanActivityStatus.UNKNOWN); 388// break; 389// default: 390// tgt.setValue(org.hl7.fhir.r5.model.CarePlan.CarePlanActivityStatus.NULL); 391// break; 392// } 393// return tgt; 394// } 395// 396// static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityStatus> convertCarePlanActivityStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CarePlan.CarePlanActivityStatus> src) throws FHIRException { 397// if (src == null || src.isEmpty()) 398// return null; 399// org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityStatus> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityStatusEnumFactory()); 400// ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 401// switch (src.getValue()) { 402// case CANCELLED: 403// tgt.setValue(org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityStatus.CANCELLED); 404// break; 405// case COMPLETED: 406// tgt.setValue(org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityStatus.COMPLETED); 407// break; 408// case INPROGRESS: 409// tgt.setValue(org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityStatus.INPROGRESS); 410// break; 411// case NOTSTARTED: 412// tgt.setValue(org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityStatus.NOTSTARTED); 413// break; 414// case ONHOLD: 415// tgt.setValue(org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityStatus.ONHOLD); 416// break; 417// case SCHEDULED: 418// tgt.setValue(org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityStatus.SCHEDULED); 419// break; 420// case UNKNOWN: 421// tgt.setValue(org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityStatus.UNKNOWN); 422// break; 423// default: 424// tgt.setValue(org.hl7.fhir.dstu3.model.CarePlan.CarePlanActivityStatus.NULL); 425// break; 426// } 427// return tgt; 428// } 429 430 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CarePlan.CarePlanIntent> convertCarePlanIntent(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CarePlan.CarePlanIntent> src) throws FHIRException { 431 if (src == null || src.isEmpty()) 432 return null; 433 Enumeration<CarePlan.CarePlanIntent> tgt = new Enumeration<>(new CarePlan.CarePlanIntentEnumFactory()); 434 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 435 if (src.getValue() == null) { 436 tgt.setValue(null); 437 } else { 438 switch (src.getValue()) { 439 case OPTION: 440 tgt.setValue(CarePlan.CarePlanIntent.OPTION); 441 break; 442 case ORDER: 443 tgt.setValue(CarePlan.CarePlanIntent.ORDER); 444 break; 445 case PLAN: 446 tgt.setValue(CarePlan.CarePlanIntent.PLAN); 447 break; 448 case PROPOSAL: 449 tgt.setValue(CarePlan.CarePlanIntent.PROPOSAL); 450 break; 451 default: 452 tgt.setValue(CarePlan.CarePlanIntent.NULL); 453 break; 454 } 455 } 456 return tgt; 457 } 458 459 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CarePlan.CarePlanIntent> convertCarePlanIntent(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CarePlan.CarePlanIntent> src) throws FHIRException { 460 if (src == null || src.isEmpty()) 461 return null; 462 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CarePlan.CarePlanIntent> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.CarePlan.CarePlanIntentEnumFactory()); 463 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 464 if (src.getValue() == null) { 465 tgt.setValue(null); 466 } else { 467 switch (src.getValue()) { 468 case OPTION: 469 tgt.setValue(org.hl7.fhir.r5.model.CarePlan.CarePlanIntent.OPTION); 470 break; 471 case ORDER: 472 tgt.setValue(org.hl7.fhir.r5.model.CarePlan.CarePlanIntent.ORDER); 473 break; 474 case PLAN: 475 tgt.setValue(org.hl7.fhir.r5.model.CarePlan.CarePlanIntent.PLAN); 476 break; 477 case PROPOSAL: 478 tgt.setValue(org.hl7.fhir.r5.model.CarePlan.CarePlanIntent.PROPOSAL); 479 break; 480 default: 481 tgt.setValue(org.hl7.fhir.r5.model.CarePlan.CarePlanIntent.NULL); 482 break; 483 } 484 } 485 return tgt; 486 } 487 488 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CarePlan.CarePlanStatus> convertCarePlanStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.RequestStatus> src) throws FHIRException { 489 if (src == null || src.isEmpty()) 490 return null; 491 Enumeration<CarePlan.CarePlanStatus> tgt = new Enumeration<>(new CarePlan.CarePlanStatusEnumFactory()); 492 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 493 if (src.getValue() == null) { 494 tgt.setValue(null); 495 } else { 496 switch (src.getValue()) { 497 case ACTIVE: 498 tgt.setValue(CarePlan.CarePlanStatus.ACTIVE); 499 break; 500 case REVOKED: 501 tgt.setValue(CarePlan.CarePlanStatus.CANCELLED); 502 break; 503 case COMPLETED: 504 tgt.setValue(CarePlan.CarePlanStatus.COMPLETED); 505 break; 506 case DRAFT: 507 tgt.setValue(CarePlan.CarePlanStatus.DRAFT); 508 break; 509 case ENTEREDINERROR: 510 tgt.setValue(CarePlan.CarePlanStatus.ENTEREDINERROR); 511 break; 512 case ONHOLD: 513 tgt.setValue(CarePlan.CarePlanStatus.SUSPENDED); 514 break; 515 case UNKNOWN: 516 tgt.setValue(CarePlan.CarePlanStatus.UNKNOWN); 517 break; 518 default: 519 tgt.setValue(CarePlan.CarePlanStatus.NULL); 520 break; 521 } 522 } 523 return tgt; 524 } 525 526 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.RequestStatus> convertCarePlanStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CarePlan.CarePlanStatus> src) throws FHIRException { 527 if (src == null || src.isEmpty()) 528 return null; 529 org.hl7.fhir.r5.model.Enumeration<Enumerations.RequestStatus> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new Enumerations.RequestStatusEnumFactory()); 530 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 531 if (src.getValue() == null) { 532 tgt.setValue(null); 533 } else { 534 switch (src.getValue()) { 535 case ACTIVE: 536 tgt.setValue(Enumerations.RequestStatus.ACTIVE); 537 break; 538 case CANCELLED: 539 tgt.setValue(Enumerations.RequestStatus.REVOKED); 540 break; 541 case COMPLETED: 542 tgt.setValue(Enumerations.RequestStatus.COMPLETED); 543 break; 544 case DRAFT: 545 tgt.setValue(Enumerations.RequestStatus.DRAFT); 546 break; 547 case ENTEREDINERROR: 548 tgt.setValue(Enumerations.RequestStatus.ENTEREDINERROR); 549 break; 550 case SUSPENDED: 551 tgt.setValue(Enumerations.RequestStatus.ONHOLD); 552 break; 553 case UNKNOWN: 554 tgt.setValue(Enumerations.RequestStatus.UNKNOWN); 555 break; 556 default: 557 tgt.setValue(Enumerations.RequestStatus.NULL); 558 break; 559 } 560 } 561 return tgt; 562 } 563}