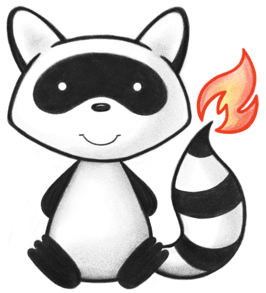
001package org.hl7.fhir.convertors.conv30_50.resources30_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_50; 004import org.hl7.fhir.convertors.conv30_50.datatypes30_50.Reference30_50; 005import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Annotation30_50; 006import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.CodeableConcept30_50; 007import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Identifier30_50; 008import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Period30_50; 009import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.String30_50; 010import org.hl7.fhir.dstu3.model.CareTeam; 011import org.hl7.fhir.dstu3.model.Enumeration; 012import org.hl7.fhir.exceptions.FHIRException; 013import org.hl7.fhir.r5.model.CodeableReference; 014 015public class CareTeam30_50 { 016 017 public static org.hl7.fhir.r5.model.CareTeam convertCareTeam(org.hl7.fhir.dstu3.model.CareTeam src) throws FHIRException { 018 if (src == null) 019 return null; 020 org.hl7.fhir.r5.model.CareTeam tgt = new org.hl7.fhir.r5.model.CareTeam(); 021 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 022 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 023 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 024 if (src.hasStatus()) 025 tgt.setStatusElement(convertCareTeamStatus(src.getStatusElement())); 026 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getCategory()) 027 tgt.addCategory(CodeableConcept30_50.convertCodeableConcept(t)); 028 if (src.hasName()) 029 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 030 if (src.hasSubject()) 031 tgt.setSubject(Reference30_50.convertReference(src.getSubject())); 032 if (src.hasPeriod()) 033 tgt.setPeriod(Period30_50.convertPeriod(src.getPeriod())); 034 for (org.hl7.fhir.dstu3.model.CareTeam.CareTeamParticipantComponent t : src.getParticipant()) 035 tgt.addParticipant(convertCareTeamParticipantComponent(t)); 036 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getReasonCode()) 037 tgt.addReason(Reference30_50.convertCodeableConceptToCodableReference(t)); 038 for (org.hl7.fhir.dstu3.model.Reference t : src.getReasonReference()) 039 tgt.addReason(Reference30_50.convertReferenceToCodableReference(t)); 040 for (org.hl7.fhir.dstu3.model.Reference t : src.getManagingOrganization()) 041 tgt.addManagingOrganization(Reference30_50.convertReference(t)); 042 for (org.hl7.fhir.dstu3.model.Annotation t : src.getNote()) tgt.addNote(Annotation30_50.convertAnnotation(t)); 043 return tgt; 044 } 045 046 public static org.hl7.fhir.dstu3.model.CareTeam convertCareTeam(org.hl7.fhir.r5.model.CareTeam src) throws FHIRException { 047 if (src == null) 048 return null; 049 org.hl7.fhir.dstu3.model.CareTeam tgt = new org.hl7.fhir.dstu3.model.CareTeam(); 050 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 051 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 052 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 053 if (src.hasStatus()) 054 tgt.setStatusElement(convertCareTeamStatus(src.getStatusElement())); 055 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getCategory()) 056 tgt.addCategory(CodeableConcept30_50.convertCodeableConcept(t)); 057 if (src.hasName()) 058 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 059 if (src.hasSubject()) 060 tgt.setSubject(Reference30_50.convertReference(src.getSubject())); 061 if (src.hasPeriod()) 062 tgt.setPeriod(Period30_50.convertPeriod(src.getPeriod())); 063 for (org.hl7.fhir.r5.model.CareTeam.CareTeamParticipantComponent t : src.getParticipant()) 064 tgt.addParticipant(convertCareTeamParticipantComponent(t)); 065 for (CodeableReference t : src.getReason()) 066 if (t.hasConcept()) 067 tgt.addReasonCode(CodeableConcept30_50.convertCodeableConcept(t.getConcept())); 068 for (CodeableReference t : src.getReason()) 069 if (t.hasReference()) 070 tgt.addReasonReference(Reference30_50.convertReference(t.getReference())); 071 for (org.hl7.fhir.r5.model.Reference t : src.getManagingOrganization()) 072 tgt.addManagingOrganization(Reference30_50.convertReference(t)); 073 for (org.hl7.fhir.r5.model.Annotation t : src.getNote()) tgt.addNote(Annotation30_50.convertAnnotation(t)); 074 return tgt; 075 } 076 077 public static org.hl7.fhir.dstu3.model.CareTeam.CareTeamParticipantComponent convertCareTeamParticipantComponent(org.hl7.fhir.r5.model.CareTeam.CareTeamParticipantComponent src) throws FHIRException { 078 if (src == null) 079 return null; 080 org.hl7.fhir.dstu3.model.CareTeam.CareTeamParticipantComponent tgt = new org.hl7.fhir.dstu3.model.CareTeam.CareTeamParticipantComponent(); 081 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 082 if (src.hasRole()) 083 tgt.setRole(CodeableConcept30_50.convertCodeableConcept(src.getRole())); 084 if (src.hasMember()) 085 tgt.setMember(Reference30_50.convertReference(src.getMember())); 086 if (src.hasOnBehalfOf()) 087 tgt.setOnBehalfOf(Reference30_50.convertReference(src.getOnBehalfOf())); 088 if (src.hasCoveragePeriod()) 089 tgt.setPeriod(Period30_50.convertPeriod(src.getCoveragePeriod())); 090 return tgt; 091 } 092 093 public static org.hl7.fhir.r5.model.CareTeam.CareTeamParticipantComponent convertCareTeamParticipantComponent(org.hl7.fhir.dstu3.model.CareTeam.CareTeamParticipantComponent src) throws FHIRException { 094 if (src == null) 095 return null; 096 org.hl7.fhir.r5.model.CareTeam.CareTeamParticipantComponent tgt = new org.hl7.fhir.r5.model.CareTeam.CareTeamParticipantComponent(); 097 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 098 if (src.hasRole()) 099 tgt.setRole(CodeableConcept30_50.convertCodeableConcept(src.getRole())); 100 if (src.hasMember()) 101 tgt.setMember(Reference30_50.convertReference(src.getMember())); 102 if (src.hasOnBehalfOf()) 103 tgt.setOnBehalfOf(Reference30_50.convertReference(src.getOnBehalfOf())); 104 if (src.hasPeriod()) 105 tgt.setCoverage(Period30_50.convertPeriod(src.getPeriod())); 106 return tgt; 107 } 108 109 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CareTeam.CareTeamStatus> convertCareTeamStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CareTeam.CareTeamStatus> src) throws FHIRException { 110 if (src == null || src.isEmpty()) 111 return null; 112 Enumeration<CareTeam.CareTeamStatus> tgt = new Enumeration<>(new CareTeam.CareTeamStatusEnumFactory()); 113 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 114 if (src.getValue() == null) { 115 tgt.setValue(null); 116 } else { 117 switch (src.getValue()) { 118 case PROPOSED: 119 tgt.setValue(CareTeam.CareTeamStatus.PROPOSED); 120 break; 121 case ACTIVE: 122 tgt.setValue(CareTeam.CareTeamStatus.ACTIVE); 123 break; 124 case SUSPENDED: 125 tgt.setValue(CareTeam.CareTeamStatus.SUSPENDED); 126 break; 127 case INACTIVE: 128 tgt.setValue(CareTeam.CareTeamStatus.INACTIVE); 129 break; 130 case ENTEREDINERROR: 131 tgt.setValue(CareTeam.CareTeamStatus.ENTEREDINERROR); 132 break; 133 default: 134 tgt.setValue(CareTeam.CareTeamStatus.NULL); 135 break; 136 } 137 } 138 return tgt; 139 } 140 141 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CareTeam.CareTeamStatus> convertCareTeamStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CareTeam.CareTeamStatus> src) throws FHIRException { 142 if (src == null || src.isEmpty()) 143 return null; 144 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CareTeam.CareTeamStatus> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.CareTeam.CareTeamStatusEnumFactory()); 145 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 146 if (src.getValue() == null) { 147 tgt.setValue(null); 148 } else { 149 switch (src.getValue()) { 150 case PROPOSED: 151 tgt.setValue(org.hl7.fhir.r5.model.CareTeam.CareTeamStatus.PROPOSED); 152 break; 153 case ACTIVE: 154 tgt.setValue(org.hl7.fhir.r5.model.CareTeam.CareTeamStatus.ACTIVE); 155 break; 156 case SUSPENDED: 157 tgt.setValue(org.hl7.fhir.r5.model.CareTeam.CareTeamStatus.SUSPENDED); 158 break; 159 case INACTIVE: 160 tgt.setValue(org.hl7.fhir.r5.model.CareTeam.CareTeamStatus.INACTIVE); 161 break; 162 case ENTEREDINERROR: 163 tgt.setValue(org.hl7.fhir.r5.model.CareTeam.CareTeamStatus.ENTEREDINERROR); 164 break; 165 default: 166 tgt.setValue(org.hl7.fhir.r5.model.CareTeam.CareTeamStatus.NULL); 167 break; 168 } 169 } 170 return tgt; 171 } 172}