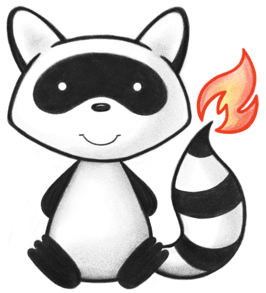
001package org.hl7.fhir.convertors.conv30_50.resources30_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_50; 004import org.hl7.fhir.convertors.conv30_50.datatypes30_50.Reference30_50; 005import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Annotation30_50; 006import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.CodeableConcept30_50; 007import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Identifier30_50; 008import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.DateTime30_50; 009import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.String30_50; 010import org.hl7.fhir.dstu3.model.ClinicalImpression; 011import org.hl7.fhir.dstu3.model.Enumeration; 012import org.hl7.fhir.exceptions.FHIRException; 013import org.hl7.fhir.r5.model.Enumerations; 014 015public class ClinicalImpression30_50 { 016 017 public static org.hl7.fhir.dstu3.model.ClinicalImpression convertClinicalImpression(org.hl7.fhir.r5.model.ClinicalImpression src) throws FHIRException { 018 if (src == null) 019 return null; 020 org.hl7.fhir.dstu3.model.ClinicalImpression tgt = new org.hl7.fhir.dstu3.model.ClinicalImpression(); 021 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 022 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 023 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 024 if (src.hasStatus()) 025 tgt.setStatusElement(convertClinicalImpressionStatus(src.getStatusElement())); 026 if (src.hasDescription()) 027 tgt.setDescriptionElement(String30_50.convertString(src.getDescriptionElement())); 028 if (src.hasSubject()) 029 tgt.setSubject(Reference30_50.convertReference(src.getSubject())); 030 if (src.hasEncounter()) 031 tgt.setContext(Reference30_50.convertReference(src.getEncounter())); 032 if (src.hasEffective()) 033 tgt.setEffective(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getEffective())); 034 if (src.hasDate()) 035 tgt.setDateElement(DateTime30_50.convertDateTime(src.getDateElement())); 036 if (src.hasPerformer()) 037 tgt.setAssessor(Reference30_50.convertReference(src.getPerformer())); 038 if (src.hasPrevious()) 039 tgt.setPrevious(Reference30_50.convertReference(src.getPrevious())); 040 for (org.hl7.fhir.r5.model.Reference t : src.getProblem()) tgt.addProblem(Reference30_50.convertReference(t)); 041 for (org.hl7.fhir.r5.model.UriType t : src.getProtocol()) tgt.addProtocol(t.getValue()); 042 if (src.hasSummary()) 043 tgt.setSummaryElement(String30_50.convertString(src.getSummaryElement())); 044 for (org.hl7.fhir.r5.model.ClinicalImpression.ClinicalImpressionFindingComponent t : src.getFinding()) 045 tgt.addFinding(convertClinicalImpressionFindingComponent(t)); 046 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getPrognosisCodeableConcept()) 047 tgt.addPrognosisCodeableConcept(CodeableConcept30_50.convertCodeableConcept(t)); 048 for (org.hl7.fhir.r5.model.Reference t : src.getPrognosisReference()) 049 tgt.addPrognosisReference(Reference30_50.convertReference(t)); 050 for (org.hl7.fhir.r5.model.Annotation t : src.getNote()) tgt.addNote(Annotation30_50.convertAnnotation(t)); 051 return tgt; 052 } 053 054 public static org.hl7.fhir.r5.model.ClinicalImpression convertClinicalImpression(org.hl7.fhir.dstu3.model.ClinicalImpression src) throws FHIRException { 055 if (src == null) 056 return null; 057 org.hl7.fhir.r5.model.ClinicalImpression tgt = new org.hl7.fhir.r5.model.ClinicalImpression(); 058 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 059 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 060 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 061 if (src.hasStatus()) 062 tgt.setStatusElement(convertClinicalImpressionStatus(src.getStatusElement())); 063 if (src.hasDescription()) 064 tgt.setDescriptionElement(String30_50.convertString(src.getDescriptionElement())); 065 if (src.hasSubject()) 066 tgt.setSubject(Reference30_50.convertReference(src.getSubject())); 067 if (src.hasContext()) 068 tgt.setEncounter(Reference30_50.convertReference(src.getContext())); 069 if (src.hasEffective()) 070 tgt.setEffective(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getEffective())); 071 if (src.hasDate()) 072 tgt.setDateElement(DateTime30_50.convertDateTime(src.getDateElement())); 073 if (src.hasAssessor()) 074 tgt.setPerformer(Reference30_50.convertReference(src.getAssessor())); 075 if (src.hasPrevious()) 076 tgt.setPrevious(Reference30_50.convertReference(src.getPrevious())); 077 for (org.hl7.fhir.dstu3.model.Reference t : src.getProblem()) tgt.addProblem(Reference30_50.convertReference(t)); 078 for (org.hl7.fhir.dstu3.model.UriType t : src.getProtocol()) tgt.addProtocol(t.getValue()); 079 if (src.hasSummary()) 080 tgt.setSummaryElement(String30_50.convertString(src.getSummaryElement())); 081 for (org.hl7.fhir.dstu3.model.ClinicalImpression.ClinicalImpressionFindingComponent t : src.getFinding()) 082 tgt.addFinding(convertClinicalImpressionFindingComponent(t)); 083 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getPrognosisCodeableConcept()) 084 tgt.addPrognosisCodeableConcept(CodeableConcept30_50.convertCodeableConcept(t)); 085 for (org.hl7.fhir.dstu3.model.Reference t : src.getPrognosisReference()) 086 tgt.addPrognosisReference(Reference30_50.convertReference(t)); 087 for (org.hl7.fhir.dstu3.model.Annotation t : src.getNote()) tgt.addNote(Annotation30_50.convertAnnotation(t)); 088 return tgt; 089 } 090 091 public static org.hl7.fhir.dstu3.model.ClinicalImpression.ClinicalImpressionFindingComponent convertClinicalImpressionFindingComponent(org.hl7.fhir.r5.model.ClinicalImpression.ClinicalImpressionFindingComponent src) throws FHIRException { 092 if (src == null) 093 return null; 094 org.hl7.fhir.dstu3.model.ClinicalImpression.ClinicalImpressionFindingComponent tgt = new org.hl7.fhir.dstu3.model.ClinicalImpression.ClinicalImpressionFindingComponent(); 095 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 096 if (src.hasItem() && src.getItem().hasConcept()) 097 tgt.setItem(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getItem().getConcept())); 098 else if (src.hasItem() && src.getItem().hasReference()) 099 tgt.setItem(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getItem().getReference())); 100 if (src.hasBasis()) 101 tgt.setBasisElement(String30_50.convertString(src.getBasisElement())); 102 return tgt; 103 } 104 105 public static org.hl7.fhir.r5.model.ClinicalImpression.ClinicalImpressionFindingComponent convertClinicalImpressionFindingComponent(org.hl7.fhir.dstu3.model.ClinicalImpression.ClinicalImpressionFindingComponent src) throws FHIRException { 106 if (src == null) 107 return null; 108 org.hl7.fhir.r5.model.ClinicalImpression.ClinicalImpressionFindingComponent tgt = new org.hl7.fhir.r5.model.ClinicalImpression.ClinicalImpressionFindingComponent(); 109 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 110 if (src.hasItemCodeableConcept()) 111 tgt.setItem(Reference30_50.convertCodeableConceptToCodableReference(src.getItemCodeableConcept())); 112 if (src.hasItemReference()) 113 tgt.setItem(Reference30_50.convertReferenceToCodableReference(src.getItemReference())); 114 if (src.hasBasis()) 115 tgt.setBasisElement(String30_50.convertString(src.getBasisElement())); 116 return tgt; 117 } 118 119 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ClinicalImpression.ClinicalImpressionStatus> convertClinicalImpressionStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.EventStatus> src) throws FHIRException { 120 if (src == null || src.isEmpty()) 121 return null; 122 Enumeration<ClinicalImpression.ClinicalImpressionStatus> tgt = new Enumeration<>(new ClinicalImpression.ClinicalImpressionStatusEnumFactory()); 123 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 124 if (src.getValue() == null) { 125 tgt.setValue(null); 126 } else { 127 switch (src.getValue()) { 128 case INPROGRESS: 129 tgt.setValue(ClinicalImpression.ClinicalImpressionStatus.DRAFT); 130 break; 131 case COMPLETED: 132 tgt.setValue(ClinicalImpression.ClinicalImpressionStatus.COMPLETED); 133 break; 134 case ENTEREDINERROR: 135 tgt.setValue(ClinicalImpression.ClinicalImpressionStatus.ENTEREDINERROR); 136 break; 137 default: 138 tgt.setValue(ClinicalImpression.ClinicalImpressionStatus.NULL); 139 break; 140 } 141 } 142 return tgt; 143 } 144 145 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.EventStatus> convertClinicalImpressionStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ClinicalImpression.ClinicalImpressionStatus> src) throws FHIRException { 146 if (src == null || src.isEmpty()) 147 return null; 148 org.hl7.fhir.r5.model.Enumeration<Enumerations.EventStatus> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new Enumerations.EventStatusEnumFactory()); 149 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 150 if (src.getValue() == null) { 151 tgt.setValue(null); 152 } else { 153 switch (src.getValue()) { 154 case DRAFT: 155 tgt.setValue(Enumerations.EventStatus.INPROGRESS); 156 break; 157 case COMPLETED: 158 tgt.setValue(Enumerations.EventStatus.COMPLETED); 159 break; 160 case ENTEREDINERROR: 161 tgt.setValue(Enumerations.EventStatus.ENTEREDINERROR); 162 break; 163 default: 164 tgt.setValue(Enumerations.EventStatus.NULL); 165 break; 166 } 167 } 168 return tgt; 169 } 170}