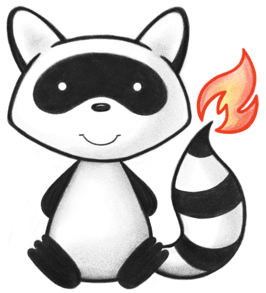
001package org.hl7.fhir.convertors.conv30_50.resources30_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_50; 004import org.hl7.fhir.convertors.conv30_50.datatypes30_50.Reference30_50; 005import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Annotation30_50; 006import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.CodeableConcept30_50; 007import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Identifier30_50; 008import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.DateTime30_50; 009import org.hl7.fhir.exceptions.FHIRException; 010import org.hl7.fhir.r5.model.CodeableReference; 011 012public class Communication30_50 { 013 014 public static org.hl7.fhir.dstu3.model.Communication convertCommunication(org.hl7.fhir.r5.model.Communication src) throws FHIRException { 015 if (src == null) 016 return null; 017 org.hl7.fhir.dstu3.model.Communication tgt = new org.hl7.fhir.dstu3.model.Communication(); 018 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 019 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 020 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 021 for (org.hl7.fhir.r5.model.UriType t : src.getInstantiatesCanonical()) 022 tgt.addDefinition(new org.hl7.fhir.dstu3.model.Reference(t.getValue())); 023 for (org.hl7.fhir.r5.model.Reference t : src.getBasedOn()) tgt.addBasedOn(Reference30_50.convertReference(t)); 024 for (org.hl7.fhir.r5.model.Reference t : src.getPartOf()) tgt.addPartOf(Reference30_50.convertReference(t)); 025 if (src.hasStatus()) 026 if (src.getStatus() == org.hl7.fhir.r5.model.Enumerations.EventStatus.NOTDONE) 027 tgt.setNotDone(true); 028 else 029 tgt.setStatusElement(convertCommunicationStatus(src.getStatusElement())); 030 if (src.hasStatusReason()) 031 tgt.setNotDoneReason(CodeableConcept30_50.convertCodeableConcept(src.getStatusReason())); 032 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getCategory()) 033 tgt.addCategory(CodeableConcept30_50.convertCodeableConcept(t)); 034 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getMedium()) 035 tgt.addMedium(CodeableConcept30_50.convertCodeableConcept(t)); 036 if (src.hasSubject()) 037 tgt.setSubject(Reference30_50.convertReference(src.getSubject())); 038 for (org.hl7.fhir.r5.model.Reference t : src.getRecipient()) tgt.addRecipient(Reference30_50.convertReference(t)); 039 if (src.hasEncounter()) 040 tgt.setContext(Reference30_50.convertReference(src.getEncounter())); 041 if (src.hasSent()) 042 tgt.setSentElement(DateTime30_50.convertDateTime(src.getSentElement())); 043 if (src.hasReceived()) 044 tgt.setReceivedElement(DateTime30_50.convertDateTime(src.getReceivedElement())); 045 if (src.hasSender()) 046 tgt.setSender(Reference30_50.convertReference(src.getSender())); 047 for (CodeableReference t : src.getReason()) 048 if (t.hasConcept()) 049 tgt.addReasonCode(CodeableConcept30_50.convertCodeableConcept(t.getConcept())); 050 for (CodeableReference t : src.getReason()) 051 if (t.hasReference()) 052 tgt.addReasonReference(Reference30_50.convertReference(t.getReference())); 053 for (org.hl7.fhir.r5.model.Communication.CommunicationPayloadComponent t : src.getPayload()) 054 tgt.addPayload(convertCommunicationPayloadComponent(t)); 055 for (org.hl7.fhir.r5.model.Annotation t : src.getNote()) tgt.addNote(Annotation30_50.convertAnnotation(t)); 056 return tgt; 057 } 058 059 public static org.hl7.fhir.r5.model.Communication convertCommunication(org.hl7.fhir.dstu3.model.Communication src) throws FHIRException { 060 if (src == null) 061 return null; 062 org.hl7.fhir.r5.model.Communication tgt = new org.hl7.fhir.r5.model.Communication(); 063 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 064 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 065 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 066 for (org.hl7.fhir.dstu3.model.Reference t : src.getDefinition()) tgt.addInstantiatesCanonical(t.getReference()); 067 for (org.hl7.fhir.dstu3.model.Reference t : src.getBasedOn()) tgt.addBasedOn(Reference30_50.convertReference(t)); 068 for (org.hl7.fhir.dstu3.model.Reference t : src.getPartOf()) tgt.addPartOf(Reference30_50.convertReference(t)); 069 if (src.hasNotDone()) 070 tgt.setStatus(org.hl7.fhir.r5.model.Enumerations.EventStatus.NOTDONE); 071 else if (src.hasStatus()) 072 tgt.setStatusElement(convertCommunicationStatus(src.getStatusElement())); 073 if (src.hasNotDoneReason()) 074 tgt.setStatusReason(CodeableConcept30_50.convertCodeableConcept(src.getNotDoneReason())); 075 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getCategory()) 076 tgt.addCategory(CodeableConcept30_50.convertCodeableConcept(t)); 077 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getMedium()) 078 tgt.addMedium(CodeableConcept30_50.convertCodeableConcept(t)); 079 if (src.hasSubject()) 080 tgt.setSubject(Reference30_50.convertReference(src.getSubject())); 081 for (org.hl7.fhir.dstu3.model.Reference t : src.getRecipient()) 082 tgt.addRecipient(Reference30_50.convertReference(t)); 083 if (src.hasContext()) 084 tgt.setEncounter(Reference30_50.convertReference(src.getContext())); 085 if (src.hasSent()) 086 tgt.setSentElement(DateTime30_50.convertDateTime(src.getSentElement())); 087 if (src.hasReceived()) 088 tgt.setReceivedElement(DateTime30_50.convertDateTime(src.getReceivedElement())); 089 if (src.hasSender()) 090 tgt.setSender(Reference30_50.convertReference(src.getSender())); 091 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getReasonCode()) 092 tgt.addReason(Reference30_50.convertCodeableConceptToCodableReference(t)); 093 for (org.hl7.fhir.dstu3.model.Reference t : src.getReasonReference()) 094 tgt.addReason(Reference30_50.convertReferenceToCodableReference(t)); 095 for (org.hl7.fhir.dstu3.model.Communication.CommunicationPayloadComponent t : src.getPayload()) 096 tgt.addPayload(convertCommunicationPayloadComponent(t)); 097 for (org.hl7.fhir.dstu3.model.Annotation t : src.getNote()) tgt.addNote(Annotation30_50.convertAnnotation(t)); 098 return tgt; 099 } 100 101 public static org.hl7.fhir.dstu3.model.Communication.CommunicationPayloadComponent convertCommunicationPayloadComponent(org.hl7.fhir.r5.model.Communication.CommunicationPayloadComponent src) throws FHIRException { 102 if (src == null) 103 return null; 104 org.hl7.fhir.dstu3.model.Communication.CommunicationPayloadComponent tgt = new org.hl7.fhir.dstu3.model.Communication.CommunicationPayloadComponent(); 105 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 106 if (src.hasContent()) 107 tgt.setContent(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getContent())); 108 return tgt; 109 } 110 111 public static org.hl7.fhir.r5.model.Communication.CommunicationPayloadComponent convertCommunicationPayloadComponent(org.hl7.fhir.dstu3.model.Communication.CommunicationPayloadComponent src) throws FHIRException { 112 if (src == null) 113 return null; 114 org.hl7.fhir.r5.model.Communication.CommunicationPayloadComponent tgt = new org.hl7.fhir.r5.model.Communication.CommunicationPayloadComponent(); 115 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 116 if (src.hasContent()) 117 tgt.setContent(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getContent())); 118 return tgt; 119 } 120 121 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Communication.CommunicationStatus> convertCommunicationStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.EventStatus> src) throws FHIRException { 122 if (src == null || src.isEmpty()) 123 return null; 124 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Communication.CommunicationStatus> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Communication.CommunicationStatusEnumFactory()); 125 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 126 switch (src.getValue()) { 127 case PREPARATION: 128 tgt.setValue(org.hl7.fhir.dstu3.model.Communication.CommunicationStatus.PREPARATION); 129 break; 130 case INPROGRESS: 131 tgt.setValue(org.hl7.fhir.dstu3.model.Communication.CommunicationStatus.INPROGRESS); 132 break; 133 case ONHOLD: 134 tgt.setValue(org.hl7.fhir.dstu3.model.Communication.CommunicationStatus.SUSPENDED); 135 break; 136 case STOPPED: 137 tgt.setValue(org.hl7.fhir.dstu3.model.Communication.CommunicationStatus.ABORTED); 138 break; 139 case COMPLETED: 140 tgt.setValue(org.hl7.fhir.dstu3.model.Communication.CommunicationStatus.COMPLETED); 141 break; 142 case ENTEREDINERROR: 143 tgt.setValue(org.hl7.fhir.dstu3.model.Communication.CommunicationStatus.ENTEREDINERROR); 144 break; 145 case UNKNOWN: 146 tgt.setValue(org.hl7.fhir.dstu3.model.Communication.CommunicationStatus.UNKNOWN); 147 break; 148 default: 149 tgt.setValue(org.hl7.fhir.dstu3.model.Communication.CommunicationStatus.NULL); 150 break; 151 } 152 return tgt; 153 } 154 155 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.EventStatus> convertCommunicationStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Communication.CommunicationStatus> src) throws FHIRException { 156 if (src == null || src.isEmpty()) 157 return null; 158 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.EventStatus> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Enumerations.EventStatusEnumFactory()); 159 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 160 switch (src.getValue()) { 161 case PREPARATION: 162 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.EventStatus.PREPARATION); 163 break; 164 case INPROGRESS: 165 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.EventStatus.INPROGRESS); 166 break; 167 case SUSPENDED: 168 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.EventStatus.ONHOLD); 169 break; 170 case ABORTED: 171 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.EventStatus.STOPPED); 172 break; 173 case COMPLETED: 174 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.EventStatus.COMPLETED); 175 break; 176 case ENTEREDINERROR: 177 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.EventStatus.ENTEREDINERROR); 178 break; 179 case UNKNOWN: 180 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.EventStatus.UNKNOWN); 181 break; 182 default: 183 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.EventStatus.NULL); 184 break; 185 } 186 return tgt; 187 } 188}