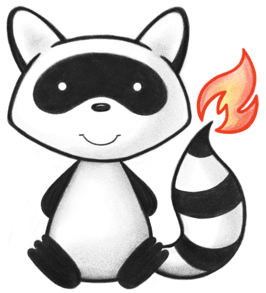
001package org.hl7.fhir.convertors.conv30_50.resources30_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_50; 004import org.hl7.fhir.convertors.conv30_50.datatypes30_50.ContactDetail30_50; 005import org.hl7.fhir.convertors.conv30_50.datatypes30_50.UsageContext30_50; 006import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Boolean30_50; 007import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Code30_50; 008import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.DateTime30_50; 009import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.MarkDown30_50; 010import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.String30_50; 011import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Uri30_50; 012import org.hl7.fhir.exceptions.FHIRException; 013 014public class CompartmentDefinition30_50 { 015 016 public static org.hl7.fhir.r5.model.CompartmentDefinition convertCompartmentDefinition(org.hl7.fhir.dstu3.model.CompartmentDefinition src) throws FHIRException { 017 if (src == null) 018 return null; 019 org.hl7.fhir.r5.model.CompartmentDefinition tgt = new org.hl7.fhir.r5.model.CompartmentDefinition(); 020 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 021 if (src.hasUrl()) 022 tgt.setUrlElement(Uri30_50.convertUri(src.getUrlElement())); 023 if (src.hasName()) 024 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 025 if (src.hasTitle()) 026 tgt.setTitleElement(String30_50.convertString(src.getTitleElement())); 027 if (src.hasStatus()) 028 tgt.setStatusElement(Enumerations30_50.convertPublicationStatus(src.getStatusElement())); 029 if (src.hasExperimental()) 030 tgt.setExperimentalElement(Boolean30_50.convertBoolean(src.getExperimentalElement())); 031 if (src.hasDate()) 032 tgt.setDateElement(DateTime30_50.convertDateTime(src.getDateElement())); 033 if (src.hasPublisher()) 034 tgt.setPublisherElement(String30_50.convertString(src.getPublisherElement())); 035 for (org.hl7.fhir.dstu3.model.ContactDetail t : src.getContact()) 036 tgt.addContact(ContactDetail30_50.convertContactDetail(t)); 037 if (src.hasDescription()) 038 tgt.setDescriptionElement(MarkDown30_50.convertMarkdown(src.getDescriptionElement())); 039 if (src.hasPurpose()) 040 tgt.setPurposeElement(MarkDown30_50.convertMarkdown(src.getPurposeElement())); 041 for (org.hl7.fhir.dstu3.model.UsageContext t : src.getUseContext()) 042 tgt.addUseContext(UsageContext30_50.convertUsageContext(t)); 043 if (src.hasCode()) 044 tgt.setCodeElement(convertCompartmentType(src.getCodeElement())); 045 if (src.hasSearch()) 046 tgt.setSearchElement(Boolean30_50.convertBoolean(src.getSearchElement())); 047 for (org.hl7.fhir.dstu3.model.CompartmentDefinition.CompartmentDefinitionResourceComponent t : src.getResource()) 048 tgt.addResource(convertCompartmentDefinitionResourceComponent(t)); 049 return tgt; 050 } 051 052 public static org.hl7.fhir.dstu3.model.CompartmentDefinition convertCompartmentDefinition(org.hl7.fhir.r5.model.CompartmentDefinition src) throws FHIRException { 053 if (src == null) 054 return null; 055 org.hl7.fhir.dstu3.model.CompartmentDefinition tgt = new org.hl7.fhir.dstu3.model.CompartmentDefinition(); 056 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 057 if (src.hasUrl()) 058 tgt.setUrlElement(Uri30_50.convertUri(src.getUrlElement())); 059 if (src.hasName()) 060 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 061 if (src.hasTitle()) 062 tgt.setTitleElement(String30_50.convertString(src.getTitleElement())); 063 if (src.hasStatus()) 064 tgt.setStatusElement(Enumerations30_50.convertPublicationStatus(src.getStatusElement())); 065 if (src.hasExperimental()) 066 tgt.setExperimentalElement(Boolean30_50.convertBoolean(src.getExperimentalElement())); 067 if (src.hasDate()) 068 tgt.setDateElement(DateTime30_50.convertDateTime(src.getDateElement())); 069 if (src.hasPublisher()) 070 tgt.setPublisherElement(String30_50.convertString(src.getPublisherElement())); 071 for (org.hl7.fhir.r5.model.ContactDetail t : src.getContact()) 072 tgt.addContact(ContactDetail30_50.convertContactDetail(t)); 073 if (src.hasDescription()) 074 tgt.setDescriptionElement(MarkDown30_50.convertMarkdown(src.getDescriptionElement())); 075 if (src.hasPurpose()) 076 tgt.setPurposeElement(MarkDown30_50.convertMarkdown(src.getPurposeElement())); 077 for (org.hl7.fhir.r5.model.UsageContext t : src.getUseContext()) 078 tgt.addUseContext(UsageContext30_50.convertUsageContext(t)); 079 if (src.hasCode()) 080 tgt.setCodeElement(convertCompartmentType(src.getCodeElement())); 081 if (src.hasSearch()) 082 tgt.setSearchElement(Boolean30_50.convertBoolean(src.getSearchElement())); 083 for (org.hl7.fhir.r5.model.CompartmentDefinition.CompartmentDefinitionResourceComponent t : src.getResource()) 084 tgt.addResource(convertCompartmentDefinitionResourceComponent(t)); 085 return tgt; 086 } 087 088 public static org.hl7.fhir.r5.model.CompartmentDefinition.CompartmentDefinitionResourceComponent convertCompartmentDefinitionResourceComponent(org.hl7.fhir.dstu3.model.CompartmentDefinition.CompartmentDefinitionResourceComponent src) throws FHIRException { 089 if (src == null) 090 return null; 091 org.hl7.fhir.r5.model.CompartmentDefinition.CompartmentDefinitionResourceComponent tgt = new org.hl7.fhir.r5.model.CompartmentDefinition.CompartmentDefinitionResourceComponent(); 092 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 093 if (src.hasCode()) 094 tgt.setCodeElement(Code30_50.convertCode(src.getCodeElement())); 095 for (org.hl7.fhir.dstu3.model.StringType t : src.getParam()) tgt.addParam(t.getValue()); 096 if (src.hasDocumentation()) 097 tgt.setDocumentationElement(String30_50.convertString(src.getDocumentationElement())); 098 return tgt; 099 } 100 101 public static org.hl7.fhir.dstu3.model.CompartmentDefinition.CompartmentDefinitionResourceComponent convertCompartmentDefinitionResourceComponent(org.hl7.fhir.r5.model.CompartmentDefinition.CompartmentDefinitionResourceComponent src) throws FHIRException { 102 if (src == null) 103 return null; 104 org.hl7.fhir.dstu3.model.CompartmentDefinition.CompartmentDefinitionResourceComponent tgt = new org.hl7.fhir.dstu3.model.CompartmentDefinition.CompartmentDefinitionResourceComponent(); 105 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 106 if (src.hasCode()) 107 tgt.setCodeElement(Code30_50.convertCode(src.getCodeElement())); 108 for (org.hl7.fhir.r5.model.StringType t : src.getParam()) tgt.addParam(t.getValue()); 109 if (src.hasDocumentation()) 110 tgt.setDocumentationElement(String30_50.convertString(src.getDocumentationElement())); 111 return tgt; 112 } 113 114 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CompartmentDefinition.CompartmentType> convertCompartmentType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.CompartmentType> src) throws FHIRException { 115 if (src == null || src.isEmpty()) 116 return null; 117 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CompartmentDefinition.CompartmentType> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.CompartmentDefinition.CompartmentTypeEnumFactory()); 118 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 119 switch (src.getValue()) { 120 case PATIENT: 121 tgt.setValue(org.hl7.fhir.dstu3.model.CompartmentDefinition.CompartmentType.PATIENT); 122 break; 123 case ENCOUNTER: 124 tgt.setValue(org.hl7.fhir.dstu3.model.CompartmentDefinition.CompartmentType.ENCOUNTER); 125 break; 126 case RELATEDPERSON: 127 tgt.setValue(org.hl7.fhir.dstu3.model.CompartmentDefinition.CompartmentType.RELATEDPERSON); 128 break; 129 case PRACTITIONER: 130 tgt.setValue(org.hl7.fhir.dstu3.model.CompartmentDefinition.CompartmentType.PRACTITIONER); 131 break; 132 case DEVICE: 133 tgt.setValue(org.hl7.fhir.dstu3.model.CompartmentDefinition.CompartmentType.DEVICE); 134 break; 135 default: 136 tgt.setValue(org.hl7.fhir.dstu3.model.CompartmentDefinition.CompartmentType.NULL); 137 break; 138 } 139 return tgt; 140 } 141 142 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.CompartmentType> convertCompartmentType(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.CompartmentDefinition.CompartmentType> src) throws FHIRException { 143 if (src == null || src.isEmpty()) 144 return null; 145 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.CompartmentType> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Enumerations.CompartmentTypeEnumFactory()); 146 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 147 switch (src.getValue()) { 148 case PATIENT: 149 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.CompartmentType.PATIENT); 150 break; 151 case ENCOUNTER: 152 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.CompartmentType.ENCOUNTER); 153 break; 154 case RELATEDPERSON: 155 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.CompartmentType.RELATEDPERSON); 156 break; 157 case PRACTITIONER: 158 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.CompartmentType.PRACTITIONER); 159 break; 160 case DEVICE: 161 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.CompartmentType.DEVICE); 162 break; 163 default: 164 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.CompartmentType.NULL); 165 break; 166 } 167 return tgt; 168 } 169}