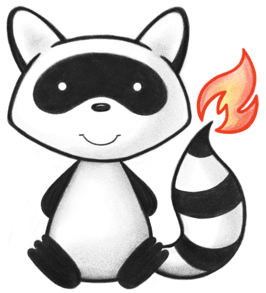
001package org.hl7.fhir.convertors.conv30_50.resources30_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_50; 004import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Uri30_50; 005import org.hl7.fhir.exceptions.FHIRException; 006 007public class Consent30_50 { 008 009 public static org.hl7.fhir.dstu3.model.Consent convertConsent(org.hl7.fhir.r5.model.Consent src) throws FHIRException { 010 if (src == null) 011 return null; 012 org.hl7.fhir.dstu3.model.Consent tgt = new org.hl7.fhir.dstu3.model.Consent(); 013 return tgt; 014 } 015 016 public static org.hl7.fhir.r5.model.Consent convertConsent(org.hl7.fhir.dstu3.model.Consent src) throws FHIRException { 017 if (src == null) 018 return null; 019 org.hl7.fhir.r5.model.Consent tgt = new org.hl7.fhir.r5.model.Consent(); 020 return tgt; 021 } 022 023 public static org.hl7.fhir.r5.model.Consent.ConsentPolicyBasisComponent convertConsentPolicyComponent(org.hl7.fhir.dstu3.model.Consent.ConsentPolicyComponent src) throws FHIRException { 024 if (src == null) return null; 025 org.hl7.fhir.r5.model.Consent.ConsentPolicyBasisComponent tgt = new org.hl7.fhir.r5.model.Consent.ConsentPolicyBasisComponent(); 026 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 027// if (src.hasAuthority()) tgt.setRefereceElement(Uri30_50.convertUri(src.getAuthorityElement())); 028 if (src.hasUri()) tgt.setUrl(src.getUri()); 029 return tgt; 030 } 031 032 public static org.hl7.fhir.dstu3.model.Consent.ConsentPolicyComponent convertConsentPolicyComponent(org.hl7.fhir.r5.model.Consent.ConsentPolicyBasisComponent src) throws FHIRException { 033 if (src == null) return null; 034 org.hl7.fhir.dstu3.model.Consent.ConsentPolicyComponent tgt = new org.hl7.fhir.dstu3.model.Consent.ConsentPolicyComponent(); 035 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 036// if (src.hasAuthority()) tgt.setAuthorityElement(Uri30_50.convertUri(src.getReferenceElement())); 037 if (src.hasUrl()) tgt.setUriElement(Uri30_50.convertUri(src.getUrlElement())); 038 return tgt; 039 } 040 041// static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Consent.ConsentDataMeaning> convertConsentDataMeaning(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Consent.ConsentDataMeaning> src) throws FHIRException { 042// if (src == null || src.isEmpty()) return null; 043// org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Consent.ConsentDataMeaning> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Consent.ConsentDataMeaningEnumFactory()); 044// ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 045// if (src.getValue() == null) { 046// tgt.setValue(org.hl7.fhir.r5.model.Consent.ConsentDataMeaning.NULL); 047// } else { 048// switch (src.getValue()) { 049// case INSTANCE: 050// tgt.setValue(org.hl7.fhir.r5.model.Consent.ConsentDataMeaning.INSTANCE); 051// break; 052// case RELATED: 053// tgt.setValue(org.hl7.fhir.r5.model.Consent.ConsentDataMeaning.RELATED); 054// break; 055// case DEPENDENTS: 056// tgt.setValue(org.hl7.fhir.r5.model.Consent.ConsentDataMeaning.DEPENDENTS); 057// break; 058// case AUTHOREDBY: 059// tgt.setValue(org.hl7.fhir.r5.model.Consent.ConsentDataMeaning.AUTHOREDBY); 060// break; 061// default: 062// tgt.setValue(org.hl7.fhir.r5.model.Consent.ConsentDataMeaning.NULL); 063// break; 064// } 065// } 066// return tgt; 067// } 068// 069// static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Consent.ConsentDataMeaning> convertConsentDataMeaning(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Consent.ConsentDataMeaning> src) throws FHIRException { 070// if (src == null || src.isEmpty()) return null; 071// org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Consent.ConsentDataMeaning> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Consent.ConsentDataMeaningEnumFactory()); 072// ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 073// if (src.getValue() == null) { 074// tgt.setValue(org.hl7.fhir.dstu3.model.Consent.ConsentDataMeaning.NULL); 075// } else { 076// switch (src.getValue()) { 077// case INSTANCE: 078// tgt.setValue(org.hl7.fhir.dstu3.model.Consent.ConsentDataMeaning.INSTANCE); 079// break; 080// case RELATED: 081// tgt.setValue(org.hl7.fhir.dstu3.model.Consent.ConsentDataMeaning.RELATED); 082// break; 083// case DEPENDENTS: 084// tgt.setValue(org.hl7.fhir.dstu3.model.Consent.ConsentDataMeaning.DEPENDENTS); 085// break; 086// case AUTHOREDBY: 087// tgt.setValue(org.hl7.fhir.dstu3.model.Consent.ConsentDataMeaning.AUTHOREDBY); 088// break; 089// default: 090// tgt.setValue(org.hl7.fhir.dstu3.model.Consent.ConsentDataMeaning.NULL); 091// break; 092// } 093// } 094// return tgt; 095// } 096}