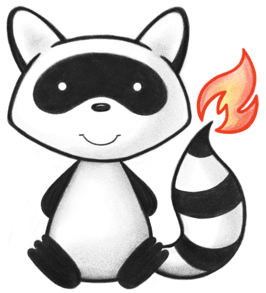
001package org.hl7.fhir.convertors.conv30_50.resources30_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_50; 004import org.hl7.fhir.convertors.conv30_50.datatypes30_50.Reference30_50; 005import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Attachment30_50; 006import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.CodeableConcept30_50; 007import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Identifier30_50; 008import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Instant30_50; 009import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.String30_50; 010import org.hl7.fhir.exceptions.FHIRException; 011import org.hl7.fhir.r5.model.DiagnosticReport; 012import org.hl7.fhir.r5.model.Enumeration; 013 014public class DiagnosticReport30_50 { 015 016 public static org.hl7.fhir.r5.model.DiagnosticReport convertDiagnosticReport(org.hl7.fhir.dstu3.model.DiagnosticReport src) throws FHIRException { 017 if (src == null) 018 return null; 019 org.hl7.fhir.r5.model.DiagnosticReport tgt = new org.hl7.fhir.r5.model.DiagnosticReport(); 020 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 021 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 022 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 023 for (org.hl7.fhir.dstu3.model.Reference t : src.getBasedOn()) tgt.addBasedOn(Reference30_50.convertReference(t)); 024 if (src.hasStatus()) 025 tgt.setStatusElement(convertDiagnosticReportStatus(src.getStatusElement())); 026 if (src.hasCategory()) 027 tgt.addCategory(CodeableConcept30_50.convertCodeableConcept(src.getCategory())); 028 if (src.hasCode()) 029 tgt.setCode(CodeableConcept30_50.convertCodeableConcept(src.getCode())); 030 if (src.hasSubject()) 031 tgt.setSubject(Reference30_50.convertReference(src.getSubject())); 032 if (src.hasContext()) 033 tgt.setEncounter(Reference30_50.convertReference(src.getContext())); 034 if (src.hasEffective()) 035 tgt.setEffective(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getEffective())); 036 if (src.hasIssued()) 037 tgt.setIssuedElement(Instant30_50.convertInstant(src.getIssuedElement())); 038 for (org.hl7.fhir.dstu3.model.Reference t : src.getSpecimen()) tgt.addSpecimen(Reference30_50.convertReference(t)); 039 for (org.hl7.fhir.dstu3.model.Reference t : src.getResult()) tgt.addResult(Reference30_50.convertReference(t)); 040 for (org.hl7.fhir.dstu3.model.DiagnosticReport.DiagnosticReportImageComponent t : src.getImage()) 041 tgt.addMedia(convertDiagnosticReportImageComponent(t)); 042 if (src.hasConclusion()) 043 tgt.setConclusionElement(String30_50.convertStringToMarkdown(src.getConclusionElement())); 044 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getCodedDiagnosis()) 045 tgt.addConclusionCode(CodeableConcept30_50.convertCodeableConcept(t)); 046 for (org.hl7.fhir.dstu3.model.Attachment t : src.getPresentedForm()) 047 tgt.addPresentedForm(Attachment30_50.convertAttachment(t)); 048 return tgt; 049 } 050 051 public static org.hl7.fhir.dstu3.model.DiagnosticReport convertDiagnosticReport(org.hl7.fhir.r5.model.DiagnosticReport src) throws FHIRException { 052 if (src == null) 053 return null; 054 org.hl7.fhir.dstu3.model.DiagnosticReport tgt = new org.hl7.fhir.dstu3.model.DiagnosticReport(); 055 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 056 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 057 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 058 for (org.hl7.fhir.r5.model.Reference t : src.getBasedOn()) tgt.addBasedOn(Reference30_50.convertReference(t)); 059 if (src.hasStatus()) 060 tgt.setStatusElement(convertDiagnosticReportStatus(src.getStatusElement())); 061 if (src.hasCategory()) 062 tgt.setCategory(CodeableConcept30_50.convertCodeableConcept(src.getCategoryFirstRep())); 063 if (src.hasCode()) 064 tgt.setCode(CodeableConcept30_50.convertCodeableConcept(src.getCode())); 065 if (src.hasSubject()) 066 tgt.setSubject(Reference30_50.convertReference(src.getSubject())); 067 if (src.hasEncounter()) 068 tgt.setContext(Reference30_50.convertReference(src.getEncounter())); 069 if (src.hasEffective()) 070 tgt.setEffective(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getEffective())); 071 if (src.hasIssued()) 072 tgt.setIssuedElement(Instant30_50.convertInstant(src.getIssuedElement())); 073 for (org.hl7.fhir.r5.model.Reference t : src.getSpecimen()) tgt.addSpecimen(Reference30_50.convertReference(t)); 074 for (org.hl7.fhir.r5.model.Reference t : src.getResult()) tgt.addResult(Reference30_50.convertReference(t)); 075 for (org.hl7.fhir.r5.model.DiagnosticReport.DiagnosticReportMediaComponent t : src.getMedia()) 076 tgt.addImage(convertDiagnosticReportImageComponent(t)); 077 if (src.hasConclusion()) 078 tgt.setConclusionElement(String30_50.convertString(src.getConclusionElement())); 079 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getConclusionCode()) 080 tgt.addCodedDiagnosis(CodeableConcept30_50.convertCodeableConcept(t)); 081 for (org.hl7.fhir.r5.model.Attachment t : src.getPresentedForm()) 082 tgt.addPresentedForm(Attachment30_50.convertAttachment(t)); 083 return tgt; 084 } 085 086 public static org.hl7.fhir.dstu3.model.DiagnosticReport.DiagnosticReportImageComponent convertDiagnosticReportImageComponent(org.hl7.fhir.r5.model.DiagnosticReport.DiagnosticReportMediaComponent src) throws FHIRException { 087 if (src == null) 088 return null; 089 org.hl7.fhir.dstu3.model.DiagnosticReport.DiagnosticReportImageComponent tgt = new org.hl7.fhir.dstu3.model.DiagnosticReport.DiagnosticReportImageComponent(); 090 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 091 if (src.hasComment()) 092 tgt.setCommentElement(String30_50.convertString(src.getCommentElement())); 093 if (src.hasLink()) 094 tgt.setLink(Reference30_50.convertReference(src.getLink())); 095 return tgt; 096 } 097 098 public static org.hl7.fhir.r5.model.DiagnosticReport.DiagnosticReportMediaComponent convertDiagnosticReportImageComponent(org.hl7.fhir.dstu3.model.DiagnosticReport.DiagnosticReportImageComponent src) throws FHIRException { 099 if (src == null) 100 return null; 101 org.hl7.fhir.r5.model.DiagnosticReport.DiagnosticReportMediaComponent tgt = new org.hl7.fhir.r5.model.DiagnosticReport.DiagnosticReportMediaComponent(); 102 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 103 if (src.hasComment()) 104 tgt.setCommentElement(String30_50.convertString(src.getCommentElement())); 105 if (src.hasLink()) 106 tgt.setLink(Reference30_50.convertReference(src.getLink())); 107 return tgt; 108 } 109 110 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.DiagnosticReport.DiagnosticReportStatus> convertDiagnosticReportStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.DiagnosticReport.DiagnosticReportStatus> src) throws FHIRException { 111 if (src == null || src.isEmpty()) 112 return null; 113 Enumeration<DiagnosticReport.DiagnosticReportStatus> tgt = new Enumeration<>(new DiagnosticReport.DiagnosticReportStatusEnumFactory()); 114 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 115 if (src.getValue() == null) { 116 tgt.setValue(null); 117 } else { 118 switch (src.getValue()) { 119 case REGISTERED: 120 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.REGISTERED); 121 break; 122 case PARTIAL: 123 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.PARTIAL); 124 break; 125 case PRELIMINARY: 126 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.PRELIMINARY); 127 break; 128 case FINAL: 129 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.FINAL); 130 break; 131 case AMENDED: 132 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.AMENDED); 133 break; 134 case CORRECTED: 135 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.CORRECTED); 136 break; 137 case APPENDED: 138 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.APPENDED); 139 break; 140 case CANCELLED: 141 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.CANCELLED); 142 break; 143 case ENTEREDINERROR: 144 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.ENTEREDINERROR); 145 break; 146 case UNKNOWN: 147 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.UNKNOWN); 148 break; 149 default: 150 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.NULL); 151 break; 152 } 153 } 154 return tgt; 155 } 156 157 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.DiagnosticReport.DiagnosticReportStatus> convertDiagnosticReportStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.DiagnosticReport.DiagnosticReportStatus> src) throws FHIRException { 158 if (src == null || src.isEmpty()) 159 return null; 160 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.DiagnosticReport.DiagnosticReportStatus> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.DiagnosticReport.DiagnosticReportStatusEnumFactory()); 161 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 162 if (src.getValue() == null) { 163 tgt.setValue(null); 164 } else { 165 switch (src.getValue()) { 166 case REGISTERED: 167 tgt.setValue(org.hl7.fhir.dstu3.model.DiagnosticReport.DiagnosticReportStatus.REGISTERED); 168 break; 169 case PARTIAL: 170 tgt.setValue(org.hl7.fhir.dstu3.model.DiagnosticReport.DiagnosticReportStatus.PARTIAL); 171 break; 172 case PRELIMINARY: 173 tgt.setValue(org.hl7.fhir.dstu3.model.DiagnosticReport.DiagnosticReportStatus.PRELIMINARY); 174 break; 175 case FINAL: 176 tgt.setValue(org.hl7.fhir.dstu3.model.DiagnosticReport.DiagnosticReportStatus.FINAL); 177 break; 178 case AMENDED: 179 tgt.setValue(org.hl7.fhir.dstu3.model.DiagnosticReport.DiagnosticReportStatus.AMENDED); 180 break; 181 case CORRECTED: 182 tgt.setValue(org.hl7.fhir.dstu3.model.DiagnosticReport.DiagnosticReportStatus.CORRECTED); 183 break; 184 case APPENDED: 185 tgt.setValue(org.hl7.fhir.dstu3.model.DiagnosticReport.DiagnosticReportStatus.APPENDED); 186 break; 187 case CANCELLED: 188 tgt.setValue(org.hl7.fhir.dstu3.model.DiagnosticReport.DiagnosticReportStatus.CANCELLED); 189 break; 190 case ENTEREDINERROR: 191 tgt.setValue(org.hl7.fhir.dstu3.model.DiagnosticReport.DiagnosticReportStatus.ENTEREDINERROR); 192 break; 193 case UNKNOWN: 194 tgt.setValue(org.hl7.fhir.dstu3.model.DiagnosticReport.DiagnosticReportStatus.UNKNOWN); 195 break; 196 default: 197 tgt.setValue(org.hl7.fhir.dstu3.model.DiagnosticReport.DiagnosticReportStatus.NULL); 198 break; 199 } 200 } 201 return tgt; 202 } 203}