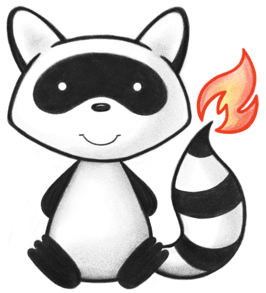
001package org.hl7.fhir.convertors.conv30_50.resources30_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_50; 004import org.hl7.fhir.convertors.conv30_50.datatypes30_50.Reference30_50; 005import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Attachment30_50; 006import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.CodeableConcept30_50; 007import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Identifier30_50; 008import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Period30_50; 009import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.String30_50; 010import org.hl7.fhir.dstu3.model.Enumeration; 011import org.hl7.fhir.dstu3.model.Enumerations; 012import org.hl7.fhir.exceptions.FHIRException; 013import org.hl7.fhir.r5.model.CodeableReference; 014import org.hl7.fhir.r5.model.DocumentReference; 015import org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceAttesterComponent; 016 017public class DocumentReference30_50 { 018 019 public static org.hl7.fhir.r5.model.DocumentReference convertDocumentReference(org.hl7.fhir.dstu3.model.DocumentReference src) throws FHIRException { 020 if (src == null) 021 return null; 022 org.hl7.fhir.r5.model.DocumentReference tgt = new org.hl7.fhir.r5.model.DocumentReference(); 023 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 024 if (src.hasMasterIdentifier()) 025 tgt.addIdentifier(Identifier30_50.convertIdentifier(src.getMasterIdentifier())); 026 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 027 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 028 if (src.hasStatus()) 029 tgt.setStatusElement(convertDocumentReferenceStatus(src.getStatusElement())); 030 if (src.hasDocStatus()) 031 tgt.setDocStatusElement(convertReferredDocumentStatus(src.getDocStatusElement())); 032 if (src.hasType()) 033 tgt.setType(CodeableConcept30_50.convertCodeableConcept(src.getType())); 034 if (src.hasClass_()) 035 tgt.addCategory(CodeableConcept30_50.convertCodeableConcept(src.getClass_())); 036 if (src.hasSubject()) 037 tgt.setSubject(Reference30_50.convertReference(src.getSubject())); 038 if (src.hasCreated()) 039 tgt.setDate(src.getCreated()); 040 if (src.hasAuthenticator()) 041 tgt.addAttester().setMode(new org.hl7.fhir.r5.model.CodeableConcept().addCoding(new org.hl7.fhir.r5.model.Coding("http://hl7.org/fhir/composition-attestation-mode","official", "Official"))) 042 .setParty(Reference30_50.convertReference(src.getAuthenticator())); 043 if (src.hasCustodian()) 044 tgt.setCustodian(Reference30_50.convertReference(src.getCustodian())); 045 for (org.hl7.fhir.dstu3.model.DocumentReference.DocumentReferenceRelatesToComponent t : src.getRelatesTo()) 046 tgt.addRelatesTo(convertDocumentReferenceRelatesToComponent(t)); 047 if (src.hasDescription()) 048 tgt.setDescriptionElement(String30_50.convertStringToMarkdown(src.getDescriptionElement())); 049 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getSecurityLabel()) 050 tgt.addSecurityLabel(CodeableConcept30_50.convertCodeableConcept(t)); 051 for (org.hl7.fhir.dstu3.model.DocumentReference.DocumentReferenceContentComponent t : src.getContent()) 052 tgt.addContent(convertDocumentReferenceContentComponent(t)); 053 if (src.hasContext()) 054 convertDocumentReferenceContextComponent(src.getContext(), tgt); 055 for (org.hl7.fhir.dstu3.model.Reference t : src.getAuthor()) 056 tgt.addAuthor(Reference30_50.convertReference(t)); 057 return tgt; 058 } 059 060 public static org.hl7.fhir.dstu3.model.DocumentReference convertDocumentReference(org.hl7.fhir.r5.model.DocumentReference src) throws FHIRException { 061 if (src == null) 062 return null; 063 org.hl7.fhir.dstu3.model.DocumentReference tgt = new org.hl7.fhir.dstu3.model.DocumentReference(); 064 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 065 // if (src.hasMasterIdentifier()) 066 // tgt.setMasterIdentifier(VersionConvertor_30_50.convertIdentifier(src.getMasterIdentifier())); 067 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 068 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 069 if (src.hasStatus()) 070 tgt.setStatusElement(convertDocumentReferenceStatus(src.getStatusElement())); 071 if (src.hasDocStatus()) 072 tgt.setDocStatusElement(convertReferredDocumentStatus(src.getDocStatusElement())); 073 if (src.hasType()) 074 tgt.setType(CodeableConcept30_50.convertCodeableConcept(src.getType())); 075 if (src.hasCategory()) 076 tgt.setClass_(CodeableConcept30_50.convertCodeableConcept(src.getCategoryFirstRep())); 077 if (src.hasSubject()) 078 tgt.setSubject(Reference30_50.convertReference(src.getSubject())); 079 if (src.hasDate()) 080 tgt.setCreated(src.getDate()); 081 for (DocumentReferenceAttesterComponent t : src.getAttester()) { 082 if (t.getMode().hasCoding("http://hl7.org/fhir/composition-attestation-mode", "official")) 083 tgt.setAuthenticator(Reference30_50.convertReference(t.getParty())); 084 } 085 if (src.hasCustodian()) 086 tgt.setCustodian(Reference30_50.convertReference(src.getCustodian())); 087 for (org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceRelatesToComponent t : src.getRelatesTo()) 088 tgt.addRelatesTo(convertDocumentReferenceRelatesToComponent(t)); 089 if (src.hasDescription()) 090 tgt.setDescriptionElement(String30_50.convertString(src.getDescriptionElement())); 091 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getSecurityLabel()) 092 tgt.addSecurityLabel(CodeableConcept30_50.convertCodeableConcept(t)); 093 for (org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceContentComponent t : src.getContent()) 094 tgt.addContent(convertDocumentReferenceContentComponent(t)); 095 convertDocumentReferenceContextComponent(src, tgt.getContext()); 096 for (org.hl7.fhir.r5.model.Reference t : src.getAuthor()) 097 tgt.addAuthor(Reference30_50.convertReference(t)); 098 return tgt; 099 } 100 101 public static org.hl7.fhir.dstu3.model.DocumentReference.DocumentReferenceContentComponent convertDocumentReferenceContentComponent(org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceContentComponent src) throws FHIRException { 102 if (src == null) 103 return null; 104 org.hl7.fhir.dstu3.model.DocumentReference.DocumentReferenceContentComponent tgt = new org.hl7.fhir.dstu3.model.DocumentReference.DocumentReferenceContentComponent(); 105 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 106 if (src.hasAttachment()) 107 tgt.setAttachment(Attachment30_50.convertAttachment(src.getAttachment())); 108 return tgt; 109 } 110 111 public static org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceContentComponent convertDocumentReferenceContentComponent(org.hl7.fhir.dstu3.model.DocumentReference.DocumentReferenceContentComponent src) throws FHIRException { 112 if (src == null) 113 return null; 114 org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceContentComponent tgt = new org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceContentComponent(); 115 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 116 if (src.hasAttachment()) 117 tgt.setAttachment(Attachment30_50.convertAttachment(src.getAttachment())); 118 return tgt; 119 } 120 121 public static void convertDocumentReferenceContextComponent(org.hl7.fhir.r5.model.DocumentReference src, org.hl7.fhir.dstu3.model.DocumentReference.DocumentReferenceContextComponent tgt) throws FHIRException { 122 if (src.hasContext()) 123 tgt.setEncounter(Reference30_50.convertReference(src.getContextFirstRep())); 124 for (CodeableReference t : src.getEvent()) 125 if (t.hasConcept()) 126 tgt.addEvent(CodeableConcept30_50.convertCodeableConcept(t.getConcept())); 127 if (src.hasPeriod()) 128 tgt.setPeriod(Period30_50.convertPeriod(src.getPeriod())); 129 if (src.hasFacilityType()) 130 tgt.setFacilityType(CodeableConcept30_50.convertCodeableConcept(src.getFacilityType())); 131 if (src.hasPracticeSetting()) 132 tgt.setPracticeSetting(CodeableConcept30_50.convertCodeableConcept(src.getPracticeSetting())); 133// if (src.hasSourcePatientInfo()) 134// tgt.setSourcePatientInfo(Reference30_50.convertReference(src.getSourcePatientInfo())); 135// for (org.hl7.fhir.r5.model.Reference t : src.getRelated()) 136// tgt.addRelated(convertDocumentReferenceContextRelatedComponent(t)); 137 } 138 139 public static void convertDocumentReferenceContextComponent(org.hl7.fhir.dstu3.model.DocumentReference.DocumentReferenceContextComponent src, org.hl7.fhir.r5.model.DocumentReference tgt) throws FHIRException { 140 if (src.hasEncounter()) 141 tgt.addContext(Reference30_50.convertReference(src.getEncounter())); 142 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getEvent()) 143 tgt.addEvent(new CodeableReference().setConcept(CodeableConcept30_50.convertCodeableConcept(t))); 144 if (src.hasPeriod()) 145 tgt.setPeriod(Period30_50.convertPeriod(src.getPeriod())); 146 if (src.hasFacilityType()) 147 tgt.setFacilityType(CodeableConcept30_50.convertCodeableConcept(src.getFacilityType())); 148 if (src.hasPracticeSetting()) 149 tgt.setPracticeSetting(CodeableConcept30_50.convertCodeableConcept(src.getPracticeSetting())); 150// if (src.hasSourcePatientInfo()) 151// tgt.setSourcePatientInfo(Reference30_50.convertReference(src.getSourcePatientInfo())); 152// for (org.hl7.fhir.dstu3.model.DocumentReference.DocumentReferenceContextRelatedComponent t : src.getRelated()) 153// tgt.addRelated(convertDocumentReferenceContextRelatedComponent(t)); 154 } 155 156 public static org.hl7.fhir.r5.model.Reference convertDocumentReferenceContextRelatedComponent(org.hl7.fhir.dstu3.model.DocumentReference.DocumentReferenceContextRelatedComponent src) throws FHIRException { 157 if (src == null) 158 return null; 159 org.hl7.fhir.r5.model.Reference tgt = Reference30_50.convertReference(src.getRef()); 160 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 161 if (src.hasIdentifier()) 162 tgt.setIdentifier(Identifier30_50.convertIdentifier(src.getIdentifier())); 163 return tgt; 164 } 165 166 public static org.hl7.fhir.dstu3.model.DocumentReference.DocumentReferenceContextRelatedComponent convertDocumentReferenceContextRelatedComponent(org.hl7.fhir.r5.model.Reference src) throws FHIRException { 167 if (src == null) 168 return null; 169 org.hl7.fhir.dstu3.model.DocumentReference.DocumentReferenceContextRelatedComponent tgt = new org.hl7.fhir.dstu3.model.DocumentReference.DocumentReferenceContextRelatedComponent(); 170 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 171 if (src.hasIdentifier()) 172 tgt.setIdentifier(Identifier30_50.convertIdentifier(src.getIdentifier())); 173 tgt.setRef(Reference30_50.convertReference(src)); 174 return tgt; 175 } 176 177 public static org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceRelatesToComponent convertDocumentReferenceRelatesToComponent(org.hl7.fhir.dstu3.model.DocumentReference.DocumentReferenceRelatesToComponent src) throws FHIRException { 178 if (src == null) 179 return null; 180 org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceRelatesToComponent tgt = new org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceRelatesToComponent(); 181 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 182 if (src.hasCode()) 183 tgt.setCode(convertDocumentRelationshipType(src.getCodeElement())); 184 if (src.hasTarget()) 185 tgt.setTarget(Reference30_50.convertReference(src.getTarget())); 186 return tgt; 187 } 188 189 public static org.hl7.fhir.dstu3.model.DocumentReference.DocumentReferenceRelatesToComponent convertDocumentReferenceRelatesToComponent(org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceRelatesToComponent src) throws FHIRException { 190 if (src == null) 191 return null; 192 org.hl7.fhir.dstu3.model.DocumentReference.DocumentReferenceRelatesToComponent tgt = new org.hl7.fhir.dstu3.model.DocumentReference.DocumentReferenceRelatesToComponent(); 193 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 194 if (src.hasCode()) 195 tgt.setCodeElement(convertDocumentRelationshipType2(src.getCode())); 196 if (src.hasTarget()) 197 tgt.setTarget(Reference30_50.convertReference(src.getTarget())); 198 return tgt; 199 } 200 201 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Enumerations.DocumentReferenceStatus> convertDocumentReferenceStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceStatus> src) throws FHIRException { 202 if (src == null || src.isEmpty()) 203 return null; 204 Enumeration<Enumerations.DocumentReferenceStatus> tgt = new Enumeration<>(new Enumerations.DocumentReferenceStatusEnumFactory()); 205 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 206 if (src.getValue() == null) { 207 tgt.setValue(null); 208 } else { 209 switch (src.getValue()) { 210 case CURRENT: 211 tgt.setValue(Enumerations.DocumentReferenceStatus.CURRENT); 212 break; 213 case SUPERSEDED: 214 tgt.setValue(Enumerations.DocumentReferenceStatus.SUPERSEDED); 215 break; 216 case ENTEREDINERROR: 217 tgt.setValue(Enumerations.DocumentReferenceStatus.ENTEREDINERROR); 218 break; 219 default: 220 tgt.setValue(Enumerations.DocumentReferenceStatus.NULL); 221 break; 222 } 223 } 224 return tgt; 225 } 226 227 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceStatus> convertDocumentReferenceStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Enumerations.DocumentReferenceStatus> src) throws FHIRException { 228 if (src == null || src.isEmpty()) 229 return null; 230 org.hl7.fhir.r5.model.Enumeration<DocumentReference.DocumentReferenceStatus> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new DocumentReference.DocumentReferenceStatusEnumFactory()); 231 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 232 if (src.getValue() == null) { 233 tgt.setValue(null); 234 } else { 235 switch (src.getValue()) { 236 case CURRENT: 237 tgt.setValue(DocumentReference.DocumentReferenceStatus.CURRENT); 238 break; 239 case SUPERSEDED: 240 tgt.setValue(DocumentReference.DocumentReferenceStatus.SUPERSEDED); 241 break; 242 case ENTEREDINERROR: 243 tgt.setValue(DocumentReference.DocumentReferenceStatus.ENTEREDINERROR); 244 break; 245 default: 246 tgt.setValue(DocumentReference.DocumentReferenceStatus.NULL); 247 break; 248 } 249 } 250 return tgt; 251 } 252 253 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.DocumentReference.DocumentRelationshipType> convertDocumentRelationshipType2(org.hl7.fhir.r5.model.CodeableConcept src) throws FHIRException { 254 if (src == null || src.isEmpty()) 255 return null; 256 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.DocumentReference.DocumentRelationshipType> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.DocumentReference.DocumentRelationshipTypeEnumFactory()); 257 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 258 switch (src.getCode("http://hl7.org/fhir/document-relationship-type")) { 259 case "replaces": 260 tgt.setValue(org.hl7.fhir.dstu3.model.DocumentReference.DocumentRelationshipType.REPLACES); 261 break; 262 case "transforms": 263 tgt.setValue(org.hl7.fhir.dstu3.model.DocumentReference.DocumentRelationshipType.TRANSFORMS); 264 break; 265 case "signs": 266 tgt.setValue(org.hl7.fhir.dstu3.model.DocumentReference.DocumentRelationshipType.SIGNS); 267 break; 268 case "appends": 269 tgt.setValue(org.hl7.fhir.dstu3.model.DocumentReference.DocumentRelationshipType.APPENDS); 270 break; 271 default: 272 tgt.setValue(org.hl7.fhir.dstu3.model.DocumentReference.DocumentRelationshipType.NULL); 273 break; 274 } 275 276 return tgt; 277 } 278 279 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.DocumentReference.ReferredDocumentStatus> convertReferredDocumentStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.CompositionStatus> src) throws FHIRException { 280 if (src == null || src.isEmpty()) 281 return null; 282 Enumeration<org.hl7.fhir.dstu3.model.DocumentReference.ReferredDocumentStatus> tgt = new Enumeration<>(new org.hl7.fhir.dstu3.model.DocumentReference.ReferredDocumentStatusEnumFactory()); 283 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 284 if (src.getValue() == null) { 285 tgt.setValue(null); 286 } else { 287 switch (src.getValue()) { 288 case PRELIMINARY: 289 tgt.setValue(org.hl7.fhir.dstu3.model.DocumentReference.ReferredDocumentStatus.PRELIMINARY); 290 break; 291 case FINAL: 292 tgt.setValue(org.hl7.fhir.dstu3.model.DocumentReference.ReferredDocumentStatus.FINAL); 293 break; 294 case AMENDED: 295 tgt.setValue(org.hl7.fhir.dstu3.model.DocumentReference.ReferredDocumentStatus.AMENDED); 296 break; 297 case ENTEREDINERROR: 298 tgt.setValue(org.hl7.fhir.dstu3.model.DocumentReference.ReferredDocumentStatus.ENTEREDINERROR); 299 break; 300 default: 301 tgt.setValue(org.hl7.fhir.dstu3.model.DocumentReference.ReferredDocumentStatus.NULL); 302 break; 303 } 304 } 305 return tgt; 306 } 307 308 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.CompositionStatus> convertReferredDocumentStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.DocumentReference.ReferredDocumentStatus> src) throws FHIRException { 309 if (src == null || src.isEmpty()) 310 return null; 311 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.CompositionStatus> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Enumerations.CompositionStatusEnumFactory()); 312 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 313 if (src.getValue() == null) { 314 tgt.setValue(null); 315 } else { 316 switch (src.getValue()) { 317 case PRELIMINARY: 318 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.CompositionStatus.PRELIMINARY); 319 break; 320 case FINAL: 321 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.CompositionStatus.FINAL); 322 break; 323 case AMENDED: 324 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.CompositionStatus.AMENDED); 325 break; 326 case ENTEREDINERROR: 327 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.CompositionStatus.ENTEREDINERROR); 328 break; 329 default: 330 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.CompositionStatus.NULL); 331 break; 332 } 333 } 334 return tgt; 335 } 336 337 static public org.hl7.fhir.r5.model.CodeableConcept convertDocumentRelationshipType(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.DocumentReference.DocumentRelationshipType> src) throws FHIRException { 338 if (src == null || src.isEmpty()) return null; 339 org.hl7.fhir.r5.model.CodeableConcept tgt = new org.hl7.fhir.r5.model.CodeableConcept(); 340 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 341 if (src.getValue() == null) { 342 } else { 343 if (src.getValue() == null) { 344 // Add nothing 345 } else { 346 switch (src.getValue()) { 347 case REPLACES: 348 tgt.addCoding().setSystem("http://hl7.org/fhir/document-relationship-type").setCode("replaces"); 349 break; 350 case TRANSFORMS: 351 tgt.addCoding().setSystem("http://hl7.org/fhir/document-relationship-type").setCode("transforms"); 352 break; 353 case SIGNS: 354 tgt.addCoding().setSystem("http://hl7.org/fhir/document-relationship-type").setCode("signs"); 355 break; 356 case APPENDS: 357 tgt.addCoding().setSystem("http://hl7.org/fhir/document-relationship-type").setCode("appends"); 358 break; 359 default: 360 break; 361 } 362 } 363 364 } 365 return tgt; 366 } 367}