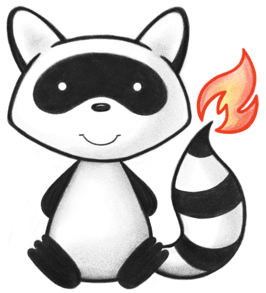
001package org.hl7.fhir.convertors.conv30_50.resources30_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_50; 004import org.hl7.fhir.convertors.conv30_50.datatypes30_50.Reference30_50; 005import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.CodeableConcept30_50; 006import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Coding30_50; 007import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Duration30_50; 008import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Identifier30_50; 009import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Period30_50; 010import org.hl7.fhir.exceptions.FHIRException; 011import org.hl7.fhir.r5.model.CodeableReference; 012import org.hl7.fhir.r5.model.Encounter; 013import org.hl7.fhir.r5.model.Encounter.ReasonComponent; 014import org.hl7.fhir.r5.model.Enumeration; 015import org.hl7.fhir.r5.model.Enumerations; 016 017public class Encounter30_50 { 018 019// public static org.hl7.fhir.dstu3.model.Encounter.ClassHistoryComponent convertClassHistoryComponent(org.hl7.fhir.r5.model.Encounter.ClassHistoryComponent src) throws FHIRException { 020// if (src == null) 021// return null; 022// org.hl7.fhir.dstu3.model.Encounter.ClassHistoryComponent tgt = new org.hl7.fhir.dstu3.model.Encounter.ClassHistoryComponent(); 023// ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 024// if (src.hasClass_()) 025// tgt.setClass_(Coding30_50.convertCoding(src.getClass_())); 026// if (src.hasPeriod()) 027// tgt.setPeriod(Period30_50.convertPeriod(src.getPeriod())); 028// return tgt; 029// } 030// 031// public static org.hl7.fhir.r5.model.Encounter.ClassHistoryComponent convertClassHistoryComponent(org.hl7.fhir.dstu3.model.Encounter.ClassHistoryComponent src) throws FHIRException { 032// if (src == null) 033// return null; 034// org.hl7.fhir.r5.model.Encounter.ClassHistoryComponent tgt = new org.hl7.fhir.r5.model.Encounter.ClassHistoryComponent(); 035// ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 036// if (src.hasClass_()) 037// tgt.setClass_(Coding30_50.convertCoding(src.getClass_())); 038// if (src.hasPeriod()) 039// tgt.setPeriod(Period30_50.convertPeriod(src.getPeriod())); 040// return tgt; 041// } 042 043 public static org.hl7.fhir.r5.model.Encounter convertEncounter(org.hl7.fhir.dstu3.model.Encounter src) throws FHIRException { 044 if (src == null) 045 return null; 046 org.hl7.fhir.r5.model.Encounter tgt = new org.hl7.fhir.r5.model.Encounter(); 047 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 048 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 049 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 050 if (src.hasStatus()) 051 tgt.setStatusElement(convertEncounterStatus(src.getStatusElement())); 052// for (org.hl7.fhir.dstu3.model.Encounter.StatusHistoryComponent t : src.getStatusHistory()) 053// tgt.addStatusHistory(convertStatusHistoryComponent(t)); 054 if (src.hasClass_()) 055 tgt.addClass_(new org.hl7.fhir.r5.model.CodeableConcept().addCoding(Coding30_50.convertCoding(src.getClass_()))); 056// for (org.hl7.fhir.dstu3.model.Encounter.ClassHistoryComponent t : src.getClassHistory()) 057// tgt.addClassHistory(convertClassHistoryComponent(t)); 058 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getType()) 059 tgt.addType(CodeableConcept30_50.convertCodeableConcept(t)); 060 if (src.hasPriority()) 061 tgt.setPriority(CodeableConcept30_50.convertCodeableConcept(src.getPriority())); 062 if (src.hasSubject()) 063 tgt.setSubject(Reference30_50.convertReference(src.getSubject())); 064 for (org.hl7.fhir.dstu3.model.Reference t : src.getEpisodeOfCare()) 065 tgt.addEpisodeOfCare(Reference30_50.convertReference(t)); 066 for (org.hl7.fhir.dstu3.model.Reference t : src.getIncomingReferral()) 067 tgt.addBasedOn(Reference30_50.convertReference(t)); 068 for (org.hl7.fhir.dstu3.model.Encounter.EncounterParticipantComponent t : src.getParticipant()) 069 tgt.addParticipant(convertEncounterParticipantComponent(t)); 070 if (src.hasAppointment()) 071 tgt.addAppointment(Reference30_50.convertReference(src.getAppointment())); 072 if (src.hasPeriod()) 073 tgt.setActualPeriod(Period30_50.convertPeriod(src.getPeriod())); 074 if (src.hasLength()) 075 tgt.setLength(Duration30_50.convertDuration(src.getLength())); 076 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getReason()) 077 tgt.addReason().addValue(Reference30_50.convertCodeableConceptToCodableReference(t)); 078 for (org.hl7.fhir.dstu3.model.Encounter.DiagnosisComponent t : src.getDiagnosis()) 079 tgt.addDiagnosis(convertDiagnosisComponent(t)); 080 for (org.hl7.fhir.dstu3.model.Reference t : src.getAccount()) tgt.addAccount(Reference30_50.convertReference(t)); 081 if (src.hasHospitalization()) 082 tgt.setAdmission(convertEncounterHospitalizationComponent(src.getHospitalization(), tgt)); 083 for (org.hl7.fhir.dstu3.model.Encounter.EncounterLocationComponent t : src.getLocation()) 084 tgt.addLocation(convertEncounterLocationComponent(t)); 085 if (src.hasServiceProvider()) 086 tgt.setServiceProvider(Reference30_50.convertReference(src.getServiceProvider())); 087 if (src.hasPartOf()) 088 tgt.setPartOf(Reference30_50.convertReference(src.getPartOf())); 089 return tgt; 090 } 091 092 public static org.hl7.fhir.dstu3.model.Encounter convertEncounter(org.hl7.fhir.r5.model.Encounter src) throws FHIRException { 093 if (src == null) 094 return null; 095 org.hl7.fhir.dstu3.model.Encounter tgt = new org.hl7.fhir.dstu3.model.Encounter(); 096 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 097 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 098 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 099 if (src.hasStatus()) 100 tgt.setStatusElement(convertEncounterStatus(src.getStatusElement())); 101// for (org.hl7.fhir.r5.model.Encounter.StatusHistoryComponent t : src.getStatusHistory()) 102// tgt.addStatusHistory(convertStatusHistoryComponent(t)); 103 if (src.hasClass_()) 104 tgt.setClass_(Coding30_50.convertCoding(src.getClass_FirstRep())); 105// for (org.hl7.fhir.r5.model.Encounter.ClassHistoryComponent t : src.getClassHistory()) 106// tgt.addClassHistory(convertClassHistoryComponent(t)); 107 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getType()) 108 tgt.addType(CodeableConcept30_50.convertCodeableConcept(t)); 109 if (src.hasPriority()) 110 tgt.setPriority(CodeableConcept30_50.convertCodeableConcept(src.getPriority())); 111 if (src.hasSubject()) 112 tgt.setSubject(Reference30_50.convertReference(src.getSubject())); 113 for (org.hl7.fhir.r5.model.Reference t : src.getEpisodeOfCare()) 114 tgt.addEpisodeOfCare(Reference30_50.convertReference(t)); 115 for (org.hl7.fhir.r5.model.Reference t : src.getBasedOn()) 116 tgt.addIncomingReferral(Reference30_50.convertReference(t)); 117 for (org.hl7.fhir.r5.model.Encounter.EncounterParticipantComponent t : src.getParticipant()) 118 tgt.addParticipant(convertEncounterParticipantComponent(t)); 119 if (src.hasAppointment()) 120 tgt.setAppointment(Reference30_50.convertReference(src.getAppointmentFirstRep())); 121 if (src.hasActualPeriod()) 122 tgt.setPeriod(Period30_50.convertPeriod(src.getActualPeriod())); 123 if (src.hasLength()) 124 tgt.setLength(Duration30_50.convertDuration(src.getLength())); 125 for (ReasonComponent t1 : src.getReason()) 126 for (CodeableReference t : t1.getValue()) 127 if (t.hasConcept()) 128 tgt.addReason(CodeableConcept30_50.convertCodeableConcept(t.getConcept())); 129 for (org.hl7.fhir.r5.model.Encounter.DiagnosisComponent t : src.getDiagnosis()) 130 tgt.addDiagnosis(convertDiagnosisComponent(t)); 131 for (org.hl7.fhir.r5.model.Reference t : src.getAccount()) tgt.addAccount(Reference30_50.convertReference(t)); 132 if (src.hasAdmission() || src.hasDietPreference() || src.hasSpecialArrangement() || src.hasSpecialCourtesy()) 133 tgt.setHospitalization(convertEncounterHospitalizationComponent(src.getAdmission(), src)); 134 for (org.hl7.fhir.r5.model.Encounter.EncounterLocationComponent t : src.getLocation()) 135 tgt.addLocation(convertEncounterLocationComponent(t)); 136 if (src.hasServiceProvider()) 137 tgt.setServiceProvider(Reference30_50.convertReference(src.getServiceProvider())); 138 if (src.hasPartOf()) 139 tgt.setPartOf(Reference30_50.convertReference(src.getPartOf())); 140 return tgt; 141 } 142 143 public static org.hl7.fhir.dstu3.model.Encounter.EncounterHospitalizationComponent convertEncounterHospitalizationComponent(org.hl7.fhir.r5.model.Encounter.EncounterAdmissionComponent src, org.hl7.fhir.r5.model.Encounter srce) throws FHIRException { 144 if (src == null) 145 return null; 146 org.hl7.fhir.dstu3.model.Encounter.EncounterHospitalizationComponent tgt = new org.hl7.fhir.dstu3.model.Encounter.EncounterHospitalizationComponent(); 147 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 148 if (src.hasPreAdmissionIdentifier()) 149 tgt.setPreAdmissionIdentifier(Identifier30_50.convertIdentifier(src.getPreAdmissionIdentifier())); 150 if (src.hasOrigin()) 151 tgt.setOrigin(Reference30_50.convertReference(src.getOrigin())); 152 if (src.hasAdmitSource()) 153 tgt.setAdmitSource(CodeableConcept30_50.convertCodeableConcept(src.getAdmitSource())); 154 if (src.hasReAdmission()) 155 tgt.setReAdmission(CodeableConcept30_50.convertCodeableConcept(src.getReAdmission())); 156 for (org.hl7.fhir.r5.model.CodeableConcept t : srce.getDietPreference()) 157 tgt.addDietPreference(CodeableConcept30_50.convertCodeableConcept(t)); 158 for (org.hl7.fhir.r5.model.CodeableConcept t : srce.getSpecialCourtesy()) 159 tgt.addSpecialCourtesy(CodeableConcept30_50.convertCodeableConcept(t)); 160 for (org.hl7.fhir.r5.model.CodeableConcept t : srce.getSpecialArrangement()) 161 tgt.addSpecialArrangement(CodeableConcept30_50.convertCodeableConcept(t)); 162 if (src.hasDestination()) 163 tgt.setDestination(Reference30_50.convertReference(src.getDestination())); 164 if (src.hasDischargeDisposition()) 165 tgt.setDischargeDisposition(CodeableConcept30_50.convertCodeableConcept(src.getDischargeDisposition())); 166 return tgt; 167 } 168 169 public static org.hl7.fhir.r5.model.Encounter.EncounterAdmissionComponent convertEncounterHospitalizationComponent(org.hl7.fhir.dstu3.model.Encounter.EncounterHospitalizationComponent src, org.hl7.fhir.r5.model.Encounter tgte) throws FHIRException { 170 if (src == null) 171 return null; 172 org.hl7.fhir.r5.model.Encounter.EncounterAdmissionComponent tgt = new org.hl7.fhir.r5.model.Encounter.EncounterAdmissionComponent(); 173 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 174 if (src.hasPreAdmissionIdentifier()) 175 tgt.setPreAdmissionIdentifier(Identifier30_50.convertIdentifier(src.getPreAdmissionIdentifier())); 176 if (src.hasOrigin()) 177 tgt.setOrigin(Reference30_50.convertReference(src.getOrigin())); 178 if (src.hasAdmitSource()) 179 tgt.setAdmitSource(CodeableConcept30_50.convertCodeableConcept(src.getAdmitSource())); 180 if (src.hasReAdmission()) 181 tgt.setReAdmission(CodeableConcept30_50.convertCodeableConcept(src.getReAdmission())); 182 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getDietPreference()) 183 tgte.addDietPreference(CodeableConcept30_50.convertCodeableConcept(t)); 184 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getSpecialCourtesy()) 185 tgte.addSpecialCourtesy(CodeableConcept30_50.convertCodeableConcept(t)); 186 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getSpecialArrangement()) 187 tgte.addSpecialArrangement(CodeableConcept30_50.convertCodeableConcept(t)); 188 if (src.hasDestination()) 189 tgt.setDestination(Reference30_50.convertReference(src.getDestination())); 190 if (src.hasDischargeDisposition()) 191 tgt.setDischargeDisposition(CodeableConcept30_50.convertCodeableConcept(src.getDischargeDisposition())); 192 return tgt; 193 } 194 195 public static org.hl7.fhir.dstu3.model.Encounter.EncounterLocationComponent convertEncounterLocationComponent(org.hl7.fhir.r5.model.Encounter.EncounterLocationComponent src) throws FHIRException { 196 if (src == null) 197 return null; 198 org.hl7.fhir.dstu3.model.Encounter.EncounterLocationComponent tgt = new org.hl7.fhir.dstu3.model.Encounter.EncounterLocationComponent(); 199 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 200 if (src.hasLocation()) 201 tgt.setLocation(Reference30_50.convertReference(src.getLocation())); 202 if (src.hasStatus()) 203 tgt.setStatusElement(convertEncounterLocationStatus(src.getStatusElement())); 204 if (src.hasPeriod()) 205 tgt.setPeriod(Period30_50.convertPeriod(src.getPeriod())); 206 return tgt; 207 } 208 209 public static org.hl7.fhir.r5.model.Encounter.EncounterLocationComponent convertEncounterLocationComponent(org.hl7.fhir.dstu3.model.Encounter.EncounterLocationComponent src) throws FHIRException { 210 if (src == null) 211 return null; 212 org.hl7.fhir.r5.model.Encounter.EncounterLocationComponent tgt = new org.hl7.fhir.r5.model.Encounter.EncounterLocationComponent(); 213 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 214 if (src.hasLocation()) 215 tgt.setLocation(Reference30_50.convertReference(src.getLocation())); 216 if (src.hasStatus()) 217 tgt.setStatusElement(convertEncounterLocationStatus(src.getStatusElement())); 218 if (src.hasPeriod()) 219 tgt.setPeriod(Period30_50.convertPeriod(src.getPeriod())); 220 return tgt; 221 } 222 223 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Encounter.EncounterLocationStatus> convertEncounterLocationStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Encounter.EncounterLocationStatus> src) throws FHIRException { 224 if (src == null || src.isEmpty()) 225 return null; 226 Enumeration<Encounter.EncounterLocationStatus> tgt = new Enumeration<>(new Encounter.EncounterLocationStatusEnumFactory()); 227 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 228 if (src.getValue() == null) { 229 tgt.setValue(null); 230 } else { 231 switch (src.getValue()) { 232 case PLANNED: 233 tgt.setValue(Encounter.EncounterLocationStatus.PLANNED); 234 break; 235 case ACTIVE: 236 tgt.setValue(Encounter.EncounterLocationStatus.ACTIVE); 237 break; 238 case RESERVED: 239 tgt.setValue(Encounter.EncounterLocationStatus.RESERVED); 240 break; 241 case COMPLETED: 242 tgt.setValue(Encounter.EncounterLocationStatus.COMPLETED); 243 break; 244 default: 245 tgt.setValue(Encounter.EncounterLocationStatus.NULL); 246 break; 247 } 248 } 249 return tgt; 250 } 251 252 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Encounter.EncounterLocationStatus> convertEncounterLocationStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Encounter.EncounterLocationStatus> src) throws FHIRException { 253 if (src == null || src.isEmpty()) 254 return null; 255 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Encounter.EncounterLocationStatus> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Encounter.EncounterLocationStatusEnumFactory()); 256 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 257 if (src.getValue() == null) { 258 tgt.setValue(null); 259 } else { 260 switch (src.getValue()) { 261 case PLANNED: 262 tgt.setValue(org.hl7.fhir.dstu3.model.Encounter.EncounterLocationStatus.PLANNED); 263 break; 264 case ACTIVE: 265 tgt.setValue(org.hl7.fhir.dstu3.model.Encounter.EncounterLocationStatus.ACTIVE); 266 break; 267 case RESERVED: 268 tgt.setValue(org.hl7.fhir.dstu3.model.Encounter.EncounterLocationStatus.RESERVED); 269 break; 270 case COMPLETED: 271 tgt.setValue(org.hl7.fhir.dstu3.model.Encounter.EncounterLocationStatus.COMPLETED); 272 break; 273 default: 274 tgt.setValue(org.hl7.fhir.dstu3.model.Encounter.EncounterLocationStatus.NULL); 275 break; 276 } 277 } 278 return tgt; 279 } 280 281 public static org.hl7.fhir.dstu3.model.Encounter.EncounterParticipantComponent convertEncounterParticipantComponent(org.hl7.fhir.r5.model.Encounter.EncounterParticipantComponent src) throws FHIRException { 282 if (src == null) 283 return null; 284 org.hl7.fhir.dstu3.model.Encounter.EncounterParticipantComponent tgt = new org.hl7.fhir.dstu3.model.Encounter.EncounterParticipantComponent(); 285 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 286 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getType()) 287 tgt.addType(CodeableConcept30_50.convertCodeableConcept(t)); 288 if (src.hasPeriod()) 289 tgt.setPeriod(Period30_50.convertPeriod(src.getPeriod())); 290 if (src.hasActor()) 291 tgt.setIndividual(Reference30_50.convertReference(src.getActor())); 292 return tgt; 293 } 294 295 public static org.hl7.fhir.r5.model.Encounter.EncounterParticipantComponent convertEncounterParticipantComponent(org.hl7.fhir.dstu3.model.Encounter.EncounterParticipantComponent src) throws FHIRException { 296 if (src == null) 297 return null; 298 org.hl7.fhir.r5.model.Encounter.EncounterParticipantComponent tgt = new org.hl7.fhir.r5.model.Encounter.EncounterParticipantComponent(); 299 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 300 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getType()) 301 tgt.addType(CodeableConcept30_50.convertCodeableConcept(t)); 302 if (src.hasPeriod()) 303 tgt.setPeriod(Period30_50.convertPeriod(src.getPeriod())); 304 if (src.hasIndividual()) 305 tgt.setActor(Reference30_50.convertReference(src.getIndividual())); 306 return tgt; 307 } 308 309 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Encounter.EncounterStatus> convertEncounterStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.EncounterStatus> src) throws FHIRException { 310 if (src == null || src.isEmpty()) 311 return null; 312 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Encounter.EncounterStatus> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Encounter.EncounterStatusEnumFactory()); 313 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 314 if (src.getValue() == null) { 315 tgt.setValue(null); 316 } else { 317 switch (src.getValue()) { 318 case PLANNED: 319 tgt.setValue(org.hl7.fhir.dstu3.model.Encounter.EncounterStatus.PLANNED); 320 break; 321 case INPROGRESS: 322 tgt.setValue(org.hl7.fhir.dstu3.model.Encounter.EncounterStatus.INPROGRESS); 323 break; 324 case COMPLETED: 325 tgt.setValue(org.hl7.fhir.dstu3.model.Encounter.EncounterStatus.FINISHED); 326 break; 327 case CANCELLED: 328 tgt.setValue(org.hl7.fhir.dstu3.model.Encounter.EncounterStatus.CANCELLED); 329 break; 330 case ENTEREDINERROR: 331 tgt.setValue(org.hl7.fhir.dstu3.model.Encounter.EncounterStatus.ENTEREDINERROR); 332 break; 333 case UNKNOWN: 334 tgt.setValue(org.hl7.fhir.dstu3.model.Encounter.EncounterStatus.UNKNOWN); 335 break; 336 default: 337 tgt.setValue(org.hl7.fhir.dstu3.model.Encounter.EncounterStatus.NULL); 338 break; 339 } 340 } 341 return tgt; 342 } 343 344 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.EncounterStatus> convertEncounterStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Encounter.EncounterStatus> src) throws FHIRException { 345 if (src == null || src.isEmpty()) 346 return null; 347 Enumeration<Enumerations.EncounterStatus> tgt = new Enumeration<>(new Enumerations.EncounterStatusEnumFactory()); 348 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 349 if (src.getValue() == null) { 350 tgt.setValue(null); 351 } else { 352 switch (src.getValue()) { 353 case PLANNED: 354 tgt.setValue(Enumerations.EncounterStatus.PLANNED); 355 break; 356 case ARRIVED: 357 tgt.setValue(Enumerations.EncounterStatus.INPROGRESS); 358 break; 359 case TRIAGED: 360 tgt.setValue(Enumerations.EncounterStatus.INPROGRESS); 361 break; 362 case INPROGRESS: 363 tgt.setValue(Enumerations.EncounterStatus.INPROGRESS); 364 break; 365 case ONLEAVE: 366 tgt.setValue(Enumerations.EncounterStatus.INPROGRESS); 367 break; 368 case FINISHED: 369 tgt.setValue(Enumerations.EncounterStatus.COMPLETED); 370 break; 371 case CANCELLED: 372 tgt.setValue(Enumerations.EncounterStatus.CANCELLED); 373 break; 374 case ENTEREDINERROR: 375 tgt.setValue(Enumerations.EncounterStatus.ENTEREDINERROR); 376 break; 377 case UNKNOWN: 378 tgt.setValue(Enumerations.EncounterStatus.UNKNOWN); 379 break; 380 default: 381 tgt.setValue(Enumerations.EncounterStatus.NULL); 382 break; 383 } 384 } 385 return tgt; 386 } 387 388// public static org.hl7.fhir.r5.model.Encounter.StatusHistoryComponent convertStatusHistoryComponent(org.hl7.fhir.dstu3.model.Encounter.StatusHistoryComponent src) throws FHIRException { 389// if (src == null) 390// return null; 391// org.hl7.fhir.r5.model.Encounter.StatusHistoryComponent tgt = new org.hl7.fhir.r5.model.Encounter.StatusHistoryComponent(); 392// ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 393// if (src.hasStatus()) 394// tgt.setStatusElement(convertEncounterStatus(src.getStatusElement())); 395// if (src.hasPeriod()) 396// tgt.setPeriod(Period30_50.convertPeriod(src.getPeriod())); 397// return tgt; 398// } 399// 400// public static org.hl7.fhir.dstu3.model.Encounter.StatusHistoryComponent convertStatusHistoryComponent(org.hl7.fhir.r5.model.Encounter.StatusHistoryComponent src) throws FHIRException { 401// if (src == null) 402// return null; 403// org.hl7.fhir.dstu3.model.Encounter.StatusHistoryComponent tgt = new org.hl7.fhir.dstu3.model.Encounter.StatusHistoryComponent(); 404// ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 405// if (src.hasStatus()) 406// tgt.setStatusElement(convertEncounterStatus(src.getStatusElement())); 407// if (src.hasPeriod()) 408// tgt.setPeriod(Period30_50.convertPeriod(src.getPeriod())); 409// return tgt; 410// } 411 412 public static org.hl7.fhir.r5.model.Encounter.DiagnosisComponent convertDiagnosisComponent(org.hl7.fhir.dstu3.model.Encounter.DiagnosisComponent src) throws FHIRException { 413 if (src == null) return null; 414 org.hl7.fhir.r5.model.Encounter.DiagnosisComponent tgt = new org.hl7.fhir.r5.model.Encounter.DiagnosisComponent(); 415 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 416 if (src.hasCondition()) tgt.addCondition(Reference30_50.convertReferenceToCodableReference(src.getCondition())); 417 if (src.hasRole()) tgt.addUse(CodeableConcept30_50.convertCodeableConcept(src.getRole())); 418// if (src.hasRank()) tgt.setRankElement(PositiveInt30_50.convertPositiveInt(src.getRankElement())); 419 return tgt; 420 } 421 422 public static org.hl7.fhir.dstu3.model.Encounter.DiagnosisComponent convertDiagnosisComponent(org.hl7.fhir.r5.model.Encounter.DiagnosisComponent src) throws FHIRException { 423 if (src == null) return null; 424 org.hl7.fhir.dstu3.model.Encounter.DiagnosisComponent tgt = new org.hl7.fhir.dstu3.model.Encounter.DiagnosisComponent(); 425 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 426 if (src.hasCondition() && src.getConditionFirstRep().hasReference()) tgt.setCondition(Reference30_50.convertReference(src.getConditionFirstRep().getReference())); 427 if (src.hasUse()) tgt.setRole(CodeableConcept30_50.convertCodeableConcept(src.getUseFirstRep())); 428// if (src.hasRank()) tgt.setRankElement(PositiveInt30_50.convertPositiveInt(src.getRankElement())); 429 return tgt; 430 } 431}