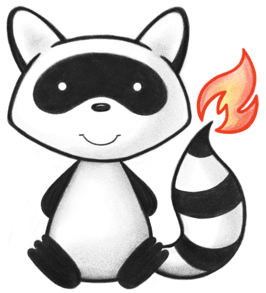
001package org.hl7.fhir.convertors.conv30_50.resources30_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_50; 004import org.hl7.fhir.convertors.conv30_50.datatypes30_50.Reference30_50; 005import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.CodeableConcept30_50; 006import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Coding30_50; 007import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.ContactPoint30_50; 008import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Identifier30_50; 009import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Period30_50; 010import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Code30_50; 011import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.String30_50; 012import org.hl7.fhir.exceptions.FHIRException; 013import org.hl7.fhir.r5.model.Endpoint; 014import org.hl7.fhir.r5.model.Endpoint.EndpointPayloadComponent; 015import org.hl7.fhir.r5.model.Enumeration; 016 017public class Endpoint30_50 { 018 019 public static org.hl7.fhir.dstu3.model.Endpoint convertEndpoint(org.hl7.fhir.r5.model.Endpoint src) throws FHIRException { 020 if (src == null) 021 return null; 022 org.hl7.fhir.dstu3.model.Endpoint tgt = new org.hl7.fhir.dstu3.model.Endpoint(); 023 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 024 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 025 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 026 if (src.hasStatus()) 027 tgt.setStatusElement(convertEndpointStatus(src.getStatusElement())); 028 if (src.hasConnectionType()) 029 tgt.setConnectionType(Coding30_50.convertCoding(src.getConnectionTypeFirstRep())); 030 if (src.hasName()) 031 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 032 if (src.hasManagingOrganization()) 033 tgt.setManagingOrganization(Reference30_50.convertReference(src.getManagingOrganization())); 034 for (org.hl7.fhir.r5.model.ContactPoint t : src.getContact()) 035 tgt.addContact(ContactPoint30_50.convertContactPoint(t)); 036 if (src.hasPeriod()) 037 tgt.setPeriod(Period30_50.convertPeriod(src.getPeriod())); 038 for (EndpointPayloadComponent t : src.getPayload()) 039 if (t.hasType()) 040 tgt.addPayloadType(CodeableConcept30_50.convertCodeableConcept(t.getTypeFirstRep())); 041 for (EndpointPayloadComponent t : src.getPayload()) 042 if (t.hasMimeType()) 043 tgt.getPayloadMimeType().add(Code30_50.convertCode(t.getMimeType().get(0))); 044 if (src.hasAddress()) 045 tgt.setAddress(src.getAddress()); 046 for (org.hl7.fhir.r5.model.StringType t : src.getHeader()) tgt.addHeader(t.getValue()); 047 return tgt; 048 } 049 050 public static org.hl7.fhir.r5.model.Endpoint convertEndpoint(org.hl7.fhir.dstu3.model.Endpoint src) throws FHIRException { 051 if (src == null) 052 return null; 053 org.hl7.fhir.r5.model.Endpoint tgt = new org.hl7.fhir.r5.model.Endpoint(); 054 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 055 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 056 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 057 if (src.hasStatus()) 058 tgt.setStatusElement(convertEndpointStatus(src.getStatusElement())); 059 if (src.hasConnectionType()) 060 tgt.addConnectionType(Coding30_50.convertCodingToCodeableConcept(src.getConnectionType())); 061 if (src.hasName()) 062 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 063 if (src.hasManagingOrganization()) 064 tgt.setManagingOrganization(Reference30_50.convertReference(src.getManagingOrganization())); 065 for (org.hl7.fhir.dstu3.model.ContactPoint t : src.getContact()) 066 tgt.addContact(ContactPoint30_50.convertContactPoint(t)); 067 if (src.hasPeriod()) 068 tgt.setPeriod(Period30_50.convertPeriod(src.getPeriod())); 069 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getPayloadType()) 070 tgt.addPayload().addType(CodeableConcept30_50.convertCodeableConcept(t)); 071 for (org.hl7.fhir.dstu3.model.CodeType t : src.getPayloadMimeType()) tgt.addPayload().addMimeType(t.getValue()); 072 if (src.hasAddress()) 073 tgt.setAddress(src.getAddress()); 074 for (org.hl7.fhir.dstu3.model.StringType t : src.getHeader()) tgt.addHeader(t.getValue()); 075 return tgt; 076 } 077 078 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Endpoint.EndpointStatus> convertEndpointStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Endpoint.EndpointStatus> src) throws FHIRException { 079 if (src == null || src.isEmpty()) 080 return null; 081 Enumeration<Endpoint.EndpointStatus> tgt = new Enumeration<>(new Endpoint.EndpointStatusEnumFactory()); 082 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 083 if (src.getValue() == null) { 084 tgt.setValue(null); 085 } else { 086 switch (src.getValue()) { 087 case ACTIVE: 088 tgt.setValue(Endpoint.EndpointStatus.ACTIVE); 089 break; 090 case SUSPENDED: 091 tgt.setValue(Endpoint.EndpointStatus.SUSPENDED); 092 break; 093 case ERROR: 094 tgt.setValue(Endpoint.EndpointStatus.ERROR); 095 break; 096 case OFF: 097 tgt.setValue(Endpoint.EndpointStatus.OFF); 098 break; 099 case ENTEREDINERROR: 100 tgt.setValue(Endpoint.EndpointStatus.ENTEREDINERROR); 101 break; 102 default: 103 tgt.setValue(Endpoint.EndpointStatus.NULL); 104 break; 105 } 106 } 107 return tgt; 108 } 109 110 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Endpoint.EndpointStatus> convertEndpointStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Endpoint.EndpointStatus> src) throws FHIRException { 111 if (src == null || src.isEmpty()) 112 return null; 113 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Endpoint.EndpointStatus> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Endpoint.EndpointStatusEnumFactory()); 114 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 115 if (src.getValue() == null) { 116 tgt.setValue(null); 117 } else { 118 switch (src.getValue()) { 119 case ACTIVE: 120 tgt.setValue(org.hl7.fhir.dstu3.model.Endpoint.EndpointStatus.ACTIVE); 121 break; 122 case SUSPENDED: 123 tgt.setValue(org.hl7.fhir.dstu3.model.Endpoint.EndpointStatus.SUSPENDED); 124 break; 125 case ERROR: 126 tgt.setValue(org.hl7.fhir.dstu3.model.Endpoint.EndpointStatus.ERROR); 127 break; 128 case OFF: 129 tgt.setValue(org.hl7.fhir.dstu3.model.Endpoint.EndpointStatus.OFF); 130 break; 131 case ENTEREDINERROR: 132 tgt.setValue(org.hl7.fhir.dstu3.model.Endpoint.EndpointStatus.ENTEREDINERROR); 133 break; 134 default: 135 tgt.setValue(org.hl7.fhir.dstu3.model.Endpoint.EndpointStatus.NULL); 136 break; 137 } 138 } 139 return tgt; 140 } 141}