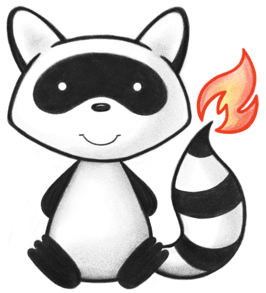
001package org.hl7.fhir.convertors.conv30_50.resources30_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_50; 004import org.hl7.fhir.convertors.conv30_50.datatypes30_50.Reference30_50; 005import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.CodeableConcept30_50; 006import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Identifier30_50; 007import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Period30_50; 008import org.hl7.fhir.dstu3.model.Enumeration; 009import org.hl7.fhir.dstu3.model.Flag; 010import org.hl7.fhir.exceptions.FHIRException; 011 012public class Flag30_50 { 013 014 public static org.hl7.fhir.r5.model.Flag convertFlag(org.hl7.fhir.dstu3.model.Flag src) throws FHIRException { 015 if (src == null) 016 return null; 017 org.hl7.fhir.r5.model.Flag tgt = new org.hl7.fhir.r5.model.Flag(); 018 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 019 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 020 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 021 if (src.hasStatus()) 022 tgt.setStatusElement(convertFlagStatus(src.getStatusElement())); 023 if (src.hasCategory()) 024 tgt.addCategory(CodeableConcept30_50.convertCodeableConcept(src.getCategory())); 025 if (src.hasCode()) 026 tgt.setCode(CodeableConcept30_50.convertCodeableConcept(src.getCode())); 027 if (src.hasSubject()) 028 tgt.setSubject(Reference30_50.convertReference(src.getSubject())); 029 if (src.hasPeriod()) 030 tgt.setPeriod(Period30_50.convertPeriod(src.getPeriod())); 031 if (src.hasEncounter()) 032 tgt.setEncounter(Reference30_50.convertReference(src.getEncounter())); 033 if (src.hasAuthor()) 034 tgt.setAuthor(Reference30_50.convertReference(src.getAuthor())); 035 return tgt; 036 } 037 038 public static org.hl7.fhir.dstu3.model.Flag convertFlag(org.hl7.fhir.r5.model.Flag src) throws FHIRException { 039 if (src == null) 040 return null; 041 org.hl7.fhir.dstu3.model.Flag tgt = new org.hl7.fhir.dstu3.model.Flag(); 042 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 043 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 044 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 045 if (src.hasStatus()) 046 tgt.setStatusElement(convertFlagStatus(src.getStatusElement())); 047 if (src.hasCategory()) 048 tgt.setCategory(CodeableConcept30_50.convertCodeableConcept(src.getCategoryFirstRep())); 049 if (src.hasCode()) 050 tgt.setCode(CodeableConcept30_50.convertCodeableConcept(src.getCode())); 051 if (src.hasSubject()) 052 tgt.setSubject(Reference30_50.convertReference(src.getSubject())); 053 if (src.hasPeriod()) 054 tgt.setPeriod(Period30_50.convertPeriod(src.getPeriod())); 055 if (src.hasEncounter()) 056 tgt.setEncounter(Reference30_50.convertReference(src.getEncounter())); 057 if (src.hasAuthor()) 058 tgt.setAuthor(Reference30_50.convertReference(src.getAuthor())); 059 return tgt; 060 } 061 062 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Flag.FlagStatus> convertFlagStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Flag.FlagStatus> src) throws FHIRException { 063 if (src == null || src.isEmpty()) 064 return null; 065 Enumeration<Flag.FlagStatus> tgt = new Enumeration<>(new Flag.FlagStatusEnumFactory()); 066 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 067 if (src.getValue() == null) { 068 tgt.setValue(null); 069 } else { 070 switch (src.getValue()) { 071 case ACTIVE: 072 tgt.setValue(Flag.FlagStatus.ACTIVE); 073 break; 074 case INACTIVE: 075 tgt.setValue(Flag.FlagStatus.INACTIVE); 076 break; 077 case ENTEREDINERROR: 078 tgt.setValue(Flag.FlagStatus.ENTEREDINERROR); 079 break; 080 default: 081 tgt.setValue(Flag.FlagStatus.NULL); 082 break; 083 } 084 } 085 return tgt; 086 } 087 088 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Flag.FlagStatus> convertFlagStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Flag.FlagStatus> src) throws FHIRException { 089 if (src == null || src.isEmpty()) 090 return null; 091 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Flag.FlagStatus> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Flag.FlagStatusEnumFactory()); 092 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 093 if (src.getValue() == null) { 094 tgt.setValue(null); 095 } else { 096 switch (src.getValue()) { 097 case ACTIVE: 098 tgt.setValue(org.hl7.fhir.r5.model.Flag.FlagStatus.ACTIVE); 099 break; 100 case INACTIVE: 101 tgt.setValue(org.hl7.fhir.r5.model.Flag.FlagStatus.INACTIVE); 102 break; 103 case ENTEREDINERROR: 104 tgt.setValue(org.hl7.fhir.r5.model.Flag.FlagStatus.ENTEREDINERROR); 105 break; 106 default: 107 tgt.setValue(org.hl7.fhir.r5.model.Flag.FlagStatus.NULL); 108 break; 109 } 110 } 111 return tgt; 112 } 113}