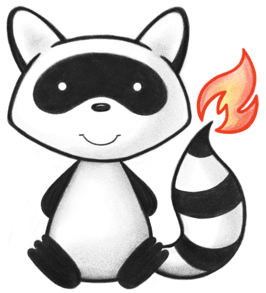
001package org.hl7.fhir.convertors.conv30_50.resources30_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_50; 004import org.hl7.fhir.convertors.conv30_50.datatypes30_50.Reference30_50; 005import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Annotation30_50; 006import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.CodeableConcept30_50; 007import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Identifier30_50; 008import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Date30_50; 009import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.String30_50; 010import org.hl7.fhir.dstu3.model.Enumeration; 011import org.hl7.fhir.dstu3.model.Goal; 012import org.hl7.fhir.exceptions.FHIRException; 013import org.hl7.fhir.r5.model.CodeableReference; 014 015public class Goal30_50 { 016 017 public static org.hl7.fhir.dstu3.model.Goal convertGoal(org.hl7.fhir.r5.model.Goal src) throws FHIRException { 018 if (src == null) 019 return null; 020 org.hl7.fhir.dstu3.model.Goal tgt = new org.hl7.fhir.dstu3.model.Goal(); 021 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 022 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 023 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 024 if (src.hasLifecycleStatus()) 025 tgt.setStatusElement(convertGoalStatus(src.getLifecycleStatusElement())); 026 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getCategory()) 027 tgt.addCategory(CodeableConcept30_50.convertCodeableConcept(t)); 028 if (src.hasPriority()) 029 tgt.setPriority(CodeableConcept30_50.convertCodeableConcept(src.getPriority())); 030 if (src.hasDescription()) 031 tgt.setDescription(CodeableConcept30_50.convertCodeableConcept(src.getDescription())); 032 if (src.hasSubject()) 033 tgt.setSubject(Reference30_50.convertReference(src.getSubject())); 034 if (src.hasStart()) 035 tgt.setStart(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getStart())); 036 if (src.hasTarget()) 037 tgt.setTarget(convertGoalTargetComponent(src.getTargetFirstRep())); 038 if (src.hasStatusDate()) 039 tgt.setStatusDateElement(Date30_50.convertDate(src.getStatusDateElement())); 040 if (src.hasStatusReason()) 041 tgt.setStatusReasonElement(String30_50.convertString(src.getStatusReasonElement())); 042 for (org.hl7.fhir.r5.model.Reference t : src.getAddresses()) tgt.addAddresses(Reference30_50.convertReference(t)); 043 for (org.hl7.fhir.r5.model.Annotation t : src.getNote()) tgt.addNote(Annotation30_50.convertAnnotation(t)); 044 for (CodeableReference t : src.getOutcome()) 045 if (t.hasConcept()) 046 tgt.addOutcomeCode(CodeableConcept30_50.convertCodeableConcept(t.getConcept())); 047 for (CodeableReference t : src.getOutcome()) 048 if (t.hasReference()) 049 tgt.addOutcomeReference(Reference30_50.convertReference(t.getReference())); 050 return tgt; 051 } 052 053 public static org.hl7.fhir.r5.model.Goal convertGoal(org.hl7.fhir.dstu3.model.Goal src) throws FHIRException { 054 if (src == null) 055 return null; 056 org.hl7.fhir.r5.model.Goal tgt = new org.hl7.fhir.r5.model.Goal(); 057 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 058 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 059 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 060 if (src.hasStatus()) 061 tgt.setLifecycleStatusElement(convertGoalStatus(src.getStatusElement())); 062 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getCategory()) 063 tgt.addCategory(CodeableConcept30_50.convertCodeableConcept(t)); 064 if (src.hasPriority()) 065 tgt.setPriority(CodeableConcept30_50.convertCodeableConcept(src.getPriority())); 066 if (src.hasDescription()) 067 tgt.setDescription(CodeableConcept30_50.convertCodeableConcept(src.getDescription())); 068 if (src.hasSubject()) 069 tgt.setSubject(Reference30_50.convertReference(src.getSubject())); 070 if (src.hasStart()) 071 tgt.setStart(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getStart())); 072 if (src.hasTarget()) 073 tgt.addTarget(convertGoalTargetComponent(src.getTarget())); 074 if (src.hasStatusDate()) 075 tgt.setStatusDateElement(Date30_50.convertDate(src.getStatusDateElement())); 076 if (src.hasStatusReason()) 077 tgt.setStatusReasonElement(String30_50.convertString(src.getStatusReasonElement())); 078 for (org.hl7.fhir.dstu3.model.Reference t : src.getAddresses()) 079 tgt.addAddresses(Reference30_50.convertReference(t)); 080 for (org.hl7.fhir.dstu3.model.Annotation t : src.getNote()) tgt.addNote(Annotation30_50.convertAnnotation(t)); 081 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getOutcomeCode()) 082 tgt.addOutcome(Reference30_50.convertCodeableConceptToCodableReference(t)); 083 for (org.hl7.fhir.dstu3.model.Reference t : src.getOutcomeReference()) 084 tgt.addOutcome(Reference30_50.convertReferenceToCodableReference(t)); 085 return tgt; 086 } 087 088 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Goal.GoalStatus> convertGoalStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Goal.GoalLifecycleStatus> src) throws FHIRException { 089 if (src == null || src.isEmpty()) 090 return null; 091 Enumeration<Goal.GoalStatus> tgt = new Enumeration<>(new Goal.GoalStatusEnumFactory()); 092 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 093 if (src.getValue() == null) { 094 tgt.setValue(null); 095 } else { 096 switch (src.getValue()) { 097 case PROPOSED: 098 tgt.setValue(Goal.GoalStatus.PROPOSED); 099 break; 100 case ACCEPTED: 101 tgt.setValue(Goal.GoalStatus.ACCEPTED); 102 break; 103 case PLANNED: 104 tgt.setValue(Goal.GoalStatus.PLANNED); 105 break; 106 case ACTIVE: 107 tgt.setValue(Goal.GoalStatus.INPROGRESS); 108 break; 109 case COMPLETED: 110 tgt.setValue(Goal.GoalStatus.ACHIEVED); 111 break; 112 case ONHOLD: 113 tgt.setValue(Goal.GoalStatus.ONHOLD); 114 break; 115 case CANCELLED: 116 tgt.setValue(Goal.GoalStatus.CANCELLED); 117 break; 118 case ENTEREDINERROR: 119 tgt.setValue(Goal.GoalStatus.ENTEREDINERROR); 120 break; 121 case REJECTED: 122 tgt.setValue(Goal.GoalStatus.REJECTED); 123 break; 124 default: 125 tgt.setValue(Goal.GoalStatus.NULL); 126 break; 127 } 128 } 129 return tgt; 130 } 131 132 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Goal.GoalLifecycleStatus> convertGoalStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Goal.GoalStatus> src) throws FHIRException { 133 if (src == null || src.isEmpty()) 134 return null; 135 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Goal.GoalLifecycleStatus> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Goal.GoalLifecycleStatusEnumFactory()); 136 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 137 if (src.getValue() == null) { 138 tgt.setValue(null); 139 } else { 140 switch (src.getValue()) { 141 case PROPOSED: 142 tgt.setValue(org.hl7.fhir.r5.model.Goal.GoalLifecycleStatus.PROPOSED); 143 break; 144 case ACCEPTED: 145 tgt.setValue(org.hl7.fhir.r5.model.Goal.GoalLifecycleStatus.ACCEPTED); 146 break; 147 case PLANNED: 148 tgt.setValue(org.hl7.fhir.r5.model.Goal.GoalLifecycleStatus.PLANNED); 149 break; 150 case INPROGRESS: 151 tgt.setValue(org.hl7.fhir.r5.model.Goal.GoalLifecycleStatus.ACTIVE); 152 break; 153 case ONTARGET: 154 tgt.setValue(org.hl7.fhir.r5.model.Goal.GoalLifecycleStatus.ACTIVE); 155 break; 156 case AHEADOFTARGET: 157 tgt.setValue(org.hl7.fhir.r5.model.Goal.GoalLifecycleStatus.ACTIVE); 158 break; 159 case BEHINDTARGET: 160 tgt.setValue(org.hl7.fhir.r5.model.Goal.GoalLifecycleStatus.ACTIVE); 161 break; 162 case SUSTAINING: 163 tgt.setValue(org.hl7.fhir.r5.model.Goal.GoalLifecycleStatus.ACTIVE); 164 break; 165 case ACHIEVED: 166 tgt.setValue(org.hl7.fhir.r5.model.Goal.GoalLifecycleStatus.COMPLETED); 167 break; 168 case ONHOLD: 169 tgt.setValue(org.hl7.fhir.r5.model.Goal.GoalLifecycleStatus.ONHOLD); 170 break; 171 case CANCELLED: 172 tgt.setValue(org.hl7.fhir.r5.model.Goal.GoalLifecycleStatus.CANCELLED); 173 break; 174 case ENTEREDINERROR: 175 tgt.setValue(org.hl7.fhir.r5.model.Goal.GoalLifecycleStatus.ENTEREDINERROR); 176 break; 177 case REJECTED: 178 tgt.setValue(org.hl7.fhir.r5.model.Goal.GoalLifecycleStatus.REJECTED); 179 break; 180 default: 181 tgt.setValue(org.hl7.fhir.r5.model.Goal.GoalLifecycleStatus.NULL); 182 break; 183 } 184 } 185 return tgt; 186 } 187 188 public static org.hl7.fhir.r5.model.Goal.GoalTargetComponent convertGoalTargetComponent(org.hl7.fhir.dstu3.model.Goal.GoalTargetComponent src) throws FHIRException { 189 if (src == null) 190 return null; 191 org.hl7.fhir.r5.model.Goal.GoalTargetComponent tgt = new org.hl7.fhir.r5.model.Goal.GoalTargetComponent(); 192 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 193 if (src.hasMeasure()) 194 tgt.setMeasure(CodeableConcept30_50.convertCodeableConcept(src.getMeasure())); 195 if (src.hasDetail()) 196 tgt.setDetail(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getDetail())); 197 if (src.hasDue()) 198 tgt.setDue(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getDue())); 199 return tgt; 200 } 201 202 public static org.hl7.fhir.dstu3.model.Goal.GoalTargetComponent convertGoalTargetComponent(org.hl7.fhir.r5.model.Goal.GoalTargetComponent src) throws FHIRException { 203 if (src == null) 204 return null; 205 org.hl7.fhir.dstu3.model.Goal.GoalTargetComponent tgt = new org.hl7.fhir.dstu3.model.Goal.GoalTargetComponent(); 206 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 207 if (src.hasMeasure()) 208 tgt.setMeasure(CodeableConcept30_50.convertCodeableConcept(src.getMeasure())); 209 if (src.hasDetail()) 210 tgt.setDetail(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getDetail())); 211 if (src.hasDue()) 212 tgt.setDue(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getDue())); 213 return tgt; 214 } 215}