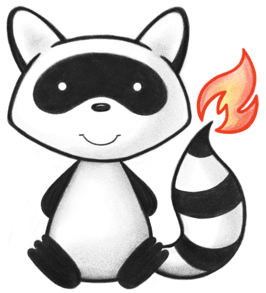
001package org.hl7.fhir.convertors.conv30_50.resources30_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_50; 004import org.hl7.fhir.convertors.conv30_50.datatypes30_50.ContactDetail30_50; 005import org.hl7.fhir.convertors.conv30_50.datatypes30_50.UsageContext30_50; 006import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.CodeableConcept30_50; 007import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Boolean30_50; 008import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.DateTime30_50; 009import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.MarkDown30_50; 010import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.String30_50; 011import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Uri30_50; 012import org.hl7.fhir.dstu3.model.Enumeration; 013import org.hl7.fhir.dstu3.model.GraphDefinition; 014import org.hl7.fhir.exceptions.FHIRException; 015import org.hl7.fhir.r5.model.Enumerations; 016 017public class GraphDefinition30_50 { 018 019 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.GraphDefinition.CompartmentCode> convertCompartmentCode(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.CompartmentType> src) throws FHIRException { 020 if (src == null || src.isEmpty()) 021 return null; 022 Enumeration<GraphDefinition.CompartmentCode> tgt = new Enumeration<>(new GraphDefinition.CompartmentCodeEnumFactory()); 023 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 024 if (src.getValue() == null) { 025 tgt.setValue(null); 026 } else { 027 switch (src.getValue()) { 028 case PATIENT: 029 tgt.setValue(GraphDefinition.CompartmentCode.PATIENT); 030 break; 031 case ENCOUNTER: 032 tgt.setValue(GraphDefinition.CompartmentCode.ENCOUNTER); 033 break; 034 case RELATEDPERSON: 035 tgt.setValue(GraphDefinition.CompartmentCode.RELATEDPERSON); 036 break; 037 case PRACTITIONER: 038 tgt.setValue(GraphDefinition.CompartmentCode.PRACTITIONER); 039 break; 040 case DEVICE: 041 tgt.setValue(GraphDefinition.CompartmentCode.DEVICE); 042 break; 043 default: 044 tgt.setValue(GraphDefinition.CompartmentCode.NULL); 045 break; 046 } 047 } 048 return tgt; 049 } 050 051 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.CompartmentType> convertCompartmentCode(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.GraphDefinition.CompartmentCode> src) throws FHIRException { 052 if (src == null || src.isEmpty()) 053 return null; 054 org.hl7.fhir.r5.model.Enumeration<Enumerations.CompartmentType> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new Enumerations.CompartmentTypeEnumFactory()); 055 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 056 if (src.getValue() == null) { 057 tgt.setValue(null); 058 } else { 059 switch (src.getValue()) { 060 case PATIENT: 061 tgt.setValue(Enumerations.CompartmentType.PATIENT); 062 break; 063 case ENCOUNTER: 064 tgt.setValue(Enumerations.CompartmentType.ENCOUNTER); 065 break; 066 case RELATEDPERSON: 067 tgt.setValue(Enumerations.CompartmentType.RELATEDPERSON); 068 break; 069 case PRACTITIONER: 070 tgt.setValue(Enumerations.CompartmentType.PRACTITIONER); 071 break; 072 case DEVICE: 073 tgt.setValue(Enumerations.CompartmentType.DEVICE); 074 break; 075 default: 076 tgt.setValue(Enumerations.CompartmentType.NULL); 077 break; 078 } 079 } 080 return tgt; 081 } 082 083 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.GraphDefinition.GraphCompartmentRule> convertGraphCompartmentRule(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.GraphDefinition.GraphCompartmentRule> src) throws FHIRException { 084 if (src == null || src.isEmpty()) 085 return null; 086 Enumeration<GraphDefinition.GraphCompartmentRule> tgt = new Enumeration<>(new GraphDefinition.GraphCompartmentRuleEnumFactory()); 087 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 088 if (src.getValue() == null) { 089 tgt.setValue(null); 090 } else { 091 switch (src.getValue()) { 092 case IDENTICAL: 093 tgt.setValue(GraphDefinition.GraphCompartmentRule.IDENTICAL); 094 break; 095 case MATCHING: 096 tgt.setValue(GraphDefinition.GraphCompartmentRule.MATCHING); 097 break; 098 case DIFFERENT: 099 tgt.setValue(GraphDefinition.GraphCompartmentRule.DIFFERENT); 100 break; 101 case CUSTOM: 102 tgt.setValue(GraphDefinition.GraphCompartmentRule.CUSTOM); 103 break; 104 default: 105 tgt.setValue(GraphDefinition.GraphCompartmentRule.NULL); 106 break; 107 } 108 } 109 return tgt; 110 } 111 112 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.GraphDefinition.GraphCompartmentRule> convertGraphCompartmentRule(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.GraphDefinition.GraphCompartmentRule> src) throws FHIRException { 113 if (src == null || src.isEmpty()) 114 return null; 115 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.GraphDefinition.GraphCompartmentRule> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.GraphDefinition.GraphCompartmentRuleEnumFactory()); 116 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 117 if (src.getValue() == null) { 118 tgt.setValue(null); 119 } else { 120 switch (src.getValue()) { 121 case IDENTICAL: 122 tgt.setValue(org.hl7.fhir.r5.model.GraphDefinition.GraphCompartmentRule.IDENTICAL); 123 break; 124 case MATCHING: 125 tgt.setValue(org.hl7.fhir.r5.model.GraphDefinition.GraphCompartmentRule.MATCHING); 126 break; 127 case DIFFERENT: 128 tgt.setValue(org.hl7.fhir.r5.model.GraphDefinition.GraphCompartmentRule.DIFFERENT); 129 break; 130 case CUSTOM: 131 tgt.setValue(org.hl7.fhir.r5.model.GraphDefinition.GraphCompartmentRule.CUSTOM); 132 break; 133 default: 134 tgt.setValue(org.hl7.fhir.r5.model.GraphDefinition.GraphCompartmentRule.NULL); 135 break; 136 } 137 } 138 return tgt; 139 } 140 141 public static org.hl7.fhir.r5.model.GraphDefinition convertGraphDefinition(org.hl7.fhir.dstu3.model.GraphDefinition src) throws FHIRException { 142 if (src == null) 143 return null; 144 org.hl7.fhir.r5.model.GraphDefinition tgt = new org.hl7.fhir.r5.model.GraphDefinition(); 145 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 146 if (src.hasUrl()) 147 tgt.setUrlElement(Uri30_50.convertUri(src.getUrlElement())); 148 if (src.hasVersion()) 149 tgt.setVersionElement(String30_50.convertString(src.getVersionElement())); 150 if (src.hasName()) 151 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 152 if (src.hasStatus()) 153 tgt.setStatusElement(Enumerations30_50.convertPublicationStatus(src.getStatusElement())); 154 if (src.hasExperimental()) 155 tgt.setExperimentalElement(Boolean30_50.convertBoolean(src.getExperimentalElement())); 156 if (src.hasDate()) 157 tgt.setDateElement(DateTime30_50.convertDateTime(src.getDateElement())); 158 if (src.hasPublisher()) 159 tgt.setPublisherElement(String30_50.convertString(src.getPublisherElement())); 160 for (org.hl7.fhir.dstu3.model.ContactDetail t : src.getContact()) 161 tgt.addContact(ContactDetail30_50.convertContactDetail(t)); 162 if (src.hasDescription()) 163 tgt.setDescriptionElement(MarkDown30_50.convertMarkdown(src.getDescriptionElement())); 164 for (org.hl7.fhir.dstu3.model.UsageContext t : src.getUseContext()) 165 tgt.addUseContext(UsageContext30_50.convertUsageContext(t)); 166 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getJurisdiction()) 167 tgt.addJurisdiction(CodeableConcept30_50.convertCodeableConcept(t)); 168 if (src.hasPurpose()) 169 tgt.setPurposeElement(MarkDown30_50.convertMarkdown(src.getPurposeElement())); 170// if (src.hasStart()) 171// tgt.setStartElement(Code30_50.convertCode(src.getStartElement())); 172// if (src.hasProfile()) 173// tgt.setProfile(src.getProfile()); 174// for (org.hl7.fhir.dstu3.model.GraphDefinition.GraphDefinitionLinkComponent t : src.getLink()) 175// tgt.addLink(convertGraphDefinitionLinkComponent(t)); 176 return tgt; 177 } 178 179 public static org.hl7.fhir.dstu3.model.GraphDefinition convertGraphDefinition(org.hl7.fhir.r5.model.GraphDefinition src) throws FHIRException { 180 if (src == null) 181 return null; 182 org.hl7.fhir.dstu3.model.GraphDefinition tgt = new org.hl7.fhir.dstu3.model.GraphDefinition(); 183 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 184 if (src.hasUrl()) 185 tgt.setUrlElement(Uri30_50.convertUri(src.getUrlElement())); 186 if (src.hasVersion()) 187 tgt.setVersionElement(String30_50.convertString(src.getVersionElement())); 188 if (src.hasName()) 189 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 190 if (src.hasStatus()) 191 tgt.setStatusElement(Enumerations30_50.convertPublicationStatus(src.getStatusElement())); 192 if (src.hasExperimental()) 193 tgt.setExperimentalElement(Boolean30_50.convertBoolean(src.getExperimentalElement())); 194 if (src.hasDate()) 195 tgt.setDateElement(DateTime30_50.convertDateTime(src.getDateElement())); 196 if (src.hasPublisher()) 197 tgt.setPublisherElement(String30_50.convertString(src.getPublisherElement())); 198 for (org.hl7.fhir.r5.model.ContactDetail t : src.getContact()) 199 tgt.addContact(ContactDetail30_50.convertContactDetail(t)); 200 if (src.hasDescription()) 201 tgt.setDescriptionElement(MarkDown30_50.convertMarkdown(src.getDescriptionElement())); 202 for (org.hl7.fhir.r5.model.UsageContext t : src.getUseContext()) 203 tgt.addUseContext(UsageContext30_50.convertUsageContext(t)); 204 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getJurisdiction()) 205 tgt.addJurisdiction(CodeableConcept30_50.convertCodeableConcept(t)); 206 if (src.hasPurpose()) 207 tgt.setPurposeElement(MarkDown30_50.convertMarkdown(src.getPurposeElement())); 208// if (src.hasStart()) 209// tgt.setStartElement(Code30_50.convertCode(src.getStartElement())); 210// if (src.hasProfile()) 211// tgt.setProfile(src.getProfile()); 212// for (org.hl7.fhir.r5.model.GraphDefinition.GraphDefinitionLinkComponent t : src.getLink()) 213// tgt.addLink(convertGraphDefinitionLinkComponent(t)); 214 return tgt; 215 } 216 217// public static org.hl7.fhir.r5.model.GraphDefinition.GraphDefinitionLinkComponent convertGraphDefinitionLinkComponent(org.hl7.fhir.dstu3.model.GraphDefinition.GraphDefinitionLinkComponent src) throws FHIRException { 218// if (src == null) 219// return null; 220// org.hl7.fhir.r5.model.GraphDefinition.GraphDefinitionLinkComponent tgt = new org.hl7.fhir.r5.model.GraphDefinition.GraphDefinitionLinkComponent(); 221// ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 222// if (src.hasPath()) 223// tgt.setPathElement(String30_50.convertString(src.getPathElement())); 224// if (src.hasSliceName()) 225// tgt.setSliceNameElement(String30_50.convertString(src.getSliceNameElement())); 226// if (src.hasMin()) 227// tgt.setMinElement(Integer30_50.convertInteger(src.getMinElement())); 228// if (src.hasMax()) 229// tgt.setMaxElement(String30_50.convertString(src.getMaxElement())); 230// if (src.hasDescription()) 231// tgt.setDescriptionElement(String30_50.convertString(src.getDescriptionElement())); 232// for (org.hl7.fhir.dstu3.model.GraphDefinition.GraphDefinitionLinkTargetComponent t : src.getTarget()) 233// tgt.addTarget(convertGraphDefinitionLinkTargetComponent(t)); 234// return tgt; 235// } 236// 237// public static org.hl7.fhir.dstu3.model.GraphDefinition.GraphDefinitionLinkComponent convertGraphDefinitionLinkComponent(org.hl7.fhir.r5.model.GraphDefinition.GraphDefinitionLinkComponent src) throws FHIRException { 238// if (src == null) 239// return null; 240// org.hl7.fhir.dstu3.model.GraphDefinition.GraphDefinitionLinkComponent tgt = new org.hl7.fhir.dstu3.model.GraphDefinition.GraphDefinitionLinkComponent(); 241// ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 242// if (src.hasPath()) 243// tgt.setPathElement(String30_50.convertString(src.getPathElement())); 244// if (src.hasSliceName()) 245// tgt.setSliceNameElement(String30_50.convertString(src.getSliceNameElement())); 246// if (src.hasMin()) 247// tgt.setMinElement(Integer30_50.convertInteger(src.getMinElement())); 248// if (src.hasMax()) 249// tgt.setMaxElement(String30_50.convertString(src.getMaxElement())); 250// if (src.hasDescription()) 251// tgt.setDescriptionElement(String30_50.convertString(src.getDescriptionElement())); 252// for (org.hl7.fhir.r5.model.GraphDefinition.GraphDefinitionLinkTargetComponent t : src.getTarget()) 253// tgt.addTarget(convertGraphDefinitionLinkTargetComponent(t)); 254// return tgt; 255// } 256// 257// public static org.hl7.fhir.r5.model.GraphDefinition.GraphDefinitionLinkTargetCompartmentComponent convertGraphDefinitionLinkTargetCompartmentComponent(org.hl7.fhir.dstu3.model.GraphDefinition.GraphDefinitionLinkTargetCompartmentComponent src) throws FHIRException { 258// if (src == null) 259// return null; 260// org.hl7.fhir.r5.model.GraphDefinition.GraphDefinitionLinkTargetCompartmentComponent tgt = new org.hl7.fhir.r5.model.GraphDefinition.GraphDefinitionLinkTargetCompartmentComponent(); 261// ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 262// if (src.hasCode()) 263// tgt.setCodeElement(convertCompartmentCode(src.getCodeElement())); 264// if (src.hasRule()) 265// tgt.setRuleElement(convertGraphCompartmentRule(src.getRuleElement())); 266// if (src.hasExpression()) 267// tgt.setExpressionElement(String30_50.convertString(src.getExpressionElement())); 268// if (src.hasDescription()) 269// tgt.setDescriptionElement(String30_50.convertString(src.getDescriptionElement())); 270// return tgt; 271// } 272// 273// public static org.hl7.fhir.dstu3.model.GraphDefinition.GraphDefinitionLinkTargetCompartmentComponent convertGraphDefinitionLinkTargetCompartmentComponent(org.hl7.fhir.r5.model.GraphDefinition.GraphDefinitionLinkTargetCompartmentComponent src) throws FHIRException { 274// if (src == null) 275// return null; 276// org.hl7.fhir.dstu3.model.GraphDefinition.GraphDefinitionLinkTargetCompartmentComponent tgt = new org.hl7.fhir.dstu3.model.GraphDefinition.GraphDefinitionLinkTargetCompartmentComponent(); 277// ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 278// if (src.hasCode()) 279// tgt.setCodeElement(convertCompartmentCode(src.getCodeElement())); 280// if (src.hasRule()) 281// tgt.setRuleElement(convertGraphCompartmentRule(src.getRuleElement())); 282// if (src.hasExpression()) 283// tgt.setExpressionElement(String30_50.convertString(src.getExpressionElement())); 284// if (src.hasDescription()) 285// tgt.setDescriptionElement(String30_50.convertString(src.getDescriptionElement())); 286// return tgt; 287// } 288// 289// public static org.hl7.fhir.dstu3.model.GraphDefinition.GraphDefinitionLinkTargetComponent convertGraphDefinitionLinkTargetComponent(org.hl7.fhir.r5.model.GraphDefinition.GraphDefinitionLinkTargetComponent src) throws FHIRException { 290// if (src == null) 291// return null; 292// org.hl7.fhir.dstu3.model.GraphDefinition.GraphDefinitionLinkTargetComponent tgt = new org.hl7.fhir.dstu3.model.GraphDefinition.GraphDefinitionLinkTargetComponent(); 293// ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 294// if (src.hasType()) 295// tgt.setTypeElement(Code30_50.convertResourceEnum(src.getTypeElement())); 296// if (src.hasProfile()) 297// tgt.setProfile(src.getProfile()); 298// for (org.hl7.fhir.r5.model.GraphDefinition.GraphDefinitionLinkTargetCompartmentComponent t : src.getCompartment()) 299// tgt.addCompartment(convertGraphDefinitionLinkTargetCompartmentComponent(t)); 300// for (org.hl7.fhir.r5.model.GraphDefinition.GraphDefinitionLinkComponent t : src.getLink()) 301// tgt.addLink(convertGraphDefinitionLinkComponent(t)); 302// return tgt; 303// } 304// 305// public static org.hl7.fhir.r5.model.GraphDefinition.GraphDefinitionLinkTargetComponent convertGraphDefinitionLinkTargetComponent(org.hl7.fhir.dstu3.model.GraphDefinition.GraphDefinitionLinkTargetComponent src) throws FHIRException { 306// if (src == null) 307// return null; 308// org.hl7.fhir.r5.model.GraphDefinition.GraphDefinitionLinkTargetComponent tgt = new org.hl7.fhir.r5.model.GraphDefinition.GraphDefinitionLinkTargetComponent(); 309// ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 310// if (src.hasType()) 311// tgt.setTypeElement(Code30_50.convertResourceEnum(src.getTypeElement())); 312// if (src.hasProfile()) 313// tgt.setProfile(src.getProfile()); 314// for (org.hl7.fhir.dstu3.model.GraphDefinition.GraphDefinitionLinkTargetCompartmentComponent t : src.getCompartment()) 315// tgt.addCompartment(convertGraphDefinitionLinkTargetCompartmentComponent(t)); 316// for (org.hl7.fhir.dstu3.model.GraphDefinition.GraphDefinitionLinkComponent t : src.getLink()) 317// tgt.addLink(convertGraphDefinitionLinkComponent(t)); 318// return tgt; 319// } 320}