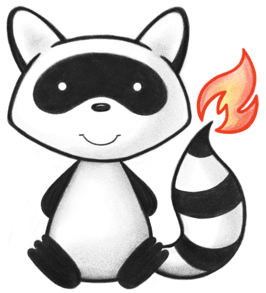
001package org.hl7.fhir.convertors.conv30_50.resources30_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_50; 004import org.hl7.fhir.convertors.conv30_50.datatypes30_50.Reference30_50; 005import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Attachment30_50; 006import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.CodeableConcept30_50; 007import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.ContactPoint30_50; 008import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Identifier30_50; 009import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Boolean30_50; 010import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.String30_50; 011import org.hl7.fhir.dstu3.model.Enumeration; 012import org.hl7.fhir.dstu3.model.HealthcareService; 013import org.hl7.fhir.exceptions.FHIRException; 014import org.hl7.fhir.r5.model.CodeableConcept; 015import org.hl7.fhir.r5.model.Enumerations; 016import org.hl7.fhir.r5.model.ExtendedContactDetail; 017import org.hl7.fhir.r5.model.HealthcareService.HealthcareServiceEligibilityComponent; 018 019public class HealthcareService30_50 { 020 021 public static org.hl7.fhir.r5.model.HealthcareService convertHealthcareService(org.hl7.fhir.dstu3.model.HealthcareService src) throws FHIRException { 022 if (src == null) 023 return null; 024 org.hl7.fhir.r5.model.HealthcareService tgt = new org.hl7.fhir.r5.model.HealthcareService(); 025 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 026 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 027 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 028 if (src.hasActive()) 029 tgt.setActiveElement(Boolean30_50.convertBoolean(src.getActiveElement())); 030 if (src.hasProvidedBy()) 031 tgt.setProvidedBy(Reference30_50.convertReference(src.getProvidedBy())); 032 if (src.hasCategory()) 033 tgt.addCategory(CodeableConcept30_50.convertCodeableConcept(src.getCategory())); 034 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getType()) 035 tgt.addType(CodeableConcept30_50.convertCodeableConcept(t)); 036 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getSpecialty()) 037 tgt.addSpecialty(CodeableConcept30_50.convertCodeableConcept(t)); 038 for (org.hl7.fhir.dstu3.model.Reference t : src.getLocation()) tgt.addLocation(Reference30_50.convertReference(t)); 039 if (src.hasName()) 040 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 041 if (src.hasComment()) 042 tgt.setCommentElement(String30_50.convertStringToMarkdown(src.getCommentElement())); 043 if (src.hasExtraDetails()) 044 tgt.setExtraDetails(src.getExtraDetails()); 045 if (src.hasPhoto()) 046 tgt.setPhoto(Attachment30_50.convertAttachment(src.getPhoto())); 047 for (org.hl7.fhir.dstu3.model.ContactPoint t : src.getTelecom()) 048 tgt.getContactFirstRep().addTelecom(ContactPoint30_50.convertContactPoint(t)); 049 for (org.hl7.fhir.dstu3.model.Reference t : src.getCoverageArea()) 050 tgt.addCoverageArea(Reference30_50.convertReference(t)); 051 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getServiceProvisionCode()) 052 tgt.addServiceProvisionCode(CodeableConcept30_50.convertCodeableConcept(t)); 053 if (src.hasEligibility() || src.hasEligibilityNote()) { 054 HealthcareServiceEligibilityComponent t = tgt.addEligibility(); 055 t.setCode(CodeableConcept30_50.convertCodeableConcept(src.getEligibility())); 056 if (src.hasEligibilityNote()) 057 t.setComment(src.getEligibilityNote()); 058 } 059 for (org.hl7.fhir.dstu3.model.StringType t : src.getProgramName()) tgt.addProgram().setText(t.getValue()); 060 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getCharacteristic()) 061 tgt.addCharacteristic(CodeableConcept30_50.convertCodeableConcept(t)); 062 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getReferralMethod()) 063 tgt.addReferralMethod(CodeableConcept30_50.convertCodeableConcept(t)); 064 if (src.hasAppointmentRequired()) 065 tgt.setAppointmentRequiredElement(Boolean30_50.convertBoolean(src.getAppointmentRequiredElement())); 066// for (org.hl7.fhir.dstu3.model.HealthcareService.HealthcareServiceAvailableTimeComponent t : src.getAvailableTime()) 067// tgt.addAvailableTime(convertHealthcareServiceAvailableTimeComponent(t)); 068// for (org.hl7.fhir.dstu3.model.HealthcareService.HealthcareServiceNotAvailableComponent t : src.getNotAvailable()) 069// tgt.addNotAvailable(convertHealthcareServiceNotAvailableComponent(t)); 070// if (src.hasAvailabilityExceptions()) 071// tgt.setAvailabilityExceptionsElement(String30_50.convertString(src.getAvailabilityExceptionsElement())); 072 for (org.hl7.fhir.dstu3.model.Reference t : src.getEndpoint()) tgt.addEndpoint(Reference30_50.convertReference(t)); 073 return tgt; 074 } 075 076 public static org.hl7.fhir.dstu3.model.HealthcareService convertHealthcareService(org.hl7.fhir.r5.model.HealthcareService src) throws FHIRException { 077 if (src == null) 078 return null; 079 org.hl7.fhir.dstu3.model.HealthcareService tgt = new org.hl7.fhir.dstu3.model.HealthcareService(); 080 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 081 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 082 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 083 if (src.hasActive()) 084 tgt.setActiveElement(Boolean30_50.convertBoolean(src.getActiveElement())); 085 if (src.hasProvidedBy()) 086 tgt.setProvidedBy(Reference30_50.convertReference(src.getProvidedBy())); 087 if (src.hasCategory()) 088 tgt.setCategory(CodeableConcept30_50.convertCodeableConcept(src.getCategoryFirstRep())); 089 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getType()) 090 tgt.addType(CodeableConcept30_50.convertCodeableConcept(t)); 091 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getSpecialty()) 092 tgt.addSpecialty(CodeableConcept30_50.convertCodeableConcept(t)); 093 for (org.hl7.fhir.r5.model.Reference t : src.getLocation()) tgt.addLocation(Reference30_50.convertReference(t)); 094 if (src.hasName()) 095 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 096 if (src.hasComment()) 097 tgt.setCommentElement(String30_50.convertString(src.getCommentElement())); 098 if (src.hasExtraDetails()) 099 tgt.setExtraDetails(src.getExtraDetails()); 100 if (src.hasPhoto()) 101 tgt.setPhoto(Attachment30_50.convertAttachment(src.getPhoto())); 102 for (ExtendedContactDetail t1 : src.getContact()) 103 for (org.hl7.fhir.r5.model.ContactPoint t : t1.getTelecom()) 104 tgt.addTelecom(ContactPoint30_50.convertContactPoint(t)); 105 for (org.hl7.fhir.r5.model.Reference t : src.getCoverageArea()) 106 tgt.addCoverageArea(Reference30_50.convertReference(t)); 107 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getServiceProvisionCode()) 108 tgt.addServiceProvisionCode(CodeableConcept30_50.convertCodeableConcept(t)); 109 if (src.hasEligibility()) { 110 tgt.setEligibility(CodeableConcept30_50.convertCodeableConcept(src.getEligibilityFirstRep().getCode())); 111 if (src.getEligibilityFirstRep().hasComment()) 112 tgt.setEligibilityNoteElement(String30_50.convertString(src.getCommentElement())); 113 } 114 for (CodeableConcept t : src.getProgram()) tgt.addProgramName(t.getText()); 115 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getCharacteristic()) 116 tgt.addCharacteristic(CodeableConcept30_50.convertCodeableConcept(t)); 117 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getReferralMethod()) 118 tgt.addReferralMethod(CodeableConcept30_50.convertCodeableConcept(t)); 119 if (src.hasAppointmentRequired()) 120 tgt.setAppointmentRequiredElement(Boolean30_50.convertBoolean(src.getAppointmentRequiredElement())); 121// for (org.hl7.fhir.r5.model.HealthcareService.HealthcareServiceAvailableTimeComponent t : src.getAvailableTime()) 122// tgt.addAvailableTime(convertHealthcareServiceAvailableTimeComponent(t)); 123// for (org.hl7.fhir.r5.model.HealthcareService.HealthcareServiceNotAvailableComponent t : src.getNotAvailable()) 124// tgt.addNotAvailable(convertHealthcareServiceNotAvailableComponent(t)); 125// if (src.hasAvailabilityExceptions()) 126// tgt.setAvailabilityExceptionsElement(String30_50.convertString(src.getAvailabilityExceptionsElement())); 127 for (org.hl7.fhir.r5.model.Reference t : src.getEndpoint()) tgt.addEndpoint(Reference30_50.convertReference(t)); 128 return tgt; 129 } 130 131// public static org.hl7.fhir.r5.model.HealthcareService.HealthcareServiceAvailableTimeComponent convertHealthcareServiceAvailableTimeComponent(org.hl7.fhir.dstu3.model.HealthcareService.HealthcareServiceAvailableTimeComponent src) throws FHIRException { 132// if (src == null) 133// return null; 134// org.hl7.fhir.r5.model.HealthcareService.HealthcareServiceAvailableTimeComponent tgt = new org.hl7.fhir.r5.model.HealthcareService.HealthcareServiceAvailableTimeComponent(); 135// ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 136// tgt.setDaysOfWeek(src.getDaysOfWeek().stream() 137// .map(HealthcareService30_50::convertDaysOfWeek) 138// .collect(Collectors.toList())); 139// if (src.hasAllDay()) 140// tgt.setAllDayElement(Boolean30_50.convertBoolean(src.getAllDayElement())); 141// if (src.hasAvailableStartTime()) 142// tgt.setAvailableStartTimeElement(Time30_50.convertTime(src.getAvailableStartTimeElement())); 143// if (src.hasAvailableEndTime()) 144// tgt.setAvailableEndTimeElement(Time30_50.convertTime(src.getAvailableEndTimeElement())); 145// return tgt; 146// } 147// 148// public static org.hl7.fhir.dstu3.model.HealthcareService.HealthcareServiceAvailableTimeComponent convertHealthcareServiceAvailableTimeComponent(org.hl7.fhir.r5.model.HealthcareService.HealthcareServiceAvailableTimeComponent src) throws FHIRException { 149// if (src == null) 150// return null; 151// org.hl7.fhir.dstu3.model.HealthcareService.HealthcareServiceAvailableTimeComponent tgt = new org.hl7.fhir.dstu3.model.HealthcareService.HealthcareServiceAvailableTimeComponent(); 152// ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 153// tgt.setDaysOfWeek(src.getDaysOfWeek().stream() 154// .map(HealthcareService30_50::convertDaysOfWeek) 155// .collect(Collectors.toList())); 156// if (src.hasAllDay()) 157// tgt.setAllDayElement(Boolean30_50.convertBoolean(src.getAllDayElement())); 158// if (src.hasAvailableStartTime()) 159// tgt.setAvailableStartTimeElement(Time30_50.convertTime(src.getAvailableStartTimeElement())); 160// if (src.hasAvailableEndTime()) 161// tgt.setAvailableEndTimeElement(Time30_50.convertTime(src.getAvailableEndTimeElement())); 162// return tgt; 163// } 164 165 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.HealthcareService.DaysOfWeek> convertDaysOfWeek(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.DaysOfWeek> src) throws FHIRException { 166 if (src == null || src.isEmpty()) 167 return null; 168 Enumeration<HealthcareService.DaysOfWeek> tgt = new Enumeration<>(new HealthcareService.DaysOfWeekEnumFactory()); 169 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 170 if (src.getValue() == null) { 171 tgt.setValue(null); 172 } else { 173 switch (src.getValue()) { 174 case MON: 175 tgt.setValue(HealthcareService.DaysOfWeek.MON); 176 break; 177 case TUE: 178 tgt.setValue(HealthcareService.DaysOfWeek.TUE); 179 break; 180 case WED: 181 tgt.setValue(HealthcareService.DaysOfWeek.WED); 182 break; 183 case THU: 184 tgt.setValue(HealthcareService.DaysOfWeek.THU); 185 break; 186 case FRI: 187 tgt.setValue(HealthcareService.DaysOfWeek.FRI); 188 break; 189 case SAT: 190 tgt.setValue(HealthcareService.DaysOfWeek.SAT); 191 break; 192 case SUN: 193 tgt.setValue(HealthcareService.DaysOfWeek.SUN); 194 break; 195 default: 196 tgt.setValue(HealthcareService.DaysOfWeek.NULL); 197 break; 198 } 199 } 200 return tgt; 201 } 202 203 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.DaysOfWeek> convertDaysOfWeek(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.HealthcareService.DaysOfWeek> src) throws FHIRException { 204 if (src == null || src.isEmpty()) 205 return null; 206 org.hl7.fhir.r5.model.Enumeration<Enumerations.DaysOfWeek> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new Enumerations.DaysOfWeekEnumFactory()); 207 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 208 if (src.getValue() == null) { 209 tgt.setValue(null); 210 } else { 211 switch (src.getValue()) { 212 case MON: 213 tgt.setValue(Enumerations.DaysOfWeek.MON); 214 break; 215 case TUE: 216 tgt.setValue(Enumerations.DaysOfWeek.TUE); 217 break; 218 case WED: 219 tgt.setValue(Enumerations.DaysOfWeek.WED); 220 break; 221 case THU: 222 tgt.setValue(Enumerations.DaysOfWeek.THU); 223 break; 224 case FRI: 225 tgt.setValue(Enumerations.DaysOfWeek.FRI); 226 break; 227 case SAT: 228 tgt.setValue(Enumerations.DaysOfWeek.SAT); 229 break; 230 case SUN: 231 tgt.setValue(Enumerations.DaysOfWeek.SUN); 232 break; 233 default: 234 tgt.setValue(Enumerations.DaysOfWeek.NULL); 235 break; 236 } 237 } 238 return tgt; 239 } 240// 241// public static org.hl7.fhir.r5.model.HealthcareService.HealthcareServiceNotAvailableComponent convertHealthcareServiceNotAvailableComponent(org.hl7.fhir.dstu3.model.HealthcareService.HealthcareServiceNotAvailableComponent src) throws FHIRException { 242// if (src == null) 243// return null; 244// org.hl7.fhir.r5.model.HealthcareService.HealthcareServiceNotAvailableComponent tgt = new org.hl7.fhir.r5.model.HealthcareService.HealthcareServiceNotAvailableComponent(); 245// ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 246// if (src.hasDescription()) 247// tgt.setDescriptionElement(String30_50.convertString(src.getDescriptionElement())); 248// if (src.hasDuring()) 249// tgt.setDuring(Period30_50.convertPeriod(src.getDuring())); 250// return tgt; 251// } 252// 253// public static org.hl7.fhir.dstu3.model.HealthcareService.HealthcareServiceNotAvailableComponent convertHealthcareServiceNotAvailableComponent(org.hl7.fhir.r5.model.HealthcareService.HealthcareServiceNotAvailableComponent src) throws FHIRException { 254// if (src == null) 255// return null; 256// org.hl7.fhir.dstu3.model.HealthcareService.HealthcareServiceNotAvailableComponent tgt = new org.hl7.fhir.dstu3.model.HealthcareService.HealthcareServiceNotAvailableComponent(); 257// ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 258// if (src.hasDescription()) 259// tgt.setDescriptionElement(String30_50.convertString(src.getDescriptionElement())); 260// if (src.hasDuring()) 261// tgt.setDuring(Period30_50.convertPeriod(src.getDuring())); 262// return tgt; 263// } 264}