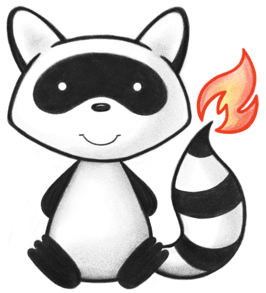
001package org.hl7.fhir.convertors.conv30_50.resources30_50; 002 003import java.util.List; 004 005import org.hl7.fhir.convertors.VersionConvertorConstants; 006import org.hl7.fhir.convertors.context.ConversionContext30_50; 007import org.hl7.fhir.convertors.conv30_50.datatypes30_50.ContactDetail30_50; 008import org.hl7.fhir.convertors.conv30_50.datatypes30_50.Reference30_50; 009import org.hl7.fhir.convertors.conv30_50.datatypes30_50.UsageContext30_50; 010import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.CodeableConcept30_50; 011import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Boolean30_50; 012import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Code30_50; 013import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.DateTime30_50; 014import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.MarkDown30_50; 015import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.String30_50; 016import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Uri30_50; 017import org.hl7.fhir.dstu3.model.ImplementationGuide; 018import org.hl7.fhir.exceptions.FHIRException; 019import org.hl7.fhir.r4.model.Extension; 020import org.hl7.fhir.r5.model.CanonicalType; 021import org.hl7.fhir.r5.model.Enumeration; 022import org.hl7.fhir.utilities.VersionUtilities; 023 024public class ImplementationGuide30_50 { 025 026 static final String EXT_IG_DEFINITION_RESOURCE_PROFILE = "http://hl7.org/fhir/5.0/StructureDefinition/extension-ImplementationGuide.definition.resource.profile"; 027 028 public static org.hl7.fhir.dstu3.model.ImplementationGuide convertImplementationGuide(org.hl7.fhir.r5.model.ImplementationGuide src) throws FHIRException { 029 if (src == null) 030 return null; 031 org.hl7.fhir.dstu3.model.ImplementationGuide tgt = new org.hl7.fhir.dstu3.model.ImplementationGuide(); 032 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 033 if (src.hasUrl()) 034 tgt.setUrlElement(Uri30_50.convertUri(src.getUrlElement())); 035 if (src.hasVersion()) 036 tgt.setVersionElement(String30_50.convertString(src.getVersionElement())); 037 if (src.hasName()) 038 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 039 if (src.hasStatus()) 040 tgt.setStatusElement(Enumerations30_50.convertPublicationStatus(src.getStatusElement())); 041 if (src.hasExperimental()) 042 tgt.setExperimentalElement(Boolean30_50.convertBoolean(src.getExperimentalElement())); 043 if (src.hasDate()) 044 tgt.setDateElement(DateTime30_50.convertDateTime(src.getDateElement())); 045 if (src.hasPublisher()) 046 tgt.setPublisherElement(String30_50.convertString(src.getPublisherElement())); 047 for (org.hl7.fhir.r5.model.ContactDetail t : src.getContact()) 048 tgt.addContact(ContactDetail30_50.convertContactDetail(t)); 049 if (src.hasDescription()) 050 tgt.setDescriptionElement(MarkDown30_50.convertMarkdown(src.getDescriptionElement())); 051 for (org.hl7.fhir.r5.model.UsageContext t : src.getUseContext()) 052 tgt.addUseContext(UsageContext30_50.convertUsageContext(t)); 053 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getJurisdiction()) 054 tgt.addJurisdiction(CodeableConcept30_50.convertCodeableConcept(t)); 055 if (src.hasCopyright()) 056 tgt.setCopyrightElement(MarkDown30_50.convertMarkdown(src.getCopyrightElement())); 057 if (src.hasFhirVersion()) 058 for (Enumeration<org.hl7.fhir.r5.model.Enumerations.FHIRVersion> v : src.getFhirVersion()) { 059 tgt.setFhirVersion(v.asStringValue()); 060 break; 061 } 062 for (org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideDependsOnComponent t : src.getDependsOn()) 063 tgt.addDependency(convertImplementationGuideDependencyComponent(t)); 064 for (org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideDefinitionGroupingComponent t : src.getDefinition().getGrouping()) 065 tgt.addPackage(convertImplementationGuidePackageComponent(t)); 066 for (org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideDefinitionResourceComponent t : src.getDefinition().getResource()) { 067 findPackage(tgt.getPackage(), t.getGroupingId()).addResource(convertImplementationGuidePackageResourceComponent(t)); 068 } 069 for (org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideGlobalComponent t : src.getGlobal()) 070 tgt.addGlobal(convertImplementationGuideGlobalComponent(t)); 071 if (src.getDefinition().hasPage()) 072 tgt.setPage(convertImplementationGuidePageComponent(src.getDefinition().getPage())); 073 return tgt; 074 } 075 076 public static org.hl7.fhir.r5.model.ImplementationGuide convertImplementationGuide(org.hl7.fhir.dstu3.model.ImplementationGuide src) throws FHIRException { 077 if (src == null) 078 return null; 079 org.hl7.fhir.r5.model.ImplementationGuide tgt = new org.hl7.fhir.r5.model.ImplementationGuide(); 080 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 081 if (src.hasUrl()) 082 tgt.setUrlElement(Uri30_50.convertUri(src.getUrlElement())); 083 if (src.hasVersion()) 084 tgt.setVersionElement(String30_50.convertString(src.getVersionElement())); 085 if (src.hasName()) 086 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 087 if (src.hasStatus()) 088 tgt.setStatusElement(Enumerations30_50.convertPublicationStatus(src.getStatusElement())); 089 if (src.hasExperimental()) 090 tgt.setExperimentalElement(Boolean30_50.convertBoolean(src.getExperimentalElement())); 091 if (src.hasDate()) 092 tgt.setDateElement(DateTime30_50.convertDateTime(src.getDateElement())); 093 if (src.hasPublisher()) 094 tgt.setPublisherElement(String30_50.convertString(src.getPublisherElement())); 095 for (org.hl7.fhir.dstu3.model.ContactDetail t : src.getContact()) 096 tgt.addContact(ContactDetail30_50.convertContactDetail(t)); 097 if (src.hasDescription()) 098 tgt.setDescriptionElement(MarkDown30_50.convertMarkdown(src.getDescriptionElement())); 099 for (org.hl7.fhir.dstu3.model.UsageContext t : src.getUseContext()) 100 tgt.addUseContext(UsageContext30_50.convertUsageContext(t)); 101 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getJurisdiction()) 102 tgt.addJurisdiction(CodeableConcept30_50.convertCodeableConcept(t)); 103 if (src.hasCopyright()) 104 tgt.setCopyrightElement(MarkDown30_50.convertMarkdown(src.getCopyrightElement())); 105 if (src.hasFhirVersion()) 106 tgt.addFhirVersion(org.hl7.fhir.r5.model.Enumerations.FHIRVersion.fromCode( 107 VersionUtilities.getCurrentVersion(src.getFhirVersion()))); 108 for (org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuideDependencyComponent t : src.getDependency()) 109 tgt.addDependsOn(convertImplementationGuideDependencyComponent(t)); 110 for (org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePackageComponent t : src.getPackage()) 111 tgt.getDefinition().addGrouping(convertImplementationGuidePackageComponent(tgt.getDefinition(), t)); 112 for (org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuideGlobalComponent t : src.getGlobal()) 113 tgt.addGlobal(convertImplementationGuideGlobalComponent(t)); 114 if (src.hasPage()) 115 tgt.getDefinition().setPage(convertImplementationGuidePageComponent(src.getPage())); 116 return tgt; 117 } 118 119 public static org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuideDependencyComponent convertImplementationGuideDependencyComponent(org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideDependsOnComponent src) throws FHIRException { 120 if (src == null) 121 return null; 122 org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuideDependencyComponent tgt = new org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuideDependencyComponent(); 123 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 124 tgt.setType(org.hl7.fhir.dstu3.model.ImplementationGuide.GuideDependencyType.REFERENCE); 125 if (src.hasUri()) 126 tgt.setUri(src.getUri()); 127 if (src.hasPackageId()) 128 tgt.addExtension(new org.hl7.fhir.dstu3.model.Extension(VersionConvertorConstants.EXT_IG_DEPENDSON_PACKAGE_EXTENSION, new org.hl7.fhir.dstu3.model.IdType(src.getPackageId()))); 129 if (src.hasVersion()) 130 tgt.addExtension(new org.hl7.fhir.dstu3.model.Extension(VersionConvertorConstants.EXT_IG_DEPENDSON_VERSION_EXTENSION, new org.hl7.fhir.dstu3.model.StringType(src.getVersion()))); 131 return tgt; 132 } 133 134 public static org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideDependsOnComponent convertImplementationGuideDependencyComponent(org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuideDependencyComponent src) throws FHIRException { 135 if (src == null) 136 return null; 137 org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideDependsOnComponent tgt = new org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideDependsOnComponent(); 138 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 139 if (src.hasUri()) 140 tgt.setUri(src.getUri()); 141 if (org.hl7.fhir.dstu3.utils.ToolingExtensions.hasExtension(src, VersionConvertorConstants.EXT_IG_DEPENDSON_PACKAGE_EXTENSION)) { 142 tgt.setPackageId(org.hl7.fhir.dstu3.utils.ToolingExtensions.readStringExtension(src, VersionConvertorConstants.EXT_IG_DEPENDSON_PACKAGE_EXTENSION)); 143 } 144 if (org.hl7.fhir.dstu3.utils.ToolingExtensions.hasExtension(src, VersionConvertorConstants.EXT_IG_DEPENDSON_VERSION_EXTENSION)) { 145 tgt.setVersion(org.hl7.fhir.dstu3.utils.ToolingExtensions.readStringExtension(src, VersionConvertorConstants.EXT_IG_DEPENDSON_VERSION_EXTENSION)); 146 } 147 return tgt; 148 } 149 150 public static org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideGlobalComponent convertImplementationGuideGlobalComponent(org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuideGlobalComponent src) throws FHIRException { 151 if (src == null) 152 return null; 153 org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideGlobalComponent tgt = new org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideGlobalComponent(); 154 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 155 if (src.hasType()) 156 tgt.setTypeElement(Code30_50.convertCode(src.getTypeElement())); 157 if (src.hasProfile()) 158 tgt.setProfileElement(Reference30_50.convertReferenceToCanonical(src.getProfile())); 159 return tgt; 160 } 161 162 public static org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuideGlobalComponent convertImplementationGuideGlobalComponent(org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideGlobalComponent src) throws FHIRException { 163 if (src == null) 164 return null; 165 org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuideGlobalComponent tgt = new org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuideGlobalComponent(); 166 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 167 if (src.hasType()) 168 tgt.setTypeElement(Code30_50.convertCode(src.getTypeElement())); 169 if (src.hasProfile()) 170 tgt.setProfile(Reference30_50.convertCanonicalToReference(src.getProfileElement())); 171 return tgt; 172 } 173 174 public static org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePackageComponent convertImplementationGuidePackageComponent(org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideDefinitionGroupingComponent src) throws FHIRException { 175 if (src == null) 176 return null; 177 org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePackageComponent tgt = new org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePackageComponent(); 178 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 179 tgt.setId(src.getId()); 180 if (src.hasName()) 181 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 182 if (src.hasDescription()) 183 tgt.setDescriptionElement(String30_50.convertString(src.getDescriptionElement())); 184 return tgt; 185 } 186 187 public static org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideDefinitionGroupingComponent convertImplementationGuidePackageComponent(org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideDefinitionComponent context, org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePackageComponent src) throws FHIRException { 188 if (src == null) 189 return null; 190 org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideDefinitionGroupingComponent tgt = new org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideDefinitionGroupingComponent(); 191 tgt.setId("p" + (context.getGrouping().size() + 1)); 192 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 193 if (src.hasName()) 194 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 195 if (src.hasDescription()) 196 tgt.setDescriptionElement(String30_50.convertStringToMarkdown(src.getDescriptionElement())); 197 for (org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePackageResourceComponent t : src.getResource()) { 198 org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideDefinitionResourceComponent tn = convertImplementationGuidePackageResourceComponent(t); 199 tn.setGroupingId(tgt.getId()); 200 context.addResource(tn); 201 } 202 return tgt; 203 } 204 205 public static org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePackageResourceComponent convertImplementationGuidePackageResourceComponent(org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideDefinitionResourceComponent src) throws FHIRException { 206 if (src == null) 207 return null; 208 org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePackageResourceComponent tgt = new org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePackageResourceComponent(); 209 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 210 if (src.hasProfile()) { 211 tgt.setExampleFor(Reference30_50.convertCanonicalToReference(src.getProfile().get(0))); 212 tgt.setExample(true); 213 if (src.getProfile().size() > 1) { 214 for (CanonicalType p: src.getProfile().subList(1, src.getProfile().size())) { 215 tgt.addExtension(EXT_IG_DEFINITION_RESOURCE_PROFILE, Uri30_50.convertUri(p)); 216 } 217 } 218 } else if (src.hasIsExample()) 219 tgt.setExample(src.getIsExample()); 220 else 221 tgt.setExample(false); 222 223 if (src.hasName()) 224 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 225 if (src.hasDescription()) 226 tgt.setDescriptionElement(String30_50.convertString(src.getDescriptionElement())); 227 if (src.hasReference()) 228 tgt.setSource(Reference30_50.convertReference(src.getReference())); 229 return tgt; 230 } 231 232 public static org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideDefinitionResourceComponent convertImplementationGuidePackageResourceComponent(org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePackageResourceComponent src) throws FHIRException { 233 if (src == null) 234 return null; 235 org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideDefinitionResourceComponent tgt = new org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideDefinitionResourceComponent(); 236 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 237 for (org.hl7.fhir.dstu3.model.Extension ext: src.getExtensionsByUrl(EXT_IG_DEFINITION_RESOURCE_PROFILE)) { 238 tgt.getProfile().add(Uri30_50.convertCanonical((org.hl7.fhir.dstu3.model.UriType)ext.getValue())); 239 } 240 if (src.hasExampleFor()) { 241 tgt.getProfile().add(Reference30_50.convertReferenceToCanonical(src.getExampleFor())); 242 } else if (src.hasExample()) 243 tgt.setIsExampleElement(new org.hl7.fhir.r5.model.BooleanType(src.getExample())); 244 if (src.hasName()) 245 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 246 if (src.hasDescription()) 247 tgt.setDescriptionElement(String30_50.convertStringToMarkdown(src.getDescriptionElement())); 248 if (src.hasSourceReference()) 249 tgt.setReference(Reference30_50.convertReference(src.getSourceReference())); 250 else if (src.hasSourceUriType()) 251 tgt.setReference(new org.hl7.fhir.r5.model.Reference(src.getSourceUriType().getValue())); 252 return tgt; 253 } 254 255 public static org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePageComponent convertImplementationGuidePageComponent(org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideDefinitionPageComponent src) throws FHIRException { 256 if (src == null) 257 return null; 258 org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePageComponent tgt = new org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePageComponent(); 259 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 260 if (src.hasName()) 261 tgt.setSource(src.getName()); 262 if (src.hasTitle()) 263 tgt.setTitleElement(String30_50.convertString(src.getTitleElement())); 264 if (src.hasGeneration()) 265 tgt.setKind(convertPageGeneration(src.getGeneration())); 266 for (org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideDefinitionPageComponent t : src.getPage()) 267 tgt.addPage(convertImplementationGuidePageComponent(t)); 268 return tgt; 269 } 270 271 public static org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideDefinitionPageComponent convertImplementationGuidePageComponent(org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePageComponent src) throws FHIRException { 272 if (src == null) 273 return null; 274 org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideDefinitionPageComponent tgt = new org.hl7.fhir.r5.model.ImplementationGuide.ImplementationGuideDefinitionPageComponent(); 275 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 276 if (src.hasSource()) 277 tgt.setNameElement(convertUriToUrl(src.getSourceElement())); 278 if (src.hasTitle()) 279 tgt.setTitleElement(String30_50.convertString(src.getTitleElement())); 280 if (src.hasKind()) 281 tgt.setGeneration(convertPageGeneration(src.getKind())); 282 for (org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePageComponent t : src.getPage()) 283 tgt.addPage(convertImplementationGuidePageComponent(t)); 284 return tgt; 285 } 286 287 static public org.hl7.fhir.r5.model.ImplementationGuide.GuidePageGeneration convertPageGeneration(org.hl7.fhir.dstu3.model.ImplementationGuide.GuidePageKind kind) { 288 switch (kind) { 289 case PAGE: 290 return org.hl7.fhir.r5.model.ImplementationGuide.GuidePageGeneration.HTML; 291 default: 292 return org.hl7.fhir.r5.model.ImplementationGuide.GuidePageGeneration.GENERATED; 293 } 294 } 295 296 static public org.hl7.fhir.dstu3.model.ImplementationGuide.GuidePageKind convertPageGeneration(org.hl7.fhir.r5.model.ImplementationGuide.GuidePageGeneration generation) { 297 switch (generation) { 298 case HTML: 299 return org.hl7.fhir.dstu3.model.ImplementationGuide.GuidePageKind.PAGE; 300 default: 301 return org.hl7.fhir.dstu3.model.ImplementationGuide.GuidePageKind.RESOURCE; 302 } 303 } 304 305 public static org.hl7.fhir.r5.model.UrlType convertUriToUrl(org.hl7.fhir.dstu3.model.UriType src) throws FHIRException { 306 org.hl7.fhir.r5.model.UrlType tgt = new org.hl7.fhir.r5.model.UrlType(src.getValue()); 307 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 308 return tgt; 309 } 310 311 static public org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePackageComponent findPackage(List<ImplementationGuide.ImplementationGuidePackageComponent> definition, String id) { 312 for (org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePackageComponent t : definition) 313 if (t.hasId() && t.getId().equals(id)) 314 return t; 315 org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePackageComponent t1 = new org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePackageComponent(); 316 t1.setName("Default Package"); 317 t1.setId(id); 318 return t1; 319 } 320}