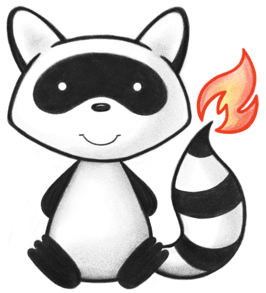
001package org.hl7.fhir.convertors.conv30_50.resources30_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_50; 004import org.hl7.fhir.convertors.conv30_50.datatypes30_50.Reference30_50; 005import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Address30_50; 006import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.CodeableConcept30_50; 007import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Coding30_50; 008import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.ContactPoint30_50; 009import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Identifier30_50; 010import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Decimal30_50; 011import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.String30_50; 012import org.hl7.fhir.dstu3.model.Enumeration; 013import org.hl7.fhir.dstu3.model.Location; 014import org.hl7.fhir.exceptions.FHIRException; 015import org.hl7.fhir.r5.model.ContactPoint; 016import org.hl7.fhir.r5.model.ExtendedContactDetail; 017 018public class Location30_50 { 019 020 public static org.hl7.fhir.r5.model.Location convertLocation(org.hl7.fhir.dstu3.model.Location src) throws FHIRException { 021 if (src == null) 022 return null; 023 org.hl7.fhir.r5.model.Location tgt = new org.hl7.fhir.r5.model.Location(); 024 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 025 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 026 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 027 if (src.hasStatus()) 028 tgt.setStatusElement(convertLocationStatus(src.getStatusElement())); 029 if (src.hasOperationalStatus()) 030 tgt.setOperationalStatus(Coding30_50.convertCoding(src.getOperationalStatus())); 031 if (src.hasName()) 032 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 033 for (org.hl7.fhir.dstu3.model.StringType t : src.getAlias()) tgt.addAlias(t.getValue()); 034 if (src.hasDescription()) 035 tgt.setDescriptionElement(String30_50.convertStringToMarkdown(src.getDescriptionElement())); 036 if (src.hasMode()) 037 tgt.setModeElement(convertLocationMode(src.getModeElement())); 038 if (src.hasType()) 039 tgt.addType(CodeableConcept30_50.convertCodeableConcept(src.getType())); 040 for (org.hl7.fhir.dstu3.model.ContactPoint t : src.getTelecom()) 041 tgt.getContactFirstRep().addTelecom(ContactPoint30_50.convertContactPoint(t)); 042 if (src.hasAddress()) 043 tgt.setAddress(Address30_50.convertAddress(src.getAddress())); 044 if (src.hasPhysicalType()) 045 tgt.setForm(CodeableConcept30_50.convertCodeableConcept(src.getPhysicalType())); 046 if (src.hasPosition()) 047 tgt.setPosition(convertLocationPositionComponent(src.getPosition())); 048 if (src.hasManagingOrganization()) 049 tgt.setManagingOrganization(Reference30_50.convertReference(src.getManagingOrganization())); 050 if (src.hasPartOf()) 051 tgt.setPartOf(Reference30_50.convertReference(src.getPartOf())); 052 for (org.hl7.fhir.dstu3.model.Reference t : src.getEndpoint()) tgt.addEndpoint(Reference30_50.convertReference(t)); 053 return tgt; 054 } 055 056 public static org.hl7.fhir.dstu3.model.Location convertLocation(org.hl7.fhir.r5.model.Location src) throws FHIRException { 057 if (src == null) 058 return null; 059 org.hl7.fhir.dstu3.model.Location tgt = new org.hl7.fhir.dstu3.model.Location(); 060 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 061 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 062 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 063 if (src.hasStatus()) 064 tgt.setStatusElement(convertLocationStatus(src.getStatusElement())); 065 if (src.hasOperationalStatus()) 066 tgt.setOperationalStatus(Coding30_50.convertCoding(src.getOperationalStatus())); 067 if (src.hasName()) 068 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 069 for (org.hl7.fhir.r5.model.StringType t : src.getAlias()) tgt.addAlias(t.getValue()); 070 if (src.hasDescription()) 071 tgt.setDescriptionElement(String30_50.convertString(src.getDescriptionElement())); 072 if (src.hasMode()) 073 tgt.setModeElement(convertLocationMode(src.getModeElement())); 074 if (src.hasType()) 075 tgt.setType(CodeableConcept30_50.convertCodeableConcept(src.getTypeFirstRep())); 076 for (ExtendedContactDetail t1 : src.getContact()) 077 for (ContactPoint t : t1.getTelecom()) 078 tgt.addTelecom(ContactPoint30_50.convertContactPoint(t)); 079 if (src.hasAddress()) 080 tgt.setAddress(Address30_50.convertAddress(src.getAddress())); 081 if (src.hasForm()) 082 tgt.setPhysicalType(CodeableConcept30_50.convertCodeableConcept(src.getForm())); 083 if (src.hasPosition()) 084 tgt.setPosition(convertLocationPositionComponent(src.getPosition())); 085 if (src.hasManagingOrganization()) 086 tgt.setManagingOrganization(Reference30_50.convertReference(src.getManagingOrganization())); 087 if (src.hasPartOf()) 088 tgt.setPartOf(Reference30_50.convertReference(src.getPartOf())); 089 for (org.hl7.fhir.r5.model.Reference t : src.getEndpoint()) tgt.addEndpoint(Reference30_50.convertReference(t)); 090 return tgt; 091 } 092 093 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Location.LocationMode> convertLocationMode(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Location.LocationMode> src) throws FHIRException { 094 if (src == null || src.isEmpty()) 095 return null; 096 Enumeration<Location.LocationMode> tgt = new Enumeration<>(new Location.LocationModeEnumFactory()); 097 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 098 if (src.getValue() == null) { 099 tgt.setValue(null); 100 } else { 101 switch (src.getValue()) { 102 case INSTANCE: 103 tgt.setValue(Location.LocationMode.INSTANCE); 104 break; 105 case KIND: 106 tgt.setValue(Location.LocationMode.KIND); 107 break; 108 default: 109 tgt.setValue(Location.LocationMode.NULL); 110 break; 111 } 112 } 113 return tgt; 114 } 115 116 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Location.LocationMode> convertLocationMode(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Location.LocationMode> src) throws FHIRException { 117 if (src == null || src.isEmpty()) 118 return null; 119 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Location.LocationMode> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Location.LocationModeEnumFactory()); 120 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 121 if (src.getValue() == null) { 122 tgt.setValue(null); 123 } else { 124 switch (src.getValue()) { 125 case INSTANCE: 126 tgt.setValue(org.hl7.fhir.r5.model.Location.LocationMode.INSTANCE); 127 break; 128 case KIND: 129 tgt.setValue(org.hl7.fhir.r5.model.Location.LocationMode.KIND); 130 break; 131 default: 132 tgt.setValue(org.hl7.fhir.r5.model.Location.LocationMode.NULL); 133 break; 134 } 135 } 136 return tgt; 137 } 138 139 public static org.hl7.fhir.dstu3.model.Location.LocationPositionComponent convertLocationPositionComponent(org.hl7.fhir.r5.model.Location.LocationPositionComponent src) throws FHIRException { 140 if (src == null) 141 return null; 142 org.hl7.fhir.dstu3.model.Location.LocationPositionComponent tgt = new org.hl7.fhir.dstu3.model.Location.LocationPositionComponent(); 143 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 144 if (src.hasLongitude()) 145 tgt.setLongitudeElement(Decimal30_50.convertDecimal(src.getLongitudeElement())); 146 if (src.hasLatitude()) 147 tgt.setLatitudeElement(Decimal30_50.convertDecimal(src.getLatitudeElement())); 148 if (src.hasAltitude()) 149 tgt.setAltitudeElement(Decimal30_50.convertDecimal(src.getAltitudeElement())); 150 return tgt; 151 } 152 153 public static org.hl7.fhir.r5.model.Location.LocationPositionComponent convertLocationPositionComponent(org.hl7.fhir.dstu3.model.Location.LocationPositionComponent src) throws FHIRException { 154 if (src == null) 155 return null; 156 org.hl7.fhir.r5.model.Location.LocationPositionComponent tgt = new org.hl7.fhir.r5.model.Location.LocationPositionComponent(); 157 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 158 if (src.hasLongitude()) 159 tgt.setLongitudeElement(Decimal30_50.convertDecimal(src.getLongitudeElement())); 160 if (src.hasLatitude()) 161 tgt.setLatitudeElement(Decimal30_50.convertDecimal(src.getLatitudeElement())); 162 if (src.hasAltitude()) 163 tgt.setAltitudeElement(Decimal30_50.convertDecimal(src.getAltitudeElement())); 164 return tgt; 165 } 166 167 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Location.LocationStatus> convertLocationStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Location.LocationStatus> src) throws FHIRException { 168 if (src == null || src.isEmpty()) 169 return null; 170 Enumeration<Location.LocationStatus> tgt = new Enumeration<>(new Location.LocationStatusEnumFactory()); 171 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 172 if (src.getValue() == null) { 173 tgt.setValue(null); 174 } else { 175 switch (src.getValue()) { 176 case ACTIVE: 177 tgt.setValue(Location.LocationStatus.ACTIVE); 178 break; 179 case SUSPENDED: 180 tgt.setValue(Location.LocationStatus.SUSPENDED); 181 break; 182 case INACTIVE: 183 tgt.setValue(Location.LocationStatus.INACTIVE); 184 break; 185 default: 186 tgt.setValue(Location.LocationStatus.NULL); 187 break; 188 } 189 } 190 return tgt; 191 } 192 193 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Location.LocationStatus> convertLocationStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Location.LocationStatus> src) throws FHIRException { 194 if (src == null || src.isEmpty()) 195 return null; 196 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Location.LocationStatus> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Location.LocationStatusEnumFactory()); 197 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 198 if (src.getValue() == null) { 199 tgt.setValue(null); 200 } else { 201 switch (src.getValue()) { 202 case ACTIVE: 203 tgt.setValue(org.hl7.fhir.r5.model.Location.LocationStatus.ACTIVE); 204 break; 205 case SUSPENDED: 206 tgt.setValue(org.hl7.fhir.r5.model.Location.LocationStatus.SUSPENDED); 207 break; 208 case INACTIVE: 209 tgt.setValue(org.hl7.fhir.r5.model.Location.LocationStatus.INACTIVE); 210 break; 211 default: 212 tgt.setValue(org.hl7.fhir.r5.model.Location.LocationStatus.NULL); 213 break; 214 } 215 } 216 return tgt; 217 } 218}