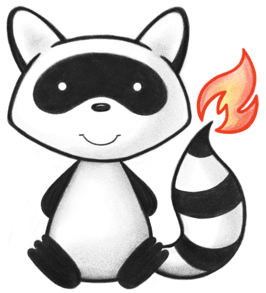
001package org.hl7.fhir.convertors.conv30_50.resources30_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_50; 004import org.hl7.fhir.convertors.conv30_50.datatypes30_50.Reference30_50; 005import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.CodeableConcept30_50; 006import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Ratio30_50; 007import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Boolean30_50; 008import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.DateTime30_50; 009import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.String30_50; 010import org.hl7.fhir.exceptions.FHIRException; 011import org.hl7.fhir.r5.model.Enumeration; 012import org.hl7.fhir.r5.model.Medication; 013 014public class Medication30_50 { 015 016 public static org.hl7.fhir.r5.model.Medication convertMedication(org.hl7.fhir.dstu3.model.Medication src) throws FHIRException { 017 if (src == null) 018 return null; 019 org.hl7.fhir.r5.model.Medication tgt = new org.hl7.fhir.r5.model.Medication(); 020 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 021 if (src.hasCode()) 022 tgt.setCode(CodeableConcept30_50.convertCodeableConcept(src.getCode())); 023 if (src.hasStatus()) 024 tgt.setStatusElement(convertMedicationStatus(src.getStatusElement())); 025 if (src.hasManufacturer()) 026 tgt.setMarketingAuthorizationHolder(Reference30_50.convertReference(src.getManufacturer())); 027 if (src.hasForm()) 028 tgt.setDoseForm(CodeableConcept30_50.convertCodeableConcept(src.getForm())); 029 for (org.hl7.fhir.dstu3.model.Medication.MedicationIngredientComponent t : src.getIngredient()) 030 tgt.addIngredient(convertMedicationIngredientComponent(t)); 031 if (src.hasPackage()) 032 tgt.setBatch(convertMedicationPackageBatchComponent(src.getPackage().getBatchFirstRep())); 033 return tgt; 034 } 035 036 public static org.hl7.fhir.dstu3.model.Medication convertMedication(org.hl7.fhir.r5.model.Medication src) throws FHIRException { 037 if (src == null) 038 return null; 039 org.hl7.fhir.dstu3.model.Medication tgt = new org.hl7.fhir.dstu3.model.Medication(); 040 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 041 if (src.hasCode()) 042 tgt.setCode(CodeableConcept30_50.convertCodeableConcept(src.getCode())); 043 if (src.hasStatus()) 044 tgt.setStatusElement(convertMedicationStatus(src.getStatusElement())); 045 if (src.hasMarketingAuthorizationHolder()) 046 tgt.setManufacturer(Reference30_50.convertReference(src.getMarketingAuthorizationHolder())); 047 if (src.hasDoseForm()) 048 tgt.setForm(CodeableConcept30_50.convertCodeableConcept(src.getDoseForm())); 049 for (org.hl7.fhir.r5.model.Medication.MedicationIngredientComponent t : src.getIngredient()) 050 tgt.addIngredient(convertMedicationIngredientComponent(t)); 051 if (src.hasBatch()) 052 tgt.getPackage().addBatch(convertMedicationPackageBatchComponent(src.getBatch())); 053 return tgt; 054 } 055 056 public static org.hl7.fhir.dstu3.model.Medication.MedicationIngredientComponent convertMedicationIngredientComponent(org.hl7.fhir.r5.model.Medication.MedicationIngredientComponent src) throws FHIRException { 057 if (src == null) 058 return null; 059 org.hl7.fhir.dstu3.model.Medication.MedicationIngredientComponent tgt = new org.hl7.fhir.dstu3.model.Medication.MedicationIngredientComponent(); 060 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 061 if (src.getItem().hasConcept()) 062 tgt.setItem(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getItem().getConcept())); 063 if (src.getItem().hasReference()) 064 tgt.setItem(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getItem().getReference())); 065 if (src.hasIsActive()) 066 tgt.setIsActiveElement(Boolean30_50.convertBoolean(src.getIsActiveElement())); 067 if (src.hasStrengthRatio()) 068 tgt.setAmount(Ratio30_50.convertRatio(src.getStrengthRatio())); 069 return tgt; 070 } 071 072 public static org.hl7.fhir.r5.model.Medication.MedicationIngredientComponent convertMedicationIngredientComponent(org.hl7.fhir.dstu3.model.Medication.MedicationIngredientComponent src) throws FHIRException { 073 if (src == null) 074 return null; 075 org.hl7.fhir.r5.model.Medication.MedicationIngredientComponent tgt = new org.hl7.fhir.r5.model.Medication.MedicationIngredientComponent(); 076 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 077 if (src.hasItemCodeableConcept()) 078 tgt.getItem().setConcept(CodeableConcept30_50.convertCodeableConcept(src.getItemCodeableConcept())); 079 if (src.hasItemReference()) 080 tgt.getItem().setReference(Reference30_50.convertReference(src.getItemReference())); 081 if (src.hasIsActive()) 082 tgt.setIsActiveElement(Boolean30_50.convertBoolean(src.getIsActiveElement())); 083 if (src.hasAmount()) 084 tgt.setStrength(Ratio30_50.convertRatio(src.getAmount())); 085 return tgt; 086 } 087 088 public static org.hl7.fhir.dstu3.model.Medication.MedicationPackageBatchComponent convertMedicationPackageBatchComponent(org.hl7.fhir.r5.model.Medication.MedicationBatchComponent src) throws FHIRException { 089 if (src == null) 090 return null; 091 org.hl7.fhir.dstu3.model.Medication.MedicationPackageBatchComponent tgt = new org.hl7.fhir.dstu3.model.Medication.MedicationPackageBatchComponent(); 092 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 093 if (src.hasLotNumber()) 094 tgt.setLotNumberElement(String30_50.convertString(src.getLotNumberElement())); 095 if (src.hasExpirationDate()) 096 tgt.setExpirationDateElement(DateTime30_50.convertDateTime(src.getExpirationDateElement())); 097 return tgt; 098 } 099 100 public static org.hl7.fhir.r5.model.Medication.MedicationBatchComponent convertMedicationPackageBatchComponent(org.hl7.fhir.dstu3.model.Medication.MedicationPackageBatchComponent src) throws FHIRException { 101 if (src == null) 102 return null; 103 org.hl7.fhir.r5.model.Medication.MedicationBatchComponent tgt = new org.hl7.fhir.r5.model.Medication.MedicationBatchComponent(); 104 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 105 if (src.hasLotNumber()) 106 tgt.setLotNumberElement(String30_50.convertString(src.getLotNumberElement())); 107 if (src.hasExpirationDate()) 108 tgt.setExpirationDateElement(DateTime30_50.convertDateTime(src.getExpirationDateElement())); 109 return tgt; 110 } 111 112 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Medication.MedicationStatusCodes> convertMedicationStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Medication.MedicationStatus> src) throws FHIRException { 113 if (src == null || src.isEmpty()) 114 return null; 115 Enumeration<Medication.MedicationStatusCodes> tgt = new Enumeration<>(new Medication.MedicationStatusCodesEnumFactory()); 116 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 117 if (src.getValue() == null) { 118 tgt.setValue(null); 119 } else { 120 switch (src.getValue()) { 121 case ACTIVE: 122 tgt.setValue(Medication.MedicationStatusCodes.ACTIVE); 123 break; 124 case INACTIVE: 125 tgt.setValue(Medication.MedicationStatusCodes.INACTIVE); 126 break; 127 case ENTEREDINERROR: 128 tgt.setValue(Medication.MedicationStatusCodes.ENTEREDINERROR); 129 break; 130 default: 131 tgt.setValue(Medication.MedicationStatusCodes.NULL); 132 break; 133 } 134 } 135 return tgt; 136 } 137 138 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Medication.MedicationStatus> convertMedicationStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Medication.MedicationStatusCodes> src) throws FHIRException { 139 if (src == null || src.isEmpty()) 140 return null; 141 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Medication.MedicationStatus> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Medication.MedicationStatusEnumFactory()); 142 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 143 if (src.getValue() == null) { 144 tgt.setValue(null); 145 } else { 146 switch (src.getValue()) { 147 case ACTIVE: 148 tgt.setValue(org.hl7.fhir.dstu3.model.Medication.MedicationStatus.ACTIVE); 149 break; 150 case INACTIVE: 151 tgt.setValue(org.hl7.fhir.dstu3.model.Medication.MedicationStatus.INACTIVE); 152 break; 153 case ENTEREDINERROR: 154 tgt.setValue(org.hl7.fhir.dstu3.model.Medication.MedicationStatus.ENTEREDINERROR); 155 break; 156 default: 157 tgt.setValue(org.hl7.fhir.dstu3.model.Medication.MedicationStatus.NULL); 158 break; 159 } 160 } 161 return tgt; 162 } 163}