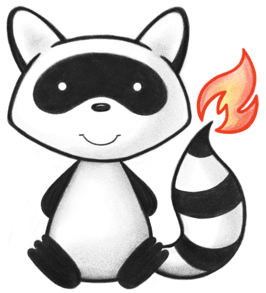
001package org.hl7.fhir.convertors.conv30_50.resources30_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_50; 004import org.hl7.fhir.convertors.conv30_50.datatypes30_50.Dosage30_50; 005import org.hl7.fhir.convertors.conv30_50.datatypes30_50.Reference30_50; 006import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Annotation30_50; 007import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.CodeableConcept30_50; 008import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Duration30_50; 009import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Identifier30_50; 010import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Period30_50; 011import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.SimpleQuantity30_50; 012import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Boolean30_50; 013import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.DateTime30_50; 014import org.hl7.fhir.exceptions.FHIRException; 015import org.hl7.fhir.r5.model.CodeableReference; 016import org.hl7.fhir.r5.model.Enumeration; 017import org.hl7.fhir.r5.model.Enumerations; 018import org.hl7.fhir.r5.model.MedicationRequest; 019 020public class MedicationRequest30_50 { 021 022 public static org.hl7.fhir.dstu3.model.MedicationRequest convertMedicationRequest(org.hl7.fhir.r5.model.MedicationRequest src) throws FHIRException { 023 if (src == null) 024 return null; 025 org.hl7.fhir.dstu3.model.MedicationRequest tgt = new org.hl7.fhir.dstu3.model.MedicationRequest(); 026 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 027 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 028 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 029 for (org.hl7.fhir.r5.model.Reference t : src.getBasedOn()) tgt.addBasedOn(Reference30_50.convertReference(t)); 030 if (src.hasGroupIdentifier()) 031 tgt.setGroupIdentifier(Identifier30_50.convertIdentifier(src.getGroupIdentifier())); 032 if (src.hasStatus()) 033 tgt.setStatusElement(convertMedicationRequestStatus(src.getStatusElement())); 034 if (src.hasIntent()) 035 tgt.setIntentElement(convertMedicationRequestIntent(src.getIntentElement())); 036 if (src.hasPriority()) 037 tgt.setPriorityElement(convertMedicationRequestPriority(src.getPriorityElement())); 038 if (src.getMedication().hasConcept()) 039 tgt.setMedication(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getMedication().getConcept())); 040 if (src.getMedication().hasReference()) 041 tgt.setMedication(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getMedication().getReference())); 042 if (src.hasSubject()) 043 tgt.setSubject(Reference30_50.convertReference(src.getSubject())); 044 if (src.hasEncounter()) 045 tgt.setContext(Reference30_50.convertReference(src.getEncounter())); 046 for (org.hl7.fhir.r5.model.Reference t : src.getSupportingInformation()) 047 tgt.addSupportingInformation(Reference30_50.convertReference(t)); 048 if (src.hasAuthoredOn()) 049 tgt.setAuthoredOnElement(DateTime30_50.convertDateTime(src.getAuthoredOnElement())); 050 if (src.hasRecorder()) 051 tgt.setRecorder(Reference30_50.convertReference(src.getRecorder())); 052 for (CodeableReference t : src.getReason()) { 053 if (t.hasConcept()) { 054 tgt.addReasonCode(CodeableConcept30_50.convertCodeableConcept(t.getConcept())); 055 } 056 if (t.hasReference()) { 057 tgt.addReasonReference(Reference30_50.convertReference(t.getReference())); 058 } 059 } 060 for (org.hl7.fhir.r5.model.Annotation t : src.getNote()) tgt.addNote(Annotation30_50.convertAnnotation(t)); 061 for (org.hl7.fhir.r5.model.Dosage t : src.getDosageInstruction()) 062 tgt.addDosageInstruction(Dosage30_50.convertDosage(t)); 063 if (src.hasDispenseRequest()) 064 tgt.setDispenseRequest(convertMedicationRequestDispenseRequestComponent(src.getDispenseRequest())); 065 if (src.hasSubstitution()) 066 tgt.setSubstitution(convertMedicationRequestSubstitutionComponent(src.getSubstitution())); 067 if (src.hasPriorPrescription()) 068 tgt.setPriorPrescription(Reference30_50.convertReference(src.getPriorPrescription())); 069// for (org.hl7.fhir.r5.model.Reference t : src.getDetectedIssue()) 070// tgt.addDetectedIssue(Reference30_50.convertReference(t)); 071 for (org.hl7.fhir.r5.model.Reference t : src.getEventHistory()) 072 tgt.addEventHistory(Reference30_50.convertReference(t)); 073 return tgt; 074 } 075 076 public static org.hl7.fhir.r5.model.MedicationRequest convertMedicationRequest(org.hl7.fhir.dstu3.model.MedicationRequest src) throws FHIRException { 077 if (src == null) 078 return null; 079 org.hl7.fhir.r5.model.MedicationRequest tgt = new org.hl7.fhir.r5.model.MedicationRequest(); 080 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 081 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 082 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 083 for (org.hl7.fhir.dstu3.model.Reference t : src.getBasedOn()) tgt.addBasedOn(Reference30_50.convertReference(t)); 084 if (src.hasGroupIdentifier()) 085 tgt.setGroupIdentifier(Identifier30_50.convertIdentifier(src.getGroupIdentifier())); 086 if (src.hasStatus()) 087 tgt.setStatusElement(convertMedicationRequestStatus(src.getStatusElement())); 088 if (src.hasIntent()) 089 tgt.setIntentElement(convertMedicationRequestIntent(src.getIntentElement())); 090 if (src.hasPriority()) 091 tgt.setPriorityElement(convertMedicationRequestPriority(src.getPriorityElement())); 092 if (src.hasMedicationCodeableConcept()) 093 tgt.getMedication().setConcept(CodeableConcept30_50.convertCodeableConcept(src.getMedicationCodeableConcept())); 094 if (src.hasMedicationReference()) 095 tgt.getMedication().setReference(Reference30_50.convertReference(src.getMedicationReference())); 096 if (src.hasSubject()) 097 tgt.setSubject(Reference30_50.convertReference(src.getSubject())); 098 if (src.hasContext()) 099 tgt.setEncounter(Reference30_50.convertReference(src.getContext())); 100 for (org.hl7.fhir.dstu3.model.Reference t : src.getSupportingInformation()) 101 tgt.addSupportingInformation(Reference30_50.convertReference(t)); 102 if (src.hasAuthoredOn()) 103 tgt.setAuthoredOnElement(DateTime30_50.convertDateTime(src.getAuthoredOnElement())); 104 if (src.hasRecorder()) 105 tgt.setRecorder(Reference30_50.convertReference(src.getRecorder())); 106 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getReasonCode()) 107 tgt.addReason(new CodeableReference().setConcept(CodeableConcept30_50.convertCodeableConcept(t))); 108 for (org.hl7.fhir.dstu3.model.Reference t : src.getReasonReference()) 109 tgt.addReason(new CodeableReference().setReference(Reference30_50.convertReference(t))); 110 for (org.hl7.fhir.dstu3.model.Annotation t : src.getNote()) tgt.addNote(Annotation30_50.convertAnnotation(t)); 111 for (org.hl7.fhir.dstu3.model.Dosage t : src.getDosageInstruction()) 112 tgt.addDosageInstruction(Dosage30_50.convertDosage(t)); 113 if (src.hasDispenseRequest()) 114 tgt.setDispenseRequest(convertMedicationRequestDispenseRequestComponent(src.getDispenseRequest())); 115 if (src.hasSubstitution()) 116 tgt.setSubstitution(convertMedicationRequestSubstitutionComponent(src.getSubstitution())); 117 if (src.hasPriorPrescription()) 118 tgt.setPriorPrescription(Reference30_50.convertReference(src.getPriorPrescription())); 119// for (org.hl7.fhir.dstu3.model.Reference t : src.getDetectedIssue()) 120// tgt.addDetectedIssue(Reference30_50.convertReference(t)); 121 for (org.hl7.fhir.dstu3.model.Reference t : src.getEventHistory()) 122 tgt.addEventHistory(Reference30_50.convertReference(t)); 123 return tgt; 124 } 125 126 public static org.hl7.fhir.r5.model.MedicationRequest.MedicationRequestDispenseRequestComponent convertMedicationRequestDispenseRequestComponent(org.hl7.fhir.dstu3.model.MedicationRequest.MedicationRequestDispenseRequestComponent src) throws FHIRException { 127 if (src == null) 128 return null; 129 org.hl7.fhir.r5.model.MedicationRequest.MedicationRequestDispenseRequestComponent tgt = new org.hl7.fhir.r5.model.MedicationRequest.MedicationRequestDispenseRequestComponent(); 130 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 131 if (src.hasValidityPeriod()) 132 tgt.setValidityPeriod(Period30_50.convertPeriod(src.getValidityPeriod())); 133 if (src.hasNumberOfRepeatsAllowed()) 134 tgt.setNumberOfRepeatsAllowed(src.getNumberOfRepeatsAllowed()); 135 if (src.hasQuantity()) 136 tgt.setQuantity(SimpleQuantity30_50.convertSimpleQuantity(src.getQuantity())); 137 if (src.hasExpectedSupplyDuration()) 138 tgt.setExpectedSupplyDuration(Duration30_50.convertDuration(src.getExpectedSupplyDuration())); 139 if (src.hasPerformer()) 140 tgt.setDispenser(Reference30_50.convertReference(src.getPerformer())); 141 return tgt; 142 } 143 144 public static org.hl7.fhir.dstu3.model.MedicationRequest.MedicationRequestDispenseRequestComponent convertMedicationRequestDispenseRequestComponent(org.hl7.fhir.r5.model.MedicationRequest.MedicationRequestDispenseRequestComponent src) throws FHIRException { 145 if (src == null) 146 return null; 147 org.hl7.fhir.dstu3.model.MedicationRequest.MedicationRequestDispenseRequestComponent tgt = new org.hl7.fhir.dstu3.model.MedicationRequest.MedicationRequestDispenseRequestComponent(); 148 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 149 if (src.hasValidityPeriod()) 150 tgt.setValidityPeriod(Period30_50.convertPeriod(src.getValidityPeriod())); 151 if (src.hasNumberOfRepeatsAllowed()) 152 tgt.setNumberOfRepeatsAllowed(src.getNumberOfRepeatsAllowed()); 153 if (src.hasQuantity()) 154 tgt.setQuantity(SimpleQuantity30_50.convertSimpleQuantity(src.getQuantity())); 155 if (src.hasExpectedSupplyDuration()) 156 tgt.setExpectedSupplyDuration(Duration30_50.convertDuration(src.getExpectedSupplyDuration())); 157 if (src.hasDispenser()) 158 tgt.setPerformer(Reference30_50.convertReference(src.getDispenser())); 159 return tgt; 160 } 161 162 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.MedicationRequest.MedicationRequestIntent> convertMedicationRequestIntent(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.MedicationRequest.MedicationRequestIntent> src) throws FHIRException { 163 if (src == null || src.isEmpty()) 164 return null; 165 Enumeration<MedicationRequest.MedicationRequestIntent> tgt = new Enumeration<>(new MedicationRequest.MedicationRequestIntentEnumFactory()); 166 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 167 if (src.getValue() == null) { 168 tgt.setValue(null); 169 } else { 170 switch (src.getValue()) { 171 case PROPOSAL: 172 tgt.setValue(MedicationRequest.MedicationRequestIntent.PROPOSAL); 173 break; 174 case PLAN: 175 tgt.setValue(MedicationRequest.MedicationRequestIntent.PLAN); 176 break; 177 case ORDER: 178 tgt.setValue(MedicationRequest.MedicationRequestIntent.ORDER); 179 break; 180 case INSTANCEORDER: 181 tgt.setValue(MedicationRequest.MedicationRequestIntent.INSTANCEORDER); 182 break; 183 default: 184 tgt.setValue(MedicationRequest.MedicationRequestIntent.NULL); 185 break; 186 } 187 } 188 return tgt; 189 } 190 191 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.MedicationRequest.MedicationRequestIntent> convertMedicationRequestIntent(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.MedicationRequest.MedicationRequestIntent> src) throws FHIRException { 192 if (src == null || src.isEmpty()) 193 return null; 194 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.MedicationRequest.MedicationRequestIntent> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.MedicationRequest.MedicationRequestIntentEnumFactory()); 195 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 196 if (src.getValue() == null) { 197 tgt.setValue(null); 198 } else { 199 switch (src.getValue()) { 200 case PROPOSAL: 201 tgt.setValue(org.hl7.fhir.dstu3.model.MedicationRequest.MedicationRequestIntent.PROPOSAL); 202 break; 203 case PLAN: 204 tgt.setValue(org.hl7.fhir.dstu3.model.MedicationRequest.MedicationRequestIntent.PLAN); 205 break; 206 case ORDER: 207 tgt.setValue(org.hl7.fhir.dstu3.model.MedicationRequest.MedicationRequestIntent.ORDER); 208 break; 209 case INSTANCEORDER: 210 tgt.setValue(org.hl7.fhir.dstu3.model.MedicationRequest.MedicationRequestIntent.INSTANCEORDER); 211 break; 212 default: 213 tgt.setValue(org.hl7.fhir.dstu3.model.MedicationRequest.MedicationRequestIntent.NULL); 214 break; 215 } 216 } 217 return tgt; 218 } 219 220 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.RequestPriority> convertMedicationRequestPriority(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.MedicationRequest.MedicationRequestPriority> src) throws FHIRException { 221 if (src == null || src.isEmpty()) 222 return null; 223 Enumeration<Enumerations.RequestPriority> tgt = new Enumeration<>(new Enumerations.RequestPriorityEnumFactory()); 224 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 225 if (src.getValue() == null) { 226 tgt.setValue(null); 227 } else { 228 switch (src.getValue()) { 229 case ROUTINE: 230 tgt.setValue(Enumerations.RequestPriority.ROUTINE); 231 break; 232 case URGENT: 233 tgt.setValue(Enumerations.RequestPriority.URGENT); 234 break; 235 case STAT: 236 tgt.setValue(Enumerations.RequestPriority.STAT); 237 break; 238 case ASAP: 239 tgt.setValue(Enumerations.RequestPriority.ASAP); 240 break; 241 default: 242 tgt.setValue(Enumerations.RequestPriority.NULL); 243 break; 244 } 245 } 246 return tgt; 247 } 248 249 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.MedicationRequest.MedicationRequestPriority> convertMedicationRequestPriority(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.RequestPriority> src) throws FHIRException { 250 if (src == null || src.isEmpty()) 251 return null; 252 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.MedicationRequest.MedicationRequestPriority> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.MedicationRequest.MedicationRequestPriorityEnumFactory()); 253 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 254 if (src.getValue() == null) { 255 tgt.setValue(null); 256 } else { 257 switch (src.getValue()) { 258 case ROUTINE: 259 tgt.setValue(org.hl7.fhir.dstu3.model.MedicationRequest.MedicationRequestPriority.ROUTINE); 260 break; 261 case URGENT: 262 tgt.setValue(org.hl7.fhir.dstu3.model.MedicationRequest.MedicationRequestPriority.URGENT); 263 break; 264 case STAT: 265 tgt.setValue(org.hl7.fhir.dstu3.model.MedicationRequest.MedicationRequestPriority.STAT); 266 break; 267 case ASAP: 268 tgt.setValue(org.hl7.fhir.dstu3.model.MedicationRequest.MedicationRequestPriority.ASAP); 269 break; 270 default: 271 tgt.setValue(org.hl7.fhir.dstu3.model.MedicationRequest.MedicationRequestPriority.NULL); 272 break; 273 } 274 } 275 return tgt; 276 } 277 278 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.MedicationRequest.MedicationrequestStatus> convertMedicationRequestStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.MedicationRequest.MedicationRequestStatus> src) throws FHIRException { 279 if (src == null || src.isEmpty()) 280 return null; 281 Enumeration<MedicationRequest.MedicationrequestStatus> tgt = new Enumeration<>(new MedicationRequest.MedicationrequestStatusEnumFactory()); 282 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 283 if (src.getValue() == null) { 284 tgt.setValue(null); 285 } else { 286 switch (src.getValue()) { 287 case ACTIVE: 288 tgt.setValue(MedicationRequest.MedicationrequestStatus.ACTIVE); 289 break; 290 case ONHOLD: 291 tgt.setValue(MedicationRequest.MedicationrequestStatus.ONHOLD); 292 break; 293 case CANCELLED: 294 tgt.setValue(MedicationRequest.MedicationrequestStatus.CANCELLED); 295 break; 296 case COMPLETED: 297 tgt.setValue(MedicationRequest.MedicationrequestStatus.COMPLETED); 298 break; 299 case ENTEREDINERROR: 300 tgt.setValue(MedicationRequest.MedicationrequestStatus.ENTEREDINERROR); 301 break; 302 case STOPPED: 303 tgt.setValue(MedicationRequest.MedicationrequestStatus.STOPPED); 304 break; 305 case DRAFT: 306 tgt.setValue(MedicationRequest.MedicationrequestStatus.DRAFT); 307 break; 308 case UNKNOWN: 309 tgt.setValue(MedicationRequest.MedicationrequestStatus.UNKNOWN); 310 break; 311 default: 312 tgt.setValue(MedicationRequest.MedicationrequestStatus.NULL); 313 break; 314 } 315 } 316 return tgt; 317 } 318 319 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.MedicationRequest.MedicationRequestStatus> convertMedicationRequestStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.MedicationRequest.MedicationrequestStatus> src) throws FHIRException { 320 if (src == null || src.isEmpty()) 321 return null; 322 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.MedicationRequest.MedicationRequestStatus> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.MedicationRequest.MedicationRequestStatusEnumFactory()); 323 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 324 if (src.getValue() == null) { 325 tgt.setValue(null); 326 } else { 327 switch (src.getValue()) { 328 case ACTIVE: 329 tgt.setValue(org.hl7.fhir.dstu3.model.MedicationRequest.MedicationRequestStatus.ACTIVE); 330 break; 331 case ONHOLD: 332 tgt.setValue(org.hl7.fhir.dstu3.model.MedicationRequest.MedicationRequestStatus.ONHOLD); 333 break; 334 case CANCELLED: 335 tgt.setValue(org.hl7.fhir.dstu3.model.MedicationRequest.MedicationRequestStatus.CANCELLED); 336 break; 337 case COMPLETED: 338 tgt.setValue(org.hl7.fhir.dstu3.model.MedicationRequest.MedicationRequestStatus.COMPLETED); 339 break; 340 case ENTEREDINERROR: 341 tgt.setValue(org.hl7.fhir.dstu3.model.MedicationRequest.MedicationRequestStatus.ENTEREDINERROR); 342 break; 343 case STOPPED: 344 tgt.setValue(org.hl7.fhir.dstu3.model.MedicationRequest.MedicationRequestStatus.STOPPED); 345 break; 346 case DRAFT: 347 tgt.setValue(org.hl7.fhir.dstu3.model.MedicationRequest.MedicationRequestStatus.DRAFT); 348 break; 349 case UNKNOWN: 350 tgt.setValue(org.hl7.fhir.dstu3.model.MedicationRequest.MedicationRequestStatus.UNKNOWN); 351 break; 352 default: 353 tgt.setValue(org.hl7.fhir.dstu3.model.MedicationRequest.MedicationRequestStatus.NULL); 354 break; 355 } 356 } 357 return tgt; 358 } 359 360 public static org.hl7.fhir.r5.model.MedicationRequest.MedicationRequestSubstitutionComponent convertMedicationRequestSubstitutionComponent(org.hl7.fhir.dstu3.model.MedicationRequest.MedicationRequestSubstitutionComponent src) throws FHIRException { 361 if (src == null) 362 return null; 363 org.hl7.fhir.r5.model.MedicationRequest.MedicationRequestSubstitutionComponent tgt = new org.hl7.fhir.r5.model.MedicationRequest.MedicationRequestSubstitutionComponent(); 364 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 365 if (src.hasAllowed()) 366 tgt.setAllowed(Boolean30_50.convertBoolean(src.getAllowedElement())); 367 if (src.hasReason()) 368 tgt.setReason(CodeableConcept30_50.convertCodeableConcept(src.getReason())); 369 return tgt; 370 } 371 372 public static org.hl7.fhir.dstu3.model.MedicationRequest.MedicationRequestSubstitutionComponent convertMedicationRequestSubstitutionComponent(org.hl7.fhir.r5.model.MedicationRequest.MedicationRequestSubstitutionComponent src) throws FHIRException { 373 if (src == null) 374 return null; 375 org.hl7.fhir.dstu3.model.MedicationRequest.MedicationRequestSubstitutionComponent tgt = new org.hl7.fhir.dstu3.model.MedicationRequest.MedicationRequestSubstitutionComponent(); 376 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 377 if (src.hasAllowedBooleanType()) 378 tgt.setAllowedElement(Boolean30_50.convertBoolean(src.getAllowedBooleanType())); 379 if (src.hasReason()) 380 tgt.setReason(CodeableConcept30_50.convertCodeableConcept(src.getReason())); 381 return tgt; 382 } 383}