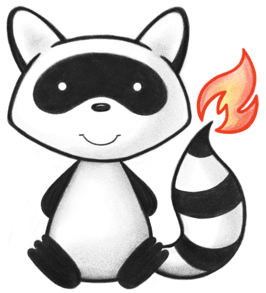
001package org.hl7.fhir.convertors.conv30_50.resources30_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_50; 004import org.hl7.fhir.convertors.conv30_50.datatypes30_50.Reference30_50; 005import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.CodeableConcept30_50; 006import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Coding30_50; 007import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.ContactPoint30_50; 008import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.String30_50; 009import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Uri30_50; 010import org.hl7.fhir.exceptions.FHIRException; 011 012public class MessageHeader30_50 { 013 014 public static org.hl7.fhir.dstu3.model.MessageHeader.MessageDestinationComponent convertMessageDestinationComponent(org.hl7.fhir.r5.model.MessageHeader.MessageDestinationComponent src) throws FHIRException { 015 if (src == null) 016 return null; 017 org.hl7.fhir.dstu3.model.MessageHeader.MessageDestinationComponent tgt = new org.hl7.fhir.dstu3.model.MessageHeader.MessageDestinationComponent(); 018 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 019 if (src.hasName()) 020 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 021 if (src.hasTarget()) 022 tgt.setTarget(Reference30_50.convertReference(src.getTarget())); 023 if (src.hasEndpointUrlType()) 024 tgt.setEndpointElement(Uri30_50.convertUrl(src.getEndpointUrlType())); 025 return tgt; 026 } 027 028 public static org.hl7.fhir.r5.model.MessageHeader.MessageDestinationComponent convertMessageDestinationComponent(org.hl7.fhir.dstu3.model.MessageHeader.MessageDestinationComponent src) throws FHIRException { 029 if (src == null) 030 return null; 031 org.hl7.fhir.r5.model.MessageHeader.MessageDestinationComponent tgt = new org.hl7.fhir.r5.model.MessageHeader.MessageDestinationComponent(); 032 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 033 if (src.hasName()) 034 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 035 if (src.hasTarget()) 036 tgt.setTarget(Reference30_50.convertReference(src.getTarget())); 037 if (src.hasEndpoint()) 038 tgt.setEndpoint(Uri30_50.convertUri(src.getEndpointElement())); 039 return tgt; 040 } 041 042 public static org.hl7.fhir.r5.model.MessageHeader convertMessageHeader(org.hl7.fhir.dstu3.model.MessageHeader src) throws FHIRException { 043 if (src == null) 044 return null; 045 org.hl7.fhir.r5.model.MessageHeader tgt = new org.hl7.fhir.r5.model.MessageHeader(); 046 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 047 if (src.hasEvent()) 048 tgt.setEvent(Coding30_50.convertCoding(src.getEvent())); 049 for (org.hl7.fhir.dstu3.model.MessageHeader.MessageDestinationComponent t : src.getDestination()) 050 tgt.addDestination(convertMessageDestinationComponent(t)); 051 if (src.hasSender()) 052 tgt.setSender(Reference30_50.convertReference(src.getSender())); 053// if (src.hasEnterer()) 054// tgt.setEnterer(Reference30_50.convertReference(src.getEnterer())); 055 if (src.hasAuthor()) 056 tgt.setAuthor(Reference30_50.convertReference(src.getAuthor())); 057 if (src.hasSource()) 058 tgt.setSource(convertMessageSourceComponent(src.getSource())); 059 if (src.hasResponsible()) 060 tgt.setResponsible(Reference30_50.convertReference(src.getResponsible())); 061 if (src.hasReason()) 062 tgt.setReason(CodeableConcept30_50.convertCodeableConcept(src.getReason())); 063 if (src.hasResponse()) 064 tgt.setResponse(convertMessageHeaderResponseComponent(src.getResponse())); 065 for (org.hl7.fhir.dstu3.model.Reference t : src.getFocus()) tgt.addFocus(Reference30_50.convertReference(t)); 066 return tgt; 067 } 068 069 public static org.hl7.fhir.dstu3.model.MessageHeader convertMessageHeader(org.hl7.fhir.r5.model.MessageHeader src) throws FHIRException { 070 if (src == null) 071 return null; 072 org.hl7.fhir.dstu3.model.MessageHeader tgt = new org.hl7.fhir.dstu3.model.MessageHeader(); 073 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 074 if (src.hasEventCoding()) 075 tgt.setEvent(Coding30_50.convertCoding(src.getEventCoding())); 076 for (org.hl7.fhir.r5.model.MessageHeader.MessageDestinationComponent t : src.getDestination()) 077 tgt.addDestination(convertMessageDestinationComponent(t)); 078 if (src.hasSender()) 079 tgt.setSender(Reference30_50.convertReference(src.getSender())); 080// if (src.hasEnterer()) 081// tgt.setEnterer(Reference30_50.convertReference(src.getEnterer())); 082 if (src.hasAuthor()) 083 tgt.setAuthor(Reference30_50.convertReference(src.getAuthor())); 084 if (src.hasSource()) 085 tgt.setSource(convertMessageSourceComponent(src.getSource())); 086 if (src.hasResponsible()) 087 tgt.setResponsible(Reference30_50.convertReference(src.getResponsible())); 088 if (src.hasReason()) 089 tgt.setReason(CodeableConcept30_50.convertCodeableConcept(src.getReason())); 090 if (src.hasResponse()) 091 tgt.setResponse(convertMessageHeaderResponseComponent(src.getResponse())); 092 for (org.hl7.fhir.r5.model.Reference t : src.getFocus()) tgt.addFocus(Reference30_50.convertReference(t)); 093 return tgt; 094 } 095 096 public static org.hl7.fhir.r5.model.MessageHeader.MessageHeaderResponseComponent convertMessageHeaderResponseComponent(org.hl7.fhir.dstu3.model.MessageHeader.MessageHeaderResponseComponent src) throws FHIRException { 097 if (src == null) 098 return null; 099 org.hl7.fhir.r5.model.MessageHeader.MessageHeaderResponseComponent tgt = new org.hl7.fhir.r5.model.MessageHeader.MessageHeaderResponseComponent(); 100 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 101 if (src.hasIdentifier()) 102 tgt.setIdentifier(new org.hl7.fhir.r5.model.Identifier().setValue(src.getIdentifier())); 103 if (src.hasCode()) 104 tgt.setCodeElement(convertResponseType(src.getCodeElement())); 105 if (src.hasDetails()) 106 tgt.setDetails(Reference30_50.convertReference(src.getDetails())); 107 return tgt; 108 } 109 110 public static org.hl7.fhir.dstu3.model.MessageHeader.MessageHeaderResponseComponent convertMessageHeaderResponseComponent(org.hl7.fhir.r5.model.MessageHeader.MessageHeaderResponseComponent src) throws FHIRException { 111 if (src == null) 112 return null; 113 org.hl7.fhir.dstu3.model.MessageHeader.MessageHeaderResponseComponent tgt = new org.hl7.fhir.dstu3.model.MessageHeader.MessageHeaderResponseComponent(); 114 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 115 if (src.hasIdentifier()) 116 tgt.setIdentifierElement(new org.hl7.fhir.dstu3.model.IdType(src.getIdentifier().getValue())); 117 if (src.hasCode()) 118 tgt.setCodeElement(convertResponseType(src.getCodeElement())); 119 if (src.hasDetails()) 120 tgt.setDetails(Reference30_50.convertReference(src.getDetails())); 121 return tgt; 122 } 123 124 public static org.hl7.fhir.dstu3.model.MessageHeader.MessageSourceComponent convertMessageSourceComponent(org.hl7.fhir.r5.model.MessageHeader.MessageSourceComponent src) throws FHIRException { 125 if (src == null) 126 return null; 127 org.hl7.fhir.dstu3.model.MessageHeader.MessageSourceComponent tgt = new org.hl7.fhir.dstu3.model.MessageHeader.MessageSourceComponent(); 128 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 129 if (src.hasName()) 130 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 131 if (src.hasSoftware()) 132 tgt.setSoftwareElement(String30_50.convertString(src.getSoftwareElement())); 133 if (src.hasVersion()) 134 tgt.setVersionElement(String30_50.convertString(src.getVersionElement())); 135 if (src.hasContact()) 136 tgt.setContact(ContactPoint30_50.convertContactPoint(src.getContact())); 137 if (src.hasEndpointUrlType()) 138 tgt.setEndpointElement(Uri30_50.convertUrl(src.getEndpointUrlType())); 139 return tgt; 140 } 141 142 public static org.hl7.fhir.r5.model.MessageHeader.MessageSourceComponent convertMessageSourceComponent(org.hl7.fhir.dstu3.model.MessageHeader.MessageSourceComponent src) throws FHIRException { 143 if (src == null) 144 return null; 145 org.hl7.fhir.r5.model.MessageHeader.MessageSourceComponent tgt = new org.hl7.fhir.r5.model.MessageHeader.MessageSourceComponent(); 146 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 147 if (src.hasName()) 148 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 149 if (src.hasSoftware()) 150 tgt.setSoftwareElement(String30_50.convertString(src.getSoftwareElement())); 151 if (src.hasVersion()) 152 tgt.setVersionElement(String30_50.convertString(src.getVersionElement())); 153 if (src.hasContact()) 154 tgt.setContact(ContactPoint30_50.convertContactPoint(src.getContact())); 155 if (src.hasEndpoint()) 156 tgt.setEndpoint(Uri30_50.convertUri(src.getEndpointElement())); 157 return tgt; 158 } 159 160 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.MessageHeader.ResponseType> convertResponseType(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.MessageHeader.ResponseType> src) throws FHIRException { 161 if (src == null || src.isEmpty()) 162 return null; 163 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.MessageHeader.ResponseType> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.MessageHeader.ResponseTypeEnumFactory()); 164 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 165 switch (src.getValue()) { 166 case OK: 167 tgt.setValue(org.hl7.fhir.r5.model.MessageHeader.ResponseType.OK); 168 break; 169 case TRANSIENTERROR: 170 tgt.setValue(org.hl7.fhir.r5.model.MessageHeader.ResponseType.TRANSIENTERROR); 171 break; 172 case FATALERROR: 173 tgt.setValue(org.hl7.fhir.r5.model.MessageHeader.ResponseType.FATALERROR); 174 break; 175 default: 176 tgt.setValue(org.hl7.fhir.r5.model.MessageHeader.ResponseType.NULL); 177 break; 178 } 179 return tgt; 180 } 181 182 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.MessageHeader.ResponseType> convertResponseType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.MessageHeader.ResponseType> src) throws FHIRException { 183 if (src == null || src.isEmpty()) 184 return null; 185 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.MessageHeader.ResponseType> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.MessageHeader.ResponseTypeEnumFactory()); 186 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 187 switch (src.getValue()) { 188 case OK: 189 tgt.setValue(org.hl7.fhir.dstu3.model.MessageHeader.ResponseType.OK); 190 break; 191 case TRANSIENTERROR: 192 tgt.setValue(org.hl7.fhir.dstu3.model.MessageHeader.ResponseType.TRANSIENTERROR); 193 break; 194 case FATALERROR: 195 tgt.setValue(org.hl7.fhir.dstu3.model.MessageHeader.ResponseType.FATALERROR); 196 break; 197 default: 198 tgt.setValue(org.hl7.fhir.dstu3.model.MessageHeader.ResponseType.NULL); 199 break; 200 } 201 return tgt; 202 } 203}