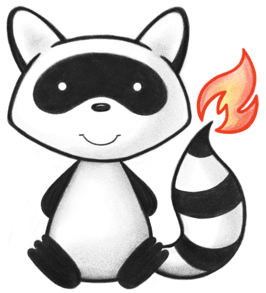
001package org.hl7.fhir.convertors.conv30_50.resources30_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_50; 004import org.hl7.fhir.convertors.conv30_50.datatypes30_50.Reference30_50; 005import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Address30_50; 006import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.CodeableConcept30_50; 007import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.ContactPoint30_50; 008import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.HumanName30_50; 009import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Identifier30_50; 010import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Boolean30_50; 011import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.String30_50; 012import org.hl7.fhir.exceptions.FHIRException; 013import org.hl7.fhir.r5.model.ExtendedContactDetail; 014 015public class Organization30_50 { 016 017 public static org.hl7.fhir.r5.model.Organization convertOrganization(org.hl7.fhir.dstu3.model.Organization src) throws FHIRException { 018 if (src == null) 019 return null; 020 org.hl7.fhir.r5.model.Organization tgt = new org.hl7.fhir.r5.model.Organization(); 021 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 022 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 023 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 024 if (src.hasActive()) 025 tgt.setActiveElement(Boolean30_50.convertBoolean(src.getActiveElement())); 026 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getType()) 027 tgt.addType(CodeableConcept30_50.convertCodeableConcept(t)); 028 if (src.hasName()) 029 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 030 for (org.hl7.fhir.dstu3.model.StringType t : src.getAlias()) tgt.addAlias(t.getValue()); 031 for (org.hl7.fhir.dstu3.model.Address t : src.getAddress()) tgt.addContact().setAddress(Address30_50.convertAddress(t)); 032 for (org.hl7.fhir.dstu3.model.ContactPoint t : src.getTelecom()) 033 tgt.getContactFirstRep().addTelecom(ContactPoint30_50.convertContactPoint(t)); 034 if (src.hasPartOf()) 035 tgt.setPartOf(Reference30_50.convertReference(src.getPartOf())); 036 for (org.hl7.fhir.dstu3.model.Organization.OrganizationContactComponent t : src.getContact()) 037 tgt.addContact(convertOrganizationContactComponent(t)); 038 for (org.hl7.fhir.dstu3.model.Reference t : src.getEndpoint()) tgt.addEndpoint(Reference30_50.convertReference(t)); 039 return tgt; 040 } 041 042 public static org.hl7.fhir.dstu3.model.Organization convertOrganization(org.hl7.fhir.r5.model.Organization src) throws FHIRException { 043 if (src == null) 044 return null; 045 org.hl7.fhir.dstu3.model.Organization tgt = new org.hl7.fhir.dstu3.model.Organization(); 046 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 047 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 048 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 049 if (src.hasActive()) 050 tgt.setActiveElement(Boolean30_50.convertBoolean(src.getActiveElement())); 051 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getType()) 052 tgt.addType(CodeableConcept30_50.convertCodeableConcept(t)); 053 if (src.hasName()) 054 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 055 for (org.hl7.fhir.r5.model.StringType t : src.getAlias()) tgt.addAlias(t.getValue()); 056 for (ExtendedContactDetail t1 : src.getContact()) 057 for (org.hl7.fhir.r5.model.ContactPoint t : t1.getTelecom()) 058 tgt.addTelecom(ContactPoint30_50.convertContactPoint(t)); 059 for (ExtendedContactDetail t : src.getContact()) 060 if (t.hasAddress()) 061 tgt.addAddress(Address30_50.convertAddress(t.getAddress())); 062 if (src.hasPartOf()) 063 tgt.setPartOf(Reference30_50.convertReference(src.getPartOf())); 064 for (org.hl7.fhir.r5.model.ExtendedContactDetail t : src.getContact()) 065 tgt.addContact(convertOrganizationContactComponent(t)); 066 for (org.hl7.fhir.r5.model.Reference t : src.getEndpoint()) tgt.addEndpoint(Reference30_50.convertReference(t)); 067 return tgt; 068 } 069 070 public static org.hl7.fhir.dstu3.model.Organization.OrganizationContactComponent convertOrganizationContactComponent(org.hl7.fhir.r5.model.ExtendedContactDetail src) throws FHIRException { 071 if (src == null) 072 return null; 073 org.hl7.fhir.dstu3.model.Organization.OrganizationContactComponent tgt = new org.hl7.fhir.dstu3.model.Organization.OrganizationContactComponent(); 074 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 075 if (src.hasPurpose()) 076 tgt.setPurpose(CodeableConcept30_50.convertCodeableConcept(src.getPurpose())); 077 for (org.hl7.fhir.r5.model.HumanName t : src.getName()) 078 tgt.setName(HumanName30_50.convertHumanName(t)); 079 for (org.hl7.fhir.r5.model.ContactPoint t : src.getTelecom()) 080 tgt.addTelecom(ContactPoint30_50.convertContactPoint(t)); 081 if (src.hasAddress()) 082 tgt.setAddress(Address30_50.convertAddress(src.getAddress())); 083 return tgt; 084 } 085 086 public static org.hl7.fhir.r5.model.ExtendedContactDetail convertOrganizationContactComponent(org.hl7.fhir.dstu3.model.Organization.OrganizationContactComponent src) throws FHIRException { 087 if (src == null) 088 return null; 089 org.hl7.fhir.r5.model.ExtendedContactDetail tgt = new org.hl7.fhir.r5.model.ExtendedContactDetail(); 090 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 091 if (src.hasPurpose()) 092 tgt.setPurpose(CodeableConcept30_50.convertCodeableConcept(src.getPurpose())); 093 if (src.hasName()) 094 tgt.addName(HumanName30_50.convertHumanName(src.getName())); 095 for (org.hl7.fhir.dstu3.model.ContactPoint t : src.getTelecom()) 096 tgt.addTelecom(ContactPoint30_50.convertContactPoint(t)); 097 if (src.hasAddress()) 098 tgt.setAddress(Address30_50.convertAddress(src.getAddress())); 099 return tgt; 100 } 101}