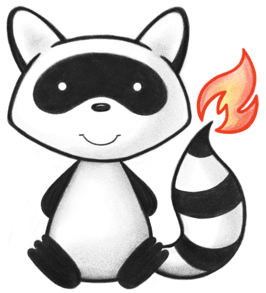
001package org.hl7.fhir.convertors.conv30_50.resources30_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_50; 004import org.hl7.fhir.convertors.conv30_50.datatypes30_50.Reference30_50; 005import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Address30_50; 006import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Attachment30_50; 007import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.ContactPoint30_50; 008import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.HumanName30_50; 009import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Identifier30_50; 010import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Boolean30_50; 011import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Date30_50; 012import org.hl7.fhir.dstu3.model.Enumeration; 013import org.hl7.fhir.dstu3.model.Person; 014import org.hl7.fhir.exceptions.FHIRException; 015 016public class Person30_50 { 017 018 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Person.IdentityAssuranceLevel> convertIdentityAssuranceLevel(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Person.IdentityAssuranceLevel> src) throws FHIRException { 019 if (src == null || src.isEmpty()) 020 return null; 021 Enumeration<Person.IdentityAssuranceLevel> tgt = new Enumeration<>(new Person.IdentityAssuranceLevelEnumFactory()); 022 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 023 if (src.getValue() == null) { 024 tgt.setValue(null); 025 } else { 026 switch (src.getValue()) { 027 case LEVEL1: 028 tgt.setValue(Person.IdentityAssuranceLevel.LEVEL1); 029 break; 030 case LEVEL2: 031 tgt.setValue(Person.IdentityAssuranceLevel.LEVEL2); 032 break; 033 case LEVEL3: 034 tgt.setValue(Person.IdentityAssuranceLevel.LEVEL3); 035 break; 036 case LEVEL4: 037 tgt.setValue(Person.IdentityAssuranceLevel.LEVEL4); 038 break; 039 default: 040 tgt.setValue(Person.IdentityAssuranceLevel.NULL); 041 break; 042 } 043 } 044 return tgt; 045 } 046 047 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Person.IdentityAssuranceLevel> convertIdentityAssuranceLevel(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Person.IdentityAssuranceLevel> src) throws FHIRException { 048 if (src == null || src.isEmpty()) 049 return null; 050 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Person.IdentityAssuranceLevel> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Person.IdentityAssuranceLevelEnumFactory()); 051 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 052 if (src.getValue() == null) { 053 tgt.setValue(null); 054 } else { 055 switch (src.getValue()) { 056 case LEVEL1: 057 tgt.setValue(org.hl7.fhir.r5.model.Person.IdentityAssuranceLevel.LEVEL1); 058 break; 059 case LEVEL2: 060 tgt.setValue(org.hl7.fhir.r5.model.Person.IdentityAssuranceLevel.LEVEL2); 061 break; 062 case LEVEL3: 063 tgt.setValue(org.hl7.fhir.r5.model.Person.IdentityAssuranceLevel.LEVEL3); 064 break; 065 case LEVEL4: 066 tgt.setValue(org.hl7.fhir.r5.model.Person.IdentityAssuranceLevel.LEVEL4); 067 break; 068 default: 069 tgt.setValue(org.hl7.fhir.r5.model.Person.IdentityAssuranceLevel.NULL); 070 break; 071 } 072 } 073 return tgt; 074 } 075 076 public static org.hl7.fhir.dstu3.model.Person convertPerson(org.hl7.fhir.r5.model.Person src) throws FHIRException { 077 if (src == null) 078 return null; 079 org.hl7.fhir.dstu3.model.Person tgt = new org.hl7.fhir.dstu3.model.Person(); 080 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 081 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 082 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 083 for (org.hl7.fhir.r5.model.HumanName t : src.getName()) tgt.addName(HumanName30_50.convertHumanName(t)); 084 for (org.hl7.fhir.r5.model.ContactPoint t : src.getTelecom()) 085 tgt.addTelecom(ContactPoint30_50.convertContactPoint(t)); 086 if (src.hasGender()) 087 tgt.setGenderElement(Enumerations30_50.convertAdministrativeGender(src.getGenderElement())); 088 if (src.hasBirthDate()) 089 tgt.setBirthDateElement(Date30_50.convertDate(src.getBirthDateElement())); 090 for (org.hl7.fhir.r5.model.Address t : src.getAddress()) tgt.addAddress(Address30_50.convertAddress(t)); 091 if (src.hasPhoto()) 092 tgt.setPhoto(Attachment30_50.convertAttachment(src.getPhotoFirstRep())); 093 if (src.hasManagingOrganization()) 094 tgt.setManagingOrganization(Reference30_50.convertReference(src.getManagingOrganization())); 095 if (src.hasActive()) 096 tgt.setActiveElement(Boolean30_50.convertBoolean(src.getActiveElement())); 097 for (org.hl7.fhir.r5.model.Person.PersonLinkComponent t : src.getLink()) tgt.addLink(convertPersonLinkComponent(t)); 098 return tgt; 099 } 100 101 public static org.hl7.fhir.r5.model.Person convertPerson(org.hl7.fhir.dstu3.model.Person src) throws FHIRException { 102 if (src == null) 103 return null; 104 org.hl7.fhir.r5.model.Person tgt = new org.hl7.fhir.r5.model.Person(); 105 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 106 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 107 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 108 for (org.hl7.fhir.dstu3.model.HumanName t : src.getName()) tgt.addName(HumanName30_50.convertHumanName(t)); 109 for (org.hl7.fhir.dstu3.model.ContactPoint t : src.getTelecom()) 110 tgt.addTelecom(ContactPoint30_50.convertContactPoint(t)); 111 if (src.hasGender()) 112 tgt.setGenderElement(Enumerations30_50.convertAdministrativeGender(src.getGenderElement())); 113 if (src.hasBirthDate()) 114 tgt.setBirthDateElement(Date30_50.convertDate(src.getBirthDateElement())); 115 for (org.hl7.fhir.dstu3.model.Address t : src.getAddress()) tgt.addAddress(Address30_50.convertAddress(t)); 116 if (src.hasPhoto()) 117 tgt.addPhoto(Attachment30_50.convertAttachment(src.getPhoto())); 118 if (src.hasManagingOrganization()) 119 tgt.setManagingOrganization(Reference30_50.convertReference(src.getManagingOrganization())); 120 if (src.hasActive()) 121 tgt.setActiveElement(Boolean30_50.convertBoolean(src.getActiveElement())); 122 for (org.hl7.fhir.dstu3.model.Person.PersonLinkComponent t : src.getLink()) 123 tgt.addLink(convertPersonLinkComponent(t)); 124 return tgt; 125 } 126 127 public static org.hl7.fhir.dstu3.model.Person.PersonLinkComponent convertPersonLinkComponent(org.hl7.fhir.r5.model.Person.PersonLinkComponent src) throws FHIRException { 128 if (src == null) 129 return null; 130 org.hl7.fhir.dstu3.model.Person.PersonLinkComponent tgt = new org.hl7.fhir.dstu3.model.Person.PersonLinkComponent(); 131 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 132 if (src.hasTarget()) 133 tgt.setTarget(Reference30_50.convertReference(src.getTarget())); 134 if (src.hasAssurance()) 135 tgt.setAssuranceElement(convertIdentityAssuranceLevel(src.getAssuranceElement())); 136 return tgt; 137 } 138 139 public static org.hl7.fhir.r5.model.Person.PersonLinkComponent convertPersonLinkComponent(org.hl7.fhir.dstu3.model.Person.PersonLinkComponent src) throws FHIRException { 140 if (src == null) 141 return null; 142 org.hl7.fhir.r5.model.Person.PersonLinkComponent tgt = new org.hl7.fhir.r5.model.Person.PersonLinkComponent(); 143 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 144 if (src.hasTarget()) 145 tgt.setTarget(Reference30_50.convertReference(src.getTarget())); 146 if (src.hasAssurance()) 147 tgt.setAssuranceElement(convertIdentityAssuranceLevel(src.getAssuranceElement())); 148 return tgt; 149 } 150}