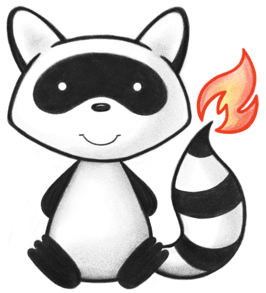
001package org.hl7.fhir.convertors.conv30_50.resources30_50; 002 003 004import org.hl7.fhir.convertors.context.ConversionContext30_50; 005import org.hl7.fhir.convertors.conv30_50.datatypes30_50.Reference30_50; 006import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.CodeableConcept30_50; 007import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Coding30_50; 008import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Period30_50; 009import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Signature30_50; 010import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Instant30_50; 011import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Uri30_50; 012import org.hl7.fhir.exceptions.FHIRException; 013import org.hl7.fhir.r5.model.CodeableReference; 014import org.hl7.fhir.r5.model.Enumeration; 015import org.hl7.fhir.r5.model.Provenance; 016 017/* 018 Copyright (c) 2011+, HL7, Inc. 019 All rights reserved. 020 021 Redistribution and use in source and binary forms, with or without modification, 022 are permitted provided that the following conditions are met: 023 024 * Redistributions of source code must retain the above copyright notice, this 025 list of conditions and the following disclaimer. 026 * Redistributions in binary form must reproduce the above copyright notice, 027 this list of conditions and the following disclaimer in the documentation 028 and/or other materials provided with the distribution. 029 * Neither the name of HL7 nor the names of its contributors may be used to 030 endorse or promote products derived from this software without specific 031 prior written permission. 032 033 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 034 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 035 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 036 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 037 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 038 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 039 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 040 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 041 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 042 POSSIBILITY OF SUCH DAMAGE. 043 044*/ 045// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 046public class Provenance30_50 { 047 048 public static org.hl7.fhir.r5.model.Provenance convertProvenance(org.hl7.fhir.dstu3.model.Provenance src) throws FHIRException { 049 if (src == null) 050 return null; 051 org.hl7.fhir.r5.model.Provenance tgt = new org.hl7.fhir.r5.model.Provenance(); 052 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 053 for (org.hl7.fhir.dstu3.model.Reference t : src.getTarget()) tgt.addTarget(Reference30_50.convertReference(t)); 054 if (src.hasPeriod()) 055 tgt.setOccurred(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getPeriod())); 056 if (src.hasRecorded()) 057 tgt.setRecordedElement(Instant30_50.convertInstant(src.getRecordedElement())); 058 for (org.hl7.fhir.dstu3.model.UriType t : src.getPolicy()) tgt.getPolicy().add(Uri30_50.convertUri(t)); 059 if (src.hasLocation()) 060 tgt.setLocation(Reference30_50.convertReference(src.getLocation())); 061 for (org.hl7.fhir.dstu3.model.Coding t : src.getReason()) 062 tgt.addAuthorization().getConcept().addCoding(Coding30_50.convertCoding(t)); 063 if (src.hasActivity()) 064 tgt.getActivity().addCoding(Coding30_50.convertCoding(src.getActivity())); 065 for (org.hl7.fhir.dstu3.model.Provenance.ProvenanceAgentComponent t : src.getAgent()) 066 tgt.addAgent(convertProvenanceAgentComponent(t)); 067 for (org.hl7.fhir.dstu3.model.Provenance.ProvenanceEntityComponent t : src.getEntity()) 068 tgt.addEntity(convertProvenanceEntityComponent(t)); 069 for (org.hl7.fhir.dstu3.model.Signature t : src.getSignature()) 070 tgt.addSignature(Signature30_50.convertSignature(t)); 071 return tgt; 072 } 073 074 public static org.hl7.fhir.dstu3.model.Provenance convertProvenance(org.hl7.fhir.r5.model.Provenance src) throws FHIRException { 075 if (src == null) 076 return null; 077 org.hl7.fhir.dstu3.model.Provenance tgt = new org.hl7.fhir.dstu3.model.Provenance(); 078 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 079 for (org.hl7.fhir.r5.model.Reference t : src.getTarget()) tgt.addTarget(Reference30_50.convertReference(t)); 080 if (src.hasOccurredPeriod()) 081 tgt.setPeriod(Period30_50.convertPeriod(src.getOccurredPeriod())); 082 if (src.hasRecorded()) 083 tgt.setRecordedElement(Instant30_50.convertInstant(src.getRecordedElement())); 084 for (org.hl7.fhir.r5.model.UriType t : src.getPolicy()) tgt.getPolicy().add(Uri30_50.convertUri(t)); 085 if (src.hasLocation()) 086 tgt.setLocation(Reference30_50.convertReference(src.getLocation())); 087 for (CodeableReference t : src.getAuthorization()) 088 if (t.hasConcept()) 089 for (org.hl7.fhir.r5.model.Coding t2 : t.getConcept().getCoding()) 090 tgt.addReason(Coding30_50.convertCoding(t2)); 091 if (src.hasActivity()) 092 tgt.setActivity(Coding30_50.convertCoding(src.getActivity().getCodingFirstRep())); 093 for (org.hl7.fhir.r5.model.Provenance.ProvenanceAgentComponent t : src.getAgent()) 094 tgt.addAgent(convertProvenanceAgentComponent(t)); 095 for (org.hl7.fhir.r5.model.Provenance.ProvenanceEntityComponent t : src.getEntity()) 096 tgt.addEntity(convertProvenanceEntityComponent(t)); 097 for (org.hl7.fhir.r5.model.Signature t : src.getSignature()) tgt.addSignature(Signature30_50.convertSignature(t)); 098 return tgt; 099 } 100 101 public static org.hl7.fhir.r5.model.Provenance.ProvenanceAgentComponent convertProvenanceAgentComponent(org.hl7.fhir.dstu3.model.Provenance.ProvenanceAgentComponent src) throws FHIRException { 102 if (src == null) 103 return null; 104 org.hl7.fhir.r5.model.Provenance.ProvenanceAgentComponent tgt = new org.hl7.fhir.r5.model.Provenance.ProvenanceAgentComponent(); 105 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 106 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getRole()) 107 tgt.addRole(CodeableConcept30_50.convertCodeableConcept(t)); 108 if (src.hasWhoReference()) 109 tgt.setWho(Reference30_50.convertReference(src.getWhoReference())); 110 if (src.hasOnBehalfOfReference()) 111 tgt.setOnBehalfOf(Reference30_50.convertReference(src.getOnBehalfOfReference())); 112 return tgt; 113 } 114 115 public static org.hl7.fhir.dstu3.model.Provenance.ProvenanceAgentComponent convertProvenanceAgentComponent(org.hl7.fhir.r5.model.Provenance.ProvenanceAgentComponent src) throws FHIRException { 116 if (src == null) 117 return null; 118 org.hl7.fhir.dstu3.model.Provenance.ProvenanceAgentComponent tgt = new org.hl7.fhir.dstu3.model.Provenance.ProvenanceAgentComponent(); 119 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 120 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getRole()) 121 tgt.addRole(CodeableConcept30_50.convertCodeableConcept(t)); 122 if (src.hasWho()) 123 tgt.setWho(Reference30_50.convertReference(src.getWho())); 124 if (src.hasOnBehalfOf()) 125 tgt.setOnBehalfOf(Reference30_50.convertReference(src.getOnBehalfOf())); 126 return tgt; 127 } 128 129 public static org.hl7.fhir.r5.model.Provenance.ProvenanceEntityComponent convertProvenanceEntityComponent(org.hl7.fhir.dstu3.model.Provenance.ProvenanceEntityComponent src) throws FHIRException { 130 if (src == null) 131 return null; 132 org.hl7.fhir.r5.model.Provenance.ProvenanceEntityComponent tgt = new org.hl7.fhir.r5.model.Provenance.ProvenanceEntityComponent(); 133 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 134 if (src.hasRole()) 135 tgt.setRoleElement(convertProvenanceEntityRole(src.getRoleElement())); 136 if (src.hasWhatReference()) 137 tgt.setWhat(Reference30_50.convertReference(src.getWhatReference())); 138 for (org.hl7.fhir.dstu3.model.Provenance.ProvenanceAgentComponent t : src.getAgent()) 139 tgt.addAgent(convertProvenanceAgentComponent(t)); 140 return tgt; 141 } 142 143 public static org.hl7.fhir.dstu3.model.Provenance.ProvenanceEntityComponent convertProvenanceEntityComponent(org.hl7.fhir.r5.model.Provenance.ProvenanceEntityComponent src) throws FHIRException { 144 if (src == null) 145 return null; 146 org.hl7.fhir.dstu3.model.Provenance.ProvenanceEntityComponent tgt = new org.hl7.fhir.dstu3.model.Provenance.ProvenanceEntityComponent(); 147 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 148 if (src.hasRole()) 149 tgt.setRoleElement(convertProvenanceEntityRole(src.getRoleElement())); 150 if (src.hasWhat()) 151 tgt.setWhat(Reference30_50.convertReference(src.getWhat())); 152 for (org.hl7.fhir.r5.model.Provenance.ProvenanceAgentComponent t : src.getAgent()) 153 tgt.addAgent(convertProvenanceAgentComponent(t)); 154 return tgt; 155 } 156 157 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Provenance.ProvenanceEntityRole> convertProvenanceEntityRole(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Provenance.ProvenanceEntityRole> src) throws FHIRException { 158 if (src == null || src.isEmpty()) 159 return null; 160 Enumeration<Provenance.ProvenanceEntityRole> tgt = new Enumeration<>(new Provenance.ProvenanceEntityRoleEnumFactory()); 161 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 162 if (src.getValue() == null) { 163 tgt.setValue(null); 164 } else { 165 switch (src.getValue()) { 166 case DERIVATION: 167 tgt.setValue(Provenance.ProvenanceEntityRole.INSTANTIATES); 168 break; 169 case REVISION: 170 tgt.setValue(Provenance.ProvenanceEntityRole.REVISION); 171 break; 172 case QUOTATION: 173 tgt.setValue(Provenance.ProvenanceEntityRole.QUOTATION); 174 break; 175 case SOURCE: 176 tgt.setValue(Provenance.ProvenanceEntityRole.SOURCE); 177 break; 178 case REMOVAL: 179 tgt.setValue(Provenance.ProvenanceEntityRole.REMOVAL); 180 break; 181 default: 182 tgt.setValue(Provenance.ProvenanceEntityRole.NULL); 183 break; 184 } 185 } 186 return tgt; 187 } 188 189 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Provenance.ProvenanceEntityRole> convertProvenanceEntityRole(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Provenance.ProvenanceEntityRole> src) throws FHIRException { 190 if (src == null || src.isEmpty()) 191 return null; 192 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Provenance.ProvenanceEntityRole> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Provenance.ProvenanceEntityRoleEnumFactory()); 193 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 194 if (src.getValue() == null) { 195 tgt.setValue(null); 196 } else { 197 switch (src.getValue()) { 198 case INSTANTIATES: 199 tgt.setValue(org.hl7.fhir.dstu3.model.Provenance.ProvenanceEntityRole.DERIVATION); 200 break; 201 case REVISION: 202 tgt.setValue(org.hl7.fhir.dstu3.model.Provenance.ProvenanceEntityRole.REVISION); 203 break; 204 case QUOTATION: 205 tgt.setValue(org.hl7.fhir.dstu3.model.Provenance.ProvenanceEntityRole.QUOTATION); 206 break; 207 case SOURCE: 208 tgt.setValue(org.hl7.fhir.dstu3.model.Provenance.ProvenanceEntityRole.SOURCE); 209 break; 210 case REMOVAL: 211 tgt.setValue(org.hl7.fhir.dstu3.model.Provenance.ProvenanceEntityRole.REMOVAL); 212 break; 213 default: 214 tgt.setValue(org.hl7.fhir.dstu3.model.Provenance.ProvenanceEntityRole.NULL); 215 break; 216 } 217 } 218 return tgt; 219 } 220}