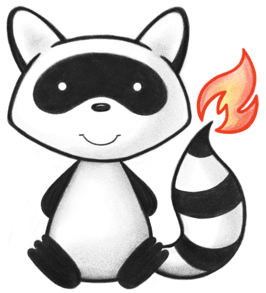
001package org.hl7.fhir.convertors.conv30_50.resources30_50; 002 003import org.hl7.fhir.convertors.VersionConvertorConstants; 004import org.hl7.fhir.convertors.context.ConversionContext30_50; 005import org.hl7.fhir.convertors.conv30_50.datatypes30_50.ContactDetail30_50; 006import org.hl7.fhir.convertors.conv30_50.datatypes30_50.Extension30_50; 007import org.hl7.fhir.convertors.conv30_50.datatypes30_50.Reference30_50; 008import org.hl7.fhir.convertors.conv30_50.datatypes30_50.UsageContext30_50; 009import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.CodeableConcept30_50; 010import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Coding30_50; 011import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Identifier30_50; 012import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Period30_50; 013import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Boolean30_50; 014import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Date30_50; 015import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.DateTime30_50; 016import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Integer30_50; 017import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.MarkDown30_50; 018import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.String30_50; 019import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Uri30_50; 020import org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType; 021import org.hl7.fhir.exceptions.FHIRException; 022import org.hl7.fhir.r5.model.CodeType; 023import org.hl7.fhir.r5.model.Enumeration; 024import org.hl7.fhir.r5.model.Questionnaire; 025import org.hl7.fhir.r5.model.Questionnaire.QuestionnaireAnswerConstraint; 026 027public class Questionnaire30_50 { 028 029 public static org.hl7.fhir.r5.model.Questionnaire convertQuestionnaire(org.hl7.fhir.dstu3.model.Questionnaire src) throws FHIRException { 030 if (src == null) 031 return null; 032 org.hl7.fhir.r5.model.Questionnaire tgt = new org.hl7.fhir.r5.model.Questionnaire(); 033 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 034 if (src.hasUrl()) 035 tgt.setUrlElement(Uri30_50.convertUri(src.getUrlElement())); 036 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 037 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 038 if (src.hasVersion()) 039 tgt.setVersionElement(String30_50.convertString(src.getVersionElement())); 040 if (src.hasName()) 041 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 042 if (src.hasTitle()) 043 tgt.setTitleElement(String30_50.convertString(src.getTitleElement())); 044 if (src.hasStatus()) 045 tgt.setStatusElement(Enumerations30_50.convertPublicationStatus(src.getStatusElement())); 046 if (src.hasExperimental()) 047 tgt.setExperimentalElement(Boolean30_50.convertBoolean(src.getExperimentalElement())); 048 if (src.hasDate()) 049 tgt.setDateElement(DateTime30_50.convertDateTime(src.getDateElement())); 050 if (src.hasPublisher()) 051 tgt.setPublisherElement(String30_50.convertString(src.getPublisherElement())); 052 if (src.hasDescription()) 053 tgt.setDescriptionElement(MarkDown30_50.convertMarkdown(src.getDescriptionElement())); 054 if (src.hasPurpose()) 055 tgt.setPurposeElement(MarkDown30_50.convertMarkdown(src.getPurposeElement())); 056 if (src.hasApprovalDate()) 057 tgt.setApprovalDateElement(Date30_50.convertDate(src.getApprovalDateElement())); 058 if (src.hasLastReviewDate()) 059 tgt.setLastReviewDateElement(Date30_50.convertDate(src.getLastReviewDateElement())); 060 if (src.hasEffectivePeriod()) 061 tgt.setEffectivePeriod(Period30_50.convertPeriod(src.getEffectivePeriod())); 062 for (org.hl7.fhir.dstu3.model.UsageContext t : src.getUseContext()) 063 tgt.addUseContext(UsageContext30_50.convertUsageContext(t)); 064 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getJurisdiction()) 065 tgt.addJurisdiction(CodeableConcept30_50.convertCodeableConcept(t)); 066 for (org.hl7.fhir.dstu3.model.ContactDetail t : src.getContact()) 067 tgt.addContact(ContactDetail30_50.convertContactDetail(t)); 068 if (src.hasCopyright()) 069 tgt.setCopyrightElement(MarkDown30_50.convertMarkdown(src.getCopyrightElement())); 070 for (org.hl7.fhir.dstu3.model.Coding t : src.getCode()) tgt.addCode(Coding30_50.convertCoding(t)); 071 for (org.hl7.fhir.dstu3.model.CodeType t : src.getSubjectType()) tgt.addSubjectType(t.getValue()); 072 for (org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemComponent t : src.getItem()) 073 tgt.addItem(convertQuestionnaireItemComponent(t)); 074 return tgt; 075 } 076 077 public static org.hl7.fhir.dstu3.model.Questionnaire convertQuestionnaire(org.hl7.fhir.r5.model.Questionnaire src) throws FHIRException { 078 if (src == null) 079 return null; 080 org.hl7.fhir.dstu3.model.Questionnaire tgt = new org.hl7.fhir.dstu3.model.Questionnaire(); 081 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 082 if (src.hasUrl()) 083 tgt.setUrlElement(Uri30_50.convertUri(src.getUrlElement())); 084 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 085 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 086 if (src.hasVersion()) 087 tgt.setVersionElement(String30_50.convertString(src.getVersionElement())); 088 if (src.hasName()) 089 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 090 if (src.hasTitle()) 091 tgt.setTitleElement(String30_50.convertString(src.getTitleElement())); 092 if (src.hasStatus()) 093 tgt.setStatusElement(Enumerations30_50.convertPublicationStatus(src.getStatusElement())); 094 if (src.hasExperimental()) 095 tgt.setExperimentalElement(Boolean30_50.convertBoolean(src.getExperimentalElement())); 096 if (src.hasDate()) 097 tgt.setDateElement(DateTime30_50.convertDateTime(src.getDateElement())); 098 if (src.hasPublisher()) 099 tgt.setPublisherElement(String30_50.convertString(src.getPublisherElement())); 100 if (src.hasDescription()) 101 tgt.setDescriptionElement(MarkDown30_50.convertMarkdown(src.getDescriptionElement())); 102 if (src.hasPurpose()) 103 tgt.setPurposeElement(MarkDown30_50.convertMarkdown(src.getPurposeElement())); 104 if (src.hasApprovalDate()) 105 tgt.setApprovalDateElement(Date30_50.convertDate(src.getApprovalDateElement())); 106 if (src.hasLastReviewDate()) 107 tgt.setLastReviewDateElement(Date30_50.convertDate(src.getLastReviewDateElement())); 108 if (src.hasEffectivePeriod()) 109 tgt.setEffectivePeriod(Period30_50.convertPeriod(src.getEffectivePeriod())); 110 for (org.hl7.fhir.r5.model.UsageContext t : src.getUseContext()) 111 tgt.addUseContext(UsageContext30_50.convertUsageContext(t)); 112 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getJurisdiction()) 113 tgt.addJurisdiction(CodeableConcept30_50.convertCodeableConcept(t)); 114 for (org.hl7.fhir.r5.model.ContactDetail t : src.getContact()) 115 tgt.addContact(ContactDetail30_50.convertContactDetail(t)); 116 if (src.hasCopyright()) 117 tgt.setCopyrightElement(MarkDown30_50.convertMarkdown(src.getCopyrightElement())); 118 for (org.hl7.fhir.r5.model.Coding t : src.getCode()) tgt.addCode(Coding30_50.convertCoding(t)); 119 for (org.hl7.fhir.r5.model.CodeType t : src.getSubjectType()) tgt.addSubjectType(t.getValue()); 120 for (org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemComponent t : src.getItem()) 121 tgt.addItem(convertQuestionnaireItemComponent(t)); 122 return tgt; 123 } 124 125 public static org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemComponent convertQuestionnaireItemComponent(org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemComponent src) throws FHIRException { 126 if (src == null) 127 return null; 128 org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemComponent tgt = new org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemComponent(); 129 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 130 if (src.hasLinkId()) 131 tgt.setLinkIdElement(String30_50.convertString(src.getLinkIdElement())); 132 if (src.hasDefinition()) 133 tgt.setDefinitionElement(Uri30_50.convertUri(src.getDefinitionElement())); 134 for (org.hl7.fhir.r5.model.Coding t : src.getCode()) tgt.addCode(Coding30_50.convertCoding(t)); 135 if (src.hasPrefix()) 136 tgt.setPrefixElement(String30_50.convertString(src.getPrefixElement())); 137 if (src.hasText()) 138 tgt.setTextElement(String30_50.convertString(src.getTextElement())); 139 if (src.hasType()) 140 tgt.setTypeElement(convertQuestionnaireItemType(src.getTypeElement(), src.getAnswerConstraint())); 141 for (org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemEnableWhenComponent t : src.getEnableWhen()) 142 tgt.addEnableWhen(convertQuestionnaireItemEnableWhenComponent(t)); 143 if (src.hasRequired()) 144 tgt.setRequiredElement(Boolean30_50.convertBoolean(src.getRequiredElement())); 145 if (src.hasRepeats()) 146 tgt.setRepeatsElement(Boolean30_50.convertBoolean(src.getRepeatsElement())); 147 if (src.hasReadOnly()) 148 tgt.setReadOnlyElement(Boolean30_50.convertBoolean(src.getReadOnlyElement())); 149 if (src.hasMaxLength()) 150 tgt.setMaxLengthElement(Integer30_50.convertInteger(src.getMaxLengthElement())); 151 if (src.hasAnswerValueSet()) 152 tgt.setOptions(Reference30_50.convertCanonicalToReference(src.getAnswerValueSetElement())); 153 for (org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemAnswerOptionComponent t : src.getAnswerOption()) 154 tgt.addOption(convertQuestionnaireItemOptionComponent(t)); 155 if (src.hasInitial()) 156 tgt.setInitial(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getInitialFirstRep().getValue())); 157 for (org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemComponent t : src.getItem()) 158 tgt.addItem(convertQuestionnaireItemComponent(t)); 159 return tgt; 160 } 161 162 public static org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemComponent convertQuestionnaireItemComponent(org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemComponent src) throws FHIRException { 163 if (src == null) 164 return null; 165 org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemComponent tgt = new org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemComponent(); 166 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 167 if (src.hasLinkId()) 168 tgt.setLinkIdElement(String30_50.convertString(src.getLinkIdElement())); 169 if (src.hasDefinition()) 170 tgt.setDefinitionElement(Uri30_50.convertUri(src.getDefinitionElement())); 171 for (org.hl7.fhir.dstu3.model.Coding t : src.getCode()) tgt.addCode(Coding30_50.convertCoding(t)); 172 if (src.hasPrefix()) 173 tgt.setPrefixElement(String30_50.convertString(src.getPrefixElement())); 174 if (src.hasText()) 175 tgt.setTextElement(String30_50.convertStringToMarkdown(src.getTextElement())); 176 if (src.hasType()) { 177 tgt.setTypeElement(convertQuestionnaireItemType(src.getTypeElement())); 178 if (src.getType() == QuestionnaireItemType.CHOICE) { 179 tgt.setAnswerConstraint(QuestionnaireAnswerConstraint.OPTIONSONLY); 180 } else if (src.getType() == QuestionnaireItemType.OPENCHOICE) { 181 tgt.setAnswerConstraint(QuestionnaireAnswerConstraint.OPTIONSORSTRING); 182 } 183 } 184 for (org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemEnableWhenComponent t : src.getEnableWhen()) 185 tgt.addEnableWhen(convertQuestionnaireItemEnableWhenComponent(t)); 186 tgt.setEnableBehavior(Questionnaire.EnableWhenBehavior.ANY); 187 if (src.hasRequired()) 188 tgt.setRequiredElement(Boolean30_50.convertBoolean(src.getRequiredElement())); 189 if (src.hasRepeats()) 190 tgt.setRepeatsElement(Boolean30_50.convertBoolean(src.getRepeatsElement())); 191 if (src.hasReadOnly()) 192 tgt.setReadOnlyElement(Boolean30_50.convertBoolean(src.getReadOnlyElement())); 193 if (src.hasMaxLength()) 194 tgt.setMaxLengthElement(Integer30_50.convertInteger(src.getMaxLengthElement())); 195 if (src.hasOptions()) 196 tgt.setAnswerValueSetElement(Reference30_50.convertReferenceToCanonical(src.getOptions())); 197 for (org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemOptionComponent t : src.getOption()) 198 tgt.addAnswerOption(convertQuestionnaireItemOptionComponent(t)); 199 if (src.hasInitial()) 200 tgt.addInitial().setValue(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getInitial())); 201 for (org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemComponent t : src.getItem()) 202 tgt.addItem(convertQuestionnaireItemComponent(t)); 203 for (org.hl7.fhir.dstu3.model.Extension t : src.getModifierExtension()) { 204 tgt.addModifierExtension(Extension30_50.convertExtension(t)); 205 } 206 return tgt; 207 } 208 209 public static org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemEnableWhenComponent convertQuestionnaireItemEnableWhenComponent(org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemEnableWhenComponent src) throws FHIRException { 210 if (src == null) 211 return null; 212 org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemEnableWhenComponent tgt = new org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemEnableWhenComponent(); 213 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 214 if (src.hasQuestion()) 215 tgt.setQuestionElement(String30_50.convertString(src.getQuestionElement())); 216 if (src.hasOperator() && src.getOperator() == org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemOperator.EXISTS) 217 tgt.setHasAnswer(src.getAnswerBooleanType().getValue()); 218 else if (src.hasAnswer()) 219 tgt.setAnswer(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getAnswer())); 220 return tgt; 221 } 222 223 public static org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemEnableWhenComponent convertQuestionnaireItemEnableWhenComponent(org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemEnableWhenComponent src) throws FHIRException { 224 if (src == null) 225 return null; 226 org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemEnableWhenComponent tgt = new org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemEnableWhenComponent(); 227 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 228 if (src.hasQuestion()) 229 tgt.setQuestionElement(String30_50.convertString(src.getQuestionElement())); 230 if (src.hasHasAnswer()) { 231 tgt.setOperator(org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemOperator.EXISTS); 232 if (src.hasHasAnswerElement()) 233 tgt.setAnswer(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getHasAnswerElement())); 234 } else if (src.hasAnswer()) { 235 tgt.setOperator(org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemOperator.EQUAL); 236 if (src.hasAnswer()) 237 tgt.setAnswer(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getAnswer())); 238 } 239 return tgt; 240 } 241 242 public static org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemAnswerOptionComponent convertQuestionnaireItemOptionComponent(org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemOptionComponent src) throws FHIRException { 243 if (src == null) 244 return null; 245 org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemAnswerOptionComponent tgt = new org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemAnswerOptionComponent(); 246 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 247 if (src.hasValue()) 248 tgt.setValue(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getValue())); 249 return tgt; 250 } 251 252 public static org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemOptionComponent convertQuestionnaireItemOptionComponent(org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemAnswerOptionComponent src) throws FHIRException { 253 if (src == null) 254 return null; 255 org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemOptionComponent tgt = new org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemOptionComponent(); 256 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 257 if (src.hasValue()) 258 tgt.setValue(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getValue())); 259 return tgt; 260 } 261 262 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType> convertQuestionnaireItemType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemType> src, QuestionnaireAnswerConstraint constraint) throws FHIRException { 263 if (src == null || src.isEmpty()) 264 return null; 265 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemTypeEnumFactory()); 266 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt, VersionConvertorConstants.EXT_QUESTIONNAIRE_ITEM_TYPE_ORIGINAL); 267 if (src.hasExtension(VersionConvertorConstants.EXT_QUESTIONNAIRE_ITEM_TYPE_ORIGINAL)) { 268 tgt.setValueAsString(src.getExtensionString(VersionConvertorConstants.EXT_QUESTIONNAIRE_ITEM_TYPE_ORIGINAL)); 269 } else { 270 if (src.getValue() == null) { 271 tgt.setValue(null); 272 } else { 273 switch (src.getValue()) { 274 case GROUP: 275 tgt.setValue(QuestionnaireItemType.GROUP); 276 break; 277 case DISPLAY: 278 tgt.setValue(QuestionnaireItemType.DISPLAY); 279 break; 280 case BOOLEAN: 281 tgt.setValue(QuestionnaireItemType.BOOLEAN); 282 break; 283 case DECIMAL: 284 tgt.setValue(QuestionnaireItemType.DECIMAL); 285 break; 286 case INTEGER: 287 tgt.setValue(QuestionnaireItemType.INTEGER); 288 break; 289 case DATE: 290 tgt.setValue(QuestionnaireItemType.DATE); 291 break; 292 case DATETIME: 293 tgt.setValue(QuestionnaireItemType.DATETIME); 294 break; 295 case TIME: 296 tgt.setValue(QuestionnaireItemType.TIME); 297 break; 298 case STRING: 299 tgt.setValue(QuestionnaireItemType.STRING); 300 break; 301 case TEXT: 302 tgt.setValue(QuestionnaireItemType.TEXT); 303 break; 304 case URL: 305 tgt.setValue(QuestionnaireItemType.URL); 306 break; 307 case CODING: 308 if (constraint == QuestionnaireAnswerConstraint.OPTIONSORSTRING) 309 tgt.setValue(QuestionnaireItemType.OPENCHOICE); 310 else 311 tgt.setValue(QuestionnaireItemType.CHOICE); 312 break; 313 case ATTACHMENT: 314 tgt.setValue(QuestionnaireItemType.ATTACHMENT); 315 break; 316 case REFERENCE: 317 tgt.setValue(QuestionnaireItemType.REFERENCE); 318 break; 319 case QUANTITY: 320 tgt.setValue(QuestionnaireItemType.QUANTITY); 321 break; 322 default: 323 tgt.setValue(QuestionnaireItemType.NULL); 324 break; 325 } 326 } 327 } 328 return tgt; 329 } 330 331 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemType> convertQuestionnaireItemType(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType> src) throws FHIRException { 332 if (src == null || src.isEmpty()) 333 return null; 334 Enumeration<Questionnaire.QuestionnaireItemType> tgt = new Enumeration<>(new Questionnaire.QuestionnaireItemTypeEnumFactory()); 335 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 336 tgt.addExtension(VersionConvertorConstants.EXT_QUESTIONNAIRE_ITEM_TYPE_ORIGINAL, new CodeType(src.getValueAsString())); 337 if (src.getValue() == null) { 338 tgt.setValue(null); 339 } else { 340 switch (src.getValue()) { 341 case GROUP: 342 tgt.setValue(Questionnaire.QuestionnaireItemType.GROUP); 343 break; 344 case DISPLAY: 345 tgt.setValue(Questionnaire.QuestionnaireItemType.DISPLAY); 346 break; 347 case BOOLEAN: 348 tgt.setValue(Questionnaire.QuestionnaireItemType.BOOLEAN); 349 break; 350 case DECIMAL: 351 tgt.setValue(Questionnaire.QuestionnaireItemType.DECIMAL); 352 break; 353 case INTEGER: 354 tgt.setValue(Questionnaire.QuestionnaireItemType.INTEGER); 355 break; 356 case DATE: 357 tgt.setValue(Questionnaire.QuestionnaireItemType.DATE); 358 break; 359 case DATETIME: 360 tgt.setValue(Questionnaire.QuestionnaireItemType.DATETIME); 361 break; 362 case TIME: 363 tgt.setValue(Questionnaire.QuestionnaireItemType.TIME); 364 break; 365 case STRING: 366 tgt.setValue(Questionnaire.QuestionnaireItemType.STRING); 367 break; 368 case TEXT: 369 tgt.setValue(Questionnaire.QuestionnaireItemType.TEXT); 370 break; 371 case URL: 372 tgt.setValue(Questionnaire.QuestionnaireItemType.URL); 373 break; 374 case CHOICE: 375 tgt.setValue(Questionnaire.QuestionnaireItemType.CODING); 376 break; 377 case OPENCHOICE: 378 tgt.setValue(Questionnaire.QuestionnaireItemType.CODING); 379 break; 380 case ATTACHMENT: 381 tgt.setValue(Questionnaire.QuestionnaireItemType.ATTACHMENT); 382 break; 383 case REFERENCE: 384 tgt.setValue(Questionnaire.QuestionnaireItemType.REFERENCE); 385 break; 386 case QUANTITY: 387 tgt.setValue(Questionnaire.QuestionnaireItemType.QUANTITY); 388 break; 389 default: 390 tgt.setValue(Questionnaire.QuestionnaireItemType.NULL); 391 break; 392 } 393 } 394 return tgt; 395 } 396}