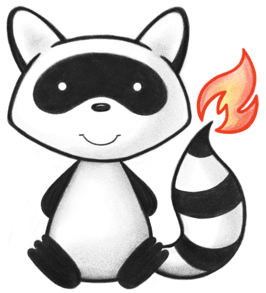
001package org.hl7.fhir.convertors.conv30_50.resources30_50; 002 003import java.util.List; 004 005import org.hl7.fhir.convertors.context.ConversionContext30_50; 006import org.hl7.fhir.convertors.conv30_50.datatypes30_50.Reference30_50; 007import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Address30_50; 008import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Attachment30_50; 009import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.CodeableConcept30_50; 010import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.ContactPoint30_50; 011import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.HumanName30_50; 012import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Identifier30_50; 013import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Period30_50; 014import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Boolean30_50; 015import org.hl7.fhir.exceptions.FHIRException; 016import org.hl7.fhir.r5.model.CodeableConcept; 017 018public class RelatedPerson30_50 { 019 020 public static org.hl7.fhir.dstu3.model.RelatedPerson convertRelatedPerson(org.hl7.fhir.r5.model.RelatedPerson src) throws FHIRException { 021 if (src == null) 022 return null; 023 org.hl7.fhir.dstu3.model.RelatedPerson tgt = new org.hl7.fhir.dstu3.model.RelatedPerson(); 024 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 025 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) { 026 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 027 } 028 if (src.hasActive()) { 029 if (src.hasActiveElement()) 030 tgt.setActiveElement(Boolean30_50.convertBoolean(src.getActiveElement())); 031 } 032 if (src.hasPatient()) { 033 if (src.hasPatient()) 034 tgt.setPatient(Reference30_50.convertReference(src.getPatient())); 035 } 036 List<CodeableConcept> relationships = src.getRelationship(); 037 if (relationships.size() > 0) { 038 tgt.setRelationship(CodeableConcept30_50.convertCodeableConcept(relationships.get(0))); 039 if (relationships.size() > 1) { 040 } 041 } 042 for (org.hl7.fhir.r5.model.HumanName t : src.getName()) { 043 tgt.addName(HumanName30_50.convertHumanName(t)); 044 } 045 for (org.hl7.fhir.r5.model.ContactPoint t : src.getTelecom()) { 046 tgt.addTelecom(ContactPoint30_50.convertContactPoint(t)); 047 } 048 if (src.hasGender()) { 049 tgt.setGenderElement(Enumerations30_50.convertAdministrativeGender(src.getGenderElement())); 050 } 051 if (src.hasBirthDate()) { 052 tgt.setBirthDate(tgt.getBirthDate()); 053 } 054 for (org.hl7.fhir.r5.model.Address t : src.getAddress()) { 055 tgt.addAddress(Address30_50.convertAddress(t)); 056 } 057 for (org.hl7.fhir.r5.model.Attachment t : src.getPhoto()) { 058 tgt.addPhoto(Attachment30_50.convertAttachment(t)); 059 } 060 if (src.hasPeriod()) { 061 if (src.hasPeriod()) 062 tgt.setPeriod(Period30_50.convertPeriod(src.getPeriod())); 063 } 064 return tgt; 065 } 066 067 public static org.hl7.fhir.r5.model.RelatedPerson convertRelatedPerson(org.hl7.fhir.dstu3.model.RelatedPerson src) throws FHIRException { 068 if (src == null) 069 return null; 070 org.hl7.fhir.r5.model.RelatedPerson tgt = new org.hl7.fhir.r5.model.RelatedPerson(); 071 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 072 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) { 073 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 074 } 075 if (src.hasActive()) { 076 if (src.hasActiveElement()) 077 tgt.setActiveElement(Boolean30_50.convertBoolean(src.getActiveElement())); 078 } 079 if (src.hasPatient()) { 080 if (src.hasPatient()) 081 tgt.setPatient(Reference30_50.convertReference(src.getPatient())); 082 } 083 if (src.hasRelationship()) { 084 if (src.hasRelationship()) 085 tgt.addRelationship(CodeableConcept30_50.convertCodeableConcept(src.getRelationship())); 086 } 087 for (org.hl7.fhir.dstu3.model.HumanName t : src.getName()) { 088 tgt.addName(HumanName30_50.convertHumanName(t)); 089 } 090 for (org.hl7.fhir.dstu3.model.ContactPoint t : src.getTelecom()) { 091 tgt.addTelecom(ContactPoint30_50.convertContactPoint(t)); 092 } 093 if (src.hasGender()) { 094 tgt.setGenderElement(Enumerations30_50.convertAdministrativeGender(src.getGenderElement())); 095 } 096 if (src.hasBirthDate()) { 097 tgt.setBirthDate(tgt.getBirthDate()); 098 } 099 for (org.hl7.fhir.dstu3.model.Address t : src.getAddress()) { 100 tgt.addAddress(Address30_50.convertAddress(t)); 101 } 102 for (org.hl7.fhir.dstu3.model.Attachment t : src.getPhoto()) { 103 tgt.addPhoto(Attachment30_50.convertAttachment(t)); 104 } 105 if (src.hasPeriod()) { 106 if (src.hasPeriod()) 107 tgt.setPeriod(Period30_50.convertPeriod(src.getPeriod())); 108 } 109 return tgt; 110 } 111}