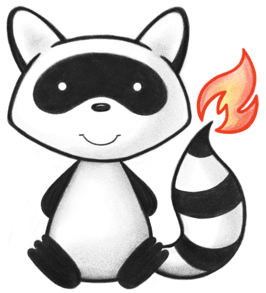
001package org.hl7.fhir.convertors.conv30_50.resources30_50; 002 003import java.util.stream.Collectors; 004 005import org.hl7.fhir.convertors.context.ConversionContext30_50; 006import org.hl7.fhir.convertors.conv30_50.datatypes30_50.ContactDetail30_50; 007import org.hl7.fhir.convertors.conv30_50.datatypes30_50.Reference30_50; 008import org.hl7.fhir.convertors.conv30_50.datatypes30_50.UsageContext30_50; 009import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.CodeableConcept30_50; 010import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Boolean30_50; 011import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Code30_50; 012import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.DateTime30_50; 013import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.MarkDown30_50; 014import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.String30_50; 015import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Uri30_50; 016import org.hl7.fhir.dstu3.model.SearchParameter; 017import org.hl7.fhir.exceptions.FHIRException; 018import org.hl7.fhir.r5.model.Enumeration; 019import org.hl7.fhir.r5.model.Enumerations; 020import org.hl7.fhir.r5.model.Enumerations.VersionIndependentResourceTypesAll; 021import org.hl7.fhir.r5.model.Enumerations.VersionIndependentResourceTypesAllEnumFactory; 022 023public class SearchParameter30_50 { 024 025 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.SearchComparator> convertSearchComparator(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.SearchParameter.SearchComparator> src) throws FHIRException { 026 if (src == null || src.isEmpty()) 027 return null; 028 Enumeration<Enumerations.SearchComparator> tgt = new Enumeration<>(new Enumerations.SearchComparatorEnumFactory()); 029 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 030 if (src.getValue() == null) { 031 tgt.setValue(null); 032 } else { 033 switch (src.getValue()) { 034 case EQ: 035 tgt.setValue(Enumerations.SearchComparator.EQ); 036 break; 037 case NE: 038 tgt.setValue(Enumerations.SearchComparator.NE); 039 break; 040 case GT: 041 tgt.setValue(Enumerations.SearchComparator.GT); 042 break; 043 case LT: 044 tgt.setValue(Enumerations.SearchComparator.LT); 045 break; 046 case GE: 047 tgt.setValue(Enumerations.SearchComparator.GE); 048 break; 049 case LE: 050 tgt.setValue(Enumerations.SearchComparator.LE); 051 break; 052 case SA: 053 tgt.setValue(Enumerations.SearchComparator.SA); 054 break; 055 case EB: 056 tgt.setValue(Enumerations.SearchComparator.EB); 057 break; 058 case AP: 059 tgt.setValue(Enumerations.SearchComparator.AP); 060 break; 061 default: 062 tgt.setValue(Enumerations.SearchComparator.NULL); 063 break; 064 } 065 } 066 return tgt; 067 } 068 069 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.SearchParameter.SearchComparator> convertSearchComparator(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.SearchComparator> src) throws FHIRException { 070 if (src == null || src.isEmpty()) 071 return null; 072 org.hl7.fhir.dstu3.model.Enumeration<SearchParameter.SearchComparator> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new SearchParameter.SearchComparatorEnumFactory()); 073 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 074 if (src.getValue() == null) { 075 tgt.setValue(null); 076 } else { 077 switch (src.getValue()) { 078 case EQ: 079 tgt.setValue(SearchParameter.SearchComparator.EQ); 080 break; 081 case NE: 082 tgt.setValue(SearchParameter.SearchComparator.NE); 083 break; 084 case GT: 085 tgt.setValue(SearchParameter.SearchComparator.GT); 086 break; 087 case LT: 088 tgt.setValue(SearchParameter.SearchComparator.LT); 089 break; 090 case GE: 091 tgt.setValue(SearchParameter.SearchComparator.GE); 092 break; 093 case LE: 094 tgt.setValue(SearchParameter.SearchComparator.LE); 095 break; 096 case SA: 097 tgt.setValue(SearchParameter.SearchComparator.SA); 098 break; 099 case EB: 100 tgt.setValue(SearchParameter.SearchComparator.EB); 101 break; 102 case AP: 103 tgt.setValue(SearchParameter.SearchComparator.AP); 104 break; 105 default: 106 tgt.setValue(SearchParameter.SearchComparator.NULL); 107 break; 108 } 109 } 110 return tgt; 111 } 112 113 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.SearchModifierCode> convertSearchModifierCode(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.SearchParameter.SearchModifierCode> src) throws FHIRException { 114 if (src == null || src.isEmpty()) 115 return null; 116 Enumeration<Enumerations.SearchModifierCode> tgt = new Enumeration<>(new Enumerations.SearchModifierCodeEnumFactory()); 117 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 118 if (src.getValue() == null) { 119 tgt.setValue(null); 120 } else { 121 switch (src.getValue()) { 122 case MISSING: 123 tgt.setValue(Enumerations.SearchModifierCode.MISSING); 124 break; 125 case EXACT: 126 tgt.setValue(Enumerations.SearchModifierCode.EXACT); 127 break; 128 case CONTAINS: 129 tgt.setValue(Enumerations.SearchModifierCode.CONTAINS); 130 break; 131 case NOT: 132 tgt.setValue(Enumerations.SearchModifierCode.NOT); 133 break; 134 case TEXT: 135 tgt.setValue(Enumerations.SearchModifierCode.TEXT); 136 break; 137 case IN: 138 tgt.setValue(Enumerations.SearchModifierCode.IN); 139 break; 140 case NOTIN: 141 tgt.setValue(Enumerations.SearchModifierCode.NOTIN); 142 break; 143 case BELOW: 144 tgt.setValue(Enumerations.SearchModifierCode.BELOW); 145 break; 146 case ABOVE: 147 tgt.setValue(Enumerations.SearchModifierCode.ABOVE); 148 break; 149 case TYPE: 150 tgt.setValue(Enumerations.SearchModifierCode.TYPE); 151 break; 152 default: 153 tgt.setValue(Enumerations.SearchModifierCode.NULL); 154 break; 155 } 156 } 157 return tgt; 158 } 159 160 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.SearchParameter.SearchModifierCode> convertSearchModifierCode(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.SearchModifierCode> src) throws FHIRException { 161 if (src == null || src.isEmpty()) 162 return null; 163 org.hl7.fhir.dstu3.model.Enumeration<SearchParameter.SearchModifierCode> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new SearchParameter.SearchModifierCodeEnumFactory()); 164 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 165 if (src.getValue() == null) { 166 tgt.setValue(null); 167 } else { 168 switch (src.getValue()) { 169 case MISSING: 170 tgt.setValue(SearchParameter.SearchModifierCode.MISSING); 171 break; 172 case EXACT: 173 tgt.setValue(SearchParameter.SearchModifierCode.EXACT); 174 break; 175 case CONTAINS: 176 tgt.setValue(SearchParameter.SearchModifierCode.CONTAINS); 177 break; 178 case NOT: 179 tgt.setValue(SearchParameter.SearchModifierCode.NOT); 180 break; 181 case TEXT: 182 tgt.setValue(SearchParameter.SearchModifierCode.TEXT); 183 break; 184 case IN: 185 tgt.setValue(SearchParameter.SearchModifierCode.IN); 186 break; 187 case NOTIN: 188 tgt.setValue(SearchParameter.SearchModifierCode.NOTIN); 189 break; 190 case BELOW: 191 tgt.setValue(SearchParameter.SearchModifierCode.BELOW); 192 break; 193 case ABOVE: 194 tgt.setValue(SearchParameter.SearchModifierCode.ABOVE); 195 break; 196 case TYPE: 197 tgt.setValue(SearchParameter.SearchModifierCode.TYPE); 198 break; 199 default: 200 tgt.setValue(SearchParameter.SearchModifierCode.NULL); 201 break; 202 } 203 } 204 return tgt; 205 } 206 207 public static org.hl7.fhir.r5.model.SearchParameter convertSearchParameter(org.hl7.fhir.dstu3.model.SearchParameter src) throws FHIRException { 208 if (src == null) 209 return null; 210 org.hl7.fhir.r5.model.SearchParameter tgt = new org.hl7.fhir.r5.model.SearchParameter(); 211 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 212 if (src.hasUrl()) 213 tgt.setUrlElement(Uri30_50.convertUri(src.getUrlElement())); 214 if (src.hasVersion()) 215 tgt.setVersionElement(String30_50.convertString(src.getVersionElement())); 216 if (src.hasName()) 217 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 218 if (src.hasStatus()) 219 tgt.setStatusElement(Enumerations30_50.convertPublicationStatus(src.getStatusElement())); 220 if (src.hasExperimental()) 221 tgt.setExperimentalElement(Boolean30_50.convertBoolean(src.getExperimentalElement())); 222 if (src.hasDate()) 223 tgt.setDateElement(DateTime30_50.convertDateTime(src.getDateElement())); 224 if (src.hasPublisher()) 225 tgt.setPublisherElement(String30_50.convertString(src.getPublisherElement())); 226 for (org.hl7.fhir.dstu3.model.ContactDetail t : src.getContact()) 227 tgt.addContact(ContactDetail30_50.convertContactDetail(t)); 228 for (org.hl7.fhir.dstu3.model.UsageContext t : src.getUseContext()) 229 tgt.addUseContext(UsageContext30_50.convertUsageContext(t)); 230 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getJurisdiction()) 231 tgt.addJurisdiction(CodeableConcept30_50.convertCodeableConcept(t)); 232 if (src.hasPurpose()) 233 tgt.setPurposeElement(MarkDown30_50.convertMarkdown(src.getPurposeElement())); 234 if (src.hasCode()) 235 tgt.setCodeElement(Code30_50.convertCode(src.getCodeElement())); 236 for (org.hl7.fhir.dstu3.model.CodeType t : src.getBase()) tgt.getBase().add(new Enumeration<VersionIndependentResourceTypesAll>(new VersionIndependentResourceTypesAllEnumFactory(), Code30_50.convertCode(t))); 237 if (src.hasType()) 238 tgt.setTypeElement(Enumerations30_50.convertSearchParamType(src.getTypeElement())); 239 if (src.hasDerivedFrom()) 240 tgt.setDerivedFrom(src.getDerivedFrom()); 241 if (src.hasDescription()) 242 tgt.setDescriptionElement(MarkDown30_50.convertMarkdown(src.getDescriptionElement())); 243 if (src.hasExpression()) 244 tgt.setExpressionElement(String30_50.convertString(src.getExpressionElement())); 245// if (src.hasXpath()) 246// tgt.setXpathElement(String30_50.convertString(src.getXpathElement())); 247 if (src.hasXpathUsage()) 248 tgt.setProcessingModeElement(convertXPathUsageType(src.getXpathUsageElement())); 249 for (org.hl7.fhir.dstu3.model.CodeType t : src.getTarget()) tgt.getTarget().add(new Enumeration<VersionIndependentResourceTypesAll>(new VersionIndependentResourceTypesAllEnumFactory(), t.getValue())); 250 tgt.setComparator(src.getComparator().stream() 251 .map(SearchParameter30_50::convertSearchComparator) 252 .collect(Collectors.toList())); 253 tgt.setModifier(src.getModifier().stream() 254 .map(SearchParameter30_50::convertSearchModifierCode) 255 .collect(Collectors.toList())); 256 for (org.hl7.fhir.dstu3.model.StringType t : src.getChain()) tgt.addChain(t.getValue()); 257 for (org.hl7.fhir.dstu3.model.SearchParameter.SearchParameterComponentComponent t : src.getComponent()) 258 tgt.addComponent(convertSearchParameterComponentComponent(t)); 259 return tgt; 260 } 261 262 public static org.hl7.fhir.dstu3.model.SearchParameter convertSearchParameter(org.hl7.fhir.r5.model.SearchParameter src) throws FHIRException { 263 if (src == null) 264 return null; 265 org.hl7.fhir.dstu3.model.SearchParameter tgt = new org.hl7.fhir.dstu3.model.SearchParameter(); 266 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 267 if (src.hasUrl()) 268 tgt.setUrlElement(Uri30_50.convertUri(src.getUrlElement())); 269 if (src.hasVersion()) 270 tgt.setVersionElement(String30_50.convertString(src.getVersionElement())); 271 if (src.hasName()) 272 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 273 if (src.hasStatus()) 274 tgt.setStatusElement(Enumerations30_50.convertPublicationStatus(src.getStatusElement())); 275 if (src.hasExperimental()) 276 tgt.setExperimentalElement(Boolean30_50.convertBoolean(src.getExperimentalElement())); 277 if (src.hasDate()) 278 tgt.setDateElement(DateTime30_50.convertDateTime(src.getDateElement())); 279 if (src.hasPublisher()) 280 tgt.setPublisherElement(String30_50.convertString(src.getPublisherElement())); 281 for (org.hl7.fhir.r5.model.ContactDetail t : src.getContact()) 282 tgt.addContact(ContactDetail30_50.convertContactDetail(t)); 283 for (org.hl7.fhir.r5.model.UsageContext t : src.getUseContext()) 284 tgt.addUseContext(UsageContext30_50.convertUsageContext(t)); 285 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getJurisdiction()) 286 tgt.addJurisdiction(CodeableConcept30_50.convertCodeableConcept(t)); 287 if (src.hasPurpose()) 288 tgt.setPurposeElement(MarkDown30_50.convertMarkdown(src.getPurposeElement())); 289 if (src.hasCode()) 290 tgt.setCodeElement(Code30_50.convertCode(src.getCodeElement())); 291 for (Enumeration<VersionIndependentResourceTypesAll> t : src.getBase()) tgt.getBase().add(Code30_50.convertCode(t.getCodeType())); 292 if (src.hasType()) 293 tgt.setTypeElement(Enumerations30_50.convertSearchParamType(src.getTypeElement())); 294 if (src.hasDerivedFrom()) 295 tgt.setDerivedFrom(src.getDerivedFrom()); 296 if (src.hasDescription()) 297 tgt.setDescriptionElement(MarkDown30_50.convertMarkdown(src.getDescriptionElement())); 298 if (src.hasExpression()) 299 tgt.setExpressionElement(String30_50.convertString(src.getExpressionElement())); 300// if (src.hasXpath()) 301// tgt.setXpathElement(String30_50.convertString(src.getXpathElement())); 302 if (src.hasProcessingMode()) 303 tgt.setXpathUsageElement(convertXPathUsageType(src.getProcessingModeElement())); 304 for (Enumeration<VersionIndependentResourceTypesAll> t : src.getTarget()) tgt.getTarget().add(Code30_50.convertCode(t.getCodeType())); 305 tgt.setComparator(src.getComparator().stream() 306 .map(SearchParameter30_50::convertSearchComparator) 307 .collect(Collectors.toList())); 308 tgt.setModifier(src.getModifier().stream() 309 .map(SearchParameter30_50::convertSearchModifierCode) 310 .collect(Collectors.toList())); 311 for (org.hl7.fhir.r5.model.StringType t : src.getChain()) tgt.addChain(t.getValue()); 312 for (org.hl7.fhir.r5.model.SearchParameter.SearchParameterComponentComponent t : src.getComponent()) 313 tgt.addComponent(convertSearchParameterComponentComponent(t)); 314 return tgt; 315 } 316 317 public static org.hl7.fhir.r5.model.SearchParameter.SearchParameterComponentComponent convertSearchParameterComponentComponent(org.hl7.fhir.dstu3.model.SearchParameter.SearchParameterComponentComponent src) throws FHIRException { 318 if (src == null) 319 return null; 320 org.hl7.fhir.r5.model.SearchParameter.SearchParameterComponentComponent tgt = new org.hl7.fhir.r5.model.SearchParameter.SearchParameterComponentComponent(); 321 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 322 if (src.hasDefinition()) 323 tgt.setDefinitionElement(Reference30_50.convertReferenceToCanonical(src.getDefinition())); 324 if (src.hasExpression()) 325 tgt.setExpressionElement(String30_50.convertString(src.getExpressionElement())); 326 return tgt; 327 } 328 329 public static org.hl7.fhir.dstu3.model.SearchParameter.SearchParameterComponentComponent convertSearchParameterComponentComponent(org.hl7.fhir.r5.model.SearchParameter.SearchParameterComponentComponent src) throws FHIRException { 330 if (src == null) 331 return null; 332 org.hl7.fhir.dstu3.model.SearchParameter.SearchParameterComponentComponent tgt = new org.hl7.fhir.dstu3.model.SearchParameter.SearchParameterComponentComponent(); 333 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 334 if (src.hasDefinition()) 335 tgt.setDefinition(Reference30_50.convertCanonicalToReference(src.getDefinitionElement())); 336 if (src.hasExpression()) 337 tgt.setExpressionElement(String30_50.convertString(src.getExpressionElement())); 338 return tgt; 339 } 340 341 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.SearchParameter.SearchProcessingModeType> convertXPathUsageType(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.SearchParameter.XPathUsageType> src) throws FHIRException { 342 if (src == null || src.isEmpty()) 343 return null; 344 Enumeration<org.hl7.fhir.r5.model.SearchParameter.SearchProcessingModeType> tgt = new Enumeration<>(new org.hl7.fhir.r5.model.SearchParameter.SearchProcessingModeTypeEnumFactory()); 345 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 346 if (src.getValue() == null) { 347 tgt.setValue(null); 348 } else { 349 switch (src.getValue()) { 350 case NORMAL: 351 tgt.setValue(org.hl7.fhir.r5.model.SearchParameter.SearchProcessingModeType.NORMAL); 352 break; 353 case PHONETIC: 354 tgt.setValue(org.hl7.fhir.r5.model.SearchParameter.SearchProcessingModeType.PHONETIC); 355 break; 356 case NEARBY: 357 tgt.setValue(org.hl7.fhir.r5.model.SearchParameter.SearchProcessingModeType.OTHER); 358 break; 359 case DISTANCE: 360 tgt.setValue(org.hl7.fhir.r5.model.SearchParameter.SearchProcessingModeType.OTHER); 361 break; 362 case OTHER: 363 tgt.setValue(org.hl7.fhir.r5.model.SearchParameter.SearchProcessingModeType.OTHER); 364 break; 365 default: 366 tgt.setValue(org.hl7.fhir.r5.model.SearchParameter.SearchProcessingModeType.NULL); 367 break; 368 } 369 } 370 return tgt; 371 } 372 373 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.SearchParameter.XPathUsageType> convertXPathUsageType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.SearchParameter.SearchProcessingModeType> src) throws FHIRException { 374 if (src == null || src.isEmpty()) 375 return null; 376 org.hl7.fhir.dstu3.model.Enumeration<SearchParameter.XPathUsageType> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new SearchParameter.XPathUsageTypeEnumFactory()); 377 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 378 if (src.getValue() == null) { 379 tgt.setValue(null); 380 } else { 381 switch (src.getValue()) { 382 case NORMAL: 383 tgt.setValue(SearchParameter.XPathUsageType.NORMAL); 384 break; 385 case PHONETIC: 386 tgt.setValue(SearchParameter.XPathUsageType.PHONETIC); 387 break; 388 case OTHER: 389 tgt.setValue(SearchParameter.XPathUsageType.OTHER); 390 break; 391 default: 392 tgt.setValue(SearchParameter.XPathUsageType.NULL); 393 break; 394 } 395 } 396 return tgt; 397 } 398}