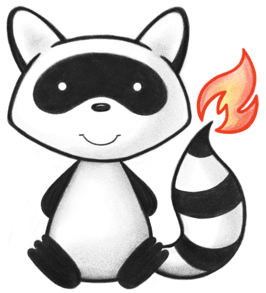
001package org.hl7.fhir.convertors.conv30_50.resources30_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_50; 004import org.hl7.fhir.convertors.conv30_50.datatypes30_50.Reference30_50; 005import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.CodeableConcept30_50; 006import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Identifier30_50; 007import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Boolean30_50; 008import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Instant30_50; 009import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.String30_50; 010import org.hl7.fhir.exceptions.FHIRException; 011import org.hl7.fhir.r5.model.CodeableReference; 012 013public class Slot30_50 { 014 015 public static org.hl7.fhir.r5.model.Slot convertSlot(org.hl7.fhir.dstu3.model.Slot src) throws FHIRException { 016 if (src == null) 017 return null; 018 org.hl7.fhir.r5.model.Slot tgt = new org.hl7.fhir.r5.model.Slot(); 019 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 020 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 021 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 022 if (src.hasServiceCategory()) 023 tgt.addServiceCategory(CodeableConcept30_50.convertCodeableConcept(src.getServiceCategory())); 024 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getServiceType()) 025 tgt.addServiceType(new CodeableReference().setConcept(CodeableConcept30_50.convertCodeableConcept(t))); 026 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getSpecialty()) 027 tgt.addSpecialty(CodeableConcept30_50.convertCodeableConcept(t)); 028 if (src.hasAppointmentType()) 029 tgt.addAppointmentType(CodeableConcept30_50.convertCodeableConcept(src.getAppointmentType())); 030 if (src.hasSchedule()) 031 tgt.setSchedule(Reference30_50.convertReference(src.getSchedule())); 032 if (src.hasStatus()) 033 tgt.setStatusElement(convertSlotStatus(src.getStatusElement())); 034 if (src.hasStart()) 035 tgt.setStartElement(Instant30_50.convertInstant(src.getStartElement())); 036 if (src.hasEnd()) 037 tgt.setEndElement(Instant30_50.convertInstant(src.getEndElement())); 038 if (src.hasOverbooked()) 039 tgt.setOverbookedElement(Boolean30_50.convertBoolean(src.getOverbookedElement())); 040 if (src.hasComment()) 041 tgt.setCommentElement(String30_50.convertString(src.getCommentElement())); 042 return tgt; 043 } 044 045 public static org.hl7.fhir.dstu3.model.Slot convertSlot(org.hl7.fhir.r5.model.Slot src) throws FHIRException { 046 if (src == null) 047 return null; 048 org.hl7.fhir.dstu3.model.Slot tgt = new org.hl7.fhir.dstu3.model.Slot(); 049 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 050 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 051 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 052 if (src.hasServiceCategory()) 053 tgt.setServiceCategory(CodeableConcept30_50.convertCodeableConcept(src.getServiceCategoryFirstRep())); 054 for (CodeableReference t : src.getServiceType()) 055 if (t.hasConcept()) 056 tgt.addServiceType(CodeableConcept30_50.convertCodeableConcept(t.getConcept())); 057 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getSpecialty()) 058 tgt.addSpecialty(CodeableConcept30_50.convertCodeableConcept(t)); 059 if (src.hasAppointmentType()) 060 tgt.setAppointmentType(CodeableConcept30_50.convertCodeableConcept(src.getAppointmentTypeFirstRep())); 061 if (src.hasSchedule()) 062 tgt.setSchedule(Reference30_50.convertReference(src.getSchedule())); 063 if (src.hasStatus()) 064 tgt.setStatusElement(convertSlotStatus(src.getStatusElement())); 065 if (src.hasStart()) 066 tgt.setStartElement(Instant30_50.convertInstant(src.getStartElement())); 067 if (src.hasEnd()) 068 tgt.setEndElement(Instant30_50.convertInstant(src.getEndElement())); 069 if (src.hasOverbooked()) 070 tgt.setOverbookedElement(Boolean30_50.convertBoolean(src.getOverbookedElement())); 071 if (src.hasComment()) 072 tgt.setCommentElement(String30_50.convertString(src.getCommentElement())); 073 return tgt; 074 } 075 076 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Slot.SlotStatus> convertSlotStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Slot.SlotStatus> src) throws FHIRException { 077 if (src == null || src.isEmpty()) 078 return null; 079 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Slot.SlotStatus> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Slot.SlotStatusEnumFactory()); 080 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 081 switch (src.getValue()) { 082 case BUSY: 083 tgt.setValue(org.hl7.fhir.dstu3.model.Slot.SlotStatus.BUSY); 084 break; 085 case FREE: 086 tgt.setValue(org.hl7.fhir.dstu3.model.Slot.SlotStatus.FREE); 087 break; 088 case BUSYUNAVAILABLE: 089 tgt.setValue(org.hl7.fhir.dstu3.model.Slot.SlotStatus.BUSYUNAVAILABLE); 090 break; 091 case BUSYTENTATIVE: 092 tgt.setValue(org.hl7.fhir.dstu3.model.Slot.SlotStatus.BUSYTENTATIVE); 093 break; 094 case ENTEREDINERROR: 095 tgt.setValue(org.hl7.fhir.dstu3.model.Slot.SlotStatus.ENTEREDINERROR); 096 break; 097 default: 098 tgt.setValue(org.hl7.fhir.dstu3.model.Slot.SlotStatus.NULL); 099 break; 100 } 101 return tgt; 102 } 103 104 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Slot.SlotStatus> convertSlotStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Slot.SlotStatus> src) throws FHIRException { 105 if (src == null || src.isEmpty()) 106 return null; 107 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Slot.SlotStatus> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Slot.SlotStatusEnumFactory()); 108 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 109 switch (src.getValue()) { 110 case BUSY: 111 tgt.setValue(org.hl7.fhir.r5.model.Slot.SlotStatus.BUSY); 112 break; 113 case FREE: 114 tgt.setValue(org.hl7.fhir.r5.model.Slot.SlotStatus.FREE); 115 break; 116 case BUSYUNAVAILABLE: 117 tgt.setValue(org.hl7.fhir.r5.model.Slot.SlotStatus.BUSYUNAVAILABLE); 118 break; 119 case BUSYTENTATIVE: 120 tgt.setValue(org.hl7.fhir.r5.model.Slot.SlotStatus.BUSYTENTATIVE); 121 break; 122 case ENTEREDINERROR: 123 tgt.setValue(org.hl7.fhir.r5.model.Slot.SlotStatus.ENTEREDINERROR); 124 break; 125 default: 126 tgt.setValue(org.hl7.fhir.r5.model.Slot.SlotStatus.NULL); 127 break; 128 } 129 return tgt; 130 } 131}