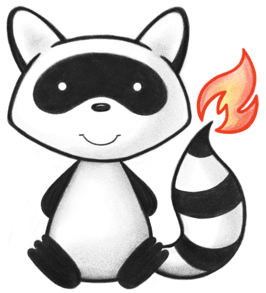
001package org.hl7.fhir.convertors.conv30_50.resources30_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_50; 004import org.hl7.fhir.convertors.conv30_50.datatypes30_50.Reference30_50; 005import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Annotation30_50; 006import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.CodeableConcept30_50; 007import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Identifier30_50; 008import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.SimpleQuantity30_50; 009import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.DateTime30_50; 010import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.String30_50; 011import org.hl7.fhir.dstu3.model.Enumeration; 012import org.hl7.fhir.dstu3.model.Specimen; 013import org.hl7.fhir.exceptions.FHIRException; 014 015public class Specimen30_50 { 016 017 public static org.hl7.fhir.dstu3.model.Specimen convertSpecimen(org.hl7.fhir.r5.model.Specimen src) throws FHIRException { 018 if (src == null) 019 return null; 020 org.hl7.fhir.dstu3.model.Specimen tgt = new org.hl7.fhir.dstu3.model.Specimen(); 021 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 022 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 023 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 024 if (src.hasAccessionIdentifier()) 025 tgt.setAccessionIdentifier(Identifier30_50.convertIdentifier(src.getAccessionIdentifier())); 026 if (src.hasStatus()) 027 tgt.setStatusElement(convertSpecimenStatus(src.getStatusElement())); 028 if (src.hasType()) 029 tgt.setType(CodeableConcept30_50.convertCodeableConcept(src.getType())); 030 if (src.hasSubject()) 031 tgt.setSubject(Reference30_50.convertReference(src.getSubject())); 032 if (src.hasReceivedTime()) 033 tgt.setReceivedTimeElement(DateTime30_50.convertDateTime(src.getReceivedTimeElement())); 034 for (org.hl7.fhir.r5.model.Reference t : src.getParent()) tgt.addParent(Reference30_50.convertReference(t)); 035 for (org.hl7.fhir.r5.model.Reference t : src.getRequest()) tgt.addRequest(Reference30_50.convertReference(t)); 036 if (src.hasCollection()) 037 tgt.setCollection(convertSpecimenCollectionComponent(src.getCollection())); 038 for (org.hl7.fhir.r5.model.Specimen.SpecimenProcessingComponent t : src.getProcessing()) 039 tgt.addProcessing(convertSpecimenProcessingComponent(t)); 040 for (org.hl7.fhir.r5.model.Specimen.SpecimenContainerComponent t : src.getContainer()) 041 tgt.addContainer(convertSpecimenContainerComponent(t)); 042 for (org.hl7.fhir.r5.model.Annotation t : src.getNote()) tgt.addNote(Annotation30_50.convertAnnotation(t)); 043 return tgt; 044 } 045 046 public static org.hl7.fhir.r5.model.Specimen convertSpecimen(org.hl7.fhir.dstu3.model.Specimen src) throws FHIRException { 047 if (src == null) 048 return null; 049 org.hl7.fhir.r5.model.Specimen tgt = new org.hl7.fhir.r5.model.Specimen(); 050 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 051 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 052 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 053 if (src.hasAccessionIdentifier()) 054 tgt.setAccessionIdentifier(Identifier30_50.convertIdentifier(src.getAccessionIdentifier())); 055 if (src.hasStatus()) 056 tgt.setStatusElement(convertSpecimenStatus(src.getStatusElement())); 057 if (src.hasType()) 058 tgt.setType(CodeableConcept30_50.convertCodeableConcept(src.getType())); 059 if (src.hasSubject()) 060 tgt.setSubject(Reference30_50.convertReference(src.getSubject())); 061 if (src.hasReceivedTime()) 062 tgt.setReceivedTimeElement(DateTime30_50.convertDateTime(src.getReceivedTimeElement())); 063 for (org.hl7.fhir.dstu3.model.Reference t : src.getParent()) tgt.addParent(Reference30_50.convertReference(t)); 064 for (org.hl7.fhir.dstu3.model.Reference t : src.getRequest()) tgt.addRequest(Reference30_50.convertReference(t)); 065 if (src.hasCollection()) 066 tgt.setCollection(convertSpecimenCollectionComponent(src.getCollection())); 067 for (org.hl7.fhir.dstu3.model.Specimen.SpecimenProcessingComponent t : src.getProcessing()) 068 tgt.addProcessing(convertSpecimenProcessingComponent(t)); 069 for (org.hl7.fhir.dstu3.model.Specimen.SpecimenContainerComponent t : src.getContainer()) 070 tgt.addContainer(convertSpecimenContainerComponent(t)); 071 for (org.hl7.fhir.dstu3.model.Annotation t : src.getNote()) tgt.addNote(Annotation30_50.convertAnnotation(t)); 072 return tgt; 073 } 074 075 public static org.hl7.fhir.r5.model.Specimen.SpecimenCollectionComponent convertSpecimenCollectionComponent(org.hl7.fhir.dstu3.model.Specimen.SpecimenCollectionComponent src) throws FHIRException { 076 if (src == null) 077 return null; 078 org.hl7.fhir.r5.model.Specimen.SpecimenCollectionComponent tgt = new org.hl7.fhir.r5.model.Specimen.SpecimenCollectionComponent(); 079 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 080 if (src.hasCollector()) 081 tgt.setCollector(Reference30_50.convertReference(src.getCollector())); 082 if (src.hasCollected()) 083 tgt.setCollected(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getCollected())); 084 if (src.hasQuantity()) 085 tgt.setQuantity(SimpleQuantity30_50.convertSimpleQuantity(src.getQuantity())); 086 if (src.hasMethod()) 087 tgt.setMethod(CodeableConcept30_50.convertCodeableConcept(src.getMethod())); 088 if (src.hasBodySite()) 089 tgt.getBodySite().setConcept(CodeableConcept30_50.convertCodeableConcept(src.getBodySite())); 090 return tgt; 091 } 092 093 public static org.hl7.fhir.dstu3.model.Specimen.SpecimenCollectionComponent convertSpecimenCollectionComponent(org.hl7.fhir.r5.model.Specimen.SpecimenCollectionComponent src) throws FHIRException { 094 if (src == null) 095 return null; 096 org.hl7.fhir.dstu3.model.Specimen.SpecimenCollectionComponent tgt = new org.hl7.fhir.dstu3.model.Specimen.SpecimenCollectionComponent(); 097 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 098 if (src.hasCollector()) 099 tgt.setCollector(Reference30_50.convertReference(src.getCollector())); 100 if (src.hasCollected()) 101 tgt.setCollected(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getCollected())); 102 if (src.hasQuantity()) 103 tgt.setQuantity(SimpleQuantity30_50.convertSimpleQuantity(src.getQuantity())); 104 if (src.hasMethod()) 105 tgt.setMethod(CodeableConcept30_50.convertCodeableConcept(src.getMethod())); 106 if (src.getBodySite().hasConcept()) 107 tgt.setBodySite(CodeableConcept30_50.convertCodeableConcept(src.getBodySite().getConcept())); 108 return tgt; 109 } 110 111 public static org.hl7.fhir.dstu3.model.Specimen.SpecimenContainerComponent convertSpecimenContainerComponent(org.hl7.fhir.r5.model.Specimen.SpecimenContainerComponent src) throws FHIRException { 112 if (src == null) 113 return null; 114 org.hl7.fhir.dstu3.model.Specimen.SpecimenContainerComponent tgt = new org.hl7.fhir.dstu3.model.Specimen.SpecimenContainerComponent(); 115 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 116// for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 117// tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 118// if (src.hasDescription()) 119// tgt.setDescriptionElement(String30_50.convertString(src.getDescriptionElement())); 120// if (src.hasType()) 121// tgt.setType(CodeableConcept30_50.convertCodeableConcept(src.getType())); 122// if (src.hasCapacity()) 123// tgt.setCapacity(SimpleQuantity30_50.convertSimpleQuantity(src.getCapacity())); 124// if (src.hasSpecimenQuantity()) 125// tgt.setSpecimenQuantity(SimpleQuantity30_50.convertSimpleQuantity(src.getSpecimenQuantity())); 126// if (src.hasAdditive()) 127// tgt.setAdditive(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getAdditive())); 128 return tgt; 129 } 130 131 public static org.hl7.fhir.r5.model.Specimen.SpecimenContainerComponent convertSpecimenContainerComponent(org.hl7.fhir.dstu3.model.Specimen.SpecimenContainerComponent src) throws FHIRException { 132 if (src == null) 133 return null; 134 org.hl7.fhir.r5.model.Specimen.SpecimenContainerComponent tgt = new org.hl7.fhir.r5.model.Specimen.SpecimenContainerComponent(); 135 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 136// for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 137// tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 138// if (src.hasDescription()) 139// tgt.setDescriptionElement(String30_50.convertString(src.getDescriptionElement())); 140// if (src.hasType()) 141// tgt.setType(CodeableConcept30_50.convertCodeableConcept(src.getType())); 142// if (src.hasCapacity()) 143// tgt.setCapacity(SimpleQuantity30_50.convertSimpleQuantity(src.getCapacity())); 144// if (src.hasSpecimenQuantity()) 145// tgt.setSpecimenQuantity(SimpleQuantity30_50.convertSimpleQuantity(src.getSpecimenQuantity())); 146// if (src.hasAdditive()) 147// tgt.setAdditive(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getAdditive())); 148 return tgt; 149 } 150 151 public static org.hl7.fhir.r5.model.Specimen.SpecimenProcessingComponent convertSpecimenProcessingComponent(org.hl7.fhir.dstu3.model.Specimen.SpecimenProcessingComponent src) throws FHIRException { 152 if (src == null) 153 return null; 154 org.hl7.fhir.r5.model.Specimen.SpecimenProcessingComponent tgt = new org.hl7.fhir.r5.model.Specimen.SpecimenProcessingComponent(); 155 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 156 if (src.hasDescription()) 157 tgt.setDescriptionElement(String30_50.convertString(src.getDescriptionElement())); 158// if (src.hasProcedure()) 159// tgt.setProcedure(CodeableConcept30_50.convertCodeableConcept(src.getProcedure())); 160 for (org.hl7.fhir.dstu3.model.Reference t : src.getAdditive()) tgt.addAdditive(Reference30_50.convertReference(t)); 161 if (src.hasTime()) 162 tgt.setTime(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getTime())); 163 return tgt; 164 } 165 166 public static org.hl7.fhir.dstu3.model.Specimen.SpecimenProcessingComponent convertSpecimenProcessingComponent(org.hl7.fhir.r5.model.Specimen.SpecimenProcessingComponent src) throws FHIRException { 167 if (src == null) 168 return null; 169 org.hl7.fhir.dstu3.model.Specimen.SpecimenProcessingComponent tgt = new org.hl7.fhir.dstu3.model.Specimen.SpecimenProcessingComponent(); 170 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 171 if (src.hasDescription()) 172 tgt.setDescriptionElement(String30_50.convertString(src.getDescriptionElement())); 173// if (src.hasProcedure()) 174// tgt.setProcedure(CodeableConcept30_50.convertCodeableConcept(src.getProcedure())); 175 for (org.hl7.fhir.r5.model.Reference t : src.getAdditive()) tgt.addAdditive(Reference30_50.convertReference(t)); 176 if (src.hasTime()) 177 tgt.setTime(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getTime())); 178 return tgt; 179 } 180 181 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Specimen.SpecimenStatus> convertSpecimenStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Specimen.SpecimenStatus> src) throws FHIRException { 182 if (src == null || src.isEmpty()) 183 return null; 184 Enumeration<Specimen.SpecimenStatus> tgt = new Enumeration<>(new Specimen.SpecimenStatusEnumFactory()); 185 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 186 if (src.getValue() == null) { 187 tgt.setValue(null); 188 } else { 189 switch (src.getValue()) { 190 case AVAILABLE: 191 tgt.setValue(Specimen.SpecimenStatus.AVAILABLE); 192 break; 193 case UNAVAILABLE: 194 tgt.setValue(Specimen.SpecimenStatus.UNAVAILABLE); 195 break; 196 case UNSATISFACTORY: 197 tgt.setValue(Specimen.SpecimenStatus.UNSATISFACTORY); 198 break; 199 case ENTEREDINERROR: 200 tgt.setValue(Specimen.SpecimenStatus.ENTEREDINERROR); 201 break; 202 default: 203 tgt.setValue(Specimen.SpecimenStatus.NULL); 204 break; 205 } 206 } 207 return tgt; 208 } 209 210 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Specimen.SpecimenStatus> convertSpecimenStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Specimen.SpecimenStatus> src) throws FHIRException { 211 if (src == null || src.isEmpty()) 212 return null; 213 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Specimen.SpecimenStatus> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Specimen.SpecimenStatusEnumFactory()); 214 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 215 if (src.getValue() == null) { 216 tgt.setValue(null); 217 } else { 218 switch (src.getValue()) { 219 case AVAILABLE: 220 tgt.setValue(org.hl7.fhir.r5.model.Specimen.SpecimenStatus.AVAILABLE); 221 break; 222 case UNAVAILABLE: 223 tgt.setValue(org.hl7.fhir.r5.model.Specimen.SpecimenStatus.UNAVAILABLE); 224 break; 225 case UNSATISFACTORY: 226 tgt.setValue(org.hl7.fhir.r5.model.Specimen.SpecimenStatus.UNSATISFACTORY); 227 break; 228 case ENTEREDINERROR: 229 tgt.setValue(org.hl7.fhir.r5.model.Specimen.SpecimenStatus.ENTEREDINERROR); 230 break; 231 default: 232 tgt.setValue(org.hl7.fhir.r5.model.Specimen.SpecimenStatus.NULL); 233 break; 234 } 235 } 236 return tgt; 237 } 238}