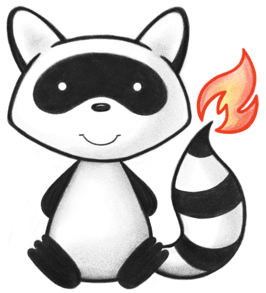
001package org.hl7.fhir.convertors.conv30_50.resources30_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_50; 004import org.hl7.fhir.convertors.conv30_50.datatypes30_50.ContactDetail30_50; 005import org.hl7.fhir.convertors.conv30_50.datatypes30_50.ElementDefinition30_50; 006import org.hl7.fhir.convertors.conv30_50.datatypes30_50.UsageContext30_50; 007import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.CodeableConcept30_50; 008import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Coding30_50; 009import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Identifier30_50; 010import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Boolean30_50; 011import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.DateTime30_50; 012import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Id30_50; 013import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.MarkDown30_50; 014import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.String30_50; 015import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Uri30_50; 016import org.hl7.fhir.exceptions.FHIRException; 017import org.hl7.fhir.r5.model.Enumeration; 018import org.hl7.fhir.r5.model.StructureDefinition; 019import org.hl7.fhir.utilities.Utilities; 020 021public class StructureDefinition30_50 { 022 023 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureDefinition.ExtensionContextType> convertExtensionContext(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureDefinition.ExtensionContext> src) throws FHIRException { 024 if (src == null || src.isEmpty()) 025 return null; 026 Enumeration<StructureDefinition.ExtensionContextType> tgt = new Enumeration<>(new StructureDefinition.ExtensionContextTypeEnumFactory()); 027 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 028 if (src.getValue() == null) { 029 tgt.setValue(null); 030 } else { 031 switch (src.getValue()) { 032 case RESOURCE: 033 tgt.setValue(StructureDefinition.ExtensionContextType.ELEMENT); 034 break; 035 case DATATYPE: 036 tgt.setValue(StructureDefinition.ExtensionContextType.ELEMENT); 037 break; 038 case EXTENSION: 039 tgt.setValue(StructureDefinition.ExtensionContextType.EXTENSION); 040 break; 041 default: 042 tgt.setValue(StructureDefinition.ExtensionContextType.NULL); 043 break; 044 } 045 } 046 return tgt; 047 } 048 049 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureDefinition.ExtensionContext> convertExtensionContext(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureDefinition.ExtensionContextType> src, String expression) throws FHIRException { 050 if (src == null || src.isEmpty()) 051 return null; 052 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureDefinition.ExtensionContext> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.StructureDefinition.ExtensionContextEnumFactory()); 053 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 054 if (src.getValue() == null) { 055 tgt.setValue(null); 056 } else { 057 switch (src.getValue()) { 058 case FHIRPATH: 059 tgt.setValue(org.hl7.fhir.dstu3.model.StructureDefinition.ExtensionContext.RESOURCE); 060 break; 061 case ELEMENT: 062 String tn = expression.contains(".") ? expression.substring(0, expression.indexOf(".")) : expression; 063 if (isResource300(tn)) { 064 tgt.setValue(org.hl7.fhir.dstu3.model.StructureDefinition.ExtensionContext.RESOURCE); 065 } else { 066 tgt.setValue(org.hl7.fhir.dstu3.model.StructureDefinition.ExtensionContext.DATATYPE); 067 } 068 break; 069 case EXTENSION: 070 tgt.setValue(org.hl7.fhir.dstu3.model.StructureDefinition.ExtensionContext.EXTENSION); 071 break; 072 default: 073 tgt.setValue(org.hl7.fhir.dstu3.model.StructureDefinition.ExtensionContext.NULL); 074 break; 075 } 076 } 077 return tgt; 078 } 079 080 public static org.hl7.fhir.r5.model.StructureDefinition convertStructureDefinition(org.hl7.fhir.dstu3.model.StructureDefinition src) throws FHIRException { 081 if (src == null) 082 return null; 083 org.hl7.fhir.r5.model.StructureDefinition tgt = new org.hl7.fhir.r5.model.StructureDefinition(); 084 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 085 if (src.hasUrl()) 086 tgt.setUrlElement(Uri30_50.convertUri(src.getUrlElement())); 087 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 088 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 089 if (src.hasVersion()) 090 tgt.setVersionElement(String30_50.convertString(src.getVersionElement())); 091 if (src.hasName()) 092 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 093 if (src.hasTitle()) 094 tgt.setTitleElement(String30_50.convertString(src.getTitleElement())); 095 if (src.hasStatus()) 096 tgt.setStatusElement(Enumerations30_50.convertPublicationStatus(src.getStatusElement())); 097 if (src.hasExperimental()) 098 tgt.setExperimentalElement(Boolean30_50.convertBoolean(src.getExperimentalElement())); 099 if (src.hasDate()) 100 tgt.setDateElement(DateTime30_50.convertDateTime(src.getDateElement())); 101 if (src.hasPublisher()) 102 tgt.setPublisherElement(String30_50.convertString(src.getPublisherElement())); 103 for (org.hl7.fhir.dstu3.model.ContactDetail t : src.getContact()) 104 tgt.addContact(ContactDetail30_50.convertContactDetail(t)); 105 if (src.hasDescription()) 106 tgt.setDescriptionElement(MarkDown30_50.convertMarkdown(src.getDescriptionElement())); 107 for (org.hl7.fhir.dstu3.model.UsageContext t : src.getUseContext()) 108 tgt.addUseContext(UsageContext30_50.convertUsageContext(t)); 109 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getJurisdiction()) 110 tgt.addJurisdiction(CodeableConcept30_50.convertCodeableConcept(t)); 111 if (src.hasPurpose()) 112 tgt.setPurposeElement(MarkDown30_50.convertMarkdown(src.getPurposeElement())); 113 if (src.hasCopyright()) 114 tgt.setCopyrightElement(MarkDown30_50.convertMarkdown(src.getCopyrightElement())); 115 for (org.hl7.fhir.dstu3.model.Coding t : src.getKeyword()) tgt.addKeyword(Coding30_50.convertCoding(t)); 116 if (src.hasFhirVersion()) 117 tgt.setFhirVersion(org.hl7.fhir.r5.model.Enumerations.FHIRVersion.fromCode(src.getFhirVersion())); 118 for (org.hl7.fhir.dstu3.model.StructureDefinition.StructureDefinitionMappingComponent t : src.getMapping()) 119 tgt.addMapping(convertStructureDefinitionMappingComponent(t)); 120 if (src.hasKind()) 121 tgt.setKindElement(convertStructureDefinitionKind(src.getKindElement())); 122 if (src.hasAbstract()) 123 tgt.setAbstractElement(Boolean30_50.convertBoolean(src.getAbstractElement())); 124 for (org.hl7.fhir.dstu3.model.StringType t : src.getContext()) { 125 org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionContextComponent ec = tgt.addContext(); 126 ec.setTypeElement(convertExtensionContext(src.getContextTypeElement())); 127 ec.setExpression("*".equals(t.getValue()) ? "Element" : t.getValue()); 128 } 129 for (org.hl7.fhir.dstu3.model.StringType t : src.getContextInvariant()) tgt.addContextInvariant(t.getValue()); 130 if (src.hasType()) 131 tgt.setType(src.getType()); 132 if (src.hasBaseDefinition()) 133 tgt.setBaseDefinition(src.getBaseDefinition()); 134 if (src.hasDerivation()) 135 tgt.setDerivationElement(convertTypeDerivationRule(src.getDerivationElement())); 136 if (src.hasSnapshot()) 137 tgt.setSnapshot(convertStructureDefinitionSnapshotComponent(src.getSnapshot())); 138 if (src.hasDifferential()) 139 tgt.setDifferential(convertStructureDefinitionDifferentialComponent(src.getDifferential())); 140 if (tgt.getDerivation() == org.hl7.fhir.r5.model.StructureDefinition.TypeDerivationRule.SPECIALIZATION) { 141 for (org.hl7.fhir.r5.model.ElementDefinition ed : tgt.getSnapshot().getElement()) { 142 if (!ed.hasBase()) { 143 ed.getBase().setPath(ed.getPath()).setMin(ed.getMin()).setMax(ed.getMax()); 144 } 145 } 146 } 147 return tgt; 148 } 149 150 public static org.hl7.fhir.dstu3.model.StructureDefinition convertStructureDefinition(org.hl7.fhir.r5.model.StructureDefinition src) throws FHIRException { 151 if (src == null) 152 return null; 153 org.hl7.fhir.dstu3.model.StructureDefinition tgt = new org.hl7.fhir.dstu3.model.StructureDefinition(); 154 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 155 if (src.hasUrl()) 156 tgt.setUrlElement(Uri30_50.convertUri(src.getUrlElement())); 157 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 158 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 159 if (src.hasVersion()) 160 tgt.setVersionElement(String30_50.convertString(src.getVersionElement())); 161 if (src.hasName()) 162 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 163 if (src.hasTitle()) 164 tgt.setTitleElement(String30_50.convertString(src.getTitleElement())); 165 if (src.hasStatus()) 166 tgt.setStatusElement(Enumerations30_50.convertPublicationStatus(src.getStatusElement())); 167 if (src.hasExperimental()) 168 tgt.setExperimentalElement(Boolean30_50.convertBoolean(src.getExperimentalElement())); 169 if (src.hasDate()) 170 tgt.setDateElement(DateTime30_50.convertDateTime(src.getDateElement())); 171 if (src.hasPublisher()) 172 tgt.setPublisherElement(String30_50.convertString(src.getPublisherElement())); 173 for (org.hl7.fhir.r5.model.ContactDetail t : src.getContact()) 174 tgt.addContact(ContactDetail30_50.convertContactDetail(t)); 175 if (src.hasDescription()) 176 tgt.setDescriptionElement(MarkDown30_50.convertMarkdown(src.getDescriptionElement())); 177 for (org.hl7.fhir.r5.model.UsageContext t : src.getUseContext()) 178 tgt.addUseContext(UsageContext30_50.convertUsageContext(t)); 179 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getJurisdiction()) 180 tgt.addJurisdiction(CodeableConcept30_50.convertCodeableConcept(t)); 181 if (src.hasPurpose()) 182 tgt.setPurposeElement(MarkDown30_50.convertMarkdown(src.getPurposeElement())); 183 if (src.hasCopyright()) 184 tgt.setCopyrightElement(MarkDown30_50.convertMarkdown(src.getCopyrightElement())); 185 for (org.hl7.fhir.r5.model.Coding t : src.getKeyword()) tgt.addKeyword(Coding30_50.convertCoding(t)); 186 if (src.hasFhirVersion()) 187 tgt.setFhirVersion(src.getFhirVersion().toCode()); 188 for (org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionMappingComponent t : src.getMapping()) 189 tgt.addMapping(convertStructureDefinitionMappingComponent(t)); 190 if (src.hasKind()) 191 tgt.setKindElement(convertStructureDefinitionKind(src.getKindElement())); 192 if (src.hasAbstract()) 193 tgt.setAbstractElement(Boolean30_50.convertBoolean(src.getAbstractElement())); 194 for (org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionContextComponent t : src.getContext()) { 195 if (!tgt.hasContextType()) 196 tgt.setContextTypeElement(convertExtensionContext(t.getTypeElement(), t.getExpression())); 197 tgt.addContext("Element".equals(t.getExpression()) ? "*" : t.getExpression()); 198 } 199 for (org.hl7.fhir.r5.model.StringType t : src.getContextInvariant()) tgt.addContextInvariant(t.getValue()); 200 if (src.hasType()) 201 tgt.setType(src.getType()); 202 if (src.hasBaseDefinition()) 203 tgt.setBaseDefinition(src.getBaseDefinition()); 204 if (src.hasDerivation()) 205 tgt.setDerivationElement(convertTypeDerivationRule(src.getDerivationElement())); 206 if (src.hasSnapshot()) 207 tgt.setSnapshot(convertStructureDefinitionSnapshotComponent(src.getSnapshot())); 208 if (src.hasDifferential()) 209 tgt.setDifferential(convertStructureDefinitionDifferentialComponent(src.getDifferential())); 210 return tgt; 211 } 212 213 public static org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionDifferentialComponent convertStructureDefinitionDifferentialComponent(org.hl7.fhir.dstu3.model.StructureDefinition.StructureDefinitionDifferentialComponent src) throws FHIRException { 214 if (src == null) 215 return null; 216 org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionDifferentialComponent tgt = new org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionDifferentialComponent(); 217 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 218 for (org.hl7.fhir.dstu3.model.ElementDefinition t : src.getElement()) 219 tgt.addElement(ElementDefinition30_50.convertElementDefinition(t)); 220 return tgt; 221 } 222 223 public static org.hl7.fhir.dstu3.model.StructureDefinition.StructureDefinitionDifferentialComponent convertStructureDefinitionDifferentialComponent(org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionDifferentialComponent src) throws FHIRException { 224 if (src == null) 225 return null; 226 org.hl7.fhir.dstu3.model.StructureDefinition.StructureDefinitionDifferentialComponent tgt = new org.hl7.fhir.dstu3.model.StructureDefinition.StructureDefinitionDifferentialComponent(); 227 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 228 for (org.hl7.fhir.r5.model.ElementDefinition t : src.getElement()) 229 tgt.addElement(ElementDefinition30_50.convertElementDefinition(t)); 230 return tgt; 231 } 232 233 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureDefinition.StructureDefinitionKind> convertStructureDefinitionKind(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionKind> src) throws FHIRException { 234 if (src == null || src.isEmpty()) 235 return null; 236 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureDefinition.StructureDefinitionKind> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.StructureDefinition.StructureDefinitionKindEnumFactory()); 237 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 238 if (src.getValue() == null) { 239 tgt.setValue(null); 240 } else { 241 switch (src.getValue()) { 242 case PRIMITIVETYPE: 243 tgt.setValue(org.hl7.fhir.dstu3.model.StructureDefinition.StructureDefinitionKind.PRIMITIVETYPE); 244 break; 245 case COMPLEXTYPE: 246 tgt.setValue(org.hl7.fhir.dstu3.model.StructureDefinition.StructureDefinitionKind.COMPLEXTYPE); 247 break; 248 case RESOURCE: 249 tgt.setValue(org.hl7.fhir.dstu3.model.StructureDefinition.StructureDefinitionKind.RESOURCE); 250 break; 251 case LOGICAL: 252 tgt.setValue(org.hl7.fhir.dstu3.model.StructureDefinition.StructureDefinitionKind.LOGICAL); 253 break; 254 default: 255 tgt.setValue(org.hl7.fhir.dstu3.model.StructureDefinition.StructureDefinitionKind.NULL); 256 break; 257 } 258 } 259 return tgt; 260 } 261 262 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionKind> convertStructureDefinitionKind(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureDefinition.StructureDefinitionKind> src) throws FHIRException { 263 if (src == null || src.isEmpty()) 264 return null; 265 Enumeration<StructureDefinition.StructureDefinitionKind> tgt = new Enumeration<>(new StructureDefinition.StructureDefinitionKindEnumFactory()); 266 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 267 if (src.getValue() == null) { 268 tgt.setValue(null); 269 } else { 270 switch (src.getValue()) { 271 case PRIMITIVETYPE: 272 tgt.setValue(StructureDefinition.StructureDefinitionKind.PRIMITIVETYPE); 273 break; 274 case COMPLEXTYPE: 275 tgt.setValue(StructureDefinition.StructureDefinitionKind.COMPLEXTYPE); 276 break; 277 case RESOURCE: 278 tgt.setValue(StructureDefinition.StructureDefinitionKind.RESOURCE); 279 break; 280 case LOGICAL: 281 tgt.setValue(StructureDefinition.StructureDefinitionKind.LOGICAL); 282 break; 283 default: 284 tgt.setValue(StructureDefinition.StructureDefinitionKind.NULL); 285 break; 286 } 287 } 288 return tgt; 289 } 290 291 public static org.hl7.fhir.dstu3.model.StructureDefinition.StructureDefinitionMappingComponent convertStructureDefinitionMappingComponent(org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionMappingComponent src) throws FHIRException { 292 if (src == null) 293 return null; 294 org.hl7.fhir.dstu3.model.StructureDefinition.StructureDefinitionMappingComponent tgt = new org.hl7.fhir.dstu3.model.StructureDefinition.StructureDefinitionMappingComponent(); 295 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 296 if (src.hasIdentity()) 297 tgt.setIdentityElement(Id30_50.convertId(src.getIdentityElement())); 298 if (src.hasUri()) 299 tgt.setUriElement(Uri30_50.convertUri(src.getUriElement())); 300 if (src.hasName()) 301 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 302 if (src.hasComment()) 303 tgt.setCommentElement(String30_50.convertString(src.getCommentElement())); 304 return tgt; 305 } 306 307 public static org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionMappingComponent convertStructureDefinitionMappingComponent(org.hl7.fhir.dstu3.model.StructureDefinition.StructureDefinitionMappingComponent src) throws FHIRException { 308 if (src == null) 309 return null; 310 org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionMappingComponent tgt = new org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionMappingComponent(); 311 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 312 if (src.hasIdentity()) 313 tgt.setIdentityElement(Id30_50.convertId(src.getIdentityElement())); 314 if (src.hasUri()) 315 tgt.setUriElement(Uri30_50.convertUri(src.getUriElement())); 316 if (src.hasName()) 317 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 318 if (src.hasComment()) 319 tgt.setCommentElement(String30_50.convertString(src.getCommentElement())); 320 return tgt; 321 } 322 323 public static org.hl7.fhir.dstu3.model.StructureDefinition.StructureDefinitionSnapshotComponent convertStructureDefinitionSnapshotComponent(org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionSnapshotComponent src) throws FHIRException { 324 if (src == null) 325 return null; 326 org.hl7.fhir.dstu3.model.StructureDefinition.StructureDefinitionSnapshotComponent tgt = new org.hl7.fhir.dstu3.model.StructureDefinition.StructureDefinitionSnapshotComponent(); 327 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 328 for (org.hl7.fhir.r5.model.ElementDefinition t : src.getElement()) 329 tgt.addElement(ElementDefinition30_50.convertElementDefinition(t)); 330 return tgt; 331 } 332 333 public static org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionSnapshotComponent convertStructureDefinitionSnapshotComponent(org.hl7.fhir.dstu3.model.StructureDefinition.StructureDefinitionSnapshotComponent src) throws FHIRException { 334 if (src == null) 335 return null; 336 org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionSnapshotComponent tgt = new org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionSnapshotComponent(); 337 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 338 for (org.hl7.fhir.dstu3.model.ElementDefinition t : src.getElement()) 339 tgt.addElement(ElementDefinition30_50.convertElementDefinition(t)); 340 return tgt; 341 } 342 343 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureDefinition.TypeDerivationRule> convertTypeDerivationRule(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureDefinition.TypeDerivationRule> src) throws FHIRException { 344 if (src == null || src.isEmpty()) 345 return null; 346 Enumeration<StructureDefinition.TypeDerivationRule> tgt = new Enumeration<>(new StructureDefinition.TypeDerivationRuleEnumFactory()); 347 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 348 if (src.getValue() == null) { 349 tgt.setValue(null); 350 } else { 351 switch (src.getValue()) { 352 case SPECIALIZATION: 353 tgt.setValue(StructureDefinition.TypeDerivationRule.SPECIALIZATION); 354 break; 355 case CONSTRAINT: 356 tgt.setValue(StructureDefinition.TypeDerivationRule.CONSTRAINT); 357 break; 358 default: 359 tgt.setValue(StructureDefinition.TypeDerivationRule.NULL); 360 break; 361 } 362 } 363 return tgt; 364 } 365 366 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureDefinition.TypeDerivationRule> convertTypeDerivationRule(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureDefinition.TypeDerivationRule> src) throws FHIRException { 367 if (src == null || src.isEmpty()) 368 return null; 369 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureDefinition.TypeDerivationRule> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.StructureDefinition.TypeDerivationRuleEnumFactory()); 370 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 371 if (src.getValue() == null) { 372 tgt.setValue(null); 373 } else { 374 switch (src.getValue()) { 375 case SPECIALIZATION: 376 tgt.setValue(org.hl7.fhir.dstu3.model.StructureDefinition.TypeDerivationRule.SPECIALIZATION); 377 break; 378 case CONSTRAINT: 379 tgt.setValue(org.hl7.fhir.dstu3.model.StructureDefinition.TypeDerivationRule.CONSTRAINT); 380 break; 381 default: 382 tgt.setValue(org.hl7.fhir.dstu3.model.StructureDefinition.TypeDerivationRule.NULL); 383 break; 384 } 385 } 386 return tgt; 387 } 388 389 static public boolean isResource300(String tn) { 390 return Utilities.existsInList(tn, "Account", "ActivityDefinition", "AllergyIntolerance", "AdverseEvent", "Appointment", "AppointmentResponse", "AuditEvent", "Basic", "Binary", "BodySite", "Bundle", "CapabilityStatement", "CarePlan", "CareTeam", "ChargeItem", "Claim", "ClaimResponse", "ClinicalImpression", "CodeSystem", "Communication", "CommunicationRequest", "CompartmentDefinition", "Composition", "ConceptMap", "Condition", "Consent", "Contract", "Coverage", "DataElement", "DetectedIssue", "Device", "DeviceComponent", "DeviceMetric", "DeviceRequest", "DeviceUseStatement", "DiagnosticReport", "DocumentManifest", "DocumentReference", "EligibilityRequest", "EligibilityResponse", "Encounter", "Endpoint", "EnrollmentRequest", "EnrollmentResponse", "EpisodeOfCare", "ExpansionProfile", "ExplanationOfBenefit", "FamilyMemberHistory", "Flag", "Goal", "GraphDefinition", "Group", "GuidanceResponse", "HealthcareService", "ImagingManifest", "ImagingStudy", "Immunization", "ImmunizationRecommendation", "ImplementationGuide", "Library", "Linkage", "List", "Location", "Measure", "MeasureReport", "Media", "Medication", "MedicationAdministration", "MedicationDispense", "MedicationRequest", "MedicationStatement", "MessageDefinition", "MessageHeader", "NamingSystem", "NutritionOrder", "Observation", "OperationDefinition", "OperationOutcome", "Organization", "Parameters", "Patient", "PaymentNotice", "PaymentReconciliation", "Person", "PlanDefinition", "Practitioner", "PractitionerRole", "Procedure", "ProcedureRequest", "ProcessRequest", "ProcessResponse", "Provenance", "Questionnaire", "QuestionnaireResponse", "ReferralRequest", "RelatedPerson", "RequestGroup", "ResearchStudy", "ResearchSubject", "RiskAssessment", "Schedule", "SearchParameter", "Sequence", "ServiceDefinition", "Slot", "Specimen", "StructureDefinition", "StructureMap", "Subscription", "Substance", "SupplyDelivery", "SupplyRequest", "Task", "TestScript", "TestReport", "ValueSet", "VisionPrescription"); 391 } 392}