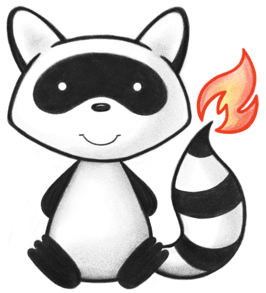
001package org.hl7.fhir.convertors.conv30_50.resources30_50; 002 003import java.util.stream.Collectors; 004 005import org.hl7.fhir.convertors.context.ConversionContext30_50; 006import org.hl7.fhir.convertors.conv30_50.datatypes30_50.ContactDetail30_50; 007import org.hl7.fhir.convertors.conv30_50.datatypes30_50.UsageContext30_50; 008import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.CodeableConcept30_50; 009import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Identifier30_50; 010import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Boolean30_50; 011import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.DateTime30_50; 012import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Id30_50; 013import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Integer30_50; 014import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.MarkDown30_50; 015import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.String30_50; 016import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Uri30_50; 017import org.hl7.fhir.exceptions.FHIRException; 018import org.hl7.fhir.r5.model.StringType; 019import org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleTargetParameterComponent; 020 021public class StructureMap30_50 { 022 023 public static org.hl7.fhir.r5.model.StructureMap convertStructureMap(org.hl7.fhir.dstu3.model.StructureMap src) throws FHIRException { 024 if (src == null) 025 return null; 026 org.hl7.fhir.r5.model.StructureMap tgt = new org.hl7.fhir.r5.model.StructureMap(); 027 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 028 if (src.hasUrl()) 029 tgt.setUrlElement(Uri30_50.convertUri(src.getUrlElement())); 030 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 031 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 032 if (src.hasVersion()) 033 tgt.setVersionElement(String30_50.convertString(src.getVersionElement())); 034 if (src.hasName()) 035 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 036 if (src.hasTitle()) 037 tgt.setTitleElement(String30_50.convertString(src.getTitleElement())); 038 if (src.hasStatus()) 039 tgt.setStatusElement(Enumerations30_50.convertPublicationStatus(src.getStatusElement())); 040 if (src.hasExperimental()) 041 tgt.setExperimentalElement(Boolean30_50.convertBoolean(src.getExperimentalElement())); 042 if (src.hasDate()) 043 tgt.setDateElement(DateTime30_50.convertDateTime(src.getDateElement())); 044 if (src.hasPublisher()) 045 tgt.setPublisherElement(String30_50.convertString(src.getPublisherElement())); 046 for (org.hl7.fhir.dstu3.model.ContactDetail t : src.getContact()) 047 tgt.addContact(ContactDetail30_50.convertContactDetail(t)); 048 if (src.hasDescription()) 049 tgt.setDescriptionElement(MarkDown30_50.convertMarkdown(src.getDescriptionElement())); 050 for (org.hl7.fhir.dstu3.model.UsageContext t : src.getUseContext()) 051 tgt.addUseContext(UsageContext30_50.convertUsageContext(t)); 052 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getJurisdiction()) 053 tgt.addJurisdiction(CodeableConcept30_50.convertCodeableConcept(t)); 054 if (src.hasPurpose()) 055 tgt.setPurposeElement(MarkDown30_50.convertMarkdown(src.getPurposeElement())); 056 if (src.hasCopyright()) 057 tgt.setCopyrightElement(MarkDown30_50.convertMarkdown(src.getCopyrightElement())); 058 for (org.hl7.fhir.dstu3.model.StructureMap.StructureMapStructureComponent t : src.getStructure()) 059 tgt.addStructure(convertStructureMapStructureComponent(t)); 060 for (org.hl7.fhir.dstu3.model.UriType t : src.getImport()) tgt.addImport(t.getValue()); 061 for (org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupComponent t : src.getGroup()) 062 tgt.addGroup(convertStructureMapGroupComponent(t)); 063 return tgt; 064 } 065 066 public static org.hl7.fhir.dstu3.model.StructureMap convertStructureMap(org.hl7.fhir.r5.model.StructureMap src) throws FHIRException { 067 if (src == null) 068 return null; 069 org.hl7.fhir.dstu3.model.StructureMap tgt = new org.hl7.fhir.dstu3.model.StructureMap(); 070 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 071 if (src.hasUrl()) 072 tgt.setUrlElement(Uri30_50.convertUri(src.getUrlElement())); 073 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 074 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 075 if (src.hasVersion()) 076 tgt.setVersionElement(String30_50.convertString(src.getVersionElement())); 077 if (src.hasName()) 078 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 079 if (src.hasTitle()) 080 tgt.setTitleElement(String30_50.convertString(src.getTitleElement())); 081 if (src.hasStatus()) 082 tgt.setStatusElement(Enumerations30_50.convertPublicationStatus(src.getStatusElement())); 083 if (src.hasExperimental()) 084 tgt.setExperimentalElement(Boolean30_50.convertBoolean(src.getExperimentalElement())); 085 if (src.hasDate()) 086 tgt.setDateElement(DateTime30_50.convertDateTime(src.getDateElement())); 087 if (src.hasPublisher()) 088 tgt.setPublisherElement(String30_50.convertString(src.getPublisherElement())); 089 for (org.hl7.fhir.r5.model.ContactDetail t : src.getContact()) 090 tgt.addContact(ContactDetail30_50.convertContactDetail(t)); 091 if (src.hasDescription()) 092 tgt.setDescriptionElement(MarkDown30_50.convertMarkdown(src.getDescriptionElement())); 093 for (org.hl7.fhir.r5.model.UsageContext t : src.getUseContext()) 094 tgt.addUseContext(UsageContext30_50.convertUsageContext(t)); 095 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getJurisdiction()) 096 tgt.addJurisdiction(CodeableConcept30_50.convertCodeableConcept(t)); 097 if (src.hasPurpose()) 098 tgt.setPurposeElement(MarkDown30_50.convertMarkdown(src.getPurposeElement())); 099 if (src.hasCopyright()) 100 tgt.setCopyrightElement(MarkDown30_50.convertMarkdown(src.getCopyrightElement())); 101 for (org.hl7.fhir.r5.model.StructureMap.StructureMapStructureComponent t : src.getStructure()) 102 tgt.addStructure(convertStructureMapStructureComponent(t)); 103 for (org.hl7.fhir.r5.model.UriType t : src.getImport()) tgt.addImport(t.getValue()); 104 for (org.hl7.fhir.r5.model.StructureMap.StructureMapGroupComponent t : src.getGroup()) 105 tgt.addGroup(convertStructureMapGroupComponent(t)); 106 return tgt; 107 } 108 109 110 public static org.hl7.fhir.r5.model.StructureMap.StructureMapGroupComponent convertStructureMapGroupComponent(org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupComponent src) throws FHIRException { 111 if (src == null) 112 return null; 113 org.hl7.fhir.r5.model.StructureMap.StructureMapGroupComponent tgt = new org.hl7.fhir.r5.model.StructureMap.StructureMapGroupComponent(); 114 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 115 if (src.hasName()) 116 tgt.setNameElement(Id30_50.convertId(src.getNameElement())); 117 if (src.hasExtends()) 118 tgt.setExtendsElement(Id30_50.convertId(src.getExtendsElement())); 119 if (src.hasTypeMode()) 120 tgt.setTypeModeElement(convertStructureMapGroupTypeMode(src.getTypeModeElement())); 121 if (src.hasDocumentation()) 122 tgt.setDocumentationElement(String30_50.convertString(src.getDocumentationElement())); 123 for (org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupInputComponent t : src.getInput()) 124 tgt.addInput(convertStructureMapGroupInputComponent(t)); 125 for (org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleComponent t : src.getRule()) 126 tgt.addRule(convertStructureMapGroupRuleComponent(t)); 127 return tgt; 128 } 129 130 public static org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupComponent convertStructureMapGroupComponent(org.hl7.fhir.r5.model.StructureMap.StructureMapGroupComponent src) throws FHIRException { 131 if (src == null) 132 return null; 133 org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupComponent tgt = new org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupComponent(); 134 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 135 if (src.hasName()) 136 tgt.setNameElement(Id30_50.convertId(src.getNameElement())); 137 if (src.hasExtends()) 138 tgt.setExtendsElement(Id30_50.convertId(src.getExtendsElement())); 139 if (src.hasTypeMode()) 140 tgt.setTypeModeElement(convertStructureMapGroupTypeMode(src.getTypeModeElement())); 141 if (src.hasDocumentation()) 142 tgt.setDocumentationElement(String30_50.convertString(src.getDocumentationElement())); 143 for (org.hl7.fhir.r5.model.StructureMap.StructureMapGroupInputComponent t : src.getInput()) 144 tgt.addInput(convertStructureMapGroupInputComponent(t)); 145 for (org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleComponent t : src.getRule()) 146 tgt.addRule(convertStructureMapGroupRuleComponent(t)); 147 return tgt; 148 } 149 150 public static org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupInputComponent convertStructureMapGroupInputComponent(org.hl7.fhir.r5.model.StructureMap.StructureMapGroupInputComponent src) throws FHIRException { 151 if (src == null) 152 return null; 153 org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupInputComponent tgt = new org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupInputComponent(); 154 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 155 if (src.hasName()) 156 tgt.setNameElement(Id30_50.convertId(src.getNameElement())); 157 if (src.hasType()) 158 tgt.setTypeElement(String30_50.convertString(src.getTypeElement())); 159 if (src.hasMode()) 160 tgt.setModeElement(convertStructureMapInputMode(src.getModeElement())); 161 if (src.hasDocumentation()) 162 tgt.setDocumentationElement(String30_50.convertString(src.getDocumentationElement())); 163 return tgt; 164 } 165 166 public static org.hl7.fhir.r5.model.StructureMap.StructureMapGroupInputComponent convertStructureMapGroupInputComponent(org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupInputComponent src) throws FHIRException { 167 if (src == null) 168 return null; 169 org.hl7.fhir.r5.model.StructureMap.StructureMapGroupInputComponent tgt = new org.hl7.fhir.r5.model.StructureMap.StructureMapGroupInputComponent(); 170 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 171 if (src.hasName()) 172 tgt.setNameElement(Id30_50.convertId(src.getNameElement())); 173 if (src.hasType()) 174 tgt.setTypeElement(String30_50.convertString(src.getTypeElement())); 175 if (src.hasMode()) 176 tgt.setModeElement(convertStructureMapInputMode(src.getModeElement())); 177 if (src.hasDocumentation()) 178 tgt.setDocumentationElement(String30_50.convertString(src.getDocumentationElement())); 179 return tgt; 180 } 181 182 public static org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleComponent convertStructureMapGroupRuleComponent(org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleComponent src) throws FHIRException { 183 if (src == null) 184 return null; 185 org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleComponent tgt = new org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleComponent(); 186 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 187 if (src.hasName()) 188 tgt.setNameElement(Id30_50.convertId(src.getNameElement())); 189 for (org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleSourceComponent t : src.getSource()) 190 tgt.addSource(convertStructureMapGroupRuleSourceComponent(t)); 191 for (org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleTargetComponent t : src.getTarget()) 192 tgt.addTarget(convertStructureMapGroupRuleTargetComponent(t)); 193 for (org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleComponent t : src.getRule()) 194 tgt.addRule(convertStructureMapGroupRuleComponent(t)); 195 for (org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleDependentComponent t : src.getDependent()) 196 tgt.addDependent(convertStructureMapGroupRuleDependentComponent(t)); 197 if (src.hasDocumentation()) 198 tgt.setDocumentationElement(String30_50.convertString(src.getDocumentationElement())); 199 return tgt; 200 } 201 202 public static org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleComponent convertStructureMapGroupRuleComponent(org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleComponent src) throws FHIRException { 203 if (src == null) 204 return null; 205 org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleComponent tgt = new org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleComponent(); 206 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 207 if (src.hasName()) 208 tgt.setNameElement(Id30_50.convertId(src.getNameElement())); 209 for (org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleSourceComponent t : src.getSource()) 210 tgt.addSource(convertStructureMapGroupRuleSourceComponent(t)); 211 for (org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleTargetComponent t : src.getTarget()) 212 tgt.addTarget(convertStructureMapGroupRuleTargetComponent(t)); 213 for (org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleComponent t : src.getRule()) 214 tgt.addRule(convertStructureMapGroupRuleComponent(t)); 215 for (org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleDependentComponent t : src.getDependent()) 216 tgt.addDependent(convertStructureMapGroupRuleDependentComponent(t)); 217 if (src.hasDocumentation()) 218 tgt.setDocumentationElement(String30_50.convertString(src.getDocumentationElement())); 219 return tgt; 220 } 221 222 public static org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleDependentComponent convertStructureMapGroupRuleDependentComponent(org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleDependentComponent src) throws FHIRException { 223 if (src == null) 224 return null; 225 org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleDependentComponent tgt = new org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleDependentComponent(); 226 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 227 if (src.hasName()) 228 tgt.setNameElement(Id30_50.convertId(src.getNameElement())); 229 for (org.hl7.fhir.dstu3.model.StringType t : src.getVariable()) tgt.addParameter().setValue(String30_50.convertString(t)); 230 return tgt; 231 } 232 233 public static org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleDependentComponent convertStructureMapGroupRuleDependentComponent(org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleDependentComponent src) throws FHIRException { 234 if (src == null) 235 return null; 236 org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleDependentComponent tgt = new org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleDependentComponent(); 237 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 238 if (src.hasName()) 239 tgt.setNameElement(Id30_50.convertId(src.getNameElement())); 240 for (StructureMapGroupRuleTargetParameterComponent t : src.getParameter()) tgt.addVariable(t.getValue().primitiveValue()); 241 return tgt; 242 } 243 244 public static org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleSourceComponent convertStructureMapGroupRuleSourceComponent(org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleSourceComponent src) throws FHIRException { 245 if (src == null) 246 return null; 247 org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleSourceComponent tgt = new org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleSourceComponent(); 248 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 249 if (src.hasContext()) 250 tgt.setContextElement(Id30_50.convertId(src.getContextElement())); 251 if (src.hasMin()) 252 tgt.setMinElement(Integer30_50.convertInteger(src.getMinElement())); 253 if (src.hasMax()) 254 tgt.setMaxElement(String30_50.convertString(src.getMaxElement())); 255 if (src.hasType()) 256 tgt.setTypeElement(String30_50.convertString(src.getTypeElement())); 257 if (src.hasDefaultValue()) 258 tgt.setDefaultValue(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getDefaultValueElement())); 259 if (src.hasElement()) 260 tgt.setElementElement(String30_50.convertString(src.getElementElement())); 261 if (src.hasListMode()) 262 tgt.setListModeElement(convertStructureMapSourceListMode(src.getListModeElement())); 263 if (src.hasVariable()) 264 tgt.setVariableElement(Id30_50.convertId(src.getVariableElement())); 265 if (src.hasCondition()) 266 tgt.setConditionElement(String30_50.convertString(src.getConditionElement())); 267 if (src.hasCheck()) 268 tgt.setCheckElement(String30_50.convertString(src.getCheckElement())); 269 return tgt; 270 } 271 272 public static org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleSourceComponent convertStructureMapGroupRuleSourceComponent(org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleSourceComponent src) throws FHIRException { 273 if (src == null) 274 return null; 275 org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleSourceComponent tgt = new org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleSourceComponent(); 276 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 277 if (src.hasContext()) 278 tgt.setContextElement(Id30_50.convertId(src.getContextElement())); 279 if (src.hasMin()) 280 tgt.setMinElement(Integer30_50.convertInteger(src.getMinElement())); 281 if (src.hasMax()) 282 tgt.setMaxElement(String30_50.convertString(src.getMaxElement())); 283 if (src.hasType()) 284 tgt.setTypeElement(String30_50.convertString(src.getTypeElement())); 285 if (src.hasDefaultValue()) 286 tgt.setDefaultValueElement((StringType) ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getDefaultValue())); 287 if (src.hasElement()) 288 tgt.setElementElement(String30_50.convertString(src.getElementElement())); 289 if (src.hasListMode()) 290 tgt.setListModeElement(convertStructureMapSourceListMode(src.getListModeElement())); 291 if (src.hasVariable()) 292 tgt.setVariableElement(Id30_50.convertId(src.getVariableElement())); 293 if (src.hasCondition()) 294 tgt.setConditionElement(String30_50.convertString(src.getConditionElement())); 295 if (src.hasCheck()) 296 tgt.setCheckElement(String30_50.convertString(src.getCheckElement())); 297 return tgt; 298 } 299 300 public static org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleTargetComponent convertStructureMapGroupRuleTargetComponent(org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleTargetComponent src) throws FHIRException { 301 if (src == null) 302 return null; 303 org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleTargetComponent tgt = new org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleTargetComponent(); 304 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 305 if (src.hasContext()) 306 tgt.setContextElement(Id30_50.convertIdToString(src.getContextElement())); 307 if (src.hasContextType() && src.getContextType() != org.hl7.fhir.dstu3.model.StructureMap.StructureMapContextType.VARIABLE) 308 throw new Error("This conversion is not supported. Consult code maintainers"); // this should never happens - no one knows what the intent was here. 309 if (src.hasElement()) 310 tgt.setElementElement(String30_50.convertString(src.getElementElement())); 311 if (src.hasVariable()) 312 tgt.setVariableElement(Id30_50.convertId(src.getVariableElement())); 313 tgt.setListMode(src.getListMode().stream() 314 .map(StructureMap30_50::convertStructureMapTargetListMode) 315 .collect(Collectors.toList())); 316 if (src.hasListRuleId()) 317 tgt.setListRuleIdElement(Id30_50.convertId(src.getListRuleIdElement())); 318 if (src.hasTransform()) 319 tgt.setTransformElement(convertStructureMapTransform(src.getTransformElement())); 320 for (org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleTargetParameterComponent t : src.getParameter()) 321 tgt.addParameter(convertStructureMapGroupRuleTargetParameterComponent(t)); 322 return tgt; 323 } 324 325 public static org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleTargetComponent convertStructureMapGroupRuleTargetComponent(org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleTargetComponent src) throws FHIRException { 326 if (src == null) 327 return null; 328 org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleTargetComponent tgt = new org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleTargetComponent(); 329 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 330 if (src.hasContext()) 331 tgt.setContextElement(Id30_50.convertId(src.getContextElement())); 332 tgt.setContextType(org.hl7.fhir.dstu3.model.StructureMap.StructureMapContextType.VARIABLE); 333 if (src.hasElement()) 334 tgt.setElementElement(String30_50.convertString(src.getElementElement())); 335 if (src.hasVariable()) 336 tgt.setVariableElement(Id30_50.convertId(src.getVariableElement())); 337 tgt.setListMode(src.getListMode().stream() 338 .map(StructureMap30_50::convertStructureMapTargetListMode) 339 .collect(Collectors.toList())); 340 if (src.hasListRuleId()) 341 tgt.setListRuleIdElement(Id30_50.convertId(src.getListRuleIdElement())); 342 if (src.hasTransform()) 343 tgt.setTransformElement(convertStructureMapTransform(src.getTransformElement())); 344 for (org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleTargetParameterComponent t : src.getParameter()) 345 tgt.addParameter(convertStructureMapGroupRuleTargetParameterComponent(t)); 346 return tgt; 347 } 348 349 public static org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleTargetParameterComponent convertStructureMapGroupRuleTargetParameterComponent(org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleTargetParameterComponent src) throws FHIRException { 350 if (src == null) 351 return null; 352 org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleTargetParameterComponent tgt = new org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleTargetParameterComponent(); 353 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 354 if (src.hasValue()) 355 tgt.setValue(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getValue())); 356 return tgt; 357 } 358 359 public static org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleTargetParameterComponent convertStructureMapGroupRuleTargetParameterComponent(org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupRuleTargetParameterComponent src) throws FHIRException { 360 if (src == null) 361 return null; 362 org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleTargetParameterComponent tgt = new org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleTargetParameterComponent(); 363 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 364 if (src.hasValue()) 365 tgt.setValue(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getValue())); 366 return tgt; 367 } 368 369 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureMap.StructureMapGroupTypeMode> convertStructureMapGroupTypeMode(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupTypeMode> src) throws FHIRException { 370 if (src == null || src.isEmpty()) 371 return null; 372 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureMap.StructureMapGroupTypeMode> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.StructureMap.StructureMapGroupTypeModeEnumFactory()); 373 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 374 switch (src.getValue()) { 375 case NONE: 376 return null; 377 case TYPES: 378 tgt.setValue(org.hl7.fhir.r5.model.StructureMap.StructureMapGroupTypeMode.TYPES); 379 break; 380 case TYPEANDTYPES: 381 tgt.setValue(org.hl7.fhir.r5.model.StructureMap.StructureMapGroupTypeMode.TYPEANDTYPES); 382 break; 383 default: 384 tgt.setValue(org.hl7.fhir.r5.model.StructureMap.StructureMapGroupTypeMode.NULL); 385 break; 386 } 387 return tgt; 388 } 389 390 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupTypeMode> convertStructureMapGroupTypeMode(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureMap.StructureMapGroupTypeMode> src) throws FHIRException { 391 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupTypeMode> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupTypeModeEnumFactory()); 392 if (src == null || src.isEmpty()) { 393 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupTypeMode.NONE); 394 return tgt; 395 } 396 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 397 switch (src.getValue()) { 398 case TYPES: 399 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupTypeMode.TYPES); 400 break; 401 case TYPEANDTYPES: 402 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupTypeMode.TYPEANDTYPES); 403 break; 404 default: 405 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapGroupTypeMode.NULL); 406 break; 407 } 408 return tgt; 409 } 410 411 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureMap.StructureMapInputMode> convertStructureMapInputMode(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureMap.StructureMapInputMode> src) throws FHIRException { 412 if (src == null || src.isEmpty()) 413 return null; 414 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureMap.StructureMapInputMode> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.StructureMap.StructureMapInputModeEnumFactory()); 415 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 416 switch (src.getValue()) { 417 case SOURCE: 418 tgt.setValue(org.hl7.fhir.r5.model.StructureMap.StructureMapInputMode.SOURCE); 419 break; 420 case TARGET: 421 tgt.setValue(org.hl7.fhir.r5.model.StructureMap.StructureMapInputMode.TARGET); 422 break; 423 default: 424 tgt.setValue(org.hl7.fhir.r5.model.StructureMap.StructureMapInputMode.NULL); 425 break; 426 } 427 return tgt; 428 } 429 430 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureMap.StructureMapInputMode> convertStructureMapInputMode(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureMap.StructureMapInputMode> src) throws FHIRException { 431 if (src == null || src.isEmpty()) 432 return null; 433 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureMap.StructureMapInputMode> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.StructureMap.StructureMapInputModeEnumFactory()); 434 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 435 switch (src.getValue()) { 436 case SOURCE: 437 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapInputMode.SOURCE); 438 break; 439 case TARGET: 440 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapInputMode.TARGET); 441 break; 442 default: 443 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapInputMode.NULL); 444 break; 445 } 446 return tgt; 447 } 448 449 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureMap.StructureMapModelMode> convertStructureMapModelMode(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureMap.StructureMapModelMode> src) throws FHIRException { 450 if (src == null || src.isEmpty()) 451 return null; 452 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureMap.StructureMapModelMode> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.StructureMap.StructureMapModelModeEnumFactory()); 453 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 454 switch (src.getValue()) { 455 case SOURCE: 456 tgt.setValue(org.hl7.fhir.r5.model.StructureMap.StructureMapModelMode.SOURCE); 457 break; 458 case QUERIED: 459 tgt.setValue(org.hl7.fhir.r5.model.StructureMap.StructureMapModelMode.QUERIED); 460 break; 461 case TARGET: 462 tgt.setValue(org.hl7.fhir.r5.model.StructureMap.StructureMapModelMode.TARGET); 463 break; 464 case PRODUCED: 465 tgt.setValue(org.hl7.fhir.r5.model.StructureMap.StructureMapModelMode.PRODUCED); 466 break; 467 default: 468 tgt.setValue(org.hl7.fhir.r5.model.StructureMap.StructureMapModelMode.NULL); 469 break; 470 } 471 return tgt; 472 } 473 474 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureMap.StructureMapModelMode> convertStructureMapModelMode(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureMap.StructureMapModelMode> src) throws FHIRException { 475 if (src == null || src.isEmpty()) 476 return null; 477 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureMap.StructureMapModelMode> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.StructureMap.StructureMapModelModeEnumFactory()); 478 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 479 switch (src.getValue()) { 480 case SOURCE: 481 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapModelMode.SOURCE); 482 break; 483 case QUERIED: 484 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapModelMode.QUERIED); 485 break; 486 case TARGET: 487 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapModelMode.TARGET); 488 break; 489 case PRODUCED: 490 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapModelMode.PRODUCED); 491 break; 492 default: 493 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapModelMode.NULL); 494 break; 495 } 496 return tgt; 497 } 498 499 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureMap.StructureMapSourceListMode> convertStructureMapSourceListMode(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureMap.StructureMapSourceListMode> src) throws FHIRException { 500 if (src == null || src.isEmpty()) 501 return null; 502 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureMap.StructureMapSourceListMode> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.StructureMap.StructureMapSourceListModeEnumFactory()); 503 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 504 switch (src.getValue()) { 505 case FIRST: 506 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapSourceListMode.FIRST); 507 break; 508 case NOTFIRST: 509 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapSourceListMode.NOTFIRST); 510 break; 511 case LAST: 512 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapSourceListMode.LAST); 513 break; 514 case NOTLAST: 515 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapSourceListMode.NOTLAST); 516 break; 517 case ONLYONE: 518 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapSourceListMode.ONLYONE); 519 break; 520 default: 521 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapSourceListMode.NULL); 522 break; 523 } 524 return tgt; 525 } 526 527 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureMap.StructureMapSourceListMode> convertStructureMapSourceListMode(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureMap.StructureMapSourceListMode> src) throws FHIRException { 528 if (src == null || src.isEmpty()) 529 return null; 530 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureMap.StructureMapSourceListMode> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.StructureMap.StructureMapSourceListModeEnumFactory()); 531 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 532 switch (src.getValue()) { 533 case FIRST: 534 tgt.setValue(org.hl7.fhir.r5.model.StructureMap.StructureMapSourceListMode.FIRST); 535 break; 536 case NOTFIRST: 537 tgt.setValue(org.hl7.fhir.r5.model.StructureMap.StructureMapSourceListMode.NOTFIRST); 538 break; 539 case LAST: 540 tgt.setValue(org.hl7.fhir.r5.model.StructureMap.StructureMapSourceListMode.LAST); 541 break; 542 case NOTLAST: 543 tgt.setValue(org.hl7.fhir.r5.model.StructureMap.StructureMapSourceListMode.NOTLAST); 544 break; 545 case ONLYONE: 546 tgt.setValue(org.hl7.fhir.r5.model.StructureMap.StructureMapSourceListMode.ONLYONE); 547 break; 548 default: 549 tgt.setValue(org.hl7.fhir.r5.model.StructureMap.StructureMapSourceListMode.NULL); 550 break; 551 } 552 return tgt; 553 } 554 555 public static org.hl7.fhir.dstu3.model.StructureMap.StructureMapStructureComponent convertStructureMapStructureComponent(org.hl7.fhir.r5.model.StructureMap.StructureMapStructureComponent src) throws FHIRException { 556 if (src == null) 557 return null; 558 org.hl7.fhir.dstu3.model.StructureMap.StructureMapStructureComponent tgt = new org.hl7.fhir.dstu3.model.StructureMap.StructureMapStructureComponent(); 559 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 560 if (src.hasUrl()) 561 tgt.setUrl(src.getUrl()); 562 if (src.hasMode()) 563 tgt.setModeElement(convertStructureMapModelMode(src.getModeElement())); 564 if (src.hasAlias()) 565 tgt.setAliasElement(String30_50.convertString(src.getAliasElement())); 566 if (src.hasDocumentation()) 567 tgt.setDocumentationElement(String30_50.convertString(src.getDocumentationElement())); 568 return tgt; 569 } 570 571 public static org.hl7.fhir.r5.model.StructureMap.StructureMapStructureComponent convertStructureMapStructureComponent(org.hl7.fhir.dstu3.model.StructureMap.StructureMapStructureComponent src) throws FHIRException { 572 if (src == null) 573 return null; 574 org.hl7.fhir.r5.model.StructureMap.StructureMapStructureComponent tgt = new org.hl7.fhir.r5.model.StructureMap.StructureMapStructureComponent(); 575 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 576 if (src.hasUrl()) 577 tgt.setUrl(src.getUrl()); 578 if (src.hasMode()) 579 tgt.setModeElement(convertStructureMapModelMode(src.getModeElement())); 580 if (src.hasAlias()) 581 tgt.setAliasElement(String30_50.convertString(src.getAliasElement())); 582 if (src.hasDocumentation()) 583 tgt.setDocumentationElement(String30_50.convertString(src.getDocumentationElement())); 584 return tgt; 585 } 586 587 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureMap.StructureMapTargetListMode> convertStructureMapTargetListMode(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureMap.StructureMapTargetListMode> src) throws FHIRException { 588 if (src == null || src.isEmpty()) 589 return null; 590 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureMap.StructureMapTargetListMode> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.StructureMap.StructureMapTargetListModeEnumFactory()); 591 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 592 switch (src.getValue()) { 593 case FIRST: 594 tgt.setValue(org.hl7.fhir.r5.model.StructureMap.StructureMapTargetListMode.FIRST); 595 break; 596 case SHARE: 597 tgt.setValue(org.hl7.fhir.r5.model.StructureMap.StructureMapTargetListMode.SHARE); 598 break; 599 case LAST: 600 tgt.setValue(org.hl7.fhir.r5.model.StructureMap.StructureMapTargetListMode.LAST); 601 break; 602 case COLLATE: 603 tgt.setValue(org.hl7.fhir.r5.model.StructureMap.StructureMapTargetListMode.SINGLE); 604 break; 605 default: 606 tgt.setValue(org.hl7.fhir.r5.model.StructureMap.StructureMapTargetListMode.NULL); 607 break; 608 } 609 return tgt; 610 } 611 612 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureMap.StructureMapTargetListMode> convertStructureMapTargetListMode(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureMap.StructureMapTargetListMode> src) throws FHIRException { 613 if (src == null || src.isEmpty()) 614 return null; 615 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureMap.StructureMapTargetListMode> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.StructureMap.StructureMapTargetListModeEnumFactory()); 616 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 617 switch (src.getValue()) { 618 case FIRST: 619 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapTargetListMode.FIRST); 620 break; 621 case SHARE: 622 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapTargetListMode.SHARE); 623 break; 624 case LAST: 625 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapTargetListMode.LAST); 626 break; 627 case SINGLE: 628 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapTargetListMode.COLLATE); 629 break; 630 default: 631 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapTargetListMode.NULL); 632 break; 633 } 634 return tgt; 635 } 636 637 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureMap.StructureMapTransform> convertStructureMapTransform(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureMap.StructureMapTransform> src) throws FHIRException { 638 if (src == null || src.isEmpty()) 639 return null; 640 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureMap.StructureMapTransform> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.StructureMap.StructureMapTransformEnumFactory()); 641 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 642 switch (src.getValue()) { 643 case CREATE: 644 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapTransform.CREATE); 645 break; 646 case COPY: 647 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapTransform.COPY); 648 break; 649 case TRUNCATE: 650 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapTransform.TRUNCATE); 651 break; 652 case ESCAPE: 653 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapTransform.ESCAPE); 654 break; 655 case CAST: 656 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapTransform.CAST); 657 break; 658 case APPEND: 659 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapTransform.APPEND); 660 break; 661 case TRANSLATE: 662 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapTransform.TRANSLATE); 663 break; 664 case REFERENCE: 665 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapTransform.REFERENCE); 666 break; 667 case DATEOP: 668 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapTransform.DATEOP); 669 break; 670 case UUID: 671 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapTransform.UUID); 672 break; 673 case POINTER: 674 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapTransform.POINTER); 675 break; 676 case EVALUATE: 677 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapTransform.EVALUATE); 678 break; 679 case CC: 680 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapTransform.CC); 681 break; 682 case C: 683 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapTransform.C); 684 break; 685 case QTY: 686 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapTransform.QTY); 687 break; 688 case ID: 689 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapTransform.ID); 690 break; 691 case CP: 692 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapTransform.CP); 693 break; 694 default: 695 tgt.setValue(org.hl7.fhir.dstu3.model.StructureMap.StructureMapTransform.NULL); 696 break; 697 } 698 return tgt; 699 } 700 701 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureMap.StructureMapTransform> convertStructureMapTransform(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.StructureMap.StructureMapTransform> src) throws FHIRException { 702 if (src == null || src.isEmpty()) 703 return null; 704 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureMap.StructureMapTransform> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.StructureMap.StructureMapTransformEnumFactory()); 705 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 706 switch (src.getValue()) { 707 case CREATE: 708 tgt.setValue(org.hl7.fhir.r5.model.StructureMap.StructureMapTransform.CREATE); 709 break; 710 case COPY: 711 tgt.setValue(org.hl7.fhir.r5.model.StructureMap.StructureMapTransform.COPY); 712 break; 713 case TRUNCATE: 714 tgt.setValue(org.hl7.fhir.r5.model.StructureMap.StructureMapTransform.TRUNCATE); 715 break; 716 case ESCAPE: 717 tgt.setValue(org.hl7.fhir.r5.model.StructureMap.StructureMapTransform.ESCAPE); 718 break; 719 case CAST: 720 tgt.setValue(org.hl7.fhir.r5.model.StructureMap.StructureMapTransform.CAST); 721 break; 722 case APPEND: 723 tgt.setValue(org.hl7.fhir.r5.model.StructureMap.StructureMapTransform.APPEND); 724 break; 725 case TRANSLATE: 726 tgt.setValue(org.hl7.fhir.r5.model.StructureMap.StructureMapTransform.TRANSLATE); 727 break; 728 case REFERENCE: 729 tgt.setValue(org.hl7.fhir.r5.model.StructureMap.StructureMapTransform.REFERENCE); 730 break; 731 case DATEOP: 732 tgt.setValue(org.hl7.fhir.r5.model.StructureMap.StructureMapTransform.DATEOP); 733 break; 734 case UUID: 735 tgt.setValue(org.hl7.fhir.r5.model.StructureMap.StructureMapTransform.UUID); 736 break; 737 case POINTER: 738 tgt.setValue(org.hl7.fhir.r5.model.StructureMap.StructureMapTransform.POINTER); 739 break; 740 case EVALUATE: 741 tgt.setValue(org.hl7.fhir.r5.model.StructureMap.StructureMapTransform.EVALUATE); 742 break; 743 case CC: 744 tgt.setValue(org.hl7.fhir.r5.model.StructureMap.StructureMapTransform.CC); 745 break; 746 case C: 747 tgt.setValue(org.hl7.fhir.r5.model.StructureMap.StructureMapTransform.C); 748 break; 749 case QTY: 750 tgt.setValue(org.hl7.fhir.r5.model.StructureMap.StructureMapTransform.QTY); 751 break; 752 case ID: 753 tgt.setValue(org.hl7.fhir.r5.model.StructureMap.StructureMapTransform.ID); 754 break; 755 case CP: 756 tgt.setValue(org.hl7.fhir.r5.model.StructureMap.StructureMapTransform.CP); 757 break; 758 default: 759 tgt.setValue(org.hl7.fhir.r5.model.StructureMap.StructureMapTransform.NULL); 760 break; 761 } 762 return tgt; 763 } 764}