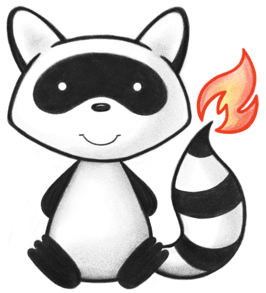
001package org.hl7.fhir.convertors.conv30_50.resources30_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_50; 004import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.CodeableConcept30_50; 005import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Identifier30_50; 006import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Ratio30_50; 007import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.SimpleQuantity30_50; 008import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.DateTime30_50; 009import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.String30_50; 010import org.hl7.fhir.exceptions.FHIRException; 011import org.hl7.fhir.r5.model.Enumeration; 012import org.hl7.fhir.r5.model.Identifier; 013import org.hl7.fhir.r5.model.Substance; 014 015public class Substance30_50 { 016 017 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Substance.FHIRSubstanceStatus> convertFHIRSubstanceStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Substance.FHIRSubstanceStatus> src) throws FHIRException { 018 if (src == null || src.isEmpty()) 019 return null; 020 Enumeration<Substance.FHIRSubstanceStatus> tgt = new Enumeration<>(new Substance.FHIRSubstanceStatusEnumFactory()); 021 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 022 if (src.getValue() == null) { 023 tgt.setValue(null); 024 } else { 025 switch (src.getValue()) { 026 case ACTIVE: 027 tgt.setValue(Substance.FHIRSubstanceStatus.ACTIVE); 028 break; 029 case INACTIVE: 030 tgt.setValue(Substance.FHIRSubstanceStatus.INACTIVE); 031 break; 032 case ENTEREDINERROR: 033 tgt.setValue(Substance.FHIRSubstanceStatus.ENTEREDINERROR); 034 break; 035 default: 036 tgt.setValue(Substance.FHIRSubstanceStatus.NULL); 037 break; 038 } 039 } 040 return tgt; 041 } 042 043 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Substance.FHIRSubstanceStatus> convertFHIRSubstanceStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Substance.FHIRSubstanceStatus> src) throws FHIRException { 044 if (src == null || src.isEmpty()) 045 return null; 046 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Substance.FHIRSubstanceStatus> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Substance.FHIRSubstanceStatusEnumFactory()); 047 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 048 if (src.getValue() == null) { 049 tgt.setValue(null); 050 } else { 051 switch (src.getValue()) { 052 case ACTIVE: 053 tgt.setValue(org.hl7.fhir.dstu3.model.Substance.FHIRSubstanceStatus.ACTIVE); 054 break; 055 case INACTIVE: 056 tgt.setValue(org.hl7.fhir.dstu3.model.Substance.FHIRSubstanceStatus.INACTIVE); 057 break; 058 case ENTEREDINERROR: 059 tgt.setValue(org.hl7.fhir.dstu3.model.Substance.FHIRSubstanceStatus.ENTEREDINERROR); 060 break; 061 default: 062 tgt.setValue(org.hl7.fhir.dstu3.model.Substance.FHIRSubstanceStatus.NULL); 063 break; 064 } 065 } 066 return tgt; 067 } 068 069 public static org.hl7.fhir.r5.model.Substance convertSubstance(org.hl7.fhir.dstu3.model.Substance src) throws FHIRException { 070 if (src == null) 071 return null; 072 org.hl7.fhir.r5.model.Substance tgt = new org.hl7.fhir.r5.model.Substance(); 073 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 074 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 075 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 076 if (src.hasStatus()) 077 tgt.setStatusElement(convertFHIRSubstanceStatus(src.getStatusElement())); 078 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getCategory()) 079 tgt.addCategory(CodeableConcept30_50.convertCodeableConcept(t)); 080 if (src.hasCode()) 081 tgt.getCode().setConcept(CodeableConcept30_50.convertCodeableConcept(src.getCode())); 082 if (src.hasDescription()) 083 tgt.setDescriptionElement(String30_50.convertStringToMarkdown(src.getDescriptionElement())); 084 for (org.hl7.fhir.dstu3.model.Substance.SubstanceInstanceComponent t : src.getInstance()) 085 convertSubstanceInstanceComponent(t, tgt); 086 for (org.hl7.fhir.dstu3.model.Substance.SubstanceIngredientComponent t : src.getIngredient()) 087 tgt.addIngredient(convertSubstanceIngredientComponent(t)); 088 return tgt; 089 } 090 091 public static org.hl7.fhir.dstu3.model.Substance convertSubstance(org.hl7.fhir.r5.model.Substance src) throws FHIRException { 092 if (src == null) 093 return null; 094 org.hl7.fhir.dstu3.model.Substance tgt = new org.hl7.fhir.dstu3.model.Substance(); 095 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 096 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 097 tgt.addIdentifier(Identifier30_50.convertIdentifier(t)); 098 if (src.hasStatus()) 099 tgt.setStatusElement(convertFHIRSubstanceStatus(src.getStatusElement())); 100 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getCategory()) 101 tgt.addCategory(CodeableConcept30_50.convertCodeableConcept(t)); 102 if (src.getCode().hasConcept()) 103 tgt.setCode(CodeableConcept30_50.convertCodeableConcept(src.getCode().getConcept())); 104 if (src.hasDescription()) 105 tgt.setDescriptionElement(String30_50.convertString(src.getDescriptionElement())); 106 if (src.getInstance()) { 107 tgt.addInstance(convertSubstanceInstanceComponent(src)); 108 } 109 for (org.hl7.fhir.r5.model.Substance.SubstanceIngredientComponent t : src.getIngredient()) 110 tgt.addIngredient(convertSubstanceIngredientComponent(t)); 111 return tgt; 112 } 113 114 public static org.hl7.fhir.dstu3.model.Substance.SubstanceIngredientComponent convertSubstanceIngredientComponent(org.hl7.fhir.r5.model.Substance.SubstanceIngredientComponent src) throws FHIRException { 115 if (src == null) 116 return null; 117 org.hl7.fhir.dstu3.model.Substance.SubstanceIngredientComponent tgt = new org.hl7.fhir.dstu3.model.Substance.SubstanceIngredientComponent(); 118 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 119 if (src.hasQuantity()) 120 tgt.setQuantity(Ratio30_50.convertRatio(src.getQuantity())); 121 if (src.hasSubstance()) 122 tgt.setSubstance(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getSubstance())); 123 return tgt; 124 } 125 126 public static org.hl7.fhir.r5.model.Substance.SubstanceIngredientComponent convertSubstanceIngredientComponent(org.hl7.fhir.dstu3.model.Substance.SubstanceIngredientComponent src) throws FHIRException { 127 if (src == null) 128 return null; 129 org.hl7.fhir.r5.model.Substance.SubstanceIngredientComponent tgt = new org.hl7.fhir.r5.model.Substance.SubstanceIngredientComponent(); 130 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 131 if (src.hasQuantity()) 132 tgt.setQuantity(Ratio30_50.convertRatio(src.getQuantity())); 133 if (src.hasSubstance()) 134 tgt.setSubstance(ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().convertType(src.getSubstance())); 135 return tgt; 136 } 137 138 public static void convertSubstanceInstanceComponent(org.hl7.fhir.dstu3.model.Substance.SubstanceInstanceComponent src, org.hl7.fhir.r5.model.Substance tgt) throws FHIRException { 139 tgt.setInstance(true); 140 if (src.hasIdentifier()) 141 tgt.addIdentifier(Identifier30_50.convertIdentifier(src.getIdentifier())); 142 if (src.hasExpiry()) 143 tgt.setExpiryElement(DateTime30_50.convertDateTime(src.getExpiryElement())); 144 if (src.hasQuantity()) 145 tgt.setQuantity(SimpleQuantity30_50.convertSimpleQuantity(src.getQuantity())); 146 } 147 148 public static org.hl7.fhir.dstu3.model.Substance.SubstanceInstanceComponent convertSubstanceInstanceComponent(org.hl7.fhir.r5.model.Substance src) throws FHIRException { 149 org.hl7.fhir.dstu3.model.Substance.SubstanceInstanceComponent tgt = new org.hl7.fhir.dstu3.model.Substance.SubstanceInstanceComponent(); 150 for (Identifier t : src.getIdentifier()) { 151 tgt.setIdentifier(Identifier30_50.convertIdentifier(t)); 152 } 153 if (src.hasExpiry()) 154 tgt.setExpiryElement(DateTime30_50.convertDateTime(src.getExpiryElement())); 155 if (src.hasQuantity()) 156 tgt.setQuantity(SimpleQuantity30_50.convertSimpleQuantity(src.getQuantity())); 157 return tgt; 158 159 } 160}