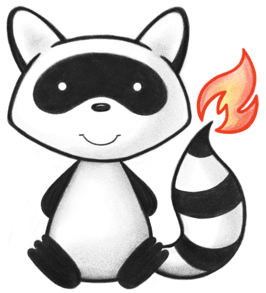
001package org.hl7.fhir.convertors.conv30_50.resources30_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext30_50; 004import org.hl7.fhir.convertors.conv30_50.datatypes30_50.ContactDetail30_50; 005import org.hl7.fhir.convertors.conv30_50.datatypes30_50.Reference30_50; 006import org.hl7.fhir.convertors.conv30_50.datatypes30_50.UsageContext30_50; 007import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.CodeableConcept30_50; 008import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Coding30_50; 009import org.hl7.fhir.convertors.conv30_50.datatypes30_50.complextypes30_50.Identifier30_50; 010import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Boolean30_50; 011import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.DateTime30_50; 012import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Id30_50; 013import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Integer30_50; 014import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.MarkDown30_50; 015import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.String30_50; 016import org.hl7.fhir.convertors.conv30_50.datatypes30_50.primitivetypes30_50.Uri30_50; 017import org.hl7.fhir.exceptions.FHIRException; 018import org.hl7.fhir.r5.model.CanonicalType; 019import org.hl7.fhir.r5.model.TestScript; 020 021public class TestScript30_50 { 022 023 public static org.hl7.fhir.r5.model.TestScript convertTestScript(org.hl7.fhir.dstu3.model.TestScript src) throws FHIRException { 024 if (src == null) 025 return null; 026 org.hl7.fhir.r5.model.TestScript tgt = new org.hl7.fhir.r5.model.TestScript(); 027 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 028 if (src.hasUrl()) 029 tgt.setUrlElement(Uri30_50.convertUri(src.getUrlElement())); 030 if (src.hasIdentifier()) 031 tgt.addIdentifier(Identifier30_50.convertIdentifier(src.getIdentifier())); 032 if (src.hasVersion()) 033 tgt.setVersionElement(String30_50.convertString(src.getVersionElement())); 034 if (src.hasName()) 035 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 036 if (src.hasTitle()) 037 tgt.setTitleElement(String30_50.convertString(src.getTitleElement())); 038 if (src.hasStatus()) 039 tgt.setStatusElement(Enumerations30_50.convertPublicationStatus(src.getStatusElement())); 040 if (src.hasExperimental()) 041 tgt.setExperimentalElement(Boolean30_50.convertBoolean(src.getExperimentalElement())); 042 if (src.hasDate()) 043 tgt.setDateElement(DateTime30_50.convertDateTime(src.getDateElement())); 044 if (src.hasPublisher()) 045 tgt.setPublisherElement(String30_50.convertString(src.getPublisherElement())); 046 for (org.hl7.fhir.dstu3.model.ContactDetail t : src.getContact()) 047 tgt.addContact(ContactDetail30_50.convertContactDetail(t)); 048 if (src.hasDescription()) 049 tgt.setDescriptionElement(MarkDown30_50.convertMarkdown(src.getDescriptionElement())); 050 for (org.hl7.fhir.dstu3.model.UsageContext t : src.getUseContext()) 051 tgt.addUseContext(UsageContext30_50.convertUsageContext(t)); 052 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getJurisdiction()) 053 tgt.addJurisdiction(CodeableConcept30_50.convertCodeableConcept(t)); 054 if (src.hasPurpose()) 055 tgt.setPurposeElement(MarkDown30_50.convertMarkdown(src.getPurposeElement())); 056 if (src.hasCopyright()) 057 tgt.setCopyrightElement(MarkDown30_50.convertMarkdown(src.getCopyrightElement())); 058 for (org.hl7.fhir.dstu3.model.TestScript.TestScriptOriginComponent t : src.getOrigin()) 059 tgt.addOrigin(convertTestScriptOriginComponent(t)); 060 for (org.hl7.fhir.dstu3.model.TestScript.TestScriptDestinationComponent t : src.getDestination()) 061 tgt.addDestination(convertTestScriptDestinationComponent(t)); 062 if (src.hasMetadata()) 063 tgt.setMetadata(convertTestScriptMetadataComponent(src.getMetadata())); 064 for (org.hl7.fhir.dstu3.model.TestScript.TestScriptFixtureComponent t : src.getFixture()) 065 tgt.addFixture(convertTestScriptFixtureComponent(t)); 066 for (org.hl7.fhir.dstu3.model.Reference t : src.getProfile()) tgt.getProfile().add(Reference30_50.convertReferenceToCanonical(t)); 067 for (org.hl7.fhir.dstu3.model.TestScript.TestScriptVariableComponent t : src.getVariable()) 068 tgt.addVariable(convertTestScriptVariableComponent(t)); 069 if (src.hasSetup()) 070 tgt.setSetup(convertTestScriptSetupComponent(src.getSetup())); 071 for (org.hl7.fhir.dstu3.model.TestScript.TestScriptTestComponent t : src.getTest()) 072 tgt.addTest(convertTestScriptTestComponent(t)); 073 if (src.hasTeardown()) 074 tgt.setTeardown(convertTestScriptTeardownComponent(src.getTeardown())); 075 return tgt; 076 } 077 078 public static org.hl7.fhir.dstu3.model.TestScript convertTestScript(org.hl7.fhir.r5.model.TestScript src) throws FHIRException { 079 if (src == null) 080 return null; 081 org.hl7.fhir.dstu3.model.TestScript tgt = new org.hl7.fhir.dstu3.model.TestScript(); 082 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyDomainResource(src, tgt); 083 if (src.hasUrl()) 084 tgt.setUrlElement(Uri30_50.convertUri(src.getUrlElement())); 085 if (src.hasIdentifier()) 086 tgt.setIdentifier(Identifier30_50.convertIdentifier(src.getIdentifierFirstRep())); 087 if (src.hasVersion()) 088 tgt.setVersionElement(String30_50.convertString(src.getVersionElement())); 089 if (src.hasName()) 090 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 091 if (src.hasTitle()) 092 tgt.setTitleElement(String30_50.convertString(src.getTitleElement())); 093 if (src.hasStatus()) 094 tgt.setStatusElement(Enumerations30_50.convertPublicationStatus(src.getStatusElement())); 095 if (src.hasExperimental()) 096 tgt.setExperimentalElement(Boolean30_50.convertBoolean(src.getExperimentalElement())); 097 if (src.hasDate()) 098 tgt.setDateElement(DateTime30_50.convertDateTime(src.getDateElement())); 099 if (src.hasPublisher()) 100 tgt.setPublisherElement(String30_50.convertString(src.getPublisherElement())); 101 for (org.hl7.fhir.r5.model.ContactDetail t : src.getContact()) 102 tgt.addContact(ContactDetail30_50.convertContactDetail(t)); 103 if (src.hasDescription()) 104 tgt.setDescriptionElement(MarkDown30_50.convertMarkdown(src.getDescriptionElement())); 105 for (org.hl7.fhir.r5.model.UsageContext t : src.getUseContext()) 106 tgt.addUseContext(UsageContext30_50.convertUsageContext(t)); 107 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getJurisdiction()) 108 tgt.addJurisdiction(CodeableConcept30_50.convertCodeableConcept(t)); 109 if (src.hasPurpose()) 110 tgt.setPurposeElement(MarkDown30_50.convertMarkdown(src.getPurposeElement())); 111 if (src.hasCopyright()) 112 tgt.setCopyrightElement(MarkDown30_50.convertMarkdown(src.getCopyrightElement())); 113 for (org.hl7.fhir.r5.model.TestScript.TestScriptOriginComponent t : src.getOrigin()) 114 tgt.addOrigin(convertTestScriptOriginComponent(t)); 115 for (org.hl7.fhir.r5.model.TestScript.TestScriptDestinationComponent t : src.getDestination()) 116 tgt.addDestination(convertTestScriptDestinationComponent(t)); 117 if (src.hasMetadata()) 118 tgt.setMetadata(convertTestScriptMetadataComponent(src.getMetadata())); 119 for (org.hl7.fhir.r5.model.TestScript.TestScriptFixtureComponent t : src.getFixture()) 120 tgt.addFixture(convertTestScriptFixtureComponent(t)); 121 for (CanonicalType t : src.getProfile()) tgt.addProfile(Reference30_50.convertCanonicalToReference(t)); 122 for (org.hl7.fhir.r5.model.TestScript.TestScriptVariableComponent t : src.getVariable()) 123 tgt.addVariable(convertTestScriptVariableComponent(t)); 124 if (src.hasSetup()) 125 tgt.setSetup(convertTestScriptSetupComponent(src.getSetup())); 126 for (org.hl7.fhir.r5.model.TestScript.TestScriptTestComponent t : src.getTest()) 127 tgt.addTest(convertTestScriptTestComponent(t)); 128 if (src.hasTeardown()) 129 tgt.setTeardown(convertTestScriptTeardownComponent(src.getTeardown())); 130 return tgt; 131 } 132 133 public static org.hl7.fhir.dstu3.model.TestScript.TestScriptDestinationComponent convertTestScriptDestinationComponent(org.hl7.fhir.r5.model.TestScript.TestScriptDestinationComponent src) throws FHIRException { 134 if (src == null) 135 return null; 136 org.hl7.fhir.dstu3.model.TestScript.TestScriptDestinationComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TestScriptDestinationComponent(); 137 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 138 if (src.hasIndex()) 139 tgt.setIndexElement(Integer30_50.convertInteger(src.getIndexElement())); 140 if (src.hasProfile()) 141 tgt.setProfile(Coding30_50.convertCoding(src.getProfile())); 142 return tgt; 143 } 144 145 public static org.hl7.fhir.r5.model.TestScript.TestScriptDestinationComponent convertTestScriptDestinationComponent(org.hl7.fhir.dstu3.model.TestScript.TestScriptDestinationComponent src) throws FHIRException { 146 if (src == null) 147 return null; 148 org.hl7.fhir.r5.model.TestScript.TestScriptDestinationComponent tgt = new org.hl7.fhir.r5.model.TestScript.TestScriptDestinationComponent(); 149 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 150 if (src.hasIndex()) 151 tgt.setIndexElement(Integer30_50.convertInteger(src.getIndexElement())); 152 if (src.hasProfile()) 153 tgt.setProfile(Coding30_50.convertCoding(src.getProfile())); 154 return tgt; 155 } 156 157 public static org.hl7.fhir.dstu3.model.TestScript.TestScriptFixtureComponent convertTestScriptFixtureComponent(org.hl7.fhir.r5.model.TestScript.TestScriptFixtureComponent src) throws FHIRException { 158 if (src == null) 159 return null; 160 org.hl7.fhir.dstu3.model.TestScript.TestScriptFixtureComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TestScriptFixtureComponent(); 161 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 162 if (src.hasAutocreate()) 163 tgt.setAutocreateElement(Boolean30_50.convertBoolean(src.getAutocreateElement())); 164 if (src.hasAutodelete()) 165 tgt.setAutodeleteElement(Boolean30_50.convertBoolean(src.getAutodeleteElement())); 166 if (src.hasResource()) 167 tgt.setResource(Reference30_50.convertReference(src.getResource())); 168 return tgt; 169 } 170 171 public static org.hl7.fhir.r5.model.TestScript.TestScriptFixtureComponent convertTestScriptFixtureComponent(org.hl7.fhir.dstu3.model.TestScript.TestScriptFixtureComponent src) throws FHIRException { 172 if (src == null) 173 return null; 174 org.hl7.fhir.r5.model.TestScript.TestScriptFixtureComponent tgt = new org.hl7.fhir.r5.model.TestScript.TestScriptFixtureComponent(); 175 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 176 if (src.hasAutocreate()) 177 tgt.setAutocreateElement(Boolean30_50.convertBoolean(src.getAutocreateElement())); 178 if (src.hasAutodelete()) 179 tgt.setAutodeleteElement(Boolean30_50.convertBoolean(src.getAutodeleteElement())); 180 if (src.hasResource()) 181 tgt.setResource(Reference30_50.convertReference(src.getResource())); 182 return tgt; 183 } 184 185 public static org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataCapabilityComponent convertTestScriptMetadataCapabilityComponent(org.hl7.fhir.r5.model.TestScript.TestScriptMetadataCapabilityComponent src) throws FHIRException { 186 if (src == null) 187 return null; 188 org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataCapabilityComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataCapabilityComponent(); 189 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 190 if (src.hasRequired()) 191 tgt.setRequiredElement(Boolean30_50.convertBoolean(src.getRequiredElement())); 192 if (src.hasValidated()) 193 tgt.setValidatedElement(Boolean30_50.convertBoolean(src.getValidatedElement())); 194 if (src.hasDescription()) 195 tgt.setDescriptionElement(String30_50.convertString(src.getDescriptionElement())); 196 for (org.hl7.fhir.r5.model.IntegerType t : src.getOrigin()) tgt.addOrigin(t.getValue()); 197 if (src.hasDestination()) 198 tgt.setDestinationElement(Integer30_50.convertInteger(src.getDestinationElement())); 199 for (org.hl7.fhir.r5.model.UriType t : src.getLink()) tgt.addLink(t.getValue()); 200 if (src.hasCapabilities()) 201 tgt.setCapabilities(Reference30_50.convertCanonicalToReference(src.getCapabilitiesElement())); 202 return tgt; 203 } 204 205 public static org.hl7.fhir.r5.model.TestScript.TestScriptMetadataCapabilityComponent convertTestScriptMetadataCapabilityComponent(org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataCapabilityComponent src) throws FHIRException { 206 if (src == null) 207 return null; 208 org.hl7.fhir.r5.model.TestScript.TestScriptMetadataCapabilityComponent tgt = new org.hl7.fhir.r5.model.TestScript.TestScriptMetadataCapabilityComponent(); 209 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 210 if (src.hasRequired()) 211 tgt.setRequiredElement(Boolean30_50.convertBoolean(src.getRequiredElement())); 212 if (src.hasValidated()) 213 tgt.setValidatedElement(Boolean30_50.convertBoolean(src.getValidatedElement())); 214 if (src.hasDescription()) 215 tgt.setDescriptionElement(String30_50.convertString(src.getDescriptionElement())); 216 for (org.hl7.fhir.dstu3.model.IntegerType t : src.getOrigin()) tgt.addOrigin(t.getValue()); 217 if (src.hasDestination()) 218 tgt.setDestinationElement(Integer30_50.convertInteger(src.getDestinationElement())); 219 for (org.hl7.fhir.dstu3.model.UriType t : src.getLink()) tgt.addLink(t.getValue()); 220 if (src.hasCapabilities()) 221 tgt.setCapabilitiesElement(Reference30_50.convertReferenceToCanonical(src.getCapabilities())); 222 return tgt; 223 } 224 225 public static org.hl7.fhir.r5.model.TestScript.TestScriptMetadataComponent convertTestScriptMetadataComponent(org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataComponent src) throws FHIRException { 226 if (src == null) 227 return null; 228 org.hl7.fhir.r5.model.TestScript.TestScriptMetadataComponent tgt = new org.hl7.fhir.r5.model.TestScript.TestScriptMetadataComponent(); 229 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 230 for (org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataLinkComponent t : src.getLink()) 231 tgt.addLink(convertTestScriptMetadataLinkComponent(t)); 232 for (org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataCapabilityComponent t : src.getCapability()) 233 tgt.addCapability(convertTestScriptMetadataCapabilityComponent(t)); 234 return tgt; 235 } 236 237 public static org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataComponent convertTestScriptMetadataComponent(org.hl7.fhir.r5.model.TestScript.TestScriptMetadataComponent src) throws FHIRException { 238 if (src == null) 239 return null; 240 org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataComponent(); 241 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 242 for (org.hl7.fhir.r5.model.TestScript.TestScriptMetadataLinkComponent t : src.getLink()) 243 tgt.addLink(convertTestScriptMetadataLinkComponent(t)); 244 for (org.hl7.fhir.r5.model.TestScript.TestScriptMetadataCapabilityComponent t : src.getCapability()) 245 tgt.addCapability(convertTestScriptMetadataCapabilityComponent(t)); 246 return tgt; 247 } 248 249 public static org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataLinkComponent convertTestScriptMetadataLinkComponent(org.hl7.fhir.r5.model.TestScript.TestScriptMetadataLinkComponent src) throws FHIRException { 250 if (src == null) 251 return null; 252 org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataLinkComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataLinkComponent(); 253 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 254 if (src.hasUrl()) 255 tgt.setUrlElement(Uri30_50.convertUri(src.getUrlElement())); 256 if (src.hasDescription()) 257 tgt.setDescriptionElement(String30_50.convertString(src.getDescriptionElement())); 258 return tgt; 259 } 260 261 public static org.hl7.fhir.r5.model.TestScript.TestScriptMetadataLinkComponent convertTestScriptMetadataLinkComponent(org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataLinkComponent src) throws FHIRException { 262 if (src == null) 263 return null; 264 org.hl7.fhir.r5.model.TestScript.TestScriptMetadataLinkComponent tgt = new org.hl7.fhir.r5.model.TestScript.TestScriptMetadataLinkComponent(); 265 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 266 if (src.hasUrl()) 267 tgt.setUrlElement(Uri30_50.convertUri(src.getUrlElement())); 268 if (src.hasDescription()) 269 tgt.setDescriptionElement(String30_50.convertString(src.getDescriptionElement())); 270 return tgt; 271 } 272 273 public static org.hl7.fhir.dstu3.model.TestScript.TestScriptOriginComponent convertTestScriptOriginComponent(org.hl7.fhir.r5.model.TestScript.TestScriptOriginComponent src) throws FHIRException { 274 if (src == null) 275 return null; 276 org.hl7.fhir.dstu3.model.TestScript.TestScriptOriginComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TestScriptOriginComponent(); 277 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 278 if (src.hasIndex()) 279 tgt.setIndexElement(Integer30_50.convertInteger(src.getIndexElement())); 280 if (src.hasProfile()) 281 tgt.setProfile(Coding30_50.convertCoding(src.getProfile())); 282 return tgt; 283 } 284 285 public static org.hl7.fhir.r5.model.TestScript.TestScriptOriginComponent convertTestScriptOriginComponent(org.hl7.fhir.dstu3.model.TestScript.TestScriptOriginComponent src) throws FHIRException { 286 if (src == null) 287 return null; 288 org.hl7.fhir.r5.model.TestScript.TestScriptOriginComponent tgt = new org.hl7.fhir.r5.model.TestScript.TestScriptOriginComponent(); 289 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 290 if (src.hasIndex()) 291 tgt.setIndexElement(Integer30_50.convertInteger(src.getIndexElement())); 292 if (src.hasProfile()) 293 tgt.setProfile(Coding30_50.convertCoding(src.getProfile())); 294 return tgt; 295 } 296 297 public static org.hl7.fhir.r5.model.TestScript.TestScriptSetupComponent convertTestScriptSetupComponent(org.hl7.fhir.dstu3.model.TestScript.TestScriptSetupComponent src) throws FHIRException { 298 if (src == null) 299 return null; 300 org.hl7.fhir.r5.model.TestScript.TestScriptSetupComponent tgt = new org.hl7.fhir.r5.model.TestScript.TestScriptSetupComponent(); 301 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 302 for (org.hl7.fhir.dstu3.model.TestScript.SetupActionComponent t : src.getAction()) 303 tgt.addAction(convertSetupActionComponent(t)); 304 return tgt; 305 } 306 307 public static org.hl7.fhir.dstu3.model.TestScript.TestScriptSetupComponent convertTestScriptSetupComponent(org.hl7.fhir.r5.model.TestScript.TestScriptSetupComponent src) throws FHIRException { 308 if (src == null) 309 return null; 310 org.hl7.fhir.dstu3.model.TestScript.TestScriptSetupComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TestScriptSetupComponent(); 311 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 312 for (org.hl7.fhir.r5.model.TestScript.SetupActionComponent t : src.getAction()) 313 tgt.addAction(convertSetupActionComponent(t)); 314 return tgt; 315 } 316 317 public static org.hl7.fhir.r5.model.TestScript.TestScriptTeardownComponent convertTestScriptTeardownComponent(org.hl7.fhir.dstu3.model.TestScript.TestScriptTeardownComponent src) throws FHIRException { 318 if (src == null) 319 return null; 320 org.hl7.fhir.r5.model.TestScript.TestScriptTeardownComponent tgt = new org.hl7.fhir.r5.model.TestScript.TestScriptTeardownComponent(); 321 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 322 for (org.hl7.fhir.dstu3.model.TestScript.TeardownActionComponent t : src.getAction()) 323 tgt.addAction(convertTeardownActionComponent(t)); 324 return tgt; 325 } 326 327 public static org.hl7.fhir.dstu3.model.TestScript.TestScriptTeardownComponent convertTestScriptTeardownComponent(org.hl7.fhir.r5.model.TestScript.TestScriptTeardownComponent src) throws FHIRException { 328 if (src == null) 329 return null; 330 org.hl7.fhir.dstu3.model.TestScript.TestScriptTeardownComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TestScriptTeardownComponent(); 331 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 332 for (org.hl7.fhir.r5.model.TestScript.TeardownActionComponent t : src.getAction()) 333 tgt.addAction(convertTeardownActionComponent(t)); 334 return tgt; 335 } 336 337 public static org.hl7.fhir.dstu3.model.TestScript.TestScriptTestComponent convertTestScriptTestComponent(org.hl7.fhir.r5.model.TestScript.TestScriptTestComponent src) throws FHIRException { 338 if (src == null) 339 return null; 340 org.hl7.fhir.dstu3.model.TestScript.TestScriptTestComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TestScriptTestComponent(); 341 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 342 if (src.hasName()) 343 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 344 if (src.hasDescription()) 345 tgt.setDescriptionElement(String30_50.convertString(src.getDescriptionElement())); 346 for (org.hl7.fhir.r5.model.TestScript.TestActionComponent t : src.getAction()) 347 tgt.addAction(convertTestActionComponent(t)); 348 return tgt; 349 } 350 351 public static org.hl7.fhir.r5.model.TestScript.TestScriptTestComponent convertTestScriptTestComponent(org.hl7.fhir.dstu3.model.TestScript.TestScriptTestComponent src) throws FHIRException { 352 if (src == null) 353 return null; 354 org.hl7.fhir.r5.model.TestScript.TestScriptTestComponent tgt = new org.hl7.fhir.r5.model.TestScript.TestScriptTestComponent(); 355 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 356 if (src.hasName()) 357 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 358 if (src.hasDescription()) 359 tgt.setDescriptionElement(String30_50.convertString(src.getDescriptionElement())); 360 for (org.hl7.fhir.dstu3.model.TestScript.TestActionComponent t : src.getAction()) 361 tgt.addAction(convertTestActionComponent(t)); 362 return tgt; 363 } 364 365 public static org.hl7.fhir.r5.model.TestScript.TestScriptVariableComponent convertTestScriptVariableComponent(org.hl7.fhir.dstu3.model.TestScript.TestScriptVariableComponent src) throws FHIRException { 366 if (src == null) 367 return null; 368 org.hl7.fhir.r5.model.TestScript.TestScriptVariableComponent tgt = new org.hl7.fhir.r5.model.TestScript.TestScriptVariableComponent(); 369 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 370 if (src.hasName()) 371 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 372 if (src.hasDefaultValue()) 373 tgt.setDefaultValueElement(String30_50.convertString(src.getDefaultValueElement())); 374 if (src.hasDescription()) 375 tgt.setDescriptionElement(String30_50.convertString(src.getDescriptionElement())); 376 if (src.hasExpression()) 377 tgt.setExpressionElement(String30_50.convertString(src.getExpressionElement())); 378 if (src.hasHeaderField()) 379 tgt.setHeaderFieldElement(String30_50.convertString(src.getHeaderFieldElement())); 380 if (src.hasHint()) 381 tgt.setHintElement(String30_50.convertString(src.getHintElement())); 382 if (src.hasPath()) 383 tgt.setPathElement(String30_50.convertString(src.getPathElement())); 384 if (src.hasSourceId()) 385 tgt.setSourceIdElement(Id30_50.convertId(src.getSourceIdElement())); 386 return tgt; 387 } 388 389 public static org.hl7.fhir.dstu3.model.TestScript.TestScriptVariableComponent convertTestScriptVariableComponent(org.hl7.fhir.r5.model.TestScript.TestScriptVariableComponent src) throws FHIRException { 390 if (src == null) 391 return null; 392 org.hl7.fhir.dstu3.model.TestScript.TestScriptVariableComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TestScriptVariableComponent(); 393 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 394 if (src.hasName()) 395 tgt.setNameElement(String30_50.convertString(src.getNameElement())); 396 if (src.hasDefaultValue()) 397 tgt.setDefaultValueElement(String30_50.convertString(src.getDefaultValueElement())); 398 if (src.hasDescription()) 399 tgt.setDescriptionElement(String30_50.convertString(src.getDescriptionElement())); 400 if (src.hasExpression()) 401 tgt.setExpressionElement(String30_50.convertString(src.getExpressionElement())); 402 if (src.hasHeaderField()) 403 tgt.setHeaderFieldElement(String30_50.convertString(src.getHeaderFieldElement())); 404 if (src.hasHint()) 405 tgt.setHintElement(String30_50.convertString(src.getHintElement())); 406 if (src.hasPath()) 407 tgt.setPathElement(String30_50.convertString(src.getPathElement())); 408 if (src.hasSourceId()) 409 tgt.setSourceIdElement(Id30_50.convertId(src.getSourceIdElement())); 410 return tgt; 411 } 412 413 public static org.hl7.fhir.r5.model.TestScript.SetupActionComponent convertSetupActionComponent(org.hl7.fhir.dstu3.model.TestScript.SetupActionComponent src) throws FHIRException { 414 if (src == null) return null; 415 org.hl7.fhir.r5.model.TestScript.SetupActionComponent tgt = new org.hl7.fhir.r5.model.TestScript.SetupActionComponent(); 416 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 417 if (src.hasOperation()) tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 418 if (src.hasAssert()) tgt.setAssert(convertSetupActionAssertComponent(src.getAssert())); 419 return tgt; 420 } 421 422 public static org.hl7.fhir.dstu3.model.TestScript.SetupActionComponent convertSetupActionComponent(org.hl7.fhir.r5.model.TestScript.SetupActionComponent src) throws FHIRException { 423 if (src == null) return null; 424 org.hl7.fhir.dstu3.model.TestScript.SetupActionComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.SetupActionComponent(); 425 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 426 if (src.hasOperation()) tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 427 if (src.hasAssert()) tgt.setAssert(convertSetupActionAssertComponent(src.getAssert())); 428 return tgt; 429 } 430 431 public static org.hl7.fhir.r5.model.TestScript.SetupActionOperationComponent convertSetupActionOperationComponent(org.hl7.fhir.dstu3.model.TestScript.SetupActionOperationComponent src) throws FHIRException { 432 if (src == null) return null; 433 org.hl7.fhir.r5.model.TestScript.SetupActionOperationComponent tgt = new org.hl7.fhir.r5.model.TestScript.SetupActionOperationComponent(); 434 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 435 if (src.hasType()) tgt.setType(Coding30_50.convertCoding(src.getType())); 436 if (src.hasResource()) 437 tgt.setResource(src.getResource()); 438 if (src.hasLabel()) tgt.setLabelElement(String30_50.convertString(src.getLabelElement())); 439 if (src.hasDescription()) tgt.setDescriptionElement(String30_50.convertString(src.getDescriptionElement())); 440 if (src.hasAccept()) tgt.setAccept(convertContentType(src.getAccept())); 441 if (src.hasContentType()) tgt.setContentType(convertContentType(src.getContentType())); 442 if (src.hasDestination()) tgt.setDestinationElement(Integer30_50.convertInteger(src.getDestinationElement())); 443 if (src.hasEncodeRequestUrl()) 444 tgt.setEncodeRequestUrlElement(Boolean30_50.convertBoolean(src.getEncodeRequestUrlElement())); 445 if (src.hasOrigin()) tgt.setOriginElement(Integer30_50.convertInteger(src.getOriginElement())); 446 if (src.hasParams()) tgt.setParamsElement(String30_50.convertString(src.getParamsElement())); 447 for (org.hl7.fhir.dstu3.model.TestScript.SetupActionOperationRequestHeaderComponent t : src.getRequestHeader()) 448 tgt.addRequestHeader(convertSetupActionOperationRequestHeaderComponent(t)); 449 if (src.hasRequestId()) tgt.setRequestIdElement(Id30_50.convertId(src.getRequestIdElement())); 450 if (src.hasResponseId()) tgt.setResponseIdElement(Id30_50.convertId(src.getResponseIdElement())); 451 if (src.hasSourceId()) tgt.setSourceIdElement(Id30_50.convertId(src.getSourceIdElement())); 452 if (src.hasTargetId()) tgt.setTargetId(src.getTargetId()); 453 if (src.hasUrl()) tgt.setUrlElement(String30_50.convertString(src.getUrlElement())); 454 return tgt; 455 } 456 457 public static org.hl7.fhir.dstu3.model.TestScript.SetupActionOperationComponent convertSetupActionOperationComponent(org.hl7.fhir.r5.model.TestScript.SetupActionOperationComponent src) throws FHIRException { 458 if (src == null) return null; 459 org.hl7.fhir.dstu3.model.TestScript.SetupActionOperationComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.SetupActionOperationComponent(); 460 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 461 if (src.hasType()) tgt.setType(Coding30_50.convertCoding(src.getType())); 462 if (src.hasResource()) tgt.setResource(src.getResource()); 463 if (src.hasLabel()) tgt.setLabelElement(String30_50.convertString(src.getLabelElement())); 464 if (src.hasDescription()) tgt.setDescriptionElement(String30_50.convertString(src.getDescriptionElement())); 465 if (src.hasAccept()) tgt.setAccept(convertContentType(src.getAccept())); 466 if (src.hasContentType()) tgt.setContentType(convertContentType(src.getContentType())); 467 if (src.hasDestination()) tgt.setDestinationElement(Integer30_50.convertInteger(src.getDestinationElement())); 468 if (src.hasEncodeRequestUrl()) 469 tgt.setEncodeRequestUrlElement(Boolean30_50.convertBoolean(src.getEncodeRequestUrlElement())); 470 if (src.hasOrigin()) tgt.setOriginElement(Integer30_50.convertInteger(src.getOriginElement())); 471 if (src.hasParams()) tgt.setParamsElement(String30_50.convertString(src.getParamsElement())); 472 for (org.hl7.fhir.r5.model.TestScript.SetupActionOperationRequestHeaderComponent t : src.getRequestHeader()) 473 tgt.addRequestHeader(convertSetupActionOperationRequestHeaderComponent(t)); 474 if (src.hasRequestId()) tgt.setRequestIdElement(Id30_50.convertId(src.getRequestIdElement())); 475 if (src.hasResponseId()) tgt.setResponseIdElement(Id30_50.convertId(src.getResponseIdElement())); 476 if (src.hasSourceId()) tgt.setSourceIdElement(Id30_50.convertId(src.getSourceIdElement())); 477 if (src.hasTargetId()) tgt.setTargetId(src.getTargetId()); 478 if (src.hasUrl()) tgt.setUrlElement(String30_50.convertString(src.getUrlElement())); 479 return tgt; 480 } 481 482 static public String convertContentType(org.hl7.fhir.dstu3.model.TestScript.ContentType src) throws FHIRException { 483 if (src == null) return null; 484 switch (src) { 485 case XML: 486 return "application/fhir+xml"; 487 case JSON: 488 return "application/fhir+json"; 489 case TTL: 490 return "text/turtle"; 491 case NONE: 492 return null; 493 default: 494 return null; 495 } 496 } 497 498 static public org.hl7.fhir.dstu3.model.TestScript.ContentType convertContentType(String src) throws FHIRException { 499 if (src == null) return null; 500 if (src.contains("xml")) return org.hl7.fhir.dstu3.model.TestScript.ContentType.XML; 501 if (src.contains("json")) return org.hl7.fhir.dstu3.model.TestScript.ContentType.JSON; 502 if (src.contains("tu")) return org.hl7.fhir.dstu3.model.TestScript.ContentType.TTL; 503 return org.hl7.fhir.dstu3.model.TestScript.ContentType.NONE; 504 } 505 506 public static org.hl7.fhir.r5.model.TestScript.SetupActionOperationRequestHeaderComponent convertSetupActionOperationRequestHeaderComponent(org.hl7.fhir.dstu3.model.TestScript.SetupActionOperationRequestHeaderComponent src) throws FHIRException { 507 if (src == null) return null; 508 org.hl7.fhir.r5.model.TestScript.SetupActionOperationRequestHeaderComponent tgt = new org.hl7.fhir.r5.model.TestScript.SetupActionOperationRequestHeaderComponent(); 509 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 510 if (src.hasField()) tgt.setFieldElement(String30_50.convertString(src.getFieldElement())); 511 if (src.hasValue()) tgt.setValueElement(String30_50.convertString(src.getValueElement())); 512 return tgt; 513 } 514 515 public static org.hl7.fhir.dstu3.model.TestScript.SetupActionOperationRequestHeaderComponent convertSetupActionOperationRequestHeaderComponent(org.hl7.fhir.r5.model.TestScript.SetupActionOperationRequestHeaderComponent src) throws FHIRException { 516 if (src == null) return null; 517 org.hl7.fhir.dstu3.model.TestScript.SetupActionOperationRequestHeaderComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.SetupActionOperationRequestHeaderComponent(); 518 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 519 if (src.hasField()) tgt.setFieldElement(String30_50.convertString(src.getFieldElement())); 520 if (src.hasValue()) tgt.setValueElement(String30_50.convertString(src.getValueElement())); 521 return tgt; 522 } 523 524 public static org.hl7.fhir.r5.model.TestScript.SetupActionAssertComponent convertSetupActionAssertComponent(org.hl7.fhir.dstu3.model.TestScript.SetupActionAssertComponent src) throws FHIRException { 525 if (src == null) return null; 526 org.hl7.fhir.r5.model.TestScript.SetupActionAssertComponent tgt = new org.hl7.fhir.r5.model.TestScript.SetupActionAssertComponent(); 527 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 528 if (src.hasLabel()) tgt.setLabelElement(String30_50.convertString(src.getLabelElement())); 529 if (src.hasDescription()) tgt.setDescriptionElement(String30_50.convertString(src.getDescriptionElement())); 530 if (src.hasDirection()) tgt.setDirectionElement(convertAssertionDirectionType(src.getDirectionElement())); 531 if (src.hasCompareToSourceId()) 532 tgt.setCompareToSourceIdElement(String30_50.convertString(src.getCompareToSourceIdElement())); 533 if (src.hasCompareToSourceExpression()) 534 tgt.setCompareToSourceExpressionElement(String30_50.convertString(src.getCompareToSourceExpressionElement())); 535 if (src.hasCompareToSourcePath()) 536 tgt.setCompareToSourcePathElement(String30_50.convertString(src.getCompareToSourcePathElement())); 537 if (src.hasContentType()) tgt.setContentType(convertContentType(src.getContentType())); 538 if (src.hasExpression()) tgt.setExpressionElement(String30_50.convertString(src.getExpressionElement())); 539 if (src.hasHeaderField()) tgt.setHeaderFieldElement(String30_50.convertString(src.getHeaderFieldElement())); 540 if (src.hasMinimumId()) tgt.setMinimumIdElement(String30_50.convertString(src.getMinimumIdElement())); 541 if (src.hasNavigationLinks()) 542 tgt.setNavigationLinksElement(Boolean30_50.convertBoolean(src.getNavigationLinksElement())); 543 if (src.hasOperator()) tgt.setOperatorElement(convertAssertionOperatorType(src.getOperatorElement())); 544 if (src.hasPath()) tgt.setPathElement(String30_50.convertString(src.getPathElement())); 545 if (src.hasRequestMethod()) 546 tgt.setRequestMethodElement(convertTestScriptRequestMethodCode(src.getRequestMethodElement())); 547 if (src.hasRequestURL()) tgt.setRequestURLElement(String30_50.convertString(src.getRequestURLElement())); 548 if (src.hasResource()) 549 tgt.setResource(src.getResource()); 550 if (src.hasResponse()) tgt.setResponseElement(convertAssertionResponseTypes(src.getResponseElement())); 551 if (src.hasResponseCode()) tgt.setResponseCodeElement(String30_50.convertString(src.getResponseCodeElement())); 552 if (src.hasSourceId()) tgt.setSourceIdElement(Id30_50.convertId(src.getSourceIdElement())); 553 if (src.hasValidateProfileId()) 554 tgt.setValidateProfileIdElement(Id30_50.convertId(src.getValidateProfileIdElement())); 555 if (src.hasValue()) tgt.setValueElement(String30_50.convertString(src.getValueElement())); 556 if (src.hasWarningOnly()) tgt.setWarningOnlyElement(Boolean30_50.convertBoolean(src.getWarningOnlyElement())); 557 return tgt; 558 } 559 560 public static org.hl7.fhir.dstu3.model.TestScript.SetupActionAssertComponent convertSetupActionAssertComponent(org.hl7.fhir.r5.model.TestScript.SetupActionAssertComponent src) throws FHIRException { 561 if (src == null) return null; 562 org.hl7.fhir.dstu3.model.TestScript.SetupActionAssertComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.SetupActionAssertComponent(); 563 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 564 if (src.hasLabel()) tgt.setLabelElement(String30_50.convertString(src.getLabelElement())); 565 if (src.hasDescription()) tgt.setDescriptionElement(String30_50.convertString(src.getDescriptionElement())); 566 if (src.hasDirection()) tgt.setDirectionElement(convertAssertionDirectionType(src.getDirectionElement())); 567 if (src.hasCompareToSourceId()) 568 tgt.setCompareToSourceIdElement(String30_50.convertString(src.getCompareToSourceIdElement())); 569 if (src.hasCompareToSourceExpression()) 570 tgt.setCompareToSourceExpressionElement(String30_50.convertString(src.getCompareToSourceExpressionElement())); 571 if (src.hasCompareToSourcePath()) 572 tgt.setCompareToSourcePathElement(String30_50.convertString(src.getCompareToSourcePathElement())); 573 if (src.hasContentType()) tgt.setContentType(convertContentType(src.getContentType())); 574 if (src.hasExpression()) tgt.setExpressionElement(String30_50.convertString(src.getExpressionElement())); 575 if (src.hasHeaderField()) tgt.setHeaderFieldElement(String30_50.convertString(src.getHeaderFieldElement())); 576 if (src.hasMinimumId()) tgt.setMinimumIdElement(String30_50.convertString(src.getMinimumIdElement())); 577 if (src.hasNavigationLinks()) 578 tgt.setNavigationLinksElement(Boolean30_50.convertBoolean(src.getNavigationLinksElement())); 579 if (src.hasOperator()) tgt.setOperatorElement(convertAssertionOperatorType(src.getOperatorElement())); 580 if (src.hasPath()) tgt.setPathElement(String30_50.convertString(src.getPathElement())); 581 if (src.hasRequestMethod()) 582 tgt.setRequestMethodElement(convertTestScriptRequestMethodCode(src.getRequestMethodElement())); 583 if (src.hasRequestURL()) tgt.setRequestURLElement(String30_50.convertString(src.getRequestURLElement())); 584 if (src.hasResource()) tgt.setResource(src.getResource()); 585 if (src.hasResponse()) tgt.setResponseElement(convertAssertionResponseTypes(src.getResponseElement())); 586 if (src.hasResponseCode()) tgt.setResponseCodeElement(String30_50.convertString(src.getResponseCodeElement())); 587 if (src.hasSourceId()) tgt.setSourceIdElement(Id30_50.convertId(src.getSourceIdElement())); 588 if (src.hasValidateProfileId()) 589 tgt.setValidateProfileIdElement(Id30_50.convertId(src.getValidateProfileIdElement())); 590 if (src.hasValue()) tgt.setValueElement(String30_50.convertString(src.getValueElement())); 591 if (src.hasWarningOnly()) tgt.setWarningOnlyElement(Boolean30_50.convertBoolean(src.getWarningOnlyElement())); 592 return tgt; 593 } 594 595 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.TestScript.AssertionDirectionType> convertAssertionDirectionType(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestScript.AssertionDirectionType> src) throws FHIRException { 596 if (src == null || src.isEmpty()) return null; 597 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.TestScript.AssertionDirectionType> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.TestScript.AssertionDirectionTypeEnumFactory()); 598 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 599 if (src.getValue() == null) { 600 tgt.setValue(null); 601} else { 602 switch(src.getValue()) { 603 case RESPONSE: 604 tgt.setValue(TestScript.AssertionDirectionType.RESPONSE); 605 break; 606 case REQUEST: 607 tgt.setValue(TestScript.AssertionDirectionType.REQUEST); 608 break; 609 default: 610 tgt.setValue(TestScript.AssertionDirectionType.NULL); 611 break; 612 } 613} 614 return tgt; 615 } 616 617 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestScript.AssertionDirectionType> convertAssertionDirectionType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.TestScript.AssertionDirectionType> src) throws FHIRException { 618 if (src == null || src.isEmpty()) return null; 619 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestScript.AssertionDirectionType> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.TestScript.AssertionDirectionTypeEnumFactory()); 620 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 621 if (src.getValue() == null) { 622 tgt.setValue(null); 623} else { 624 switch(src.getValue()) { 625 case RESPONSE: 626 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionDirectionType.RESPONSE); 627 break; 628 case REQUEST: 629 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionDirectionType.REQUEST); 630 break; 631 default: 632 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionDirectionType.NULL); 633 break; 634 } 635} 636 return tgt; 637 } 638 639 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.TestScript.AssertionOperatorType> convertAssertionOperatorType(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorType> src) throws FHIRException { 640 if (src == null || src.isEmpty()) return null; 641 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.TestScript.AssertionOperatorType> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.TestScript.AssertionOperatorTypeEnumFactory()); 642 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 643 if (src.getValue() == null) { 644 tgt.setValue(null); 645} else { 646 switch(src.getValue()) { 647 case EQUALS: 648 tgt.setValue(TestScript.AssertionOperatorType.EQUALS); 649 break; 650 case NOTEQUALS: 651 tgt.setValue(TestScript.AssertionOperatorType.NOTEQUALS); 652 break; 653 case IN: 654 tgt.setValue(TestScript.AssertionOperatorType.IN); 655 break; 656 case NOTIN: 657 tgt.setValue(TestScript.AssertionOperatorType.NOTIN); 658 break; 659 case GREATERTHAN: 660 tgt.setValue(TestScript.AssertionOperatorType.GREATERTHAN); 661 break; 662 case LESSTHAN: 663 tgt.setValue(TestScript.AssertionOperatorType.LESSTHAN); 664 break; 665 case EMPTY: 666 tgt.setValue(TestScript.AssertionOperatorType.EMPTY); 667 break; 668 case NOTEMPTY: 669 tgt.setValue(TestScript.AssertionOperatorType.NOTEMPTY); 670 break; 671 case CONTAINS: 672 tgt.setValue(TestScript.AssertionOperatorType.CONTAINS); 673 break; 674 case NOTCONTAINS: 675 tgt.setValue(TestScript.AssertionOperatorType.NOTCONTAINS); 676 break; 677 case EVAL: 678 tgt.setValue(TestScript.AssertionOperatorType.EVAL); 679 break; 680 default: 681 tgt.setValue(TestScript.AssertionOperatorType.NULL); 682 break; 683 } 684} 685 return tgt; 686 } 687 688 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorType> convertAssertionOperatorType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.TestScript.AssertionOperatorType> src) throws FHIRException { 689 if (src == null || src.isEmpty()) return null; 690 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorType> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorTypeEnumFactory()); 691 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 692 if (src.getValue() == null) { 693 tgt.setValue(null); 694} else { 695 switch(src.getValue()) { 696 case EQUALS: 697 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorType.EQUALS); 698 break; 699 case NOTEQUALS: 700 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorType.NOTEQUALS); 701 break; 702 case IN: 703 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorType.IN); 704 break; 705 case NOTIN: 706 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorType.NOTIN); 707 break; 708 case GREATERTHAN: 709 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorType.GREATERTHAN); 710 break; 711 case LESSTHAN: 712 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorType.LESSTHAN); 713 break; 714 case EMPTY: 715 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorType.EMPTY); 716 break; 717 case NOTEMPTY: 718 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorType.NOTEMPTY); 719 break; 720 case CONTAINS: 721 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorType.CONTAINS); 722 break; 723 case NOTCONTAINS: 724 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorType.NOTCONTAINS); 725 break; 726 case EVAL: 727 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorType.EVAL); 728 break; 729 default: 730 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorType.NULL); 731 break; 732 } 733} 734 return tgt; 735 } 736 737 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.TestScript.TestScriptRequestMethodCode> convertTestScriptRequestMethodCode(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestScript.TestScriptRequestMethodCode> src) throws FHIRException { 738 if (src == null || src.isEmpty()) return null; 739 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.TestScript.TestScriptRequestMethodCode> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.TestScript.TestScriptRequestMethodCodeEnumFactory()); 740 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 741 if (src.getValue() == null) { 742 tgt.setValue(null); 743} else { 744 switch(src.getValue()) { 745 case DELETE: 746 tgt.setValue(TestScript.TestScriptRequestMethodCode.DELETE); 747 break; 748 case GET: 749 tgt.setValue(TestScript.TestScriptRequestMethodCode.GET); 750 break; 751 case OPTIONS: 752 tgt.setValue(TestScript.TestScriptRequestMethodCode.OPTIONS); 753 break; 754 case PATCH: 755 tgt.setValue(TestScript.TestScriptRequestMethodCode.PATCH); 756 break; 757 case POST: 758 tgt.setValue(TestScript.TestScriptRequestMethodCode.POST); 759 break; 760 case PUT: 761 tgt.setValue(TestScript.TestScriptRequestMethodCode.PUT); 762 break; 763 default: 764 tgt.setValue(TestScript.TestScriptRequestMethodCode.NULL); 765 break; 766 } 767} 768 return tgt; 769 } 770 771 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestScript.TestScriptRequestMethodCode> convertTestScriptRequestMethodCode(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.TestScript.TestScriptRequestMethodCode> src) throws FHIRException { 772 if (src == null || src.isEmpty()) return null; 773 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestScript.TestScriptRequestMethodCode> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.TestScript.TestScriptRequestMethodCodeEnumFactory()); 774 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 775 if (src.getValue() == null) { 776 tgt.setValue(null); 777} else { 778 switch(src.getValue()) { 779 case DELETE: 780 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.TestScriptRequestMethodCode.DELETE); 781 break; 782 case GET: 783 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.TestScriptRequestMethodCode.GET); 784 break; 785 case OPTIONS: 786 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.TestScriptRequestMethodCode.OPTIONS); 787 break; 788 case PATCH: 789 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.TestScriptRequestMethodCode.PATCH); 790 break; 791 case POST: 792 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.TestScriptRequestMethodCode.POST); 793 break; 794 case PUT: 795 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.TestScriptRequestMethodCode.PUT); 796 break; 797 default: 798 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.TestScriptRequestMethodCode.NULL); 799 break; 800 } 801} 802 return tgt; 803 } 804 805 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.TestScript.AssertionResponseTypes> convertAssertionResponseTypes(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes> src) throws FHIRException { 806 if (src == null || src.isEmpty()) return null; 807 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.TestScript.AssertionResponseTypes> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.TestScript.AssertionResponseTypesEnumFactory()); 808 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 809 if (src.getValue() == null) { 810 tgt.setValue(null); 811} else { 812 switch(src.getValue()) { 813 case OKAY: 814 tgt.setValue(TestScript.AssertionResponseTypes.OKAY); 815 break; 816 case CREATED: 817 tgt.setValue(TestScript.AssertionResponseTypes.CREATED); 818 break; 819 case NOCONTENT: 820 tgt.setValue(TestScript.AssertionResponseTypes.NOCONTENT); 821 break; 822 case NOTMODIFIED: 823 tgt.setValue(TestScript.AssertionResponseTypes.NOTMODIFIED); 824 break; 825 case BAD: 826 tgt.setValue(TestScript.AssertionResponseTypes.BADREQUEST); 827 break; 828 case FORBIDDEN: 829 tgt.setValue(TestScript.AssertionResponseTypes.FORBIDDEN); 830 break; 831 case NOTFOUND: 832 tgt.setValue(TestScript.AssertionResponseTypes.NOTFOUND); 833 break; 834 case METHODNOTALLOWED: 835 tgt.setValue(TestScript.AssertionResponseTypes.METHODNOTALLOWED); 836 break; 837 case CONFLICT: 838 tgt.setValue(TestScript.AssertionResponseTypes.CONFLICT); 839 break; 840 case GONE: 841 tgt.setValue(TestScript.AssertionResponseTypes.GONE); 842 break; 843 case PRECONDITIONFAILED: 844 tgt.setValue(TestScript.AssertionResponseTypes.PRECONDITIONFAILED); 845 break; 846 case UNPROCESSABLE: 847 tgt.setValue(TestScript.AssertionResponseTypes.UNPROCESSABLECONTENT); 848 break; 849 default: 850 tgt.setValue(TestScript.AssertionResponseTypes.NULL); 851 break; 852 } 853} 854 return tgt; 855 } 856 857 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes> convertAssertionResponseTypes(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.TestScript.AssertionResponseTypes> src) throws FHIRException { 858 if (src == null || src.isEmpty()) return null; 859 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypesEnumFactory()); 860 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyElement(src, tgt); 861 if (src.getValue() == null) { 862 tgt.setValue(null); 863} else { 864 switch(src.getValue()) { 865 case OKAY: 866 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes.OKAY); 867 break; 868 case CREATED: 869 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes.CREATED); 870 break; 871 case NOCONTENT: 872 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes.NOCONTENT); 873 break; 874 case NOTMODIFIED: 875 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes.NOTMODIFIED); 876 break; 877 case BADREQUEST: 878 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes.BAD); 879 break; 880 case FORBIDDEN: 881 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes.FORBIDDEN); 882 break; 883 case NOTFOUND: 884 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes.NOTFOUND); 885 break; 886 case METHODNOTALLOWED: 887 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes.METHODNOTALLOWED); 888 break; 889 case CONFLICT: 890 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes.CONFLICT); 891 break; 892 case GONE: 893 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes.GONE); 894 break; 895 case PRECONDITIONFAILED: 896 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes.PRECONDITIONFAILED); 897 break; 898 case UNPROCESSABLECONTENT: 899 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes.UNPROCESSABLE); 900 break; 901 default: 902 tgt.setValue(org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes.NULL); 903 break; 904 } 905} 906 return tgt; 907 } 908 909 public static org.hl7.fhir.r5.model.TestScript.TestActionComponent convertTestActionComponent(org.hl7.fhir.dstu3.model.TestScript.TestActionComponent src) throws FHIRException { 910 if (src == null) return null; 911 org.hl7.fhir.r5.model.TestScript.TestActionComponent tgt = new org.hl7.fhir.r5.model.TestScript.TestActionComponent(); 912 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 913 if (src.hasOperation()) tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 914 if (src.hasAssert()) tgt.setAssert(convertSetupActionAssertComponent(src.getAssert())); 915 return tgt; 916 } 917 918 public static org.hl7.fhir.dstu3.model.TestScript.TestActionComponent convertTestActionComponent(org.hl7.fhir.r5.model.TestScript.TestActionComponent src) throws FHIRException { 919 if (src == null) return null; 920 org.hl7.fhir.dstu3.model.TestScript.TestActionComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TestActionComponent(); 921 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 922 if (src.hasOperation()) tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 923 if (src.hasAssert()) tgt.setAssert(convertSetupActionAssertComponent(src.getAssert())); 924 return tgt; 925 } 926 927 public static org.hl7.fhir.r5.model.TestScript.TeardownActionComponent convertTeardownActionComponent(org.hl7.fhir.dstu3.model.TestScript.TeardownActionComponent src) throws FHIRException { 928 if (src == null) return null; 929 org.hl7.fhir.r5.model.TestScript.TeardownActionComponent tgt = new org.hl7.fhir.r5.model.TestScript.TeardownActionComponent(); 930 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 931 if (src.hasOperation()) tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 932 return tgt; 933 } 934 935 public static org.hl7.fhir.dstu3.model.TestScript.TeardownActionComponent convertTeardownActionComponent(org.hl7.fhir.r5.model.TestScript.TeardownActionComponent src) throws FHIRException { 936 if (src == null) return null; 937 org.hl7.fhir.dstu3.model.TestScript.TeardownActionComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TeardownActionComponent(); 938 ConversionContext30_50.INSTANCE.getVersionConvertor_30_50().copyBackboneElement(src,tgt); 939 if (src.hasOperation()) tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 940 return tgt; 941 } 942}