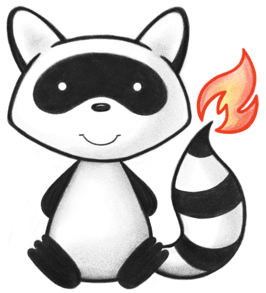
001package org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext40_50; 004import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Boolean40_50; 005import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Code40_50; 006import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.String40_50; 007import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Uri40_50; 008import org.hl7.fhir.exceptions.FHIRException; 009 010public class Coding40_50 { 011 public static org.hl7.fhir.r5.model.Coding convertCoding(org.hl7.fhir.r4.model.Coding src) throws FHIRException { 012 if (src == null) return null; 013 org.hl7.fhir.r5.model.Coding tgt = new org.hl7.fhir.r5.model.Coding(); 014 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 015 if (src.hasSystem()) tgt.setSystemElement(Uri40_50.convertUri(src.getSystemElement())); 016 if (src.hasVersion()) tgt.setVersionElement(String40_50.convertString(src.getVersionElement())); 017 if (src.hasCode()) tgt.setCodeElement(Code40_50.convertCode(src.getCodeElement())); 018 if (src.hasDisplay()) tgt.setDisplayElement(String40_50.convertString(src.getDisplayElement())); 019 if (src.hasUserSelected()) tgt.setUserSelectedElement(Boolean40_50.convertBoolean(src.getUserSelectedElement())); 020 return tgt; 021 } 022 023 public static org.hl7.fhir.r4.model.Coding convertCoding(org.hl7.fhir.r5.model.Coding src) throws FHIRException { 024 if (src == null) return null; 025 org.hl7.fhir.r4.model.Coding tgt = new org.hl7.fhir.r4.model.Coding(); 026 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 027 if (src.hasSystem()) tgt.setSystemElement(Uri40_50.convertUri(src.getSystemElement())); 028 if (src.hasVersion()) tgt.setVersionElement(String40_50.convertString(src.getVersionElement())); 029 if (src.hasCode()) tgt.setCodeElement(Code40_50.convertCode(src.getCodeElement())); 030 if (src.hasDisplay()) tgt.setDisplayElement(String40_50.convertString(src.getDisplayElement())); 031 if (src.hasUserSelected()) tgt.setUserSelectedElement(Boolean40_50.convertBoolean(src.getUserSelectedElement())); 032 return tgt; 033 } 034 035 public static org.hl7.fhir.r5.model.CodeableConcept convertCodingToCodeableConcept(org.hl7.fhir.r4.model.Coding src) throws FHIRException { 036 if (src == null) return null; 037 org.hl7.fhir.r5.model.CodeableConcept tgt = new org.hl7.fhir.r5.model.CodeableConcept(); 038 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 039 if (src.hasSystem()) tgt.getCodingFirstRep().setSystem(src.getSystem()); 040 if (src.hasVersion()) tgt.getCodingFirstRep().setVersion(src.getVersion()); 041 if (src.hasCode()) tgt.getCodingFirstRep().setCode(src.getCode()); 042 if (src.hasDisplay()) tgt.getCodingFirstRep().setDisplay(src.getDisplay()); 043 if (src.hasUserSelected()) tgt.getCodingFirstRep().setUserSelected(src.getUserSelected()); 044 return tgt; 045 } 046 047 public static org.hl7.fhir.r5.model.Coding convertCoding(org.hl7.fhir.r4.model.CodeableConcept src) throws FHIRException { 048 if (src == null) return null; 049 org.hl7.fhir.r5.model.Coding tgt = new org.hl7.fhir.r5.model.Coding(); 050 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 051 if (src.hasCoding()) { 052 if (src.getCodingFirstRep().hasSystem()) tgt.setSystem(src.getCodingFirstRep().getSystem()); 053 if (src.getCodingFirstRep().hasVersion()) tgt.setVersion(src.getCodingFirstRep().getVersion()); 054 if (src.getCodingFirstRep().hasCode()) tgt.setCode(src.getCodingFirstRep().getCode()); 055 if (src.getCodingFirstRep().hasDisplay()) tgt.setDisplay(src.getCodingFirstRep().getDisplay()); 056 if (src.getCodingFirstRep().hasUserSelected()) tgt.setUserSelected(src.getCodingFirstRep().getUserSelected()); 057 } 058 return tgt; 059 } 060 061 062 public static org.hl7.fhir.r4.model.Coding convertCoding(org.hl7.fhir.r5.model.CodeableConcept src) throws FHIRException { 063 if (src == null) return null; 064 org.hl7.fhir.r4.model.Coding tgt = new org.hl7.fhir.r4.model.Coding(); 065 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 066 if (src.hasCoding()) { 067 if (src.getCodingFirstRep().hasSystem()) tgt.setSystem(src.getCodingFirstRep().getSystem()); 068 if (src.getCodingFirstRep().hasVersion()) tgt.setVersion(src.getCodingFirstRep().getVersion()); 069 if (src.getCodingFirstRep().hasCode()) tgt.setCode(src.getCodingFirstRep().getCode()); 070 if (src.getCodingFirstRep().hasDisplay()) tgt.setDisplay(src.getCodingFirstRep().getDisplay()); 071 if (src.getCodingFirstRep().hasUserSelected()) tgt.setUserSelected(src.getCodingFirstRep().getUserSelected()); 072 } 073 return tgt; 074 } 075 076 077}