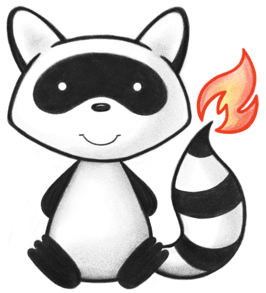
001package org.hl7.fhir.convertors.conv40_50.datatypes40_50.metadata40_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext40_50; 004import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Attachment40_50; 005import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Canonical40_50; 006import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.MarkDown40_50; 007import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.String40_50; 008import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Url40_50; 009import org.hl7.fhir.exceptions.FHIRException; 010import org.hl7.fhir.r5.model.RelatedArtifact; 011 012public class RelatedArtifact40_50 { 013 public static org.hl7.fhir.r5.model.RelatedArtifact convertRelatedArtifact(org.hl7.fhir.r4.model.RelatedArtifact src) throws FHIRException { 014 if (src == null) return null; 015 org.hl7.fhir.r5.model.RelatedArtifact tgt = new org.hl7.fhir.r5.model.RelatedArtifact(); 016 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 017 if (src.hasType()) tgt.setTypeElement(convertRelatedArtifactType(src.getTypeElement())); 018 if (src.hasLabel()) tgt.setLabelElement(String40_50.convertString(src.getLabelElement())); 019 if (src.hasDisplay()) tgt.setDisplayElement(String40_50.convertString(src.getDisplayElement())); 020 if (src.hasCitation()) tgt.setCitationElement(MarkDown40_50.convertMarkdown(src.getCitationElement())); 021 if (src.hasUrl()) tgt.getDocument().setUrlElement(Url40_50.convertUrl(src.getUrlElement())); 022 if (src.hasDocument()) tgt.setDocument(Attachment40_50.convertAttachment(src.getDocument())); 023 if (src.hasResource()) tgt.setResourceElement(Canonical40_50.convertCanonical(src.getResourceElement())); 024 return tgt; 025 } 026 027 public static org.hl7.fhir.r4.model.RelatedArtifact convertRelatedArtifact(org.hl7.fhir.r5.model.RelatedArtifact src) throws FHIRException { 028 if (src == null) return null; 029 org.hl7.fhir.r4.model.RelatedArtifact tgt = new org.hl7.fhir.r4.model.RelatedArtifact(); 030 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 031 if (src.hasType()) tgt.setTypeElement(convertRelatedArtifactType(src.getTypeElement())); 032 if (src.hasLabel()) tgt.setLabelElement(String40_50.convertString(src.getLabelElement())); 033 if (src.hasDisplay()) tgt.setDisplayElement(String40_50.convertString(src.getDisplayElement())); 034 if (src.hasCitation()) tgt.setCitationElement(MarkDown40_50.convertMarkdown(src.getCitationElement())); 035 if (src.getDocument().hasUrl()) tgt.setUrlElement(Url40_50.convertUrl(src.getDocument().getUrlElement())); 036 if (src.hasDocument()) tgt.setDocument(Attachment40_50.convertAttachment(src.getDocument())); 037 if (src.hasResource()) tgt.setResourceElement(Canonical40_50.convertCanonical(src.getResourceElement())); 038 return tgt; 039 } 040 041 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.RelatedArtifact.RelatedArtifactType> convertRelatedArtifactType(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.RelatedArtifact.RelatedArtifactType> src) throws FHIRException { 042 if (src == null || src.isEmpty()) return null; 043 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.RelatedArtifact.RelatedArtifactType> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.RelatedArtifact.RelatedArtifactTypeEnumFactory()); 044 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 045 if (src.getValue() == null) { 046 tgt.setValue(null); 047} else { 048 switch(src.getValue()) { 049 case DOCUMENTATION: 050 tgt.setValue(RelatedArtifact.RelatedArtifactType.DOCUMENTATION); 051 break; 052 case JUSTIFICATION: 053 tgt.setValue(RelatedArtifact.RelatedArtifactType.JUSTIFICATION); 054 break; 055 case CITATION: 056 tgt.setValue(RelatedArtifact.RelatedArtifactType.CITATION); 057 break; 058 case PREDECESSOR: 059 tgt.setValue(RelatedArtifact.RelatedArtifactType.PREDECESSOR); 060 break; 061 case SUCCESSOR: 062 tgt.setValue(RelatedArtifact.RelatedArtifactType.SUCCESSOR); 063 break; 064 case DERIVEDFROM: 065 tgt.setValue(RelatedArtifact.RelatedArtifactType.DERIVEDFROM); 066 break; 067 case DEPENDSON: 068 tgt.setValue(RelatedArtifact.RelatedArtifactType.DEPENDSON); 069 break; 070 case COMPOSEDOF: 071 tgt.setValue(RelatedArtifact.RelatedArtifactType.COMPOSEDOF); 072 break; 073 default: 074 tgt.setValue(RelatedArtifact.RelatedArtifactType.NULL); 075 break; 076 } 077} 078 return tgt; 079 } 080 081 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.RelatedArtifact.RelatedArtifactType> convertRelatedArtifactType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.RelatedArtifact.RelatedArtifactType> src) throws FHIRException { 082 if (src == null || src.isEmpty()) return null; 083 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.RelatedArtifact.RelatedArtifactType> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.RelatedArtifact.RelatedArtifactTypeEnumFactory()); 084 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 085 if (src.getValue() == null) { 086 tgt.setValue(null); 087} else { 088 switch(src.getValue()) { 089 case DOCUMENTATION: 090 tgt.setValue(org.hl7.fhir.r4.model.RelatedArtifact.RelatedArtifactType.DOCUMENTATION); 091 break; 092 case JUSTIFICATION: 093 tgt.setValue(org.hl7.fhir.r4.model.RelatedArtifact.RelatedArtifactType.JUSTIFICATION); 094 break; 095 case CITATION: 096 tgt.setValue(org.hl7.fhir.r4.model.RelatedArtifact.RelatedArtifactType.CITATION); 097 break; 098 case PREDECESSOR: 099 tgt.setValue(org.hl7.fhir.r4.model.RelatedArtifact.RelatedArtifactType.PREDECESSOR); 100 break; 101 case SUCCESSOR: 102 tgt.setValue(org.hl7.fhir.r4.model.RelatedArtifact.RelatedArtifactType.SUCCESSOR); 103 break; 104 case DERIVEDFROM: 105 tgt.setValue(org.hl7.fhir.r4.model.RelatedArtifact.RelatedArtifactType.DERIVEDFROM); 106 break; 107 case DEPENDSON: 108 tgt.setValue(org.hl7.fhir.r4.model.RelatedArtifact.RelatedArtifactType.DEPENDSON); 109 break; 110 case COMPOSEDOF: 111 tgt.setValue(org.hl7.fhir.r4.model.RelatedArtifact.RelatedArtifactType.COMPOSEDOF); 112 break; 113 default: 114 tgt.setValue(org.hl7.fhir.r4.model.RelatedArtifact.RelatedArtifactType.NULL); 115 break; 116 } 117} 118 return tgt; 119 } 120}