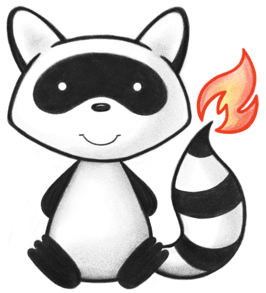
001package org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext40_50; 004import org.hl7.fhir.exceptions.FHIRException; 005 006public class Canonical40_50 { 007 public static org.hl7.fhir.r5.model.CanonicalType convertCanonical(org.hl7.fhir.r4.model.CanonicalType src) throws FHIRException { 008 org.hl7.fhir.r5.model.CanonicalType tgt = src.hasValue() ? new org.hl7.fhir.r5.model.CanonicalType(src.getValueAsString()) : new org.hl7.fhir.r5.model.CanonicalType(); 009 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 010 return tgt; 011 } 012 013 public static org.hl7.fhir.r4.model.CanonicalType convertCanonical(org.hl7.fhir.r5.model.CanonicalType src) throws FHIRException { 014 org.hl7.fhir.r4.model.CanonicalType tgt = src.hasValue() ? new org.hl7.fhir.r4.model.CanonicalType(src.getValueAsString()) : new org.hl7.fhir.r4.model.CanonicalType(); 015 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 016 return tgt; 017 } 018 019 public static org.hl7.fhir.r5.model.UriType convertCanonicalToUri(org.hl7.fhir.r4.model.CanonicalType src) throws FHIRException { 020 org.hl7.fhir.r5.model.UriType tgt = src.hasValue() ? new org.hl7.fhir.r5.model.UriType(src.getValueAsString()) : new org.hl7.fhir.r5.model.UriType(); 021 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 022 return tgt; 023 } 024 025 public static org.hl7.fhir.r4.model.UriType convertCanonicalToUri(org.hl7.fhir.r5.model.CanonicalType src) throws FHIRException { 026 org.hl7.fhir.r4.model.UriType tgt = src.hasValue() ? new org.hl7.fhir.r4.model.UriType(src.getValueAsString()) : new org.hl7.fhir.r4.model.UriType(); 027 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 028 return tgt; 029 } 030 031 032 033 public static org.hl7.fhir.r5.model.CanonicalType convertUriToCanonical(org.hl7.fhir.r4.model.UriType src) throws FHIRException { 034 org.hl7.fhir.r5.model.CanonicalType tgt = src.hasValue() ? new org.hl7.fhir.r5.model.CanonicalType(src.getValueAsString()) : new org.hl7.fhir.r5.model.CanonicalType(); 035 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 036 return tgt; 037 } 038 039 public static org.hl7.fhir.r4.model.CanonicalType convertUriToCanonical(org.hl7.fhir.r5.model.UriType src) throws FHIRException { 040 org.hl7.fhir.r4.model.CanonicalType tgt = src.hasValue() ? new org.hl7.fhir.r4.model.CanonicalType(src.getValueAsString()) : new org.hl7.fhir.r4.model.CanonicalType(); 041 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 042 return tgt; 043 } 044 045}