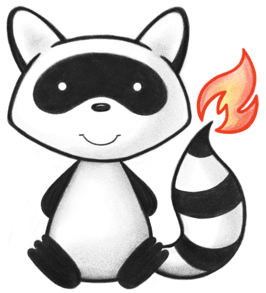
001package org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext40_50; 004import org.hl7.fhir.exceptions.FHIRException; 005 006public class Id40_50 { 007 public static org.hl7.fhir.r5.model.IdType convertId(org.hl7.fhir.r4.model.IdType src) throws FHIRException { 008 org.hl7.fhir.r5.model.IdType tgt = src.hasValue() ? new org.hl7.fhir.r5.model.IdType(src.getValueAsString()) : new org.hl7.fhir.r5.model.IdType(); 009 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 010 return tgt; 011 } 012 013 public static org.hl7.fhir.r4.model.IdType convertId(org.hl7.fhir.r5.model.IdType src) throws FHIRException { 014 org.hl7.fhir.r4.model.IdType tgt = src.hasValue() ? new org.hl7.fhir.r4.model.IdType(src.getValueAsString()) : new org.hl7.fhir.r4.model.IdType(); 015 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 016 return tgt; 017 } 018 019 020 public static org.hl7.fhir.r5.model.StringType convertIdToString(org.hl7.fhir.r4.model.IdType src) { 021 org.hl7.fhir.r5.model.StringType tgt = src.hasValue() ? new org.hl7.fhir.r5.model.StringType(src.getValueAsString()) : new org.hl7.fhir.r5.model.StringType(); 022 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 023 return tgt; 024 } 025 026 027 public static org.hl7.fhir.r4.model.IdType convertId(org.hl7.fhir.r5.model.StringType src) throws FHIRException { 028 org.hl7.fhir.r4.model.IdType tgt = src.hasValue() ? new org.hl7.fhir.r4.model.IdType(src.getValueAsString()) : new org.hl7.fhir.r4.model.IdType(); 029 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 030 return tgt; 031 } 032 033 034 public static org.hl7.fhir.r5.model.CodeType convertIdToCode(org.hl7.fhir.r4.model.IdType src) { 035 org.hl7.fhir.r5.model.CodeType tgt = src.hasValue() ? new org.hl7.fhir.r5.model.CodeType(src.getValueAsString()) : new org.hl7.fhir.r5.model.CodeType(); 036 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 037 return tgt; 038 } 039 040 041 public static org.hl7.fhir.r4.model.IdType convertId(org.hl7.fhir.r5.model.CodeType src) throws FHIRException { 042 org.hl7.fhir.r4.model.IdType tgt = src.hasValue() ? new org.hl7.fhir.r4.model.IdType(src.getValueAsString()) : new org.hl7.fhir.r4.model.IdType(); 043 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 044 return tgt; 045 } 046 047}