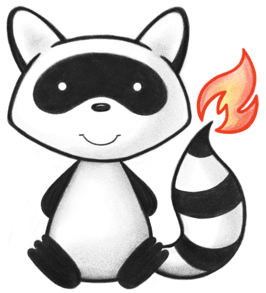
001package org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext40_50; 004import org.hl7.fhir.exceptions.FHIRException; 005 006public class Integer40_50 { 007 public static org.hl7.fhir.r5.model.IntegerType convertInteger(org.hl7.fhir.r4.model.IntegerType src) throws FHIRException { 008 org.hl7.fhir.r5.model.IntegerType tgt = src.hasValue() ? new org.hl7.fhir.r5.model.IntegerType(src.getValueAsString()) : new org.hl7.fhir.r5.model.IntegerType(); 009 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 010 return tgt; 011 } 012 013 public static org.hl7.fhir.r4.model.IntegerType convertInteger(org.hl7.fhir.r5.model.IntegerType src) throws FHIRException { 014 org.hl7.fhir.r4.model.IntegerType tgt = src.hasValue() ? new org.hl7.fhir.r4.model.IntegerType(src.getValueAsString()) : new org.hl7.fhir.r4.model.IntegerType(); 015 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 016 return tgt; 017 } 018 019 public static org.hl7.fhir.r5.model.Integer64Type convertInteger64(org.hl7.fhir.r4.model.IntegerType src) throws FHIRException { 020 org.hl7.fhir.r5.model.Integer64Type tgt = src.hasValue() ? new org.hl7.fhir.r5.model.Integer64Type(src.getValueAsString()) : new org.hl7.fhir.r5.model.Integer64Type(); 021 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 022 return tgt; 023 } 024 025 public static org.hl7.fhir.r4.model.IntegerType convertInteger64(org.hl7.fhir.r5.model.Integer64Type src) throws FHIRException { 026 org.hl7.fhir.r4.model.IntegerType tgt = new org.hl7.fhir.r4.model.IntegerType(); 027 if (src.hasValue()) { 028 try { 029 tgt.setValueAsString(src.getValueAsString()); 030 } catch (Exception e) { 031 // nothing? 032 tgt.setValueAsString("0"); 033 } 034 } 035 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 036 return tgt; 037 } 038 039}