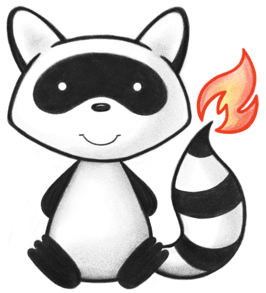
001package org.hl7.fhir.convertors.conv40_50.datatypes40_50.special40_50; 002 003import java.util.stream.Collectors; 004 005import org.hl7.fhir.convertors.VersionConvertorConstants; 006import org.hl7.fhir.convertors.context.ConversionContext40_50; 007import org.hl7.fhir.convertors.conv40_50.datatypes40_50.BackboneElement40_50; 008import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Coding40_50; 009import org.hl7.fhir.convertors.conv40_50.datatypes40_50.metadata40_50.UsageContext40_50; 010import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Boolean40_50; 011import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Canonical40_50; 012import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Code40_50; 013import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Id40_50; 014import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Integer40_50; 015import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.MarkDown40_50; 016import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.String40_50; 017import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.UnsignedInt40_50; 018import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Uri40_50; 019import org.hl7.fhir.convertors.conv40_50.resources40_50.Enumerations40_50; 020import org.hl7.fhir.exceptions.FHIRException; 021import org.hl7.fhir.r4.model.BooleanType; 022import org.hl7.fhir.r4.model.CanonicalType; 023import org.hl7.fhir.r4.model.Extension; 024import org.hl7.fhir.r4.model.MarkdownType; 025import org.hl7.fhir.r4.model.StringType; 026import org.hl7.fhir.r5.model.CodeType; 027import org.hl7.fhir.r5.model.ElementDefinition; 028import org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionBindingAdditionalComponent; 029import org.hl7.fhir.r5.model.UsageContext; 030import org.hl7.fhir.r5.utils.ToolingExtensions; 031 032public class ElementDefinition40_50 { 033 034 public static org.hl7.fhir.r5.model.ElementDefinition convertElementDefinition(org.hl7.fhir.r4.model.ElementDefinition src) throws FHIRException { 035 if (src == null) return null; 036 org.hl7.fhir.r5.model.ElementDefinition tgt = new org.hl7.fhir.r5.model.ElementDefinition(); 037 BackboneElement40_50.copyBackboneElement(src, tgt, 038 VersionConvertorConstants.EXT_MUST_VALUE, 039 VersionConvertorConstants.EXT_VALUE_ALT); 040 if (src.hasPath()) tgt.setPathElement(String40_50.convertString(src.getPathElement())); 041 tgt.setRepresentation(src.getRepresentation().stream().map(ElementDefinition40_50::convertPropertyRepresentation).collect(Collectors.toList())); 042 if (src.hasSliceName()) tgt.setSliceNameElement(String40_50.convertString(src.getSliceNameElement())); 043 if (src.hasSliceIsConstraining()) 044 tgt.setSliceIsConstrainingElement(Boolean40_50.convertBoolean(src.getSliceIsConstrainingElement())); 045 if (src.hasLabel()) tgt.setLabelElement(String40_50.convertString(src.getLabelElement())); 046 for (org.hl7.fhir.r4.model.Coding t : src.getCode()) tgt.addCode(Coding40_50.convertCoding(t)); 047 if (src.hasSlicing()) tgt.setSlicing(convertElementDefinitionSlicingComponent(src.getSlicing())); 048 if (src.hasShort()) tgt.setShortElement(String40_50.convertString(src.getShortElement())); 049 if (src.hasDefinition()) tgt.setDefinitionElement(MarkDown40_50.convertMarkdown(src.getDefinitionElement())); 050 if (src.hasComment()) tgt.setCommentElement(MarkDown40_50.convertMarkdown(src.getCommentElement())); 051 if (src.hasRequirements()) tgt.setRequirementsElement(MarkDown40_50.convertMarkdown(src.getRequirementsElement())); 052 for (org.hl7.fhir.r4.model.StringType t : src.getAlias()) tgt.getAlias().add(String40_50.convertString(t)); 053 if (src.hasMin()) tgt.setMinElement(UnsignedInt40_50.convertUnsignedInt(src.getMinElement())); 054 if (src.hasMax()) tgt.setMaxElement(String40_50.convertString(src.getMaxElement())); 055 if (src.hasBase()) tgt.setBase(convertElementDefinitionBaseComponent(src.getBase())); 056 if (src.hasContentReference()) 057 tgt.setContentReferenceElement(Uri40_50.convertUri(src.getContentReferenceElement())); 058 for (org.hl7.fhir.r4.model.ElementDefinition.TypeRefComponent t : src.getType()) 059 tgt.addType(convertTypeRefComponent(t)); 060 if (src.hasDefaultValue()) 061 tgt.setDefaultValue(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getDefaultValue())); 062 if (src.hasMeaningWhenMissing()) 063 tgt.setMeaningWhenMissingElement(MarkDown40_50.convertMarkdown(src.getMeaningWhenMissingElement())); 064 if (src.hasOrderMeaning()) tgt.setOrderMeaningElement(String40_50.convertString(src.getOrderMeaningElement())); 065 if (src.hasFixed()) 066 tgt.setFixed(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getFixed())); 067 if (src.hasPattern()) 068 tgt.setPattern(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getPattern())); 069 for (org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionExampleComponent t : src.getExample()) 070 tgt.addExample(convertElementDefinitionExampleComponent(t)); 071 if (src.hasMinValue()) 072 tgt.setMinValue(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getMinValue())); 073 if (src.hasMaxValue()) 074 tgt.setMaxValue(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getMaxValue())); 075 if (src.hasMaxLength()) tgt.setMaxLengthElement(Integer40_50.convertInteger(src.getMaxLengthElement())); 076 for (org.hl7.fhir.r4.model.IdType t : src.getCondition()) tgt.getCondition().add(Id40_50.convertId(t)); 077 for (org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionConstraintComponent t : src.getConstraint()) 078 tgt.addConstraint(convertElementDefinitionConstraintComponent(t)); 079 if (src.hasMustSupport()) tgt.setMustSupportElement(Boolean40_50.convertBoolean(src.getMustSupportElement())); 080 if (src.hasIsModifier()) tgt.setIsModifierElement(Boolean40_50.convertBoolean(src.getIsModifierElement())); 081 if (src.hasIsModifierReason()) 082 tgt.setIsModifierReasonElement(String40_50.convertString(src.getIsModifierReasonElement())); 083 if (src.hasIsSummary()) tgt.setIsSummaryElement(Boolean40_50.convertBoolean(src.getIsSummaryElement())); 084 if (src.hasBinding()) tgt.setBinding(convertElementDefinitionBindingComponent(src.getBinding())); 085 for (org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionMappingComponent t : src.getMapping()) 086 tgt.addMapping(convertElementDefinitionMappingComponent(t)); 087 088 if (src.hasExtension(VersionConvertorConstants.EXT_MUST_VALUE)) { 089 tgt.setMustHaveValueElement(Boolean40_50.convertBoolean((org.hl7.fhir.r4.model.BooleanType) src.getExtensionByUrl(VersionConvertorConstants.EXT_MUST_VALUE).getValueAsPrimitive())); 090 } 091 for (org.hl7.fhir.r4.model.Extension ext : src.getExtensionsByUrl(VersionConvertorConstants.EXT_VALUE_ALT)) { 092 tgt.addValueAlternative(Canonical40_50.convertCanonical((org.hl7.fhir.r4.model.CanonicalType)ext.getValue())); 093 } 094 095 return tgt; 096 } 097 098 public static org.hl7.fhir.r4.model.ElementDefinition convertElementDefinition(org.hl7.fhir.r5.model.ElementDefinition src) throws FHIRException { 099 if (src == null) return null; 100 org.hl7.fhir.r4.model.ElementDefinition tgt = new org.hl7.fhir.r4.model.ElementDefinition(); 101 BackboneElement40_50.copyBackboneElement(src, tgt); 102 if (src.hasPath()) tgt.setPathElement(String40_50.convertString(src.getPathElement())); 103 tgt.setRepresentation(src.getRepresentation().stream().map(ElementDefinition40_50::convertPropertyRepresentation).collect(Collectors.toList())); 104 if (src.hasSliceName()) tgt.setSliceNameElement(String40_50.convertString(src.getSliceNameElement())); 105 if (src.hasSliceIsConstraining()) 106 tgt.setSliceIsConstrainingElement(Boolean40_50.convertBoolean(src.getSliceIsConstrainingElement())); 107 if (src.hasLabel()) tgt.setLabelElement(String40_50.convertString(src.getLabelElement())); 108 for (org.hl7.fhir.r5.model.Coding t : src.getCode()) tgt.addCode(Coding40_50.convertCoding(t)); 109 if (src.hasSlicing()) tgt.setSlicing(convertElementDefinitionSlicingComponent(src.getSlicing())); 110 if (src.hasShort()) tgt.setShortElement(String40_50.convertString(src.getShortElement())); 111 if (src.hasDefinition()) tgt.setDefinitionElement(MarkDown40_50.convertMarkdown(src.getDefinitionElement())); 112 if (src.hasComment()) tgt.setCommentElement(MarkDown40_50.convertMarkdown(src.getCommentElement())); 113 if (src.hasRequirements()) tgt.setRequirementsElement(MarkDown40_50.convertMarkdown(src.getRequirementsElement())); 114 for (org.hl7.fhir.r5.model.StringType t : src.getAlias()) tgt.getAlias().add(String40_50.convertString(t)); 115 if (src.hasMin()) tgt.setMinElement(UnsignedInt40_50.convertUnsignedInt(src.getMinElement())); 116 if (src.hasMax()) tgt.setMaxElement(String40_50.convertString(src.getMaxElement())); 117 if (src.hasBase()) tgt.setBase(convertElementDefinitionBaseComponent(src.getBase())); 118 if (src.hasContentReference()) 119 tgt.setContentReferenceElement(Uri40_50.convertUri(src.getContentReferenceElement())); 120 for (org.hl7.fhir.r5.model.ElementDefinition.TypeRefComponent t : src.getType()) 121 tgt.addType(convertTypeRefComponent(t)); 122 if (src.hasDefaultValue()) 123 tgt.setDefaultValue(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getDefaultValue())); 124 if (src.hasMeaningWhenMissing()) 125 tgt.setMeaningWhenMissingElement(MarkDown40_50.convertMarkdown(src.getMeaningWhenMissingElement())); 126 if (src.hasOrderMeaning()) tgt.setOrderMeaningElement(String40_50.convertString(src.getOrderMeaningElement())); 127 if (src.hasFixed()) 128 tgt.setFixed(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getFixed())); 129 if (src.hasPattern()) 130 tgt.setPattern(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getPattern())); 131 for (org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionExampleComponent t : src.getExample()) 132 tgt.addExample(convertElementDefinitionExampleComponent(t)); 133 if (src.hasMinValue()) 134 tgt.setMinValue(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getMinValue())); 135 if (src.hasMaxValue()) 136 tgt.setMaxValue(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getMaxValue())); 137 if (src.hasMaxLength()) tgt.setMaxLengthElement(Integer40_50.convertInteger(src.getMaxLengthElement())); 138 for (org.hl7.fhir.r5.model.IdType t : src.getCondition()) tgt.getCondition().add(Id40_50.convertId(t)); 139 for (org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionConstraintComponent t : src.getConstraint()) 140 tgt.addConstraint(convertElementDefinitionConstraintComponent(t)); 141 if (src.hasMustSupport()) tgt.setMustSupportElement(Boolean40_50.convertBoolean(src.getMustSupportElement())); 142 if (src.hasIsModifier()) tgt.setIsModifierElement(Boolean40_50.convertBoolean(src.getIsModifierElement())); 143 if (src.hasIsModifierReason()) 144 tgt.setIsModifierReasonElement(String40_50.convertString(src.getIsModifierReasonElement())); 145 if (src.hasIsSummary()) tgt.setIsSummaryElement(Boolean40_50.convertBoolean(src.getIsSummaryElement())); 146 if (src.hasBinding()) tgt.setBinding(convertElementDefinitionBindingComponent(src.getBinding())); 147 for (org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionMappingComponent t : src.getMapping()) 148 tgt.addMapping(convertElementDefinitionMappingComponent(t)); 149 if (src.hasMustHaveValue()) { 150 tgt.addExtension(VersionConvertorConstants.EXT_MUST_VALUE, Boolean40_50.convertBoolean(src.getMustHaveValueElement())); 151 } 152 for (org.hl7.fhir.r5.model.CanonicalType ct : src.getValueAlternatives()) { 153 tgt.addExtension(VersionConvertorConstants.EXT_VALUE_ALT, Canonical40_50.convertCanonical(ct)); 154 } 155 156 return tgt; 157 } 158 159 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ElementDefinition.PropertyRepresentation> convertPropertyRepresentation(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ElementDefinition.PropertyRepresentation> src) throws FHIRException { 160 if (src == null || src.isEmpty()) return null; 161 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ElementDefinition.PropertyRepresentation> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.ElementDefinition.PropertyRepresentationEnumFactory()); 162 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 163 if (src.getValue() == null) { 164 tgt.setValue(null); 165} else { 166 switch(src.getValue()) { 167 case XMLATTR: 168 tgt.setValue(ElementDefinition.PropertyRepresentation.XMLATTR); 169 break; 170 case XMLTEXT: 171 tgt.setValue(ElementDefinition.PropertyRepresentation.XMLTEXT); 172 break; 173 case TYPEATTR: 174 tgt.setValue(ElementDefinition.PropertyRepresentation.TYPEATTR); 175 break; 176 case CDATEXT: 177 tgt.setValue(ElementDefinition.PropertyRepresentation.CDATEXT); 178 break; 179 case XHTML: 180 tgt.setValue(ElementDefinition.PropertyRepresentation.XHTML); 181 break; 182 default: 183 tgt.setValue(ElementDefinition.PropertyRepresentation.NULL); 184 break; 185 } 186} 187 return tgt; 188 } 189 190 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ElementDefinition.PropertyRepresentation> convertPropertyRepresentation(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ElementDefinition.PropertyRepresentation> src) throws FHIRException { 191 if (src == null || src.isEmpty()) return null; 192 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ElementDefinition.PropertyRepresentation> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.ElementDefinition.PropertyRepresentationEnumFactory()); 193 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 194 if (src.getValue() == null) { 195 tgt.setValue(null); 196} else { 197 switch(src.getValue()) { 198 case XMLATTR: 199 tgt.setValue(org.hl7.fhir.r4.model.ElementDefinition.PropertyRepresentation.XMLATTR); 200 break; 201 case XMLTEXT: 202 tgt.setValue(org.hl7.fhir.r4.model.ElementDefinition.PropertyRepresentation.XMLTEXT); 203 break; 204 case TYPEATTR: 205 tgt.setValue(org.hl7.fhir.r4.model.ElementDefinition.PropertyRepresentation.TYPEATTR); 206 break; 207 case CDATEXT: 208 tgt.setValue(org.hl7.fhir.r4.model.ElementDefinition.PropertyRepresentation.CDATEXT); 209 break; 210 case XHTML: 211 tgt.setValue(org.hl7.fhir.r4.model.ElementDefinition.PropertyRepresentation.XHTML); 212 break; 213 default: 214 tgt.setValue(org.hl7.fhir.r4.model.ElementDefinition.PropertyRepresentation.NULL); 215 break; 216 } 217} 218 return tgt; 219 } 220 221 public static org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionSlicingComponent convertElementDefinitionSlicingComponent(org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionSlicingComponent src) throws FHIRException { 222 if (src == null) return null; 223 org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionSlicingComponent tgt = new org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionSlicingComponent(); 224 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 225 for (org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionSlicingDiscriminatorComponent t : src.getDiscriminator()) 226 tgt.addDiscriminator(convertElementDefinitionSlicingDiscriminatorComponent(t)); 227 if (src.hasDescription()) tgt.setDescriptionElement(String40_50.convertString(src.getDescriptionElement())); 228 if (src.hasOrdered()) tgt.setOrderedElement(Boolean40_50.convertBoolean(src.getOrderedElement())); 229 if (src.hasRules()) tgt.setRulesElement(convertSlicingRules(src.getRulesElement())); 230 return tgt; 231 } 232 233 public static org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionSlicingComponent convertElementDefinitionSlicingComponent(org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionSlicingComponent src) throws FHIRException { 234 if (src == null) return null; 235 org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionSlicingComponent tgt = new org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionSlicingComponent(); 236 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 237 for (org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionSlicingDiscriminatorComponent t : src.getDiscriminator()) 238 tgt.addDiscriminator(convertElementDefinitionSlicingDiscriminatorComponent(t)); 239 if (src.hasDescription()) tgt.setDescriptionElement(String40_50.convertString(src.getDescriptionElement())); 240 if (src.hasOrdered()) tgt.setOrderedElement(Boolean40_50.convertBoolean(src.getOrderedElement())); 241 if (src.hasRules()) tgt.setRulesElement(convertSlicingRules(src.getRulesElement())); 242 return tgt; 243 } 244 245 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ElementDefinition.SlicingRules> convertSlicingRules(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ElementDefinition.SlicingRules> src) throws FHIRException { 246 if (src == null || src.isEmpty()) return null; 247 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ElementDefinition.SlicingRules> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.ElementDefinition.SlicingRulesEnumFactory()); 248 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 249 if (src.getValue() == null) { 250 tgt.setValue(null); 251} else { 252 switch(src.getValue()) { 253 case CLOSED: 254 tgt.setValue(ElementDefinition.SlicingRules.CLOSED); 255 break; 256 case OPEN: 257 tgt.setValue(ElementDefinition.SlicingRules.OPEN); 258 break; 259 case OPENATEND: 260 tgt.setValue(ElementDefinition.SlicingRules.OPENATEND); 261 break; 262 default: 263 tgt.setValue(ElementDefinition.SlicingRules.NULL); 264 break; 265 } 266} 267 return tgt; 268 } 269 270 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ElementDefinition.SlicingRules> convertSlicingRules(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ElementDefinition.SlicingRules> src) throws FHIRException { 271 if (src == null || src.isEmpty()) return null; 272 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ElementDefinition.SlicingRules> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.ElementDefinition.SlicingRulesEnumFactory()); 273 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 274 if (src.getValue() == null) { 275 tgt.setValue(null); 276} else { 277 switch(src.getValue()) { 278 case CLOSED: 279 tgt.setValue(org.hl7.fhir.r4.model.ElementDefinition.SlicingRules.CLOSED); 280 break; 281 case OPEN: 282 tgt.setValue(org.hl7.fhir.r4.model.ElementDefinition.SlicingRules.OPEN); 283 break; 284 case OPENATEND: 285 tgt.setValue(org.hl7.fhir.r4.model.ElementDefinition.SlicingRules.OPENATEND); 286 break; 287 default: 288 tgt.setValue(org.hl7.fhir.r4.model.ElementDefinition.SlicingRules.NULL); 289 break; 290 } 291} 292 return tgt; 293 } 294 295 public static org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionSlicingDiscriminatorComponent convertElementDefinitionSlicingDiscriminatorComponent(org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionSlicingDiscriminatorComponent src) throws FHIRException { 296 if (src == null) return null; 297 org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionSlicingDiscriminatorComponent tgt = new org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionSlicingDiscriminatorComponent(); 298 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 299 if (src.hasType()) tgt.setTypeElement(convertDiscriminatorType(src.getTypeElement())); 300 if (src.hasPath()) tgt.setPathElement(String40_50.convertString(src.getPathElement())); 301 return tgt; 302 } 303 304 public static org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionSlicingDiscriminatorComponent convertElementDefinitionSlicingDiscriminatorComponent(org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionSlicingDiscriminatorComponent src) throws FHIRException { 305 if (src == null) return null; 306 org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionSlicingDiscriminatorComponent tgt = new org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionSlicingDiscriminatorComponent(); 307 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 308 if (src.hasType()) tgt.setTypeElement(convertDiscriminatorType(src.getTypeElement())); 309 if (src.hasPath()) tgt.setPathElement(String40_50.convertString(src.getPathElement())); 310 return tgt; 311 } 312 313 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ElementDefinition.DiscriminatorType> convertDiscriminatorType(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ElementDefinition.DiscriminatorType> src) throws FHIRException { 314 if (src == null || src.isEmpty()) return null; 315 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ElementDefinition.DiscriminatorType> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.ElementDefinition.DiscriminatorTypeEnumFactory()); 316 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 317 if (src.getValue() == null) { 318 tgt.setValue(null); 319} else { 320 switch(src.getValue()) { 321 case VALUE: 322 tgt.setValue(ElementDefinition.DiscriminatorType.VALUE); 323 break; 324 case EXISTS: 325 tgt.setValue(ElementDefinition.DiscriminatorType.EXISTS); 326 break; 327 case PATTERN: 328 tgt.setValue(ElementDefinition.DiscriminatorType.PATTERN); 329 break; 330 case TYPE: 331 tgt.setValue(ElementDefinition.DiscriminatorType.TYPE); 332 break; 333 case PROFILE: 334 tgt.setValue(ElementDefinition.DiscriminatorType.PROFILE); 335 break; 336 default: 337 tgt.setValue(ElementDefinition.DiscriminatorType.NULL); 338 break; 339 } 340} 341 return tgt; 342 } 343 344 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ElementDefinition.DiscriminatorType> convertDiscriminatorType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ElementDefinition.DiscriminatorType> src) throws FHIRException { 345 if (src == null || src.isEmpty()) return null; 346 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ElementDefinition.DiscriminatorType> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.ElementDefinition.DiscriminatorTypeEnumFactory()); 347 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 348 if (src.getValue() == null) { 349 tgt.setValue(null); 350} else { 351 switch(src.getValue()) { 352 case VALUE: 353 tgt.setValue(org.hl7.fhir.r4.model.ElementDefinition.DiscriminatorType.VALUE); 354 break; 355 case EXISTS: 356 tgt.setValue(org.hl7.fhir.r4.model.ElementDefinition.DiscriminatorType.EXISTS); 357 break; 358 case PATTERN: 359 tgt.setValue(org.hl7.fhir.r4.model.ElementDefinition.DiscriminatorType.PATTERN); 360 break; 361 case TYPE: 362 tgt.setValue(org.hl7.fhir.r4.model.ElementDefinition.DiscriminatorType.TYPE); 363 break; 364 case PROFILE: 365 tgt.setValue(org.hl7.fhir.r4.model.ElementDefinition.DiscriminatorType.PROFILE); 366 break; 367 default: 368 tgt.setValue(org.hl7.fhir.r4.model.ElementDefinition.DiscriminatorType.NULL); 369 break; 370 } 371} 372 return tgt; 373 } 374 375 public static org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionBaseComponent convertElementDefinitionBaseComponent(org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionBaseComponent src) throws FHIRException { 376 if (src == null) return null; 377 org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionBaseComponent tgt = new org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionBaseComponent(); 378 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 379 if (src.hasPath()) tgt.setPathElement(String40_50.convertString(src.getPathElement())); 380 if (src.hasMin()) tgt.setMinElement(UnsignedInt40_50.convertUnsignedInt(src.getMinElement())); 381 if (src.hasMax()) tgt.setMaxElement(String40_50.convertString(src.getMaxElement())); 382 return tgt; 383 } 384 385 public static org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionBaseComponent convertElementDefinitionBaseComponent(org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionBaseComponent src) throws FHIRException { 386 if (src == null) return null; 387 org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionBaseComponent tgt = new org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionBaseComponent(); 388 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 389 if (src.hasPath()) tgt.setPathElement(String40_50.convertString(src.getPathElement())); 390 if (src.hasMin()) tgt.setMinElement(UnsignedInt40_50.convertUnsignedInt(src.getMinElement())); 391 if (src.hasMax()) tgt.setMaxElement(String40_50.convertString(src.getMaxElement())); 392 return tgt; 393 } 394 395 public static org.hl7.fhir.r5.model.ElementDefinition.TypeRefComponent convertTypeRefComponent(org.hl7.fhir.r4.model.ElementDefinition.TypeRefComponent src) throws FHIRException { 396 if (src == null) return null; 397 org.hl7.fhir.r5.model.ElementDefinition.TypeRefComponent tgt = new org.hl7.fhir.r5.model.ElementDefinition.TypeRefComponent(); 398 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 399 if (src.hasCode()) tgt.setCodeElement(Uri40_50.convertUri(src.getCodeElement())); 400 for (org.hl7.fhir.r4.model.CanonicalType t : src.getProfile()) 401 tgt.getProfile().add(Canonical40_50.convertCanonical(t)); 402 for (org.hl7.fhir.r4.model.CanonicalType t : src.getTargetProfile()) 403 tgt.getTargetProfile().add(Canonical40_50.convertCanonical(t)); 404 tgt.setAggregation(src.getAggregation().stream().map(ElementDefinition40_50::convertAggregationMode).collect(Collectors.toList())); 405 if (src.hasVersioning()) tgt.setVersioningElement(convertReferenceVersionRules(src.getVersioningElement())); 406 return tgt; 407 } 408 409 public static org.hl7.fhir.r4.model.ElementDefinition.TypeRefComponent convertTypeRefComponent(org.hl7.fhir.r5.model.ElementDefinition.TypeRefComponent src) throws FHIRException { 410 if (src == null) return null; 411 org.hl7.fhir.r4.model.ElementDefinition.TypeRefComponent tgt = new org.hl7.fhir.r4.model.ElementDefinition.TypeRefComponent(); 412 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 413 if (src.hasCode()) tgt.setCodeElement(Uri40_50.convertUri(src.getCodeElement())); 414 for (org.hl7.fhir.r5.model.CanonicalType t : src.getProfile()) 415 tgt.getProfile().add(Canonical40_50.convertCanonical(t)); 416 for (org.hl7.fhir.r5.model.CanonicalType t : src.getTargetProfile()) 417 tgt.getTargetProfile().add(Canonical40_50.convertCanonical(t)); 418 tgt.setAggregation(src.getAggregation().stream().map(ElementDefinition40_50::convertAggregationMode).collect(Collectors.toList())); 419 if (src.hasVersioning()) tgt.setVersioningElement(convertReferenceVersionRules(src.getVersioningElement())); 420 return tgt; 421 } 422 423 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ElementDefinition.AggregationMode> convertAggregationMode(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ElementDefinition.AggregationMode> src) throws FHIRException { 424 if (src == null || src.isEmpty()) return null; 425 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ElementDefinition.AggregationMode> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.ElementDefinition.AggregationModeEnumFactory()); 426 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 427 if (src.getValue() == null) { 428 tgt.setValue(null); 429} else { 430 switch(src.getValue()) { 431 case CONTAINED: 432 tgt.setValue(ElementDefinition.AggregationMode.CONTAINED); 433 break; 434 case REFERENCED: 435 tgt.setValue(ElementDefinition.AggregationMode.REFERENCED); 436 break; 437 case BUNDLED: 438 tgt.setValue(ElementDefinition.AggregationMode.BUNDLED); 439 break; 440 default: 441 tgt.setValue(ElementDefinition.AggregationMode.NULL); 442 break; 443 } 444} 445 return tgt; 446 } 447 448 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ElementDefinition.AggregationMode> convertAggregationMode(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ElementDefinition.AggregationMode> src) throws FHIRException { 449 if (src == null || src.isEmpty()) return null; 450 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ElementDefinition.AggregationMode> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.ElementDefinition.AggregationModeEnumFactory()); 451 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 452 if (src.getValue() == null) { 453 tgt.setValue(null); 454} else { 455 switch(src.getValue()) { 456 case CONTAINED: 457 tgt.setValue(org.hl7.fhir.r4.model.ElementDefinition.AggregationMode.CONTAINED); 458 break; 459 case REFERENCED: 460 tgt.setValue(org.hl7.fhir.r4.model.ElementDefinition.AggregationMode.REFERENCED); 461 break; 462 case BUNDLED: 463 tgt.setValue(org.hl7.fhir.r4.model.ElementDefinition.AggregationMode.BUNDLED); 464 break; 465 default: 466 tgt.setValue(org.hl7.fhir.r4.model.ElementDefinition.AggregationMode.NULL); 467 break; 468 } 469} 470 return tgt; 471 } 472 473 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ElementDefinition.ReferenceVersionRules> convertReferenceVersionRules(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ElementDefinition.ReferenceVersionRules> src) throws FHIRException { 474 if (src == null || src.isEmpty()) return null; 475 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ElementDefinition.ReferenceVersionRules> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.ElementDefinition.ReferenceVersionRulesEnumFactory()); 476 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 477 if (src.getValue() == null) { 478 tgt.setValue(null); 479} else { 480 switch(src.getValue()) { 481 case EITHER: 482 tgt.setValue(ElementDefinition.ReferenceVersionRules.EITHER); 483 break; 484 case INDEPENDENT: 485 tgt.setValue(ElementDefinition.ReferenceVersionRules.INDEPENDENT); 486 break; 487 case SPECIFIC: 488 tgt.setValue(ElementDefinition.ReferenceVersionRules.SPECIFIC); 489 break; 490 default: 491 tgt.setValue(ElementDefinition.ReferenceVersionRules.NULL); 492 break; 493 } 494} 495 return tgt; 496 } 497 498 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ElementDefinition.ReferenceVersionRules> convertReferenceVersionRules(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ElementDefinition.ReferenceVersionRules> src) throws FHIRException { 499 if (src == null || src.isEmpty()) return null; 500 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ElementDefinition.ReferenceVersionRules> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.ElementDefinition.ReferenceVersionRulesEnumFactory()); 501 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 502 if (src.getValue() == null) { 503 tgt.setValue(null); 504} else { 505 switch(src.getValue()) { 506 case EITHER: 507 tgt.setValue(org.hl7.fhir.r4.model.ElementDefinition.ReferenceVersionRules.EITHER); 508 break; 509 case INDEPENDENT: 510 tgt.setValue(org.hl7.fhir.r4.model.ElementDefinition.ReferenceVersionRules.INDEPENDENT); 511 break; 512 case SPECIFIC: 513 tgt.setValue(org.hl7.fhir.r4.model.ElementDefinition.ReferenceVersionRules.SPECIFIC); 514 break; 515 default: 516 tgt.setValue(org.hl7.fhir.r4.model.ElementDefinition.ReferenceVersionRules.NULL); 517 break; 518 } 519} 520 return tgt; 521 } 522 523 public static org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionExampleComponent convertElementDefinitionExampleComponent(org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionExampleComponent src) throws FHIRException { 524 if (src == null) return null; 525 org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionExampleComponent tgt = new org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionExampleComponent(); 526 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 527 if (src.hasLabel()) tgt.setLabelElement(String40_50.convertString(src.getLabelElement())); 528 if (src.hasValue()) 529 tgt.setValue(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getValue())); 530 return tgt; 531 } 532 533 public static org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionExampleComponent convertElementDefinitionExampleComponent(org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionExampleComponent src) throws FHIRException { 534 if (src == null) return null; 535 org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionExampleComponent tgt = new org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionExampleComponent(); 536 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 537 if (src.hasLabel()) tgt.setLabelElement(String40_50.convertString(src.getLabelElement())); 538 if (src.hasValue()) 539 tgt.setValue(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getValue())); 540 return tgt; 541 } 542 543 public static org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionConstraintComponent convertElementDefinitionConstraintComponent(org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionConstraintComponent src) throws FHIRException { 544 if (src == null) return null; 545 org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionConstraintComponent tgt = new org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionConstraintComponent(); 546 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 547 if (src.hasKey()) tgt.setKeyElement(Id40_50.convertId(src.getKeyElement())); 548 if (src.hasRequirements()) tgt.setRequirementsElement(String40_50.convertStringToMarkdown(src.getRequirementsElement())); 549 if (src.hasSeverity()) tgt.setSeverityElement(convertConstraintSeverity(src.getSeverityElement())); 550 if (src.hasHuman()) tgt.setHumanElement(String40_50.convertString(src.getHumanElement())); 551 if (src.hasExpression()) tgt.setExpressionElement(String40_50.convertString(src.getExpressionElement())); 552 if (src.hasXpath()) { 553 tgt.addExtension(new org.hl7.fhir.r5.model.Extension(org.hl7.fhir.r5.utils.ToolingExtensions.EXT_XPATH_CONSTRAINT, new org.hl7.fhir.r5.model.StringType(src.getXpath()))); 554 } 555 if (src.hasSource()) tgt.setSourceElement(Canonical40_50.convertCanonical(src.getSourceElement())); 556 return tgt; 557 } 558 559 public static org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionConstraintComponent convertElementDefinitionConstraintComponent(org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionConstraintComponent src) throws FHIRException { 560 if (src == null) return null; 561 org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionConstraintComponent tgt = new org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionConstraintComponent(); 562 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt, org.hl7.fhir.r5.utils.ToolingExtensions.EXT_XPATH_CONSTRAINT); 563 if (src.hasKey()) tgt.setKeyElement(Id40_50.convertId(src.getKeyElement())); 564 if (src.hasRequirements()) tgt.setRequirementsElement(String40_50.convertString(src.getRequirementsElement())); 565 if (src.hasSeverity()) tgt.setSeverityElement(convertConstraintSeverity(src.getSeverityElement())); 566 if (src.hasHuman()) tgt.setHumanElement(String40_50.convertString(src.getHumanElement())); 567 if (src.hasExpression()) tgt.setExpressionElement(String40_50.convertString(src.getExpressionElement())); 568 if (org.hl7.fhir.r5.utils.ToolingExtensions.hasExtension(src, org.hl7.fhir.r5.utils.ToolingExtensions.EXT_XPATH_CONSTRAINT)) { 569 tgt.setXpath(org.hl7.fhir.r5.utils.ToolingExtensions.readStringExtension(src, org.hl7.fhir.r5.utils.ToolingExtensions.EXT_XPATH_CONSTRAINT)); 570 } 571 if (src.hasSource()) tgt.setSourceElement(Canonical40_50.convertCanonical(src.getSourceElement())); 572 return tgt; 573 } 574 575 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ElementDefinition.ConstraintSeverity> convertConstraintSeverity(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ElementDefinition.ConstraintSeverity> src) throws FHIRException { 576 if (src == null || src.isEmpty()) return null; 577 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ElementDefinition.ConstraintSeverity> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.ElementDefinition.ConstraintSeverityEnumFactory()); 578 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 579 if (src.getValue() == null) { 580 tgt.setValue(null); 581} else { 582 switch(src.getValue()) { 583 case ERROR: 584 tgt.setValue(ElementDefinition.ConstraintSeverity.ERROR); 585 break; 586 case WARNING: 587 tgt.setValue(ElementDefinition.ConstraintSeverity.WARNING); 588 break; 589 default: 590 tgt.setValue(ElementDefinition.ConstraintSeverity.NULL); 591 break; 592 } 593} 594 return tgt; 595 } 596 597 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ElementDefinition.ConstraintSeverity> convertConstraintSeverity(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ElementDefinition.ConstraintSeverity> src) throws FHIRException { 598 if (src == null || src.isEmpty()) return null; 599 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ElementDefinition.ConstraintSeverity> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.ElementDefinition.ConstraintSeverityEnumFactory()); 600 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 601 if (src.getValue() == null) { 602 tgt.setValue(null); 603} else { 604 switch(src.getValue()) { 605 case ERROR: 606 tgt.setValue(org.hl7.fhir.r4.model.ElementDefinition.ConstraintSeverity.ERROR); 607 break; 608 case WARNING: 609 tgt.setValue(org.hl7.fhir.r4.model.ElementDefinition.ConstraintSeverity.WARNING); 610 break; 611 default: 612 tgt.setValue(org.hl7.fhir.r4.model.ElementDefinition.ConstraintSeverity.NULL); 613 break; 614 } 615} 616 return tgt; 617 } 618 619 public static org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionBindingComponent convertElementDefinitionBindingComponent(org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionBindingComponent src) throws FHIRException { 620 if (src == null) return null; 621 org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionBindingComponent tgt = new org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionBindingComponent(); 622 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt, 623 VersionConvertorConstants.EXT_ADDITIONAL_BINDING, ToolingExtensions.EXT_BINDING_ADDITIONAL); 624 if (src.hasStrength()) tgt.setStrengthElement(Enumerations40_50.convertBindingStrength(src.getStrengthElement())); 625 if (src.hasDescription()) tgt.setDescriptionElement(String40_50.convertStringToMarkdown(src.getDescriptionElement())); 626 if (src.hasValueSet()) tgt.setValueSetElement(Canonical40_50.convertCanonical(src.getValueSetElement())); 627 628 for (org.hl7.fhir.r4.model.Extension ext : src.getExtensionsByUrl(VersionConvertorConstants.EXT_ADDITIONAL_BINDING)) { 629 tgt.addAdditional(convertAdditional(ext)); 630 } 631 for (org.hl7.fhir.r4.model.Extension ext : src.getExtensionsByUrl(ToolingExtensions.EXT_BINDING_ADDITIONAL)) { 632 tgt.addAdditional(convertAdditional(ext)); 633 } 634 return tgt; 635 } 636 637 private static ElementDefinitionBindingAdditionalComponent convertAdditional(Extension src) { 638 if (src == null) return null; 639 ElementDefinitionBindingAdditionalComponent tgt = new ElementDefinitionBindingAdditionalComponent(); 640 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt, "valueSet", "purpose", "documentation", "shortDoco", "usage", "any"); 641 if (src.hasExtension("purpose")) { 642 tgt.getPurposeElement().setValueAsString(src.getExtensionByUrl("purpose").getValue().primitiveValue()); 643 } 644 if (src.hasExtension("valueSet")) { 645 tgt.setValueSetElement(Canonical40_50.convertCanonical((CanonicalType) src.getExtensionByUrl("valueSet").getValue())); 646 } 647 if (src.hasExtension("documentation")) { 648 tgt.setDocumentationElement(MarkDown40_50.convertMarkdown((MarkdownType) src.getExtensionByUrl("documentation").getValue())); 649 } 650 if (src.hasExtension("shortDoco")) { 651 tgt.setShortDocoElement(String40_50.convertString((StringType) src.getExtensionByUrl("shortDoco").getValue())); 652 } 653 for (Extension t : src.getExtensionsByUrl("usage")) { 654 tgt.addUsage(UsageContext40_50.convertUsageContext((org.hl7.fhir.r4.model.UsageContext) t.getValue())); 655 } 656 if (src.hasExtension("any")) { 657 tgt.setAnyElement(Boolean40_50.convertBoolean((BooleanType) src.getExtensionByUrl("any").getValue())); 658 } 659 return tgt; 660 } 661 662 private static org.hl7.fhir.r4.model.Extension convertAdditional(ElementDefinitionBindingAdditionalComponent src) { 663 if (src == null) return null; 664 org.hl7.fhir.r4.model.Extension tgt = new Extension(ToolingExtensions.EXT_BINDING_ADDITIONAL); 665 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 666 if (src.hasPurpose()) { 667 tgt.addExtension(new Extension("purpose", new org.hl7.fhir.r4.model.CodeType(src.getPurposeElement().primitiveValue()))); 668 } 669 if (src.hasValueSet()) { 670 tgt.addExtension(new Extension("valueSet", Canonical40_50.convertCanonical(src.getValueSetElement()))); 671 } 672 if (src.hasDocumentation()) { 673 tgt.addExtension(new Extension("documentation", MarkDown40_50.convertMarkdown(src.getDocumentationElement()))); 674 } 675 if (src.hasShortDoco()) { 676 tgt.addExtension(new Extension("shortDoco", String40_50.convertString(src.getShortDocoElement()))); 677 } 678 for (UsageContext t : src.getUsage()) { 679 tgt.addExtension(new Extension("usage", UsageContext40_50.convertUsageContext(t))); 680 } 681 if (src.hasAny()) { 682 tgt.addExtension(new Extension("any", Boolean40_50.convertBoolean(src.getAnyElement()))); 683 } 684 685 return tgt; 686 } 687 688 public static org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionBindingComponent convertElementDefinitionBindingComponent(org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionBindingComponent src) throws FHIRException { 689 if (src == null) return null; 690 org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionBindingComponent tgt = new org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionBindingComponent(); 691 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 692 if (src.hasStrength()) tgt.setStrengthElement(Enumerations40_50.convertBindingStrength(src.getStrengthElement())); 693 if (src.hasDescription()) tgt.setDescriptionElement(String40_50.convertString(src.getDescriptionElement())); 694 if (src.hasValueSet()) tgt.setValueSetElement(Canonical40_50.convertCanonical(src.getValueSetElement())); 695 for (ElementDefinitionBindingAdditionalComponent ab : src.getAdditional()) { 696 tgt.addExtension(convertAdditional(ab)); 697 } 698 return tgt; 699 } 700 701 public static org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionMappingComponent convertElementDefinitionMappingComponent(org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionMappingComponent src) throws FHIRException { 702 if (src == null) return null; 703 org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionMappingComponent tgt = new org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionMappingComponent(); 704 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 705 if (src.hasIdentity()) tgt.setIdentityElement(Id40_50.convertId(src.getIdentityElement())); 706 if (src.hasLanguage()) tgt.setLanguageElement(Code40_50.convertCode(src.getLanguageElement())); 707 if (src.hasMap()) tgt.setMapElement(String40_50.convertString(src.getMapElement())); 708 if (src.hasComment()) tgt.setCommentElement(String40_50.convertStringToMarkdown(src.getCommentElement())); 709 return tgt; 710 } 711 712 public static org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionMappingComponent convertElementDefinitionMappingComponent(org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionMappingComponent src) throws FHIRException { 713 if (src == null) return null; 714 org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionMappingComponent tgt = new org.hl7.fhir.r4.model.ElementDefinition.ElementDefinitionMappingComponent(); 715 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 716 if (src.hasIdentity()) tgt.setIdentityElement(Id40_50.convertId(src.getIdentityElement())); 717 if (src.hasLanguage()) tgt.setLanguageElement(Code40_50.convertCode(src.getLanguageElement())); 718 if (src.hasMap()) tgt.setMapElement(String40_50.convertString(src.getMapElement())); 719 if (src.hasComment()) tgt.setCommentElement(String40_50.convertString(src.getCommentElement())); 720 return tgt; 721 } 722}