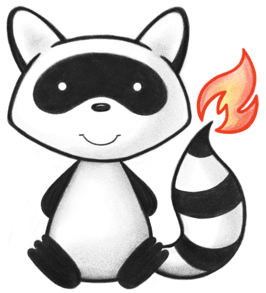
001package org.hl7.fhir.convertors.conv40_50.resources40_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext40_50; 004import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.CodeableConcept40_50; 005import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Identifier40_50; 006import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Period40_50; 007import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Boolean40_50; 008import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.PositiveInt40_50; 009import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.String40_50; 010import org.hl7.fhir.convertors.conv40_50.datatypes40_50.special40_50.Reference40_50; 011import org.hl7.fhir.exceptions.FHIRException; 012import org.hl7.fhir.r5.model.Account; 013import org.hl7.fhir.r5.model.Enumeration; 014 015/* 016 Copyright (c) 2011+, HL7, Inc. 017 All rights reserved. 018 019 Redistribution and use in source and binary forms, with or without modification, 020 are permitted provided that the following conditions are met: 021 022 * Redistributions of source code must retain the above copyright notice, this 023 list of conditions and the following disclaimer. 024 * Redistributions in binary form must reproduce the above copyright notice, 025 this list of conditions and the following disclaimer in the documentation 026 and/or other materials provided with the distribution. 027 * Neither the name of HL7 nor the names of its contributors may be used to 028 endorse or promote products derived from this software without specific 029 prior written permission. 030 031 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 032 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 033 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 034 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 035 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 036 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 037 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 038 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 039 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 040 POSSIBILITY OF SUCH DAMAGE. 041 042*/ 043// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 044public class Account40_50 { 045 046 public static org.hl7.fhir.r5.model.Account convertAccount(org.hl7.fhir.r4.model.Account src) throws FHIRException { 047 if (src == null) 048 return null; 049 org.hl7.fhir.r5.model.Account tgt = new org.hl7.fhir.r5.model.Account(); 050 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 051 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 052 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 053 if (src.hasStatus()) 054 tgt.setStatusElement(convertAccountStatus(src.getStatusElement())); 055 if (src.hasType()) 056 tgt.setType(CodeableConcept40_50.convertCodeableConcept(src.getType())); 057 if (src.hasName()) 058 tgt.setNameElement(String40_50.convertString(src.getNameElement())); 059 for (org.hl7.fhir.r4.model.Reference t : src.getSubject()) tgt.addSubject(Reference40_50.convertReference(t)); 060 if (src.hasServicePeriod()) 061 tgt.setServicePeriod(Period40_50.convertPeriod(src.getServicePeriod())); 062 for (org.hl7.fhir.r4.model.Account.CoverageComponent t : src.getCoverage()) 063 tgt.addCoverage(convertCoverageComponent(t)); 064 if (src.hasOwner()) 065 tgt.setOwner(Reference40_50.convertReference(src.getOwner())); 066 if (src.hasDescription()) 067 tgt.setDescriptionElement(String40_50.convertStringToMarkdown(src.getDescriptionElement())); 068 for (org.hl7.fhir.r4.model.Account.GuarantorComponent t : src.getGuarantor()) 069 tgt.addGuarantor(convertGuarantorComponent(t)); 070// if (src.hasPartOf()) 071// tgt.setPartOf(Reference40_50.convertReference(src.getPartOf())); 072 return tgt; 073 } 074 075 public static org.hl7.fhir.r4.model.Account convertAccount(org.hl7.fhir.r5.model.Account src) throws FHIRException { 076 if (src == null) 077 return null; 078 org.hl7.fhir.r4.model.Account tgt = new org.hl7.fhir.r4.model.Account(); 079 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 080 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 081 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 082 if (src.hasStatus()) 083 tgt.setStatusElement(convertAccountStatus(src.getStatusElement())); 084 if (src.hasType()) 085 tgt.setType(CodeableConcept40_50.convertCodeableConcept(src.getType())); 086 if (src.hasName()) 087 tgt.setNameElement(String40_50.convertString(src.getNameElement())); 088 for (org.hl7.fhir.r5.model.Reference t : src.getSubject()) tgt.addSubject(Reference40_50.convertReference(t)); 089 if (src.hasServicePeriod()) 090 tgt.setServicePeriod(Period40_50.convertPeriod(src.getServicePeriod())); 091 for (org.hl7.fhir.r5.model.Account.CoverageComponent t : src.getCoverage()) 092 tgt.addCoverage(convertCoverageComponent(t)); 093 if (src.hasOwner()) 094 tgt.setOwner(Reference40_50.convertReference(src.getOwner())); 095 if (src.hasDescription()) 096 tgt.setDescriptionElement(String40_50.convertString(src.getDescriptionElement())); 097 for (org.hl7.fhir.r5.model.Account.GuarantorComponent t : src.getGuarantor()) 098 tgt.addGuarantor(convertGuarantorComponent(t)); 099// if (src.hasPartOf()) 100// tgt.setPartOf(Reference40_50.convertReference(src.getPartOf())); 101 return tgt; 102 } 103 104 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Account.AccountStatus> convertAccountStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Account.AccountStatus> src) throws FHIRException { 105 if (src == null || src.isEmpty()) 106 return null; 107 Enumeration<Account.AccountStatus> tgt = new Enumeration<>(new Account.AccountStatusEnumFactory()); 108 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 109 if (src.getValue() == null) { 110 tgt.setValue(null); 111 } else { 112 switch (src.getValue()) { 113 case ACTIVE: 114 tgt.setValue(Account.AccountStatus.ACTIVE); 115 break; 116 case INACTIVE: 117 tgt.setValue(Account.AccountStatus.INACTIVE); 118 break; 119 case ENTEREDINERROR: 120 tgt.setValue(Account.AccountStatus.ENTEREDINERROR); 121 break; 122 case ONHOLD: 123 tgt.setValue(Account.AccountStatus.ONHOLD); 124 break; 125 case UNKNOWN: 126 tgt.setValue(Account.AccountStatus.UNKNOWN); 127 break; 128 default: 129 tgt.setValue(Account.AccountStatus.NULL); 130 break; 131 } 132 } 133 return tgt; 134 } 135 136 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Account.AccountStatus> convertAccountStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Account.AccountStatus> src) throws FHIRException { 137 if (src == null || src.isEmpty()) 138 return null; 139 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Account.AccountStatus> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Account.AccountStatusEnumFactory()); 140 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 141 if (src.getValue() == null) { 142 tgt.setValue(null); 143 } else { 144 switch (src.getValue()) { 145 case ACTIVE: 146 tgt.setValue(org.hl7.fhir.r4.model.Account.AccountStatus.ACTIVE); 147 break; 148 case INACTIVE: 149 tgt.setValue(org.hl7.fhir.r4.model.Account.AccountStatus.INACTIVE); 150 break; 151 case ENTEREDINERROR: 152 tgt.setValue(org.hl7.fhir.r4.model.Account.AccountStatus.ENTEREDINERROR); 153 break; 154 case ONHOLD: 155 tgt.setValue(org.hl7.fhir.r4.model.Account.AccountStatus.ONHOLD); 156 break; 157 case UNKNOWN: 158 tgt.setValue(org.hl7.fhir.r4.model.Account.AccountStatus.UNKNOWN); 159 break; 160 default: 161 tgt.setValue(org.hl7.fhir.r4.model.Account.AccountStatus.NULL); 162 break; 163 } 164 } 165 return tgt; 166 } 167 168 public static org.hl7.fhir.r5.model.Account.CoverageComponent convertCoverageComponent(org.hl7.fhir.r4.model.Account.CoverageComponent src) throws FHIRException { 169 if (src == null) 170 return null; 171 org.hl7.fhir.r5.model.Account.CoverageComponent tgt = new org.hl7.fhir.r5.model.Account.CoverageComponent(); 172 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 173 if (src.hasCoverage()) 174 tgt.setCoverage(Reference40_50.convertReference(src.getCoverage())); 175 if (src.hasPriority()) 176 tgt.setPriorityElement(PositiveInt40_50.convertPositiveInt(src.getPriorityElement())); 177 return tgt; 178 } 179 180 public static org.hl7.fhir.r4.model.Account.CoverageComponent convertCoverageComponent(org.hl7.fhir.r5.model.Account.CoverageComponent src) throws FHIRException { 181 if (src == null) 182 return null; 183 org.hl7.fhir.r4.model.Account.CoverageComponent tgt = new org.hl7.fhir.r4.model.Account.CoverageComponent(); 184 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 185 if (src.hasCoverage()) 186 tgt.setCoverage(Reference40_50.convertReference(src.getCoverage())); 187 if (src.hasPriority()) 188 tgt.setPriorityElement(PositiveInt40_50.convertPositiveInt(src.getPriorityElement())); 189 return tgt; 190 } 191 192 public static org.hl7.fhir.r5.model.Account.GuarantorComponent convertGuarantorComponent(org.hl7.fhir.r4.model.Account.GuarantorComponent src) throws FHIRException { 193 if (src == null) 194 return null; 195 org.hl7.fhir.r5.model.Account.GuarantorComponent tgt = new org.hl7.fhir.r5.model.Account.GuarantorComponent(); 196 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 197 if (src.hasParty()) 198 tgt.setParty(Reference40_50.convertReference(src.getParty())); 199 if (src.hasOnHold()) 200 tgt.setOnHoldElement(Boolean40_50.convertBoolean(src.getOnHoldElement())); 201 if (src.hasPeriod()) 202 tgt.setPeriod(Period40_50.convertPeriod(src.getPeriod())); 203 return tgt; 204 } 205 206 public static org.hl7.fhir.r4.model.Account.GuarantorComponent convertGuarantorComponent(org.hl7.fhir.r5.model.Account.GuarantorComponent src) throws FHIRException { 207 if (src == null) 208 return null; 209 org.hl7.fhir.r4.model.Account.GuarantorComponent tgt = new org.hl7.fhir.r4.model.Account.GuarantorComponent(); 210 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 211 if (src.hasParty()) 212 tgt.setParty(Reference40_50.convertReference(src.getParty())); 213 if (src.hasOnHold()) 214 tgt.setOnHoldElement(Boolean40_50.convertBoolean(src.getOnHoldElement())); 215 if (src.hasPeriod()) 216 tgt.setPeriod(Period40_50.convertPeriod(src.getPeriod())); 217 return tgt; 218 } 219}