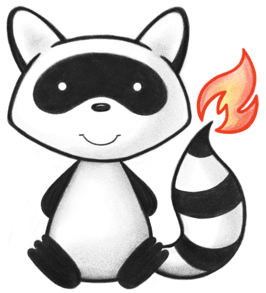
001package org.hl7.fhir.convertors.conv40_50.resources40_50; 002 003import java.util.stream.Collectors; 004 005import org.hl7.fhir.convertors.context.ConversionContext40_50; 006import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Annotation40_50; 007import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.CodeableConcept40_50; 008import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Identifier40_50; 009import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.DateTime40_50; 010import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.String40_50; 011import org.hl7.fhir.convertors.conv40_50.datatypes40_50.special40_50.Reference40_50; 012import org.hl7.fhir.exceptions.FHIRException; 013import org.hl7.fhir.r5.model.*; 014import org.hl7.fhir.r5.model.AllergyIntolerance.AllergyIntoleranceParticipantComponent; 015 016/* 017 Copyright (c) 2011+, HL7, Inc. 018 All rights reserved. 019 020 Redistribution and use in source and binary forms, with or without modification, 021 are permitted provided that the following conditions are met: 022 023 * Redistributions of source code must retain the above copyright notice, this 024 list of conditions and the following disclaimer. 025 * Redistributions in binary form must reproduce the above copyright notice, 026 this list of conditions and the following disclaimer in the documentation 027 and/or other materials provided with the distribution. 028 * Neither the name of HL7 nor the names of its contributors may be used to 029 endorse or promote products derived from this software without specific 030 prior written permission. 031 032 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 033 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 034 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 035 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 036 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 037 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 038 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 039 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 040 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 041 POSSIBILITY OF SUCH DAMAGE. 042 043*/ 044// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 045public class AllergyIntolerance40_50 { 046 047 public static org.hl7.fhir.r5.model.AllergyIntolerance convertAllergyIntolerance(org.hl7.fhir.r4.model.AllergyIntolerance src) throws FHIRException { 048 if (src == null) 049 return null; 050 org.hl7.fhir.r5.model.AllergyIntolerance tgt = new org.hl7.fhir.r5.model.AllergyIntolerance(); 051 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 052 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 053 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 054 if (src.hasClinicalStatus()) 055 tgt.setClinicalStatus(CodeableConcept40_50.convertCodeableConcept(src.getClinicalStatus())); 056 if (src.hasVerificationStatus()) 057 tgt.setVerificationStatus(CodeableConcept40_50.convertCodeableConcept(src.getVerificationStatus())); 058 if (src.hasType()) 059 tgt.setType(convertAllergyIntoleranceType(src.getTypeElement())); 060 tgt.setCategory(src.getCategory().stream() 061 .map(AllergyIntolerance40_50::convertAllergyIntoleranceCategory) 062 .collect(Collectors.toList())); 063 if (src.hasCriticality()) 064 tgt.setCriticalityElement(convertAllergyIntoleranceCriticality(src.getCriticalityElement())); 065 if (src.hasCode()) 066 tgt.setCode(CodeableConcept40_50.convertCodeableConcept(src.getCode())); 067 if (src.hasPatient()) 068 tgt.setPatient(Reference40_50.convertReference(src.getPatient())); 069 if (src.hasEncounter()) 070 tgt.setEncounter(Reference40_50.convertReference(src.getEncounter())); 071 if (src.hasOnset()) 072 tgt.setOnset(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getOnset())); 073 if (src.hasRecordedDate()) 074 tgt.setRecordedDateElement(DateTime40_50.convertDateTime(src.getRecordedDateElement())); 075 if (src.hasRecorder()) 076 tgt.addParticipant(new AllergyIntoleranceParticipantComponent() 077 .setFunction(new CodeableConcept().addCoding(new Coding("http://terminology.hl7.org/CodeSystem/provenance-participant-type", "author", "Author"))) 078 .setActor(Reference40_50.convertReference(src.getRecorder()))); 079 if (src.hasAsserter()) 080 tgt.addParticipant(new AllergyIntoleranceParticipantComponent() 081 .setFunction(new CodeableConcept().addCoding(new Coding("http://terminology.hl7.org/CodeSystem/provenance-participant-type", "attester", "Attester"))) 082 .setActor(Reference40_50.convertReference(src.getRecorder()))); 083 if (src.hasLastOccurrence()) 084 tgt.setLastOccurrenceElement(DateTime40_50.convertDateTime(src.getLastOccurrenceElement())); 085 for (org.hl7.fhir.r4.model.Annotation t : src.getNote()) tgt.addNote(Annotation40_50.convertAnnotation(t)); 086 for (org.hl7.fhir.r4.model.AllergyIntolerance.AllergyIntoleranceReactionComponent t : src.getReaction()) 087 tgt.addReaction(convertAllergyIntoleranceReactionComponent(t)); 088 return tgt; 089 } 090 091 public static org.hl7.fhir.r4.model.AllergyIntolerance convertAllergyIntolerance(org.hl7.fhir.r5.model.AllergyIntolerance src) throws FHIRException { 092 if (src == null) 093 return null; 094 org.hl7.fhir.r4.model.AllergyIntolerance tgt = new org.hl7.fhir.r4.model.AllergyIntolerance(); 095 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 096 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 097 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 098 if (src.hasClinicalStatus()) 099 tgt.setClinicalStatus(CodeableConcept40_50.convertCodeableConcept(src.getClinicalStatus())); 100 if (src.hasVerificationStatus()) 101 tgt.setVerificationStatus(CodeableConcept40_50.convertCodeableConcept(src.getVerificationStatus())); 102 if (src.hasType()) 103 tgt.setTypeElement(convertAllergyIntoleranceType(src.getType())); 104 tgt.setCategory(src.getCategory().stream() 105 .map(AllergyIntolerance40_50::convertAllergyIntoleranceCategory) 106 .collect(Collectors.toList())); 107 if (src.hasCriticality()) 108 tgt.setCriticalityElement(convertAllergyIntoleranceCriticality(src.getCriticalityElement())); 109 if (src.hasCode()) 110 tgt.setCode(CodeableConcept40_50.convertCodeableConcept(src.getCode())); 111 if (src.hasPatient()) 112 tgt.setPatient(Reference40_50.convertReference(src.getPatient())); 113 if (src.hasEncounter()) 114 tgt.setEncounter(Reference40_50.convertReference(src.getEncounter())); 115 if (src.hasOnset()) 116 tgt.setOnset(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getOnset())); 117 if (src.hasRecordedDate()) 118 tgt.setRecordedDateElement(DateTime40_50.convertDateTime(src.getRecordedDateElement())); 119 for (AllergyIntoleranceParticipantComponent t : src.getParticipant()) { 120 if (t.getFunction().hasCoding("http://terminology.hl7.org/CodeSystem/provenance-participant-type", "author")) 121 tgt.setRecorder(Reference40_50.convertReference(t.getActor())); 122 if (t.getFunction().hasCoding("http://terminology.hl7.org/CodeSystem/provenance-participant-type", "attester")) 123 tgt.setAsserter(Reference40_50.convertReference(t.getActor())); 124 } 125 if (src.hasLastOccurrence()) 126 tgt.setLastOccurrenceElement(DateTime40_50.convertDateTime(src.getLastOccurrenceElement())); 127 for (org.hl7.fhir.r5.model.Annotation t : src.getNote()) tgt.addNote(Annotation40_50.convertAnnotation(t)); 128 for (org.hl7.fhir.r5.model.AllergyIntolerance.AllergyIntoleranceReactionComponent t : src.getReaction()) 129 tgt.addReaction(convertAllergyIntoleranceReactionComponent(t)); 130 return tgt; 131 } 132 133 static public org.hl7.fhir.r5.model.CodeableConcept convertAllergyIntoleranceType(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.AllergyIntolerance.AllergyIntoleranceType> src) throws FHIRException { 134 if (src == null || src.isEmpty()) 135 return null; 136 CodeableConcept tgt = new CodeableConcept(); 137 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 138 if (src.getValue() == null) { 139 // Add nothing 140 } else { 141 switch (src.getValue()) { 142 case ALLERGY: 143 tgt.addCoding("http://hl7.org/fhir/allergy-intolerance-type", "allergy", "Allergy"); 144 break; 145 case INTOLERANCE: 146 tgt.addCoding("http://hl7.org/fhir/allergy-intolerance-type", "intolerance", "Intolerance"); 147 break; 148 default: 149 break; 150 } 151 } 152 return tgt; 153 } 154 155 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.AllergyIntolerance.AllergyIntoleranceType> convertAllergyIntoleranceType(org.hl7.fhir.r5.model.CodeableConcept src) throws FHIRException { 156 if (src == null || src.isEmpty()) 157 return null; 158 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.AllergyIntolerance.AllergyIntoleranceType> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.AllergyIntolerance.AllergyIntoleranceTypeEnumFactory()); 159 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 160 if (src.hasCoding("http://hl7.org/fhir/allergy-intolerance-type", "allergy")) { 161 tgt.setValue(org.hl7.fhir.r4.model.AllergyIntolerance.AllergyIntoleranceType.ALLERGY); 162 } else if (src.hasCoding("http://hl7.org/fhir/allergy-intolerance-type", "intolerance")) { 163 tgt.setValue(org.hl7.fhir.r4.model.AllergyIntolerance.AllergyIntoleranceType.INTOLERANCE); 164 } 165 return tgt; 166 } 167 168 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.AllergyIntolerance.AllergyIntoleranceCategory> convertAllergyIntoleranceCategory(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.AllergyIntolerance.AllergyIntoleranceCategory> src) throws FHIRException { 169 if (src == null || src.isEmpty()) 170 return null; 171 Enumeration<AllergyIntolerance.AllergyIntoleranceCategory> tgt = new Enumeration<>(new AllergyIntolerance.AllergyIntoleranceCategoryEnumFactory()); 172 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 173 if (src.getValue() == null) { 174 tgt.setValue(null); 175 } else { 176 switch (src.getValue()) { 177 case FOOD: 178 tgt.setValue(AllergyIntolerance.AllergyIntoleranceCategory.FOOD); 179 break; 180 case MEDICATION: 181 tgt.setValue(AllergyIntolerance.AllergyIntoleranceCategory.MEDICATION); 182 break; 183 case ENVIRONMENT: 184 tgt.setValue(AllergyIntolerance.AllergyIntoleranceCategory.ENVIRONMENT); 185 break; 186 case BIOLOGIC: 187 tgt.setValue(AllergyIntolerance.AllergyIntoleranceCategory.BIOLOGIC); 188 break; 189 default: 190 tgt.setValue(AllergyIntolerance.AllergyIntoleranceCategory.NULL); 191 break; 192 } 193 } 194 return tgt; 195 } 196 197 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.AllergyIntolerance.AllergyIntoleranceCategory> convertAllergyIntoleranceCategory(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.AllergyIntolerance.AllergyIntoleranceCategory> src) throws FHIRException { 198 if (src == null || src.isEmpty()) 199 return null; 200 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.AllergyIntolerance.AllergyIntoleranceCategory> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.AllergyIntolerance.AllergyIntoleranceCategoryEnumFactory()); 201 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 202 if (src.getValue() == null) { 203 tgt.setValue(null); 204 } else { 205 switch (src.getValue()) { 206 case FOOD: 207 tgt.setValue(org.hl7.fhir.r4.model.AllergyIntolerance.AllergyIntoleranceCategory.FOOD); 208 break; 209 case MEDICATION: 210 tgt.setValue(org.hl7.fhir.r4.model.AllergyIntolerance.AllergyIntoleranceCategory.MEDICATION); 211 break; 212 case ENVIRONMENT: 213 tgt.setValue(org.hl7.fhir.r4.model.AllergyIntolerance.AllergyIntoleranceCategory.ENVIRONMENT); 214 break; 215 case BIOLOGIC: 216 tgt.setValue(org.hl7.fhir.r4.model.AllergyIntolerance.AllergyIntoleranceCategory.BIOLOGIC); 217 break; 218 default: 219 tgt.setValue(org.hl7.fhir.r4.model.AllergyIntolerance.AllergyIntoleranceCategory.NULL); 220 break; 221 } 222 } 223 return tgt; 224 } 225 226 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.AllergyIntolerance.AllergyIntoleranceCriticality> convertAllergyIntoleranceCriticality(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.AllergyIntolerance.AllergyIntoleranceCriticality> src) throws FHIRException { 227 if (src == null || src.isEmpty()) 228 return null; 229 Enumeration<AllergyIntolerance.AllergyIntoleranceCriticality> tgt = new Enumeration<>(new AllergyIntolerance.AllergyIntoleranceCriticalityEnumFactory()); 230 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 231 if (src.getValue() == null) { 232 tgt.setValue(null); 233 } else { 234 switch (src.getValue()) { 235 case LOW: 236 tgt.setValue(AllergyIntolerance.AllergyIntoleranceCriticality.LOW); 237 break; 238 case HIGH: 239 tgt.setValue(AllergyIntolerance.AllergyIntoleranceCriticality.HIGH); 240 break; 241 case UNABLETOASSESS: 242 tgt.setValue(AllergyIntolerance.AllergyIntoleranceCriticality.UNABLETOASSESS); 243 break; 244 default: 245 tgt.setValue(AllergyIntolerance.AllergyIntoleranceCriticality.NULL); 246 break; 247 } 248 } 249 return tgt; 250 } 251 252 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.AllergyIntolerance.AllergyIntoleranceCriticality> convertAllergyIntoleranceCriticality(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.AllergyIntolerance.AllergyIntoleranceCriticality> src) throws FHIRException { 253 if (src == null || src.isEmpty()) 254 return null; 255 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.AllergyIntolerance.AllergyIntoleranceCriticality> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.AllergyIntolerance.AllergyIntoleranceCriticalityEnumFactory()); 256 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 257 if (src.getValue() == null) { 258 tgt.setValue(null); 259 } else { 260 switch (src.getValue()) { 261 case LOW: 262 tgt.setValue(org.hl7.fhir.r4.model.AllergyIntolerance.AllergyIntoleranceCriticality.LOW); 263 break; 264 case HIGH: 265 tgt.setValue(org.hl7.fhir.r4.model.AllergyIntolerance.AllergyIntoleranceCriticality.HIGH); 266 break; 267 case UNABLETOASSESS: 268 tgt.setValue(org.hl7.fhir.r4.model.AllergyIntolerance.AllergyIntoleranceCriticality.UNABLETOASSESS); 269 break; 270 default: 271 tgt.setValue(org.hl7.fhir.r4.model.AllergyIntolerance.AllergyIntoleranceCriticality.NULL); 272 break; 273 } 274 } 275 return tgt; 276 } 277 278 public static org.hl7.fhir.r5.model.AllergyIntolerance.AllergyIntoleranceReactionComponent convertAllergyIntoleranceReactionComponent(org.hl7.fhir.r4.model.AllergyIntolerance.AllergyIntoleranceReactionComponent src) throws FHIRException { 279 if (src == null) 280 return null; 281 org.hl7.fhir.r5.model.AllergyIntolerance.AllergyIntoleranceReactionComponent tgt = new org.hl7.fhir.r5.model.AllergyIntolerance.AllergyIntoleranceReactionComponent(); 282 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 283 if (src.hasSubstance()) 284 tgt.setSubstance(CodeableConcept40_50.convertCodeableConcept(src.getSubstance())); 285 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getManifestation()) 286 tgt.addManifestation(new CodeableReference(CodeableConcept40_50.convertCodeableConcept(t))); 287 if (src.hasDescription()) 288 tgt.setDescriptionElement(String40_50.convertString(src.getDescriptionElement())); 289 if (src.hasOnset()) 290 tgt.setOnsetElement(DateTime40_50.convertDateTime(src.getOnsetElement())); 291 if (src.hasSeverity()) 292 tgt.setSeverityElement(convertAllergyIntoleranceSeverity(src.getSeverityElement())); 293 if (src.hasExposureRoute()) 294 tgt.setExposureRoute(CodeableConcept40_50.convertCodeableConcept(src.getExposureRoute())); 295 for (org.hl7.fhir.r4.model.Annotation t : src.getNote()) tgt.addNote(Annotation40_50.convertAnnotation(t)); 296 return tgt; 297 } 298 299 public static org.hl7.fhir.r4.model.AllergyIntolerance.AllergyIntoleranceReactionComponent convertAllergyIntoleranceReactionComponent(org.hl7.fhir.r5.model.AllergyIntolerance.AllergyIntoleranceReactionComponent src) throws FHIRException { 300 if (src == null) 301 return null; 302 org.hl7.fhir.r4.model.AllergyIntolerance.AllergyIntoleranceReactionComponent tgt = new org.hl7.fhir.r4.model.AllergyIntolerance.AllergyIntoleranceReactionComponent(); 303 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 304 if (src.hasSubstance()) 305 tgt.setSubstance(CodeableConcept40_50.convertCodeableConcept(src.getSubstance())); 306 for (CodeableReference t : src.getManifestation()) 307 if (t.hasConcept()) tgt.addManifestation(CodeableConcept40_50.convertCodeableConcept(t.getConcept())); 308 if (src.hasDescription()) 309 tgt.setDescriptionElement(String40_50.convertString(src.getDescriptionElement())); 310 if (src.hasOnset()) 311 tgt.setOnsetElement(DateTime40_50.convertDateTime(src.getOnsetElement())); 312 if (src.hasSeverity()) 313 tgt.setSeverityElement(convertAllergyIntoleranceSeverity(src.getSeverityElement())); 314 if (src.hasExposureRoute()) 315 tgt.setExposureRoute(CodeableConcept40_50.convertCodeableConcept(src.getExposureRoute())); 316 for (org.hl7.fhir.r5.model.Annotation t : src.getNote()) tgt.addNote(Annotation40_50.convertAnnotation(t)); 317 return tgt; 318 } 319 320 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.AllergyIntolerance.AllergyIntoleranceSeverity> convertAllergyIntoleranceSeverity(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.AllergyIntolerance.AllergyIntoleranceSeverity> src) throws FHIRException { 321 if (src == null || src.isEmpty()) 322 return null; 323 Enumeration<AllergyIntolerance.AllergyIntoleranceSeverity> tgt = new Enumeration<>(new AllergyIntolerance.AllergyIntoleranceSeverityEnumFactory()); 324 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 325 if (src.getValue() == null) { 326 tgt.setValue(null); 327 } else { 328 switch (src.getValue()) { 329 case MILD: 330 tgt.setValue(AllergyIntolerance.AllergyIntoleranceSeverity.MILD); 331 break; 332 case MODERATE: 333 tgt.setValue(AllergyIntolerance.AllergyIntoleranceSeverity.MODERATE); 334 break; 335 case SEVERE: 336 tgt.setValue(AllergyIntolerance.AllergyIntoleranceSeverity.SEVERE); 337 break; 338 default: 339 tgt.setValue(AllergyIntolerance.AllergyIntoleranceSeverity.NULL); 340 break; 341 } 342 } 343 return tgt; 344 } 345 346 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.AllergyIntolerance.AllergyIntoleranceSeverity> convertAllergyIntoleranceSeverity(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.AllergyIntolerance.AllergyIntoleranceSeverity> src) throws FHIRException { 347 if (src == null || src.isEmpty()) 348 return null; 349 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.AllergyIntolerance.AllergyIntoleranceSeverity> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.AllergyIntolerance.AllergyIntoleranceSeverityEnumFactory()); 350 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 351 if (src.getValue() == null) { 352 tgt.setValue(null); 353 } else { 354 switch (src.getValue()) { 355 case MILD: 356 tgt.setValue(org.hl7.fhir.r4.model.AllergyIntolerance.AllergyIntoleranceSeverity.MILD); 357 break; 358 case MODERATE: 359 tgt.setValue(org.hl7.fhir.r4.model.AllergyIntolerance.AllergyIntoleranceSeverity.MODERATE); 360 break; 361 case SEVERE: 362 tgt.setValue(org.hl7.fhir.r4.model.AllergyIntolerance.AllergyIntoleranceSeverity.SEVERE); 363 break; 364 default: 365 tgt.setValue(org.hl7.fhir.r4.model.AllergyIntolerance.AllergyIntoleranceSeverity.NULL); 366 break; 367 } 368 } 369 return tgt; 370 } 371}