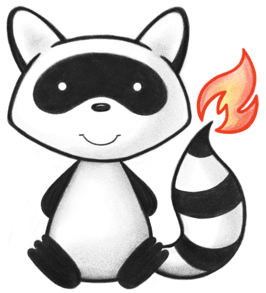
001package org.hl7.fhir.convertors.conv40_50.resources40_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext40_50; 004import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.CodeableConcept40_50; 005import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Identifier40_50; 006import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Period40_50; 007import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.DateTime40_50; 008import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Instant40_50; 009import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.MarkDown40_50; 010import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.PositiveInt40_50; 011import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.String40_50; 012import org.hl7.fhir.convertors.conv40_50.datatypes40_50.special40_50.Reference40_50; 013import org.hl7.fhir.exceptions.FHIRException; 014import org.hl7.fhir.r4.model.UnsignedIntType; 015import org.hl7.fhir.r5.model.*; 016 017/* 018 Copyright (c) 2011+, HL7, Inc. 019 All rights reserved. 020 021 Redistribution and use in source and binary forms, with or without modification, 022 are permitted provided that the following conditions are met: 023 024 * Redistributions of source code must retain the above copyright notice, this 025 list of conditions and the following disclaimer. 026 * Redistributions in binary form must reproduce the above copyright notice, 027 this list of conditions and the following disclaimer in the documentation 028 and/or other materials provided with the distribution. 029 * Neither the name of HL7 nor the names of its contributors may be used to 030 endorse or promote products derived from this software without specific 031 prior written permission. 032 033 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 034 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 035 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 036 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 037 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 038 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 039 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 040 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 041 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 042 POSSIBILITY OF SUCH DAMAGE. 043 044*/ 045// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 046public class Appointment40_50 { 047 048 public static org.hl7.fhir.r5.model.Appointment convertAppointment(org.hl7.fhir.r4.model.Appointment src) throws FHIRException { 049 if (src == null) 050 return null; 051 org.hl7.fhir.r5.model.Appointment tgt = new org.hl7.fhir.r5.model.Appointment(); 052 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 053 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 054 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 055 if (src.hasStatus()) 056 tgt.setStatusElement(convertAppointmentStatus(src.getStatusElement())); 057 if (src.hasCancelationReason()) 058 tgt.setCancellationReason(CodeableConcept40_50.convertCodeableConcept(src.getCancelationReason())); 059 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getServiceCategory()) 060 tgt.addServiceCategory(CodeableConcept40_50.convertCodeableConcept(t)); 061 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getServiceType()) 062 tgt.addServiceType().setConcept(CodeableConcept40_50.convertCodeableConcept(t)); 063 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getSpecialty()) 064 tgt.addSpecialty(CodeableConcept40_50.convertCodeableConcept(t)); 065 if (src.hasAppointmentType()) 066 tgt.setAppointmentType(CodeableConcept40_50.convertCodeableConcept(src.getAppointmentType())); 067 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getReasonCode()) 068 tgt.addReason(CodeableConcept40_50.convertCodeableConceptToCodeableReference(t)); 069 for (org.hl7.fhir.r4.model.Reference t : src.getReasonReference()) 070 tgt.addReason(Reference40_50.convertReferenceToCodeableReference(t)); 071 if (src.hasPriority()) 072 tgt.setPriority(convertAppointmentPriority(src.getPriorityElement())); 073 if (src.hasDescription()) 074 tgt.setDescriptionElement(String40_50.convertString(src.getDescriptionElement())); 075 for (org.hl7.fhir.r4.model.Reference t : src.getSupportingInformation()) 076 tgt.addSupportingInformation(Reference40_50.convertReference(t)); 077 if (src.hasStart()) 078 tgt.setStartElement(Instant40_50.convertInstant(src.getStartElement())); 079 if (src.hasEnd()) 080 tgt.setEndElement(Instant40_50.convertInstant(src.getEndElement())); 081 if (src.hasMinutesDuration()) 082 tgt.setMinutesDurationElement(PositiveInt40_50.convertPositiveInt(src.getMinutesDurationElement())); 083 for (org.hl7.fhir.r4.model.Reference t : src.getSlot()) tgt.addSlot(Reference40_50.convertReference(t)); 084 if (src.hasCreated()) 085 tgt.setCreatedElement(DateTime40_50.convertDateTime(src.getCreatedElement())); 086 if (src.hasComment()) 087 tgt.getNoteFirstRep().setTextElement(MarkDown40_50.convertStringToMarkdown(src.getCommentElement())); 088// if (src.hasPatientInstruction()) 089// tgt.setPatientInstructionElement(String40_50.convertString(src.getPatientInstructionElement())); 090 for (org.hl7.fhir.r4.model.Reference t : src.getBasedOn()) tgt.addBasedOn(Reference40_50.convertReference(t)); 091 for (org.hl7.fhir.r4.model.Appointment.AppointmentParticipantComponent t : src.getParticipant()) 092 tgt.addParticipant(convertAppointmentParticipantComponent(t)); 093 for (org.hl7.fhir.r4.model.Period t : src.getRequestedPeriod()) 094 tgt.addRequestedPeriod(Period40_50.convertPeriod(t)); 095 return tgt; 096 } 097 098 public static org.hl7.fhir.r4.model.Appointment convertAppointment(org.hl7.fhir.r5.model.Appointment src) throws FHIRException { 099 if (src == null) 100 return null; 101 org.hl7.fhir.r4.model.Appointment tgt = new org.hl7.fhir.r4.model.Appointment(); 102 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 103 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 104 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 105 if (src.hasStatus()) 106 tgt.setStatusElement(convertAppointmentStatus(src.getStatusElement())); 107 if (src.hasCancellationReason()) 108 tgt.setCancelationReason(CodeableConcept40_50.convertCodeableConcept(src.getCancellationReason())); 109 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getServiceCategory()) 110 tgt.addServiceCategory(CodeableConcept40_50.convertCodeableConcept(t)); 111 for (org.hl7.fhir.r5.model.CodeableReference t : src.getServiceType()) 112 tgt.addServiceType(CodeableConcept40_50.convertCodeableConcept(t.getConcept())); 113 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getSpecialty()) 114 tgt.addSpecialty(CodeableConcept40_50.convertCodeableConcept(t)); 115 if (src.hasAppointmentType()) 116 tgt.setAppointmentType(CodeableConcept40_50.convertCodeableConcept(src.getAppointmentType())); 117 for (CodeableReference t : src.getReason()) 118 if (t.hasConcept()) 119 tgt.addReasonCode(CodeableConcept40_50.convertCodeableConcept(t.getConcept())); 120 for (CodeableReference t : src.getReason()) 121 if (t.hasReference()) 122 tgt.addReasonReference(Reference40_50.convertReference(t.getReference())); 123 if (src.hasPriority()) 124 tgt.setPriorityElement(convertAppointmentPriority(src.getPriority())); 125 if (src.hasDescription()) 126 tgt.setDescriptionElement(String40_50.convertString(src.getDescriptionElement())); 127 for (org.hl7.fhir.r5.model.Reference t : src.getSupportingInformation()) 128 tgt.addSupportingInformation(Reference40_50.convertReference(t)); 129 if (src.hasStart()) 130 tgt.setStartElement(Instant40_50.convertInstant(src.getStartElement())); 131 if (src.hasEnd()) 132 tgt.setEndElement(Instant40_50.convertInstant(src.getEndElement())); 133 if (src.hasMinutesDuration()) 134 tgt.setMinutesDurationElement(PositiveInt40_50.convertPositiveInt(src.getMinutesDurationElement())); 135 for (org.hl7.fhir.r5.model.Reference t : src.getSlot()) tgt.addSlot(Reference40_50.convertReference(t)); 136 if (src.hasCreated()) 137 tgt.setCreatedElement(DateTime40_50.convertDateTime(src.getCreatedElement())); 138 if (src.hasNote()) 139 tgt.setCommentElement(String40_50.convertString(src.getNoteFirstRep().getTextElement())); 140// if (src.hasPatientInstruction()) 141// tgt.setPatientInstructionElement(String40_50.convertString(src.getPatientInstructionElement())); 142 for (org.hl7.fhir.r5.model.Reference t : src.getBasedOn()) tgt.addBasedOn(Reference40_50.convertReference(t)); 143 for (org.hl7.fhir.r5.model.Appointment.AppointmentParticipantComponent t : src.getParticipant()) 144 tgt.addParticipant(convertAppointmentParticipantComponent(t)); 145 for (org.hl7.fhir.r5.model.Period t : src.getRequestedPeriod()) 146 tgt.addRequestedPeriod(Period40_50.convertPeriod(t)); 147 return tgt; 148 } 149 150 151 private static UnsignedIntType convertAppointmentPriority(CodeableConcept src) { 152 UnsignedIntType tgt = new UnsignedIntType(convertAppointmentPriorityFromR5(src)); 153 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 154 return tgt; 155 } 156 157 private static CodeableConcept convertAppointmentPriority(UnsignedIntType src) { 158 CodeableConcept tgt = src.hasValue() ? convertAppointmentPriorityToR5(src.getValue()) : new CodeableConcept(); 159 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 160 return tgt; 161 } 162 163 public static org.hl7.fhir.r5.model.CodeableConcept convertAppointmentPriorityToR5(int priority) { 164 return null; 165 } 166 167 public static int convertAppointmentPriorityFromR5(org.hl7.fhir.r5.model.CodeableConcept priority) { 168 return 0; 169 } 170 171 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Appointment.AppointmentStatus> convertAppointmentStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Appointment.AppointmentStatus> src) throws FHIRException { 172 if (src == null || src.isEmpty()) 173 return null; 174 Enumeration<Appointment.AppointmentStatus> tgt = new Enumeration<>(new Appointment.AppointmentStatusEnumFactory()); 175 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 176 if (src.getValue() == null) { 177 tgt.setValue(null); 178 } else { 179 switch (src.getValue()) { 180 case PROPOSED: 181 tgt.setValue(Appointment.AppointmentStatus.PROPOSED); 182 break; 183 case PENDING: 184 tgt.setValue(Appointment.AppointmentStatus.PENDING); 185 break; 186 case BOOKED: 187 tgt.setValue(Appointment.AppointmentStatus.BOOKED); 188 break; 189 case ARRIVED: 190 tgt.setValue(Appointment.AppointmentStatus.ARRIVED); 191 break; 192 case FULFILLED: 193 tgt.setValue(Appointment.AppointmentStatus.FULFILLED); 194 break; 195 case CANCELLED: 196 tgt.setValue(Appointment.AppointmentStatus.CANCELLED); 197 break; 198 case NOSHOW: 199 tgt.setValue(Appointment.AppointmentStatus.NOSHOW); 200 break; 201 case ENTEREDINERROR: 202 tgt.setValue(Appointment.AppointmentStatus.ENTEREDINERROR); 203 break; 204 case CHECKEDIN: 205 tgt.setValue(Appointment.AppointmentStatus.CHECKEDIN); 206 break; 207 case WAITLIST: 208 tgt.setValue(Appointment.AppointmentStatus.WAITLIST); 209 break; 210 default: 211 tgt.setValue(Appointment.AppointmentStatus.NULL); 212 break; 213 } 214 } 215 return tgt; 216 } 217 218 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Appointment.AppointmentStatus> convertAppointmentStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Appointment.AppointmentStatus> src) throws FHIRException { 219 if (src == null || src.isEmpty()) 220 return null; 221 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Appointment.AppointmentStatus> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Appointment.AppointmentStatusEnumFactory()); 222 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 223 if (src.getValue() == null) { 224 tgt.setValue(null); 225 } else { 226 switch (src.getValue()) { 227 case PROPOSED: 228 tgt.setValue(org.hl7.fhir.r4.model.Appointment.AppointmentStatus.PROPOSED); 229 break; 230 case PENDING: 231 tgt.setValue(org.hl7.fhir.r4.model.Appointment.AppointmentStatus.PENDING); 232 break; 233 case BOOKED: 234 tgt.setValue(org.hl7.fhir.r4.model.Appointment.AppointmentStatus.BOOKED); 235 break; 236 case ARRIVED: 237 tgt.setValue(org.hl7.fhir.r4.model.Appointment.AppointmentStatus.ARRIVED); 238 break; 239 case FULFILLED: 240 tgt.setValue(org.hl7.fhir.r4.model.Appointment.AppointmentStatus.FULFILLED); 241 break; 242 case CANCELLED: 243 tgt.setValue(org.hl7.fhir.r4.model.Appointment.AppointmentStatus.CANCELLED); 244 break; 245 case NOSHOW: 246 tgt.setValue(org.hl7.fhir.r4.model.Appointment.AppointmentStatus.NOSHOW); 247 break; 248 case ENTEREDINERROR: 249 tgt.setValue(org.hl7.fhir.r4.model.Appointment.AppointmentStatus.ENTEREDINERROR); 250 break; 251 case CHECKEDIN: 252 tgt.setValue(org.hl7.fhir.r4.model.Appointment.AppointmentStatus.CHECKEDIN); 253 break; 254 case WAITLIST: 255 tgt.setValue(org.hl7.fhir.r4.model.Appointment.AppointmentStatus.WAITLIST); 256 break; 257 default: 258 tgt.setValue(org.hl7.fhir.r4.model.Appointment.AppointmentStatus.NULL); 259 break; 260 } 261 } 262 return tgt; 263 } 264 265 public static org.hl7.fhir.r5.model.Appointment.AppointmentParticipantComponent convertAppointmentParticipantComponent(org.hl7.fhir.r4.model.Appointment.AppointmentParticipantComponent src) throws FHIRException { 266 if (src == null) 267 return null; 268 org.hl7.fhir.r5.model.Appointment.AppointmentParticipantComponent tgt = new org.hl7.fhir.r5.model.Appointment.AppointmentParticipantComponent(); 269 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 270 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getType()) 271 tgt.addType(CodeableConcept40_50.convertCodeableConcept(t)); 272 if (src.hasActor()) 273 tgt.setActor(Reference40_50.convertReference(src.getActor())); 274 if (src.hasRequired()) 275 tgt.setRequiredElement(convertParticipantRequired(src.getRequiredElement())); 276 if (src.hasStatus()) 277 tgt.setStatusElement(convertParticipationStatus(src.getStatusElement())); 278 if (src.hasPeriod()) 279 tgt.setPeriod(Period40_50.convertPeriod(src.getPeriod())); 280 return tgt; 281 } 282 283 public static org.hl7.fhir.r4.model.Appointment.AppointmentParticipantComponent convertAppointmentParticipantComponent(org.hl7.fhir.r5.model.Appointment.AppointmentParticipantComponent src) throws FHIRException { 284 if (src == null) 285 return null; 286 org.hl7.fhir.r4.model.Appointment.AppointmentParticipantComponent tgt = new org.hl7.fhir.r4.model.Appointment.AppointmentParticipantComponent(); 287 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 288 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getType()) 289 tgt.addType(CodeableConcept40_50.convertCodeableConcept(t)); 290 if (src.hasActor()) 291 tgt.setActor(Reference40_50.convertReference(src.getActor())); 292 if (src.hasRequired()) 293 tgt.setRequiredElement(convertParticipantRequired(src.getRequiredElement())); 294 if (src.hasStatus()) 295 tgt.setStatusElement(convertParticipationStatus(src.getStatusElement())); 296 if (src.hasPeriod()) 297 tgt.setPeriod(Period40_50.convertPeriod(src.getPeriod())); 298 return tgt; 299 } 300 301 static public org.hl7.fhir.r5.model.BooleanType convertParticipantRequired(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Appointment.ParticipantRequired> src) throws FHIRException { 302 if (src == null || src.isEmpty()) 303 return null; 304 BooleanType tgt = new BooleanType(); 305 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 306 if (src.getValue() == null) { 307 tgt.setValue(null); 308 } else { 309 switch (src.getValue()) { 310 case REQUIRED: 311 tgt.setValue(true); 312 break; 313 case OPTIONAL: 314 tgt.setValue(false); 315 break; 316 case INFORMATIONONLY: 317 tgt.setValue(false); 318 break; 319 default: 320 break; 321 } 322 } 323 return tgt; 324 } 325 326 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Appointment.ParticipantRequired> convertParticipantRequired(org.hl7.fhir.r5.model.BooleanType src) throws FHIRException { 327 if (src == null || src.isEmpty()) 328 return null; 329 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Appointment.ParticipantRequired> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Appointment.ParticipantRequiredEnumFactory()); 330 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 331 if (src.getValue()) { // case REQUIRED: 332 tgt.setValue(org.hl7.fhir.r4.model.Appointment.ParticipantRequired.REQUIRED); 333 } else { // case OPTIONAL + others: 334 tgt.setValue(org.hl7.fhir.r4.model.Appointment.ParticipantRequired.OPTIONAL); 335 } 336 return tgt; 337 } 338 339 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Appointment.ParticipationStatus> convertParticipationStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Appointment.ParticipationStatus> src) throws FHIRException { 340 if (src == null || src.isEmpty()) 341 return null; 342 Enumeration<Appointment.ParticipationStatus> tgt = new Enumeration<>(new Appointment.ParticipationStatusEnumFactory()); 343 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 344 if (src.getValue() == null) { 345 tgt.setValue(null); 346 } else { 347 switch (src.getValue()) { 348 case ACCEPTED: 349 tgt.setValue(Appointment.ParticipationStatus.ACCEPTED); 350 break; 351 case DECLINED: 352 tgt.setValue(Appointment.ParticipationStatus.DECLINED); 353 break; 354 case TENTATIVE: 355 tgt.setValue(Appointment.ParticipationStatus.TENTATIVE); 356 break; 357 case NEEDSACTION: 358 tgt.setValue(Appointment.ParticipationStatus.NEEDSACTION); 359 break; 360 default: 361 tgt.setValue(Appointment.ParticipationStatus.NULL); 362 break; 363 } 364 } 365 return tgt; 366 } 367 368 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Appointment.ParticipationStatus> convertParticipationStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Appointment.ParticipationStatus> src) throws FHIRException { 369 if (src == null || src.isEmpty()) 370 return null; 371 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Appointment.ParticipationStatus> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Appointment.ParticipationStatusEnumFactory()); 372 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 373 if (src.getValue() == null) { 374 tgt.setValue(null); 375 } else { 376 switch (src.getValue()) { 377 case ACCEPTED: 378 tgt.setValue(org.hl7.fhir.r4.model.Appointment.ParticipationStatus.ACCEPTED); 379 break; 380 case DECLINED: 381 tgt.setValue(org.hl7.fhir.r4.model.Appointment.ParticipationStatus.DECLINED); 382 break; 383 case TENTATIVE: 384 tgt.setValue(org.hl7.fhir.r4.model.Appointment.ParticipationStatus.TENTATIVE); 385 break; 386 case NEEDSACTION: 387 tgt.setValue(org.hl7.fhir.r4.model.Appointment.ParticipationStatus.NEEDSACTION); 388 break; 389 default: 390 tgt.setValue(org.hl7.fhir.r4.model.Appointment.ParticipationStatus.NULL); 391 break; 392 } 393 } 394 return tgt; 395 } 396}