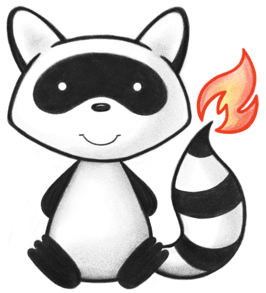
001package org.hl7.fhir.convertors.conv40_50.resources40_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext40_50; 004import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.CodeableConcept40_50; 005import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Identifier40_50; 006import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Date40_50; 007import org.hl7.fhir.convertors.conv40_50.datatypes40_50.special40_50.Reference40_50; 008import org.hl7.fhir.exceptions.FHIRException; 009 010/* 011 Copyright (c) 2011+, HL7, Inc. 012 All rights reserved. 013 014 Redistribution and use in source and binary forms, with or without modification, 015 are permitted provided that the following conditions are met: 016 017 * Redistributions of source code must retain the above copyright notice, this 018 list of conditions and the following disclaimer. 019 * Redistributions in binary form must reproduce the above copyright notice, 020 this list of conditions and the following disclaimer in the documentation 021 and/or other materials provided with the distribution. 022 * Neither the name of HL7 nor the names of its contributors may be used to 023 endorse or promote products derived from this software without specific 024 prior written permission. 025 026 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 027 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 028 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 029 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 030 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 031 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 032 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 033 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 034 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 035 POSSIBILITY OF SUCH DAMAGE. 036 037*/ 038// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 039public class Basic40_50 { 040 041 public static org.hl7.fhir.r5.model.Basic convertBasic(org.hl7.fhir.r4.model.Basic src) throws FHIRException { 042 if (src == null) 043 return null; 044 org.hl7.fhir.r5.model.Basic tgt = new org.hl7.fhir.r5.model.Basic(); 045 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 046 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 047 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 048 if (src.hasCode()) 049 tgt.setCode(CodeableConcept40_50.convertCodeableConcept(src.getCode())); 050 if (src.hasSubject()) 051 tgt.setSubject(Reference40_50.convertReference(src.getSubject())); 052 if (src.hasCreated()) 053 tgt.setCreatedElement(Date40_50.convertDatetoDateTime(src.getCreatedElement())); 054 if (src.hasAuthor()) 055 tgt.setAuthor(Reference40_50.convertReference(src.getAuthor())); 056 return tgt; 057 } 058 059 public static org.hl7.fhir.r4.model.Basic convertBasic(org.hl7.fhir.r5.model.Basic src) throws FHIRException { 060 if (src == null) 061 return null; 062 org.hl7.fhir.r4.model.Basic tgt = new org.hl7.fhir.r4.model.Basic(); 063 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 064 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 065 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 066 if (src.hasCode()) 067 tgt.setCode(CodeableConcept40_50.convertCodeableConcept(src.getCode())); 068 if (src.hasSubject()) 069 tgt.setSubject(Reference40_50.convertReference(src.getSubject())); 070 if (src.hasCreated()) 071 tgt.setCreatedElement(Date40_50.convertDateTimeToDate(src.getCreatedElement())); 072 if (src.hasAuthor()) 073 tgt.setAuthor(Reference40_50.convertReference(src.getAuthor())); 074 return tgt; 075 } 076}